“The only thing that is constant is change” is something you may have heard before. However, some things truly are consistently the same.
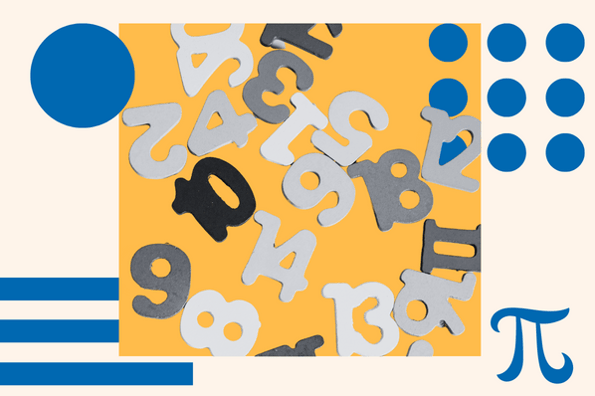
Things like the speed of light (299,792,458 meters per second), the constant of a circle pi (~3.14), or the golden ratio (1.61803) are all mathematical constants. They’re present in everyday life whether you know it or not. The same is true in JavaScript.
In this article, you will learn about the const data type in JavaScript and go over a few basic examples and quirks.
What are constants in JavaScript?
It might be pretty obvious, but just to make it abundantly clear, const in JavaScript is short for “constant.” In short, const is a variable that cannot be changed once declared.
Seems simple enough, right? There are a few quirks about constants when compared to regular variables. You’ll learn about them shortly. Let’s see it in action.
How to Use Constants in JavaScript
Below, you’ll see the basic syntax for declaring a constant in JavaScript. The value doesn’t necessarily have to be a number. It can pretty much be anything you want. Just remember, once it’s defined, it can’t be changed.
Now, if you try to change this newly created pi variable, the console will yell at you saying it can’t do that. See below for an example of this happening.
Makes sense, right? If you could change this, it wouldn’t be constant anymore and instead would be the same as any regular old variable.
Different from regular variables, you can’t declare a constant without giving it a value in the declaration statement. Again, let’s see an example of what happens if you try to do exactly that.
Before moving on, there is one more thing you should be aware of. Remember when I mentioned that you can store any value in a const variable? Well, that includes objects, and objects are mutable, which means they can be changed.
Knowing that, what do you think will happen if you store an object in a const and then attempt to overwrite the object?
Turns out, you can’t do that, or you’ll run into the same sort of TypeError that happened earlier. This is what you would expect to happen, knowing that constants are not supposed to change. However, what do you think will happen if we try to change a value in the object itself?
Uh oh! It looks like you can change values in the object itself, even after assigning it to a const variable. To prevent this, you could use the Object.freeze() method on the object after creating it.
As you can see, the object remained the same after attempting to change it once frozen. This is probably the best way to make sure it truly stays constant.
You could even declare it, freeze it, and store it in a const variable all on the same line. This ensures it’s obvious that the object is never meant to change.
That's nice and compact! It’s worth mentioning that all of this applies to arrays too. They’re just another kind of object, so even the Object.freeze() method will work the same with those.
Examples of Constants in JavaScript
Let’s see a few examples of constants in code. Imagine you were given the radius of a circle. Could you write code using the constant pi to find the circumference?
That wasn’t too bad, was it? The size of a circle can often change, but pi will always be a constant. So, using a const data type makes sense here.
Here is another example using my name. It also shows that you can use strings in constants, which we haven’t done anywhere before in this article.
I would’ve suggested changing the myName variable here, but we can’t do that with a const. Using const here doesn’t make sense since names are often changeable, but it suffices for an example with a string.
Getting Started
Constants in JavaScript are straightforward, apart from a few quirks when talking about objects. You learned about the basic syntax, a few common errors, and some details regarding the behavior of objects in a const variable. You also saw a few basic examples to really drive the point home on how they could be used in code.