You‘re probably here because you’ve already dipped your toes into the ocean of data science and machine learning with Python. Python has become a superstar, making machine learning accessible to everyone with its user-friendly syntax and a jaw-dropping range of libraries.
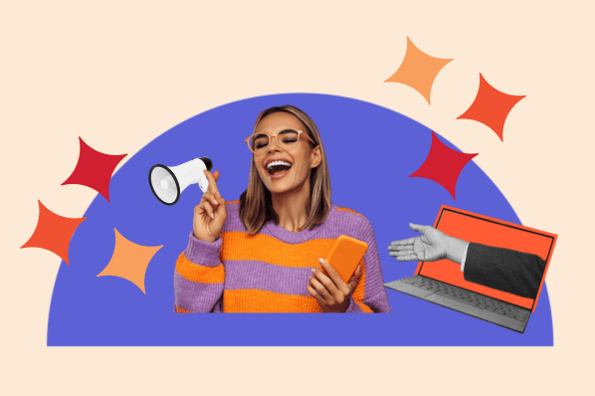
This blog is your treasure map to the lesser-known but incredibly valuable corners of Python machine learning. We‘re talking strategies that often get overshadowed but can take your models from "Hey, that’s pretty good“ to ”Wow, how did you even do that?"
So let's dive into the untapped potential that Python machine learning has to offer.
Setting the Stage: Preparing Your Environment
Before we embark on this journey, let's make sure your toolkit is as ready as you.
Python Libraries to Install
First things first: libraries. In Python, you‘ve likely used libraries like scikit-learn, TensorFlow, or PyTorch. For this deep dive, you’ll still need them, but we'll also introduce a few more specialized libraries to kick things up a notch.
- Imbalanced-learn: Great for handling imbalanced datasets, which often get overlooked.
- MLxtend: Perfect for those who want to explore the world of ensemble learning.
- TextBlob: An unsung hero for natural language processing (NLP) tasks.
- H2O: A fast, scalable machine learning platform that offers some unique algorithms.
To install these libraries, you can run the following commands in your terminal:
Setting up Jupyter Notebook
You might have your favorite IDE for Python, but for machine learning, Jupyter Notebooks are hard to beat. They provide an interactive way to code, visualize, and even document your process—all in one place. If you haven‘t yet set up Jupyter Notebook, don’t sweat it; it's as easy as pie.
Just run the following command:
After installing, launch it by running:
This will open a web browser displaying the Notebook dashboard. Now, you're all set to start your next chapter in Python machine learning!
Alright, toolkit ready, spirit ready, and you‘re ready. Up next, we’re going to shake up your understanding of preprocessing techniques.
Shaking Up the Foundations: Uncommon Preprocessing Techniques
Preprocessing—the term that often leads to eyes glazing over. It‘s the bread-and-butter of machine learning, but let’s face it, it can be a bit, well, mundane. Most tutorials talk about the usual suspects: normalization, handling missing values, one-hot encoding, and the like. But today, we‘re venturing off the beaten path. Let’s make preprocessing the star of the show for once.
Feature Engineering Like You’ve Never Seen Before
While everyone is busy with standard normalization techniques, there are so many other ways to enhance your features. Ever heard of quantile transformation? It can handle outliers and skewness in a way that conventional normalization techniques cannot. Or what about statistical binning? This can reveal categorical insights from continuous variables, and it's as easy as pie in Python.
Unique Text Processing Methods
Natural language processing (NLP) is like the Wild West of data science—exciting but a bit chaotic. Beyond the universal TF-IDF and word embeddings, there are more esoteric—but effective—methods like Word2Vec's lesser-known cousin, FastText, or the use of n-grams for sequence pattern recognition.
These techniques add nuance and depth to your text-based models, turning them into the Shakespeare of NLP (well, almost).
So, how are you doing? Still holding that cup of coffee? Good, because up next we're going to unbox some machine learning algorithms that deserve a little more spotlight. Fasten your seat belts—this is where the real fun starts! 🎢
Busting Myths: Algorithms You Might Have Overlooked
We‘ve ventured through unexplored preprocessing terrains, but now it’s time for the real meat of the matter: algorithms. Algorithms are the backbone of any machine learning project, but some of them are like those indie bands who make great music but haven't hit the mainstream yet.
