Python comes equipped with several built-in functions that you can use to perform all sorts of tasks on your website.
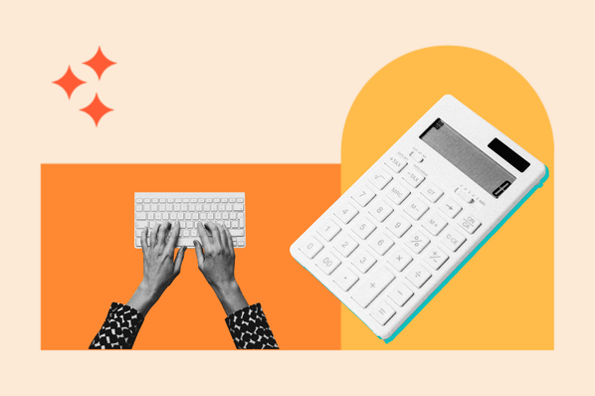
However, sometimes you need to import an additional module to perform different types of tasks within Python. A popular example of this is the math module.
The math module allows you to (you guessed it) perform a variety of mathematical functions within your Python code. This includes calculations like mean, median, and mode, plotting points on graphs, and even trigonometry.
We curated a list of math functions below to provide an overview of the most common math functions utilized in Python. Use this list as well as the interactive code module to hone your programming skills and become a stronger Python developer.
What is the Python Math Module?
In Python, you can import the math module to perform mathematical functions. To import the math module, use the following syntax:
import math
This will allow you to access the various mathematical functions available within the math module. Use these functions to calculate values such as average, mode, greatest common denominator, and more.
Python Math Functions
Use this table to navigate to specific Python functions. To try out the math functions below, copy and paste the example code into this module. From there, you should be able to execute the code's output using the play (horizontal triangle) button.
Please note that this module does not support the "statistics" Python code module. Therefore, the mean, median, and mode examples below are not interactive with the module above.
1. Sum Function: sum()
The sum() function calculates the sum of all items in an iterable or list.
Sum Function Example:
mylist = (5, 5, 5, 5, 5)
x = sum(mylist)
print(x)
Output:
25
Try with the interactive code module
2. Exponential Function: math.exp()
The exponential function in Python calculates the exponential value of a number by setting the base to the constant e (2.71828).
Exponential Function Example:
import math
print(math.exp(10))
Output:
22026.465794806718
Try with the interactive code module
3. Factorial Function: math.factorial()
The factorial function in Python calculates the factorial value of a given number.
Factorial Function Example:
import math
print(math.factorial(12))
Output:
479001600
Try with the interactive code module
4. Logarithm Function: math.log()
The logarithm or log() function calculates the natural logarithm of a number or value.
Logarithm Function Example:
import math
print(math.log(10))
Output:
2.302585092994046
Try with the interactive code module
5. Median Function: statistics.median()
The median function identifies the middle number in a dataset.
Median Function Example:
import statistics
print(statistics.median([2, 4, 6, 8, 10, 12, 14, 16, 18, 20]))
Output:
11.0
Try with the interactive code module
6. Mean Function: statistics.mean()
The mean function in Python calculates the average value of a dataset.
Mean Function Example:
import statistics
print(statistics.mean([2, 4, 6, 8, 10, 12, 14, 16, 18, 20]))
Output:
11
Try with the interactive code module
7. Mode Function: statistics.mode()
The mode function identifies the value that appears the most often in a dataset.
Mode Function Example:
import statistics
print(statistics.mode([20, 4, 6, 8, 10, 6, 12, 4, 6, 18, 20]))
Output:
6
Try with the interactive code module
8. Product Function: math.prod()
The product or prod() function in Python multiplies all of the elements in an iterable together.
Product Function Example:
import math
mylist = (2, 2,)
print(math.prod(mylist))
Output:
4
Try with the interactive code module
9. Greatest Common Divisor Function: math.gcd()
The GCD function in Python returns the greatest common divisor between two specified numbers.
GCD Function Example:
import math
print (math.gcd(25, 5))
Output:
5
Try with the interactive code module
10. Square Root Function: math.sqrt()
The square root or sqrt() function in Python returns the square root of a number.
Square Root Function Example:
import math
print (math.sqrt(64))
Output:
8
11. Average Function: Avg = (number_list)
The average function calculates the average value of a numerical list.
Average Function Example:
number_list = (1, 2, 3)
Avg = (number_list)
print(Avg)
Output:
2
Try with the interactive code module
12. Distance Function: math.dist()
The distance function in Python returns the distance between two points, also known as Euclidean distance.
Distance Function Example:
import math
x = [5]
y = [12]
print (math.dist(x, y))
Output:
7
Try with the interactive code module
13. Logistic Function: logistic
The logistic function is a mathematical function that takes any real number and returns a value between 0 and 1. This is useful for modeling certain types of problems, such as classification.
Logistic Function Example:
import numpy as np x = np.array([-2, 0, 2]) y = 1/(1 + np.exp(-x))
print(y)
Output:
array([0.11920292, 0.5, 0.88079708])
Try with the interactive code module
14. Power Function: pow()
The power or pow() function calculates the power of a base number and its exponent.
Power Function Example:
p = pow(2, 3)
print(p)
Output:
8
Try with the interactive code module
15. Ceil Function: ceil()
The ceil() function is a built-in function that returns the smallest integer greater than or equal to a given number.
Ceil Function Example:
import math
print(math.ceil(4.4))
Output:
5
// This statement will evaluate to 5 because 5 is the smallest integer greater than or equal to 4.4.
Try with the interactive code module
16. Floor Function: floor()
The floor() function is a built-in function that returns the largest integer less than or equal to a given number.
Floor Function Example:
import math
print(math.floor(4.6))
Output:
4
Try with the interactive code module
Python Trig Functions
Below is a list of trigonometry functions that you can perform in Python. These also require you to import the math module before you can execute the function.
1. Sine Function: math.sin()
The sine function or method in Python calculates the sine of a given number.
Sine Function Example:
import math
print (math.sin(21))
Output:
0.8366556385360561
2. Cosine Function: math.cos()
The cosine function in Python calculates the cosine value of a given number.
Cosine Function Example:
import math
print (math.cos(21))
Output
-0.5477292602242684
3. Tan Function: math.tan()
The tan function in Python calculates the tan value of a given number.
Tan Function Example:
import math
print (math.tan(21))
Output
-1.5274985276366035
4. Arc Sine Function: math.asin()
The arc sine function in Python calculates the inverse sine value of a given number.
Arc Sine Function Example:
import math
print (math.asin(1))
Output
1.5707963267948966
5. Arc Cosine Function: math.acos()
The arc cosine function in Python calculates the inverse cosine value of a given number.
Arc Cosine Function Example:
import math
print (math.acos(1))
Output
0.0
6. Arc Tan Function: math.atan()
The arc tan function in Python calculates the inverse tan value of a given number.
Arc Tan Function Example:
import math
print (math.atan(1))
Output
0.7853981633974483