C++ is a favorite among developers due to its vast array of features. Its rich syntax and extensive set of keywords provide programmers with a wide range of tools to create efficient and robust applications. Whether you're a seasoned professional or just starting your journey into web development, understanding the core keywords of C++ is crucial to your success.
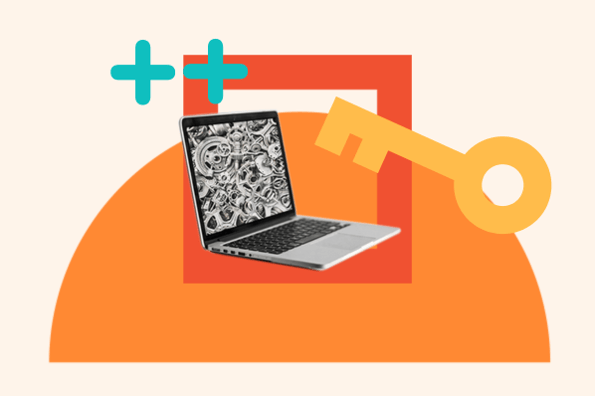
In this post, we'll review a list of C++ keywords including their definitions and an example of each. But, before we dive into that, let's review what C++ keywords are and what they do in this programming language.
What do keywords do in C++?
Keywords are reserved words that have predefined meanings and can't be used for any other purpose, such as naming a variable or function. They serve as building blocks of the language and form the foundation on which the code is written.
When used correctly, C++ keywords are used to create powerful and complex applications in a more organized and efficient way. They are essential for proper syntax in C++ programming, making code easier to read, understand, and debug.
Now that we know how these keywords are used, let's review a list of them below. If you're looking for a specific keyword, use the jump links in this table to find exactly what you are looking for.
Auto | Double | Long | Struct |
Default | Else | Namespace | Switch |
Break | Enum | New | Typedef |
Case | Float | Return | Unsigned |
Char | For | Short | Sizeof |
Const | If | Signed | Static |
Continue | Int |
C++ Keywords
1. Auto
This keyword automatically identifies the data type of a variable based on its initializer
Example:
using namespace std;
int main() {
auto number = 42;
cout << "The number is: " << number << endl;
return 0;
}
Output:
The number is: 42
2. Default
The default keyword is used to specify default behavior, whether it's related to constructors, function arguments, or switch cases.
Example:
auto number = 42; // automatically deduces the type of `number` as `int`
Output:
Identifies the number value as 42.
3. Break
This keyword exits a loop or switches a case statement.
Example:#include <iostream>
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
}
return 0;
}
Output:
Exits the loop when `i` is equal to 5
4. Case
This keyword marks a particular case in a switch statement.
Example:#include <iostream>
int main() {
int day = 3;
switch (day) {
case 1:
std::cout << "Monday";
break;
case 2:
std::cout << "Tuesday";=
break;
case 3:
std::cout << "Wednesday";
break;
default:
std::cout << "Invalid day";
break;
}
return 0;
}
Output:
Wednesday
5. Char
This keyword represents a character type (1 byte).
Example:#include <iostream>
int main() {
char grade = 'A';
return 0;
}
Output:
This assigns the value “A” to the variable “grade”
6. Const
This keyword defines a variable that cannot be changed after initialization.
Example:
#include <iostream>
const int MAX_VALUE = 100;
int main() {
std::cout << "The maximum value is: " << MAX_VALUE << std::endl;
return 0;
}
Output:
The maximum value is: 100
7. Continue
This keyword skips the current iteration of a loop and proceeds to the next one.
Example:#include <iostream>
int main() {
for (int i = 0; i < 5; i++) {
if (i == 2) {
continue;
}
std::cout << i << " ";
}
std::cout << std::endl;
return 0;
}
Output:
0134
8. Double
This keyword represents a double-precision floating-point number.
Example:
#include <iostream>
int main() {
double pi = 3.14159;
std::cout << "The value of pi is: " << pi << std::endl;
return 0;
}
Output:
The value of pi is: 3.14159
9. Else
This keyword specifies an alternative to the if statement.
Example:#include <iostream>
int main() {
int age = 20;
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are not yet an adult." << std::endl;
}
return 0;
}
Output:
Since the input age is 20, which is greater than 18. The output for this would read “You are an adult.”
10. Enum
This keyword is used to define an enumerated data type.
Example:#include <iostream>
enum Color {
RED,
GREEN,
BLUE
};
int main() {
Color selectedColor = GREEN;
if (selectedColor == RED) {
std::cout << "The selected color is RED." << std::endl;
} else if (selectedColor == GREEN) {
std::cout << "The selected color is GREEN." << std::endl;
} else if (selectedColor == BLUE) {
std::cout << "The selected color is BLUE." << std::endl;
} else {
std::cout << "Invalid color selection." << std::endl;
}
return 0;
}
Output:
The selected color is GREEN.
11. Float
This keyword represents a single-precision floating-point number.
