Node.js is a popular JavaScript framework for back-end development, so there’s a high demand for developers who know how to use it. If you're preparing for an upcoming interview for such a position, preparation is critical.
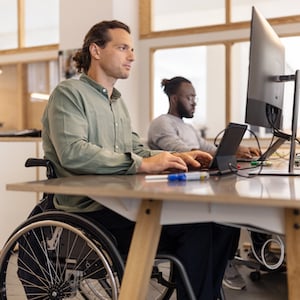
Here are 20 of the most common interview questions on Node.js and some insights on preparing for them.
Top 20 Node.js Interview Questions
Application Components
Your interview will likely start with an introductory exploration of Node.js applications. You should be ready to describe the most common components and how to structure, add to, and export your application.
These questions are the easiest to answer, even without production experience. Get comfortable with the file structures and command line tools you’ll use daily by experimenting.
What Is the Function of Modules in Node.js?
Modules are related functions imported into your code, typically as a single file. Think of them like libraries for JavaScript and other languages. You can group modules into packages for specific projects.
What Is the Role of the package.json File in a Node.js App?
The package.json file lives in a package’s root directory and holds all the metadata required to build it. The metadata includes references to all your package’s modules and dependencies, including their version numbers.
How Do You Manage Packages for a Node.js Project?
You can install or update packages using a package manager. Node.js package managers typically include a registry of packages and a CLI tool to install and manage their versions and dependencies. The most common is npm, but you can use others, such as Yarn or pnpm.
Applied Knowledge
Node.js is quickly becoming an industry standard, so you must understand how it fits into the rest of the commercial pipeline.
For these questions, you should be ready to explain why your team may consider using Node.js versus other tools. Node.js is great for some applications but can introduce significant problems in the wrong context. To address these shortcomings, developers create and use various frameworks. Although this list is long and ever-changing, you should know at least half a dozen popular frameworks and what they offer.
Node.js also provides features you’re likely to use in any production-ready application. Be prepared to talk about any you have implemented before and how. Otherwise, describe their role and compare them to similar features in other applications.
What Are the Most Popular Node.js Web Application Frameworks?
The most popular Node.js framework is the Express.js library. Other frameworks worth mentioning include Koa, Sails.js, Meteor, Fastify, Next.js, and Socket.IO.
What Are the Most Common Use Cases for Node.js?
You commonly use Node.js for data-sensitive or real-time applications that handle large volumes of requests. The most common examples include:
- Real-time chat and collaboration tools
- Streaming
- Microservice architecture
- Internet of things (IoT)
- Single-page applications (SPAs)
What Are Some Reasons for Choosing Node.js as a Back End over PHP or Another Language?
The Node.js asynchronous model is faster and more stable for applications with many concurrent requests than most other languages. Node.js also lets you develop your server and client sides using the same codebase simultaneously. It also uses JavaScript for front and back-end development, making it easier for new developers.
What Is the Role of the NODE_ENV Variable?
The NODE_ENV variable lets you optimize your application build output for its environment. You can set it to a custom value, but it’s best practice to use either of the two common values, development or production, as most tooling works with these by default.
What Is the Crypto Module in Node.js?
The crypto module provides cryptographic functionality for development or production environments as an added security measure. This includes wrappers for OpenSSL’s hash, HMAC, cipher, decipher, sign, and verify functions.
What Are Stubs in Node.js, and How Are They Used?
A stub is a routine that stands in for a larger program, typically used with unit tests. It forces code to evaluate along a specific path, acting as a placeholder for a specific program execution path.
What Are the Exit Codes Used in Node.js?
Node.js uses exit codes to indicate the status of its processes. A healthy process returns 0 when there are no more pending asynchronous operations, but Node.js provides codes for errors in the run-time, handlers, uncaught exceptions, and general high-level errors. Familiarize yourself with these and the rule for exit codes greater than 128.
Node.js Internals
Now that you've demonstrated your understanding of when and how to use Node.js, it's time to show off your technical knowledge.
