C++ class methods and constructors are two crucial aspects of object-oriented programming. These elements help you not only create classes in C++ but also execute functions on various related objects within your project.
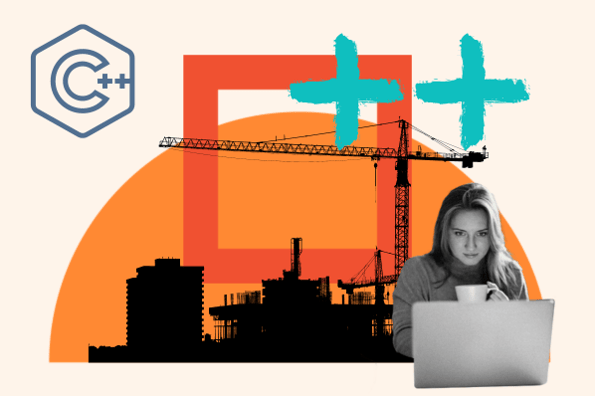
In this post, we'll explore everything you need to know about these components, including how they function and how to use them efficiently. We'll begin by explaining what C++ class methods are and what they do, then we'll wrap up by diving into constructors and reviewing how they're used alongside class methods.
What are C++ class methods?
Class methods – also known as member functions – are functions defined inside a class that operates on the class objects. They access the class's data members and other member functions and define the behavior or actions that objects of the class can perform.
Class methods are declared and defined within the class definition itself or defined outside the class definition using the scope resolution operator (::) to specify the class to which they belong.
Here’s one use case for class methods in C++:class Rectangle {
private:
double width;
double height;
public:
void setDimensions(double w, double h) {
width = w;
height = h;
}
double calculateArea() {
return width * height;
}
void printDimensions() {
std::cout << "Width: " << width << ", Height: " << height << std::endl;
}
};
int main() {
Rectangle rectangle1;
rectangle1.setDimensions(4.5, 3.2);
double area = rectangle1.calculateArea();
std::cout << "Rectangle Area: " << area << std::endl;
rectangle1.printDimensions();
return 0;
}
Output:
Rectangle Area: 14.4
Width: 4.5, Height: 3.2
In this example, the Rectangle class has three member functions: setDimensions, calculateArea, and printDimensions.
- setDimensions: sets the width and height of the rectangle object
- calculateArea: calculates the area of the rectangle based on its width and height
- printDimensions: prints the width and height of the rectangle
Inside the main function, we created an object called rectangle1 from the Rectangle class. We then used its member functions to set its dimensions, calculate its area, and print its dimensions.
Class methods access the object's data members using the dot (.) operator. In this example, we pulled the width and height of the rectangle and used that information to calculate its area.
What are C++ constructors?
Constructors are special member functions of a class that are used for initializing objects of that class. Constructors are automatically called when an object of the class is created. They're responsible for setting up the initial state of the object by initializing its data members.
Here’s an example of a constructor being used in C++:class Rectangle {
private:
double width;
double height;
public:
// Parameterized constructor
Rectangle(double w, double h) {
width = w;
height = h;
}
// Default constructor (provided by the compiler)
}
Rectangle() {
width = 0.0;
height = 0.0;
}
double calculateArea() {
return width * height;
}
};
int main() {
Rectangle rectangle1(4.5, 3.2); // Using the parameterized constructor
double area = rectangle1.calculateArea();
std::cout << "Rectangle Area: " << area << std::endl;
Rectangle rectangle2; // Using the default constructor
area = rectangle2.calculateArea();
std::cout << "Rectangle Area (Default): " << area << std::endl;
return 0;
}
Output:
Rectangle Area: 14.4
Rectangle Area (Default): 0
Using Class Methods and Constructors With C++ Functions
Classes and constructors are two of the most essential components of object-oriented programming. They're crucial building blocks that you'll need to be familiar with when developing C++ applications.
Using these fundamental concepts as your foundation, you'll be able to create more efficient and powerful code while taking your C++ programming skills to the next level.
Author's Note: This post was written/edited by a human with the assistance of generative AI.