Have you ever written JavaScript code that didn't work as expected? You may have defined a variable or function in one part of your code, but it didn't properly work when you tried to use it elsewhere. This is because JavaScript has something called scope.
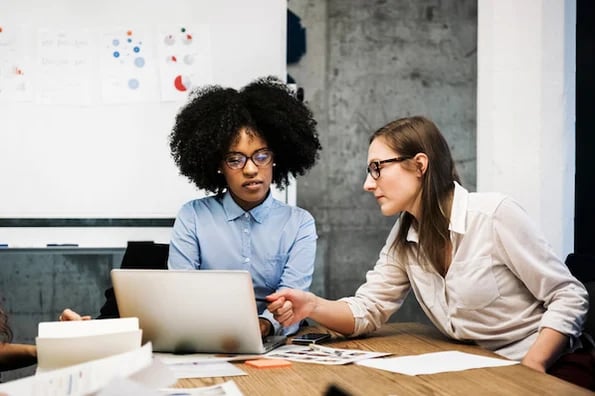
In this blog post, we'll explore scope and how to use it to your advantage when writing code. We'll also look at some common scoping issues and how to solve them. Let's get started!
What is JavaScript scope?
In JavaScript, scope refers to the current context of your code. This context determines where you can access certain variables and functions. In other words, where you decide to define a variable or function in JavaScript impacts where you have access to it later on. So, if you define a variable inside a function, you will only be able to access it inside that function.
There are several types of scope in JavaScript: global scope, local scope, function scope, block scope, and lexical scope. We'll explore each of these in more depth below.
Global Scope
Global scope means that a variable or function is available anywhere in your code. This is the default scope for variables and functions in JavaScript.
Let's take a look at an example:
In the code snippet above, we have defined a variable called 'pet' with a value of 'dog'. We have also defined a function called 'printPet', which prints the value of 'pet' to the console.
Because we have defined 'pet' in the global scope, we can access it inside the 'printPet' function. If we try to access 'pet' outside of the function, it will still be available:
However, if we try to access a variable that is not in the global scope, we will get an error:
In the code snippet above, we have defined a variable called 'pet' with a value of 'cat'. We have also defined a function called 'printPet', which prints the value of 'pet' to the console.
Because we have not defined 'otherPet' in the global scope, we cannot access it outside of the 'printPet' function. If we try to access it inside the function, it will still be available:
Local Scope
Local scope means that a variable or function is only available in the current code block. To create a local scope, we can use curly braces:
In the code snippet above, we have defined a variable called 'pet' with a value of 'cat'. We have also used curly braces to create a local scope for the variable.
Because 'pet' is in the local scope, we cannot access it outside the curly braces. If we try to access it inside the scope, it will still be available:
Function Scope
Function scope is similar to a local scope in that variables and functions defined inside a function are only available inside that function. However, there is one key difference: variables and functions defined inside a function are not available in the global scope.
Let's take a look at an example:
In the code snippet above, we have defined a function called 'printPet'. We have also defined a variable called 'pet' with a value of 'cat'.
Because we have defined 'pet' inside the 'printPet' function, we can only access it inside that function. If we try to access 'pet' outside of the function, we will get an error:
Block Scope
Block scope allows us to create variables and functions only available inside a code block. A code block is any time you use curly braces, for example:
To create a block scope, we can use the 'let' or 'const' keywords:
In the code snippet above, we have defined a variable called 'pet' with a value of 'cat'. We have also used the 'let' keyword to create a block scope for the variable inside an 'if' statement.
Because we have defined 'pet' using the 'let' keyword, we can access it inside the code block. However, 'pet' is not available in the global scope. Therefore, if we try to access 'pet' outside of the code block, we will get an error:
Note: A block scope does not create a scope for var variables.
Lexical Scope
Lexical scope can be a bit more complicated than the other types of scope. It is sometimes also called static scope or compile-time scope.
Lexical scope means that the scope of a variable is determined by its position in the code. In other words, variables are available in the same scope as their parent variables.
For example:
In the code snippet above, we have defined a variable called 'pet' with a value of 'cat'. We have also defined a function called 'printPet'.
Because we have defined the 'pet' variable in the global scope, it is available in the 'printPet' function. However, if we try to access 'pet' inside a code block, it will not be available:
In the code snippet above, we have defined a variable called 'pet' with a value of 'cat'. We have also used the 'var' keyword to create a block scope for the variable inside an 'if' statement.
Because we have defined 'pet' using the 'var' keyword, it is not available in the global scope. Therefore, if we try to access 'pet' outside of the code block, we will get an error:
Wrapping Up JavaScript Scope
Scope is an essential concept in JavaScript that determines where variables and functions are available. Const and let variables follow the same scoping rules regardless of whether they are in a code block, function, or module. On the other hand, var variables only follow these scoping rules when they are inside a function or module.
In JavaScript, using scope correctly offers benefits in terms of security and code reuse. By reducing namespace collisions, you can make your code more secure and use it again in other projects.
Now that you understand the basics of scope, you can start using it to write better and more maintainable code.