Example:
#include <iostream>
int main() {
float price = 9.99f;
std::cout << "The price is: " << price << std::endl;
return 0;
}
Output:
The price is 9.99
12. For
This keyword creates a loop that iterates over a defined range.
Example:#include <iostream>
int main() {
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
return 0;
}
Output:
01234
13. If
This keyword creates a conditional statement that evaluates whether a condition is true or false.
Example:#include <iostream>
int main() {
int x = 10;
if (x > 0) {
std::cout << "Positive Number" << std::endl;
}
return 0;
}
Output:
Positive Number
14. Int
This keyword represents an integer type (typically 2 or 4 bytes).
Example:#include <iostream>
int main() {
int age = 25;
std::cout << "Age: " << age << std::endl;
return 0;
}
Output:
Age: 25
15. Long
This keyword represents a long integer type (typically 4 or 8 bytes).
Example:#include <iostream>
int main() {
long population = 8000000000;
std::cout << "World population: " << population << std::endl;
return 0;
}
Output:
World population: 8000000000
16. Namespace
This keyword creates a namespace that can contain variables and functions.
Example:#include <iostream>
using namespace std; // imports the standard namespace
int main() {
cout << "Hello, World!";
return 0;
}
Output:
Hello, World!
17. New
This keyword dynamically allocates memory during program execution.
Example:
#include <iostream>
int main() {
int* ptr = new int;
*ptr = 42;
std::cout << "Value: " << *ptr << std::endl;
delete ptr;
return 0;
}
Output:
Value: 42
18. Return
This keyword exits a function and optionally returns a value.
Example:#include <iostream>
int add(int a, int b) {
return a + b; // returns the sum of `a` and `b`
}
int main() {
int result = add(5, 7);
std::cout << "Result: " << result << std::endl;
return 0;
}
Output:
Result: 12
19. Short
This keyword represents a short integer type (typically 2 bytes).
Example:#include <iostream>
int main() {
short distance = 100;
std::cout << "Distance: " << distance << std::endl;
return 0;
}
Output:
Distance: 100
20. Signed
This keyword specifies that a variable can hold negative and positive values.
Example:#include <iostream>
int main() {
signed int temperature = -10;
std::cout << "Temperature: " << temperature << std::endl;
return 0;
}
Output:
Temperature: -10
21. Static
This keyword specifies that a variable or function belongs to the class and is not an instance.
Example:#include <iostream>
int main() {
int size = sizeof(int);
std::cout << "Size of int: " << size << " bytes" << std::endl;
return 0;
}
Output:
Size of int: 4 bytes
22. Struct
This keyword defines a composite data type, similar to a class.
Example:
#include <iostream>
#include <string>
struct Person {
std::string name;
int age;
};
int main() {
Person person1;
Person1.name = "John";
person1.age = 25;
std::cout << "Person's Name: " << person1.name << std::endl;
std::cout << "Person's Age: " << person1.age << std::endl;
return 0;
}
Output:
Person’s Name: John
Person’s Age: 25
23. Switch
This keyword creates a switch statement that evaluates an expression against a range of possible values.
Example:
#include <iostream>
int main() {
int day = 3;
switch (day) {
case 1:
std::cout << "Monday";
break;
case 2:
std::cout << "Tuesday";
break;
case 3:
std::cout << "Wednesday";
break;
default:
std::cout << "Invalid day";
}
return 0;
}
Output:
Wednesday
24. Typedef
This keyword creates aliases for data types.
Example:#include <iostream>
typedef unsigned long long ULL; // Creates an alias for the data type unsigned long long
int main() {
ULL num = 1234567890ULL; // Uses the typedef alias `ULL` for the variable `num`
std::cout << "Value of num: " << num << std::endl;
return 0;
}
Output:
Value of num: 1234567890
25. Unsigned
This keyword specifies that a variable can hold only positive values.
Example:
#include <iostream>
int main() {
unsigned int count = 100; // Unsigned integer data type to store non-negative whole numbers
std::cout << "Count: " << count << std::endl;
return 0;
}
Output:
Count: 100
26. Sizeof
This keyword returns the size in bytes of a data type or variable.
Example:
#include <iostream>
int main() {
int size = sizeof(int); // Retrieves the size (in bytes) of the int data type
std::cout << "Size of int: " << size << " bytes" << std::endl;
return 0;
}
Output:
Size of int: 4 bytes
Using Keywords in C++
Whether you're new to C++ programming or have been coding in it for years, having a solid grasp of these keywords can only set you up for success. They not only lay the groundwork for building complex applications, but they also help you design efficient algorithms and optimize the overall performance of your code.
Keep this blog post handy as it serves not only as an introduction to C++ keywords but also as a handy reference that you can bookmark for later. Feel free to revisit it whenever you need a quick reminder or want to explore alternative ways of using these keywords.
Author's Note: This post was written/edited by a human with the assistance of generative AI.