Making the most out of Node.js's signature characteristics can be tricky for new users, especially regarding its power to handle concurrency efficiently. Most problems in Node.js applications stem from trying to fit methodologies from other frameworks and languages into the Node.js paradigm.
You'll want to give mid to high-level explanations of these foundational concepts for these questions. Ideally, you’ll show that you understand and can teach these concepts to someone else.
Framing your knowledge through an analogy is a common strategy. Many guides often use a restaurant analogy — substituting the event loop with a server, clients with guest tables, and so on. However, if you don't have experience in the service industry, feel free to tweak your analogy to make it stand out.
How Does Node.js Handle Concurrency?
Node.js uses a single-threaded event loop model rather than a multi-threaded request and response model. Operations expected to run concurrently must allow the event loop to continue calling functions while I/O occurs asynchronously.
Why Is Node.js Single-Threaded, and What Are the Implications of That?
Except for specific library calls, Node.js’ event loop model operates in a single-threaded fashion. Natively coded implementations handle most I/O operations, which abstract threading concerns. Node.js also provides multi-threading capability by letting the event loop call out to worker pool threads.
When the workload associated with each client at any moment is small, Node.js performs better than standard multi-thread-based technologies. However, to gain this advantage, you must write the application to use asynchronous functions, and the use case must be suitable for an event loop model implementation. It’s best to have a Node.js front end handling requests and a back end implemented using a different framework to handle processing-intensive tasks.
What Are Event Loops in Node.js?
The event loop is a single thread that receives and schedules requests. It delegates requests to asynchronous threads when possible, providing the non-blocking I/O that makes up the core of Node.js.
What Are the Different Types of API Functions in Node.js?
API functions in Node.js are either asynchronous (non-blocking) or synchronous (blocking). All I/O methods in the standard Node.js library provide asynchronous versions and may accept callbacks. Some also offer synchronous versions, among which the most popular is the libuv library.
What Is the Difference Between Blocking and Non-Blocking Functions?
Blocking functions prevent subsequent function calls from executing until the blocking function's operation finishes. A non-blocking function is delegated to a separate thread, allowing the server to move on to the following API call.
You should write code using non-blocking functions whenever possible to allow concurrent operations. This is especially true in environments where latency can affect the order of execution in a concurrent model.
What Are EventEmitters and What Is Their Role?
Objects belonging to the EventEmitter class are called emitters. An emitter is a function containing a method for attaching functions (registering listeners) to an event and for triggering (emitting) that event. When an event is emitted, all its listeners are called synchronously.
What Is the Difference between the process.nextTick and setImmediate Methods?
The process.nextTick and setImmediate calls fire in different phases of the event loop. setImmediate method executes a callback on the next cycle of the event loop and the process.nextTick method fires during the event loop phase immediately after the operation finishes.
What Are the Differences Between Callbacks and Promises?
Callbacks are functions passed to other functions as arguments. They are part of the same task in the event loop’s sequence, ensuring that the inner function’s value is available for the outer function at its execution.
Promises are placeholders for data that may be available later. Promises let the event loop wait through its sequence while the promise evaluates asynchronously.
What Is Callback Hell and Why Does It Occur?
Callback hell, also known as the pyramid of doom, is caused by deeply nested callbacks and is often the result of improperly implemented asynchronous logic. The nested callbacks make code difficult to read and debug.
What Are Forks in the Context of Node.js?
The fork command is a particular case of spawn that generates a new instance of the V8 engine on which Node.js runs, allowing the parent and child processes to communicate. This is useful for creating a worker pool to use multi-core processors more efficiently and send individual messages between processes.
Ace your next Node.js interview.
Node.js is a popular environment, but you must use it correctly, like any tool. As a developer, you must understand how it fits in with the rest of a development stack.
This article covered the basic questions on using Node.js you’re likely to encounter in a job interview. However, acing an interview entails more than simply memorizing correct answers. You must show that you understand the material thoroughly and can describe it in your own words.
If you lack practical experience, you should look over case studies. These can help you understand deeper concepts, such as which patterns look asynchronous but aren’t or when to create an event loop-friendly code. It doesn’t hurt to know how to use Express with Node.js as well.
Best of luck!