At the start of your developer journey, one of the first things you learn is how to call a function. Functions are an essential part of programming that allow you to group related code together.
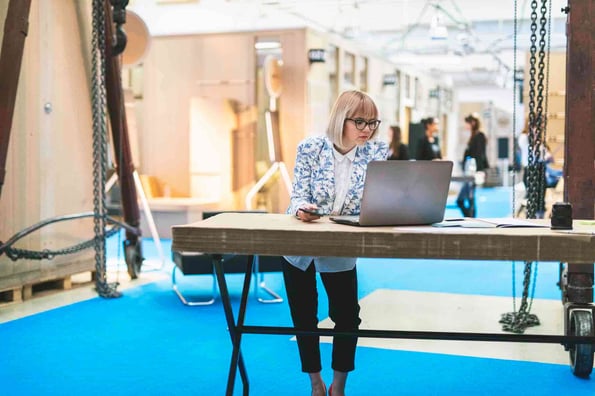
In this post, we’ll take a look at how to call a function in JavaScript. We’ll also look at some of the benefits of using functions, and see why they’re so important. Without further ado, let’s get started!
What does it mean to call a function in JavaScript?
In JavaScript, functions are first-class objects. This means they can be created and used just like any other object in the language. Functions can be passed as arguments to other functions, assigned to variables, and even returned to other functions.
One of the most common ways to call a function is by using the keyword “function.” For example, let’s take a look at the following.
We can call this function by passing in the name of the person we want to greet. For example, let’s say we want to greet John:
This will print “Hello, John” in the console.
We can also call functions by using their name. For example, we could also write:
This will also print “Hello, Sarah” in the console.
How to Call a Function in Javascript
Functions are a cornerstone of programming, and JavaScript is no exception. Functions allow you to group related code together, making your programs more organized and manageable. In this article, we’ll take a look at how to call functions in JavaScript.
There are a few different ways to call a function in JavaScript. The most common way is simply to use the function name followed by parentheses.
If you have a function stored in a variable, you can call it by using the variable name followed by parentheses.
You can also call functions using the apply() or call() methods. These allow you to specify the value of this inside the function.
As you can see, there are a few different ways to call a function in JavaScript. Meanwhile, how you call them remains the same.
What Calling a Function in Javascript Looks Like
As you can see, calling a function in JavaScript is pretty straightforward. All you need to do is use the function name followed by parentheses.
Inside the parentheses, you can specify any arguments that the function needs to run. In the example above, we don’t need to pass any arguments to our function because it just prints a message to the console.
However, if we wanted to print a different message, we could do something like this:
As you can see, we just pass in the message that we want to print as an argument to our function. This is a very simple example, but you can use this same syntax to call functions with more complex logic.
When Should You Call a Function in Javascript?
There really isn’t a definitive answer to this question. It depends on how you’ve structured your code and what you’re trying to achieve.
However, a good rule of thumb is to call functions when you need to execute a certain block of code multiple times or when you need to perform a task that can be abstracted away into a function.
For example, let’s say you have a website with a navigation bar. The navigation bar has a few different links, each of which leads to a different page on the site. When a user clicks on one of the links, you need to update the URL in the browser’s address bar and load the new page.
Rather than writing this logic out multiple times for each link, you could abstract it away into a function. Then, all you would need to do is call the function whenever a user clicks on a link.
This would make your code more DRY (Don’t Repeat Yourself) and easier to maintain.
Using Javascript Functions
Calling functions is an important part of programming in JavaScript. By abstracting away complex logic into functions, you can make your code more readable and maintainable.
Next time you’re stuck on how to call a function in JavaScript, remember that there are a few different ways to do it. And if you’re not sure when to call a function, just ask yourself whether the task at hand can be abstracted away into a function. Chances are, it can!