One benefit of the internet is quick access to information. With a few words, a search engine can interpret the user's needs and return relevant results.
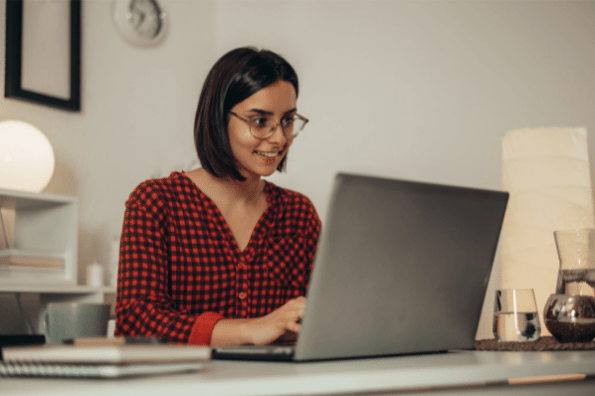
WordPress isn't Google, but you want to provide the same value for your site visitors by serving relevant content. Fortunately, WordPress provides options for users to dig deeper into your content, including categories, tags, and search bars.
However, if you're building a WordPress site or customizing an existing one, you may encounter situations where the default options aren't enough. For example, you're creating an author page and want to display all their posts. That's when you turn to the get_posts function.
What is the get_posts function?
The WordPress get_posts function is a method for retrieving a custom set of posts based on specified criteria. It's important to note that posts in this use case can also mean pages or custom post types. The results of the get_posts query can then be displayed on the page.
When do you need get_posts?
Anytime you need to retrieve a set of posts and display them. Some common use cases are displaying a list of your most popular posts, posts from the same author as the article your visitor is reading, and posts in the same category as the content being shown. You will see how these get_posts calls are written in PHP in a later section.
How does get_posts work?
get_posts takes your search criteria and then works inside the WP_Query object to convert the PHP code into a SQL query to your WordPress site's database.
The results are converted into WP_Post objects, where each object represents one post. These objects are grouped into an array that get_posts loops through to display the results based on your instructions.
Before continuing, let's take a closer look at the difference between get_posts and WP_Query.
get_posts vs. WP_Query
As discussed, get_posts is a method of the WP_Query class used to search the database for post data and content.
WP_Query queries the database to display posts using The Loop, which is the main process that WordPress uses to display posts.
You might wonder why get_posts exists if WP_Query also pulls post content and displays it. The key difference is that get_posts does not alter The Loop. The Loop is a central process to rendering posts and pages on your site, and it can lead to site issues if not reset after each use in your code.
get_posts was created to avoid this pitfall, making it a preferred method for WordPress plugin developers who want to add value without causing issues in the host's site. The function pulls information from the database and automatically resets The Loop after each call.
Now that we understand get_posts, let's walk through the different pieces of our request in the code.
Anatomy of a get_posts Query
get_posts is written in PHP, and the most common issue you'll encounter is improper syntax. To prevent these errors, this section will walk through each component of a get_posts request.
get_posts Call
At its simplest, a get_posts request is a function call.
get_posts();
This is the basic building block. However, calling the get_posts function on its own does not give you data to interact with because you have not assigned it to a variable.
$sample_array = get_posts();
Now you have an array of post objects assigned to the sample_array variable. In this use case, you did not define any parameters, so the default result is a pull of the five most recent posts. We'll review adding parameters to your get_posts call in the final section, but first, we'll look at how to display our current results.
get_posts foreach Loop
The get_posts function has returned an array, so naturally, the next step is to loop through the results to display them. To do this, use the foreach method.
foreach($sample_array as $post)
{
echo "<h3>" . $post->post_title . "</h3>";
}
The above method loops through each WP_Post object in our array and pulls the post_title property to display in an HTML H3 tag. Let's combine this with our get_posts function call.
<?php
$count = 1;
$sample_array = get_posts();
foreach($sample_array as $post)
{
echo "<h3> ${count}. " . $post->post_title . "</h3>";
++$count;
}
?>
By adding the count variable, you now see a list of the five most recent posts ordered from newest to oldest. This can also be achieved with an ordered list in HTML. The screenshot below shows the result on the page.
post_title isn't the only property accessible from the WP_Posts object. Other useful properties include:
- ID: the post's ID
- post_author: the post author's name
- post_date: the post's publish date
- post_content: the post's content, including text, images, links, and media
- comment_count: the post's comment total
Now that you understand the default get_posts call, let's add custom parameters to the query.
get_posts Parameters
The true power of get_posts comes from its ability to accept custom search criteria. Let's expand the previous example and increase the number of recent posts pulled from five to 10 in the arguments array.
