If you love lists as much as many others do, then you'll know how versatile Python tuples are. A tuple allows you to store a collection of different data items in a single variable name. But did you know that tuples can do more than just hold data? In fact, they are one of the most powerful tools in your programming arsenal.
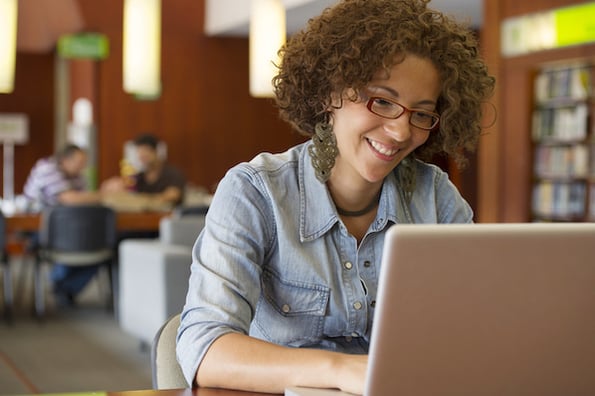
With tuples, you have a tool that lets you quickly process complex data structures without needing an extra variable. In this article, we will explore the Python tuple. We'll learn what tuples are, how to create them, and what sorts of tasks they are best suited for.
Let's take a closer look.
What is a Python Tuple?
Python tuples are a type of data structure that is very similar to lists. The main difference between the two is that tuples are immutable, meaning they cannot be changed once they are created. This makes them ideal for storing data that should not be modified, such as database records.
A tuple can have any number of items, which may be of different types, such as a string, integer, float, list, etc. Let's explore ways to create and use a tuple to make our programming tasks easier.
Creating a Python Tuple
Tuples can be created in a number of ways. The most common way is to wrap them in parentheses. Both single and multiple tuples must always be followed by a comma, like so:
You can also create a tuple without using parentheses, by separating the values with commas:
However, this can quickly become confusing, so it's best to stick with using parentheses. Let's now review the different types of tuples in Python.
Python Tuple Types
There are two main types of tuples: named tuples and unnamed tuples.
Named Tuples
Named tuples are created by subclassing the tuple class and giving the new class a name. For example:
Named tuples are often used to represent records, such as those in a database. Each element in the tuple represents a field in the record, and the tuple can be accessed by name instead of by index. Take a look at the example below:
Unnamed Tuples
Unnamed tuples are the more common type of tuple, and are simply created by separating values with commas, as we saw earlier. These tuples are often used for storing data that does not need to be named. Take a look at the example below:
In the example above, we created a tuple with three elements. The tuple can access these elements by the index, just like we would with a list.
Python Tuple Methods
There are a few methods that can be used on tuples. The most common is the index() method, which can be used to find the position of a given element in the tuple:
Another useful method is count(), which returns the number of times a given element appears in the tuple:
Finally, the len() function can be used to find the length of a tuple:
There are many other methods that can be used on tuples, but these are the most common.
Python Tuple Operations
Accessing Tuples
Tuples can be accessed just like lists, using square brackets and the index of the element you want to access. For example:
You can also access elements from the end of the tuple using negative indices:
Joining Tuples
Tuples can be joined together using the + operator:
This can also be done with the += operator:
Comparing Tuples
Tuples can be compared with each other using the comparison operators, such as == and !=. For example:
The comparison is done element by element, so tuples of different lengths can not be equal. Additionally, tuples can be compared with other data types, such as lists:
Nesting Tuples
Tuples can be nested inside each other to create complex data structures. For example:
This can be useful for representing data in a more natural way, such as a list of points in a two-dimensional space.
Converting Between Tuples and Lists
It's often useful to be able to convert between tuples and lists, as they are similar data types. This can be done using the tuple() and list() functions. For example:
This can also be done using the built-in zip() function, which takes two or more sequences and returns a list of tuples:
As you can see, there are many operations that can be performed with a Python Tuple. Let's take a look at when we should use a Python Tuple or a Python List.
Python Tuples vs Lists
As we've seen, tuples and lists are very similar data structures. So what are the main differences between them?
The main difference is that tuples are immutable, while lists are mutable. This means that tuples cannot be changed once they are created, while lists can be modified after they are created.
This also means that tuples can be used as keys in dictionaries, while lists cannot.
Another difference is that tuples are typically faster than lists. This is because Python knows that a tuple cannot be changed, so it doesn't need to allocate as much memory for it.
So when should you use a tuple, and when should you use a list? It really depends on the situation. If you need to store data that shouldn't be changed, then a tuple is the way to go. But if you need to store data that needs to be modified, then a list is probably a better choice.
For more information on Python tuples, check out this video:
Using Tuples in Your Python Program
All in all, tuples are a helpful data structure for those instances when you want to store values that shouldn't be changed. Now that you know more about tuples in Python, put them to use in your own programming. With these tools at your disposal, you're well on your way to becoming a master coder.