Are you tired of writing tedious and repetitive code for your JavaScript projects? If so, you'll be pleased to learn about the power of the JavaScript unshift() method.
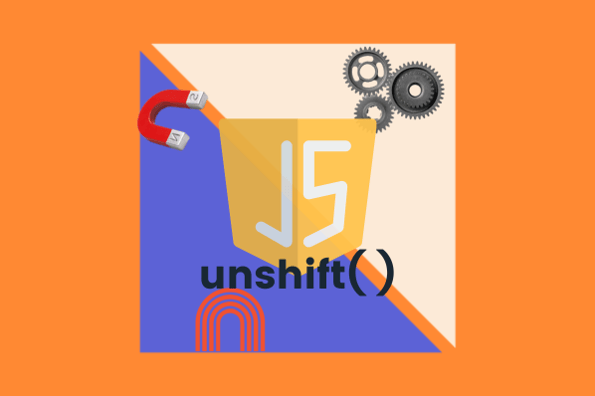
The unshift() method is an important function to learn for working with arrays in JavaScript. Using the unshift() method, you can easily manipulate arrays without worrying about the array's size or the location of the elements. This method can help you streamline your code and make your projects more efficient.
In this article, we'll take an in-depth look at the unshift() method in JavaScript. We'll cover the syntax and usage of the method and provide some examples of how it can be used in real-world applications. We'll also compare the unshift() method to other array methods and provide some tips and tricks for using it effectively. By the end of this article, you'll be well-versed in the power of the unshift() method and how it can revolutionize your code. So, let's get started!
What is JavaScript unshift() method?
What is JavaScript unshift() method?
The unshift() method is a built-in function in JavaScript that allows you to add one or more elements to the beginning of an array. This method can greatly simplify your code, making it more efficient and easier to read.
Before diving into the usage and examples of the unshift() method, let's first examine its syntax. The unshift() method is called on an array and takes one or more parameters representing the elements to be added to the beginning of the array.
The syntax of the unshift() method is as follows:
The unshift() method can take any number of elements as parameters, separated by commas. Each element will be added to the beginning of the array, in the order they are listed as parameters. The unshift() method modifies the original array and returns the new length of the array.
Let's take a closer look at the parameters of the unshift() method:
- element1, element2, ... elementN: These are the elements to be added to the beginning of the array. They can be of any data type, including strings, numbers, objects, or other arrays.
Now that we've covered the syntax of the unshift() method let's move on to its usage and examples.
How to use JavaScript unshift() method?
The unshift() method is a powerful tool for manipulating arrays in JavaScript. Using the unshift() method is simple. All you need to do is call the method on an array and pass in one or more parameters representing the elements to be added to the beginning of the array.
Here are some examples of how to use the unshift() method:
Adding elements to the beginning of an array:
In this example, we use the unshift() method to add the number 0 to the beginning of our array by calling the unshift() method on our array and passing in 0 as a parameter.
Adding multiple elements to the beginning of an array:
The same can be done with elements of any data type, including strings, objects, and other arrays. In this example, we use the unshift() method to add two elements - "fish" and "hamster" - to the beginning of our array.
Combining the unshift() and push() methods to add elements:
In this example, we use both the unshift() and push() methods to add elements to the beginning and end of our array. The unshift() method adds "fish" to the beginning of the array, while the push() method adds "hamster" to the end of the array.
When to use JavaScript unshift() method?
The unshift() method can be used in various real-world applications. Here are some examples:
- Building a Todo List app: Use the unshift() method to add new items to the beginning of the list as they are created.
- Creating a dynamic carousel: Use the unshift() method to add new images to the beginning of the carousel as they are uploaded.
- Enhancing the user experience with unshift(): Use the unshift() method to add recently viewed items to the beginning of a list, allowing users to access them quickly.
Differences Between unshift() and Other Array Methods
While the unshift() method is useful for adding elements to the beginning of an array, it's important to understand how it differs from other array methods. Here are some key differences between unshift() and other methods:
unshift() vs push():
The push() method adds elements to the end of an array, while unshift() adds them to the beginning. Additionally, push() returns the length of the array after adding elements, while unshift() does not.
unshift() vs splice():
The splice() method can add or remove elements from an array at any position, while unshift() can only add elements to the beginning of the array.
unshift() vs concat():
The concat() method creates a new array by combining two or more arrays, while unshift() modifies the original array by adding elements to the beginning.
Tips for Using the unshift() Method in JavaScript
To use the unshift() method effectively, here are some tips and tricks to keep in mind:
- Avoid using unshift() with large arrays, which can be slow and inefficient.
- If you need to add multiple elements to an array using unshift(), consider combining them into a new array first.
- When using unshift() in a loop, be careful not to create an infinite loop.
Unlock the Full Potential of Your Code With the JavaScript unshift() Method
Incorporating the unshift() method into your JavaScript code can streamline your programming, making it more efficient and adding elegance to your projects.
In this article, we've explored the power of the JavaScript unshift() method. We've covered its syntax, usage, and examples and compared it to other array methods. By understanding the unshift() method and its various applications, you can greatly simplify your code and make your projects more efficient. Remember to keep the tips and tricks we've discussed in mind, and explore how you can use the unshift() method in your own applications.
So why not try out the power of unshift() for yourself and revolutionize your code today?