Operators are a fundamental part of many programming languages — and JavaScript is no exception. Operators are used in conjunction with functions, variables, and objects to execute different tasks.
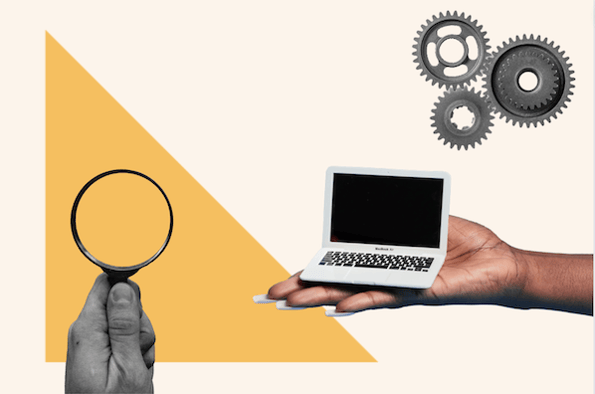
If you’re learning JavaScript, you‘ll want to familiarize yourself with these operators and how you can make the best use of them. That’s why we've put together this list, of JavaScript along with some examples of each that you can test out on your own.
There are several kinds of operators in JavaScript, including:
We’ll cover each of these categories below.
JavaScript Arithmetic Operators
Arithmetic operators perform mathematical operations on values or variables.
Addition (+)
The addition operator sums two numbers.
See the Pen JS operators: addition by HubSpot (@hubspot) on CodePen.
It can also combine two strings, like so:
See the Pen JS operators: addition (strings) by HubSpot (@hubspot) on CodePen.
Subtraction (-)
The subtraction operator subtracts one number from another.
See the Pen JS operators: subtraction by HubSpot (@hubspot) on CodePen.
Multiplication (*)
The multiplication operator multiplies two numbers.
See the Pen JS operators: multiplication by HubSpot (@hubspot) on CodePen.
Division (/)
The division operator divides one number by another.
See the Pen JS operators: division by HubSpot (@hubspot) on CodePen.
Modulus (%)
The modulus operator assigns the remainder value of a division equation. For example, 10 divided by 3 produces a remainder of 1. Therefore, the modulus value is 1.
See the Pen JS operators: modulus by HubSpot (@hubspot) on CodePen.
Exponentiation (**)
The exponentiation operator raises the first value to the power of the second value
See the Pen JS operators: exponent by HubSpot (@hubspot) on CodePen.
Incrementation (++)
The incrementation operator adds one to the value of a variable. If the operator is placed before the variable, it returns the value after the incrementation. If the operator is placed after the variable, it returns the value before the incrementation.
See the Pen JS operators: increment by HubSpot (@hubspot) on CodePen.
Decrementation (--)
The decrementation operator subtracts one from the value of a variable. The same placement rules as incrementation apply.
See the Pen JS operators: decrement by HubSpot (@hubspot) on CodePen.
JavaScript Assignment Operators
Assignment operators assign values to variables, by either giving the variable a new value or modifying its existing value in some way.
Assignment (=)
The assignment operator assigns a value to a variable.
See the Pen JS operators: assignment by HubSpot (@hubspot) on CodePen.
Addition Assignment (+=)
The addition assignment operator increments the value of a variable by a specified number.
See the Pen JS operators: add assignment by HubSpot (@hubspot) on CodePen.
This operator also works with combining strings:
See the Pen JS operators: add assignment (string) by HubSpot (@hubspot) on CodePen.
Subtraction Assignment (-=)
The subtraction assignment operator subtracts a specified number from the value of a variable.
See the Pen JS operators: subtract assignment by HubSpot (@hubspot) on CodePen.
Multiplication Assignment (*=)
The multiplication assignment operator multiplies the value of a variable by a specified number.
See the Pen JS operators: multiply assignment by HubSpot (@hubspot) on CodePen.
Division Assignment (/=)
The division assignment operator divides the value of a variable by a specified number.
See the Pen JS operators: division assignment by HubSpot (@hubspot) on CodePen.
Modulus Assignment (%=)
The modulus assignment operator returns the remainder of a variable divided by a specified number.
See the Pen JS operators: modulus assignment by HubSpot (@hubspot) on CodePen.
Exponent Assignment (**=)
The modulus assignment operator returns the value of a variable, raised to the power of a specified number.
See the Pen JS operators: exponent assignment by HubSpot (@hubspot) on CodePen.
JavaScript Comparison Operators
Comparison operators are used to check the difference between two values or variables. They’re usually used in logical statements to produce a boolean value.
Equal To (==)
The equal to operator checks whether two variables are equal. It returns true if the values are equal and false if the values are not equal.
See the Pen JS operators: equal to by HubSpot (@hubspot) on CodePen.
Not Equal To (!=)
The not equal to operator is the opposite of equal to — it returns true if two values are not equal and false if two values are equal.
See the Pen JS operators: not equal to by HubSpot (@hubspot) on CodePen.
Equal To and Same Type (===)
The not equal to and same type operator checks that (1) the two variables have equal values and (2) the two variables are of the same data type (number, string, boolean, etc.). If both of these conditions are true, it returns true. If one or both conditions are false, it returns false.
See the Pen JS operators: equal to and same type by HubSpot (@hubspot) on CodePen.
In the example above, variable c is false because, even though the numerical values of variables a and c are both 5, a is a number and c is a string.
Not Equal To or Not SameType (!==)
The not equal or not same type operator returns true if (1) the two variables have different values or (2) the two variables have different types. If the two variables have the same value and type, it returns false.
See the Pen JS operators: not equal to or not same type by HubSpot (@hubspot) on CodePen.
Greater Than (>)
The greater than operator returns true if the first variable is greater in value than the second, and false if not.
See the Pen JS operators: greater than by HubSpot (@hubspot) on CodePen.
Greater Than or Equal To (>=)
The greater than operator returns true if the first variable is greater than or equal in value to the second, and false if not.
See the Pen JS operators: greater than or equal to by HubSpot (@hubspot) on CodePen.
Less Than (<)
The less than operator returns true if the first variable is smaller in value than the second, and false if not.
See the Pen JS operators: less than by HubSpot (@hubspot) on CodePen.
Less Than or Equal To (<=)
The less than operator returns true if the first variable is smaller or equal in value to the second, and false if not.
See the Pen JS operators: less than or equal to by HubSpot (@hubspot) on CodePen.
JavaScript Logical Operators
Logical operators are used to string together multiple logical statements into a larger statement.
And (&&)
The and operator combines two or more logical statements in JavaScript. All statements must be true for the entire statement to return true.
See the Pen JS operators: and by HubSpot (@hubspot) on CodePen.
In the example above, a is true because both logical statements are true, and b is false because the second logical statement (4 < 3) is false.
Or (||)
The or operator also combines two or more logical statements, but only one statement has to be true in order for the entire statement to be true.
See the Pen JS operators: and by HubSpot (@hubspot) on CodePen.
In the above example, a and b return true because at least one of the statements is true. However, c returns false because neither statement is true.
Not (!)
The not operator reverses the boolean output of a logical statement. If a logical statement would return true, placing the not operator before it will make it return false, and vice versa.
See the Pen JS operators: not by HubSpot (@hubspot) on CodePen.