Unconventional Classification Algorithms
You're probably familiar with the A-listers like Random Forest, Logistic Regression, and SVM. But what about the Hidden Markov Models or Gaussian Processes? These underutilized models can be particularly useful when your data exhibits sequential or temporal patterns. They might take a bit more work to understand, but the payoff is totally worth it.
Here's how you could implement Gaussian Processes in Python using scikit-learn:
Lesser-Known Regression Models
In the world of regression, it's not just about Linear or Polynomial Regression. Have you ever tried Quantile Regression for more robust predictions? Or Support Vector Regression (SVR) for handling non-linearities effectively?
Here's a simple code snippet for implementing SVR:
These under-the-radar algorithms can sometimes provide more accurate results or better fit your specific problem. They are the unsung heroes of the machine learning world and definitely worth your attention.
The Untapped Potential of Ensemble Learning
Now, we‘re going to talk about something even more intriguing: Ensemble Learning. Imagine if the Avengers were machine learning models—each strong on their own but nearly invincible together. That’s ensemble learning for you!
Why You Should Consider Stacking
You‘ve likely heard of Random Forest, an ensemble method that averages multiple decision trees for a more accurate and robust model. But what about stacking? In stacking, you literally ’stack‘ the predictions of multiple models and use another model to make the final prediction. It’s like a democracy where each algorithm gets a vote but the President (final model) has the last say.
Here's a quick Python example using MLxtend:
An Introduction to Bagging and Pasting
You've likely heard of boosting, but what about its cousins, bagging and pasting? Both methods involve creating multiple subsets of the original dataset and training a model on each subset. Bagging allows for sampling with replacement, whereas pasting does not. These techniques can give weaker models the strength to improve their performance significantly.
A quick example using Bagging in scikit-learn:
By exploring these ensemble techniques, you‘re not just iterating on your models; you’re evolving them. They become smarter, more accurate, and, dare we say, more elegant.
Hands-On Python Machine Learning Tutorial: Building an Advanced Model from Scratch
Ready to put all these awesome concepts into practice? We thought so! In this section, we’ll go step-by-step through building a machine learning model that incorporates some of the lesser-known preprocessing techniques, algorithms, and ensemble methods we’ve discussed. By the end of this tutorial, you‘ll have a state-of-the-art model that you can proudly show off in your portfolio. Let’s dive in!
The Dataset
For this tutorial, we‘re going to use the classic Iris dataset. Sure, it’s a bit of a cliché in the machine learning world, but it’s a great playground to test out advanced techniques. If you’re familiar with the dataset, you know it's all about classifying iris flowers into three species based on four features: sepal length, sepal width, petal length, and petal width.
Preprocessing: Quantile Transformation
We’re going to use quantile transformation for preprocessing. Why? Because it’s great at scaling features while reducing the impact of outliers.
Algorithm: Gaussian Processes
We're stepping away from the usual suspects like Decision Trees or Random Forest and taking Gaussian Processes out for a spin.
Ensemble: Stacking with MLxtend
Finally, let's use stacking to combine Gaussian Processes with a Logistic Regression model.
And there you have it—a robust, advanced machine learning model that makes use of some truly underrated techniques in the Python ecosystem.
Wrapping It Up: The Journey Ahead
If you‘ve been following along, you’ve not only picked up some extraordinary techniques but have also built a model that‘s nothing short of impressive. But let’s be honest—this is just the beginning.
Beyond Python: Exploring Other Paradigms
As versatile as Python is for machine learning, don‘t forget that there are other languages and frameworks out there that offer unique perspectives and capabilities. Languages like R and Julia, or even domain-specific tools like Weka, might offer solutions better suited to particular challenges. So don’t limit yourself—keep exploring!
The Ever-Evolving World of Machine Learning
Machine learning isn‘t static; it’s an ever-evolving field. New algorithms and techniques are being developed regularly. Stay updated by reading academic papers, following key influencers on social media, or participating in online forums and communities like GitHub.
Don't Just Build—Deploy!
While building models is rewarding, the real magic happens when you deploy them into a live environment. Whether it's automating a task, deriving insights from data, or creating an interactive app, a model in action is a joy to behold. So, consider looking into deployment strategies and technologies like Docker, Flask, or cloud services to bring your models to life.
Keep Challenging Yourself
Machine learning is a field that rewards curiosity and persistence. Don‘t settle for the ’good enough'; always aim for the extraordinary. Participate in hackathons, contribute to open-source projects, or even develop your own machine learning library.