<?php
$count = 1;
# Custom parameters added inside arguments array
$arguments = array(
"numberposts" => 10
);
# Arguments variable provided as argument to get_posts function
$sample_array = get_posts($arguments);
foreach($sample_array as $post)
{
echo "<h3> ${count}. " . $post->post_title . "</h3>";
++$count;
}
?>
By default, the numberposts parameter is set to five, but here the count is increased to 10. As the arguments array suggests, you can provide more than one parameter. There are 15 unique parameter groups to query for in get_posts. Let's walk through the most common parameters.
Common get_post Parameters
Below are the search parameters you will likely use:
- numberposts: declares the number of posts you want returned — use -1 to retrieve all posts matching your criteria
- category: dictates whether results should fall under one or multiple categories based on their IDs
- orderby: defines the criteria that you want the retrieved posts ordered by (e.g. by date, by number of comments, etc.)
- order: determines if the results are sorted in ascending or descending order
- include: defines posts you want included in the returned array based on their IDs
- exclude: defines posts you don't want included in results based on their IDs
- post_type: decides if get_posts retrieves posts, pages, or custom post types
- post_status: determines if the query retrieves published, scheduled, in-progress, or deleted posts
The previous example has been updated with additional parameters below. Now, this request will pull 10 posts in the fiction category ordered by date from oldest to newest. Note that the fiction category is identified by its ID, which is an integer.
<?php
$arguments = array(
"numberposts" => 10,
"category" => 2,
"orderby" => "date",
"order" => "ASC"
);
$sample_array = get_posts($arguments);
foreach($sample_array as $post)
{
echo "<h3> " . $post->post_title . "</h3>";
echo "<p>" . $post->post_date . "</p>";
}
?>
Before continuing, let's take a deeper look at the post_type parameter.
WordPress get_posts Custom Post Type
In the course of building your WordPress site, you may build custom post types beyond the default posts and pages. These can be anything from testimonial blocks to product pages. To fetch these objects, use the post_type parameter. In this example, get_posts calls down the three most recent testimonials to display on the page.
<?php
$arguments = array(
"post_type" => "testimonial",
"numberposts" => 3
);
$sample_array = get_posts($arguments);
foreach($sample_array as $testimonial)
{
echo "<h3> " . $testimonial->test_client_name . "</h3>";
echo "<p>" . $testimonial->test_summary . "</p>";
}
?>
A side benefit of this function call is that any time another testimonial is added to the database, it will automatically display on any pages with this get_posts request — assuming its date is newer than the three currently showing. The custom post type is one way that get_posts supports advanced CMS features within your WordPress site.
You'll see more parameters in action as the next section examines three common get_posts queries you can use in your code.
Common get_posts Queries
Now that you understand how to write a get_posts request, let's examine a few queries you may use on your site.
1. Get most popular posts.
WordPress sites often have a widget showing the most popular blog posts. To achieve this, target the posts with the most comments in get_posts.
<?php
$arguments = array(
"orderby" => "comment_count"
);
$sample_array = get_posts($arguments);
foreach($sample_array as $post)
{
echo "<h3> " . $post->post_title . "</h3>";
echo "<p>" . $post->comment_count . "</p>";
}
?>
Note that because the default get_posts request will pull five posts and sort them in descending order, this code does not define those arguments. However, you can change these parameters and add others to customize as needed.
2. Get posts by author.
You may want to build an author page so that your audience can find more posts by the writer. You can accomplish this by calling the get_posts function with author and numberposts parameters.
<?php
$arguments = array(
"numberposts" => -1,
"author" => 2
);
$sample_array = get_posts($arguments);
foreach($sample_array as $post)
{
echo "<h3> " . $post->post_title . "</h3>";
echo "<p>" . $post->post_date . "</p>";
}
?>
Note that like our previous example, this code does not restate get_posts' default arguments to order by post date and sort in descending order. This avoids unnecessary code and simplifies debugging.
3. Get posts in same category.
Another common strategy is to display posts in the same category at the end of a post so the reader can find similar content. To achieve this, the code below uses the category parameter and limits the number of posts to three because this is the typical number displayed.
<?php
$arguments = array(
"numberposts" => 3,
"category" => 2
);
$sample_array = get_posts($arguments);
foreach($sample_array as $post)
{
echo "<h3> " . $post->post_title . "</h3>";
echo "<p>" . $post->post_date . "</p>";
}
?>
Again, the parameter defaults of sorting by post date in descending order are left in place.
get_posts Offers New Value to Your Audience
WordPress stores a wealth of data about each post and page, and the get_posts function is a primary method to dissect that information. With parameters in more than a dozen categories, you have endless possibilities for delivering new content to your audience. The get_posts function removes the need to write queries in SQL, lowering the barrier to entry and simplifying debugging.
No matter your goal, get_posts will help you better understand your content and discover new connections between your posts to better serve your audience.