Any JavaScript programmer, beginner or expert, knows that if some task takes many lines of code, there’s a good chance that a built-in method exists that makes things much easier.
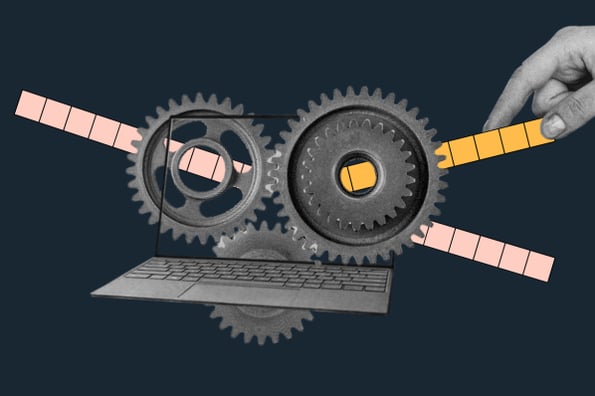
In this post, we’ll be talking about one of those methods, the JavaScript map() method. map() is a method of the array prototype and can be used to create new arrays based on existing arrays without having to deal with loops.
I’ll explain what the map() method is, what it does, and how to write it, then we’ll end with some examples of the method in action.
What is map() in JavaScript?
In JavaScript, map() is a method of the Array object. It creates a new array by calling a function on every element of the original array and storing the results in a new array. map() returns the new array, and the original array is unchanged.
JavaScript map() Syntax
The map() method is written as follows:
Let’s break this down:
- arrayName is the array that the map() method is being called on.
- function (required) is the callback function applied to each element in arrayName. It can be a named function or an anonymous function.
- currElement (required) is the current element in the array arrayName. Function must take currElement as an argument.
- currElementIndex (optional) is the index of the current element in arrayName.
- currElementArr (optional) is the array object of the current element.
- this (optional) is the value of the this operator in function. If not defined, the value is undefined.
arrayName.map() will return a new array, in which each element is the output of function with the input currElement.
So, how is this method actually used? Say I have an array of integers, and I want to add 1 to each integer in the array. This is possible with a loop:
See the Pen js map(): for loop by HubSpot (@hubspot) on CodePen.
Here, I’ve written a for loop that iterates through each element in oldArray, adds 1 to the current element, and then makes this integer an element in newArray.
Now look at the example below, which uses map() to produce the same outcome.
See the Pen js map(): map, anonymous by HubSpot (@hubspot) on CodePen.
In this example, the map() method is used on the array oldArray to create a new array of oldArray’s values plus one. This new array is then assigned the variable newArray.
I can also write this same function more efficiently with an arrow function. Here’s the same example as above but written with an arrow function:
See the Pen js map(): map, arrow by HubSpot (@hubspot) on CodePen.
Lastly, I can also use map() with a named function. Here, I have a function addOne() that is called with the map() method.
See the Pen js map(): map, named by HubSpot (@hubspot) on CodePen.
You may have noticed that map() behaves similarly to the JavaScript forEach() method. The difference is that map() creates a new array without affecting the old array, whereas forEach() alters the old array and does not create a new array. You should only use map() if you want a new array created.
Also note that if an array contains empty elements, map() ignores these elements:
See the Pen js map(): map, empty elements by HubSpot (@hubspot) on CodePen.
JavaScript map() Examples
Let’s look at some use cases for the map() method. As covered before, map() is great for carrying out mathematical functions on all elements of an array and creating a new array with the results.
This example uses map() to iterate through an array of decimals and converts them to percentage strings:
See the Pen js map(): percentage example by HubSpot (@hubspot) on CodePen.
We can also use map() to alter an array of objects. This example uses map to take in an array of objects and produce a new array containing strings with the object values combined:
See the Pen js map(): objects example by HubSpot (@hubspot) on CodePen.
To show how the second callback function argument (currElementIndex, which is the index of the current element in the original array), the function in the example below only runs on elements with an even index:
See the Pen js map(): second argument example by HubSpot (@hubspot) on CodePen.
Leverage map() in your JavaScript code.
There’s nothing particularly fancy about map() — it’s another method you can implement in your code to save yourself time and file size. And, if you find yourself frequently duplicating arrays in your code, it’s indispensable.