There’s nothing worse than being late for an important date or missing out on some relevant news until it’s too late. That is why tracking dates and timestamps are valuable in software development. The JavaScript programming language offers a way to simplify this process for developers to integrate timekeeping into their software.
This post will explore the JavaScript Date object and learn how it works. You will also see some examples and explanations of the syntax. Afterward, you will learn about the JavaScript Date object properties and how to use them. Finally, you will learn about the methods offered by the JavaScript Date object and see how they work.
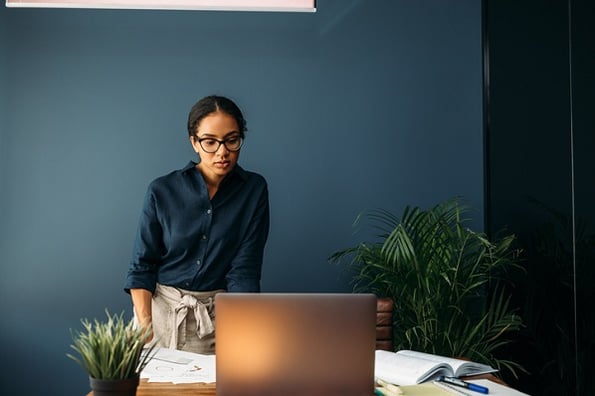
Without wasting more time, let’s get started.
JavaScript Date Object
The JavaScript Date object is an object that creates time stamps for various software needs. A large majority of JavaScript developers for tracking data entry times for different applications. Social media software uses it to display posting and comment times for tracking updates.
Take a look at the following image to understand the breakdown of the Date object timestamp.
The Date object creates a date-time stamp of the current time or a specified time. Let’s take a look at an example of the simplest form of syntax for the Date object.
const currDate = new Date();
This line of code would create a date object with the current time at execution, which would mean it would look similar to the following.
"2022-06-01T16:19:07.199Z"
JavaScript Date Object Arguments
If you want a date from the future — or past — saved, you can send in a specific date as an argument in milliseconds unless it’s a UNIX timestamp. The Date object can accept up to seven arguments; Let’s look at that next.
const rndmDate = new Date(2018, 6, 22, 7, 22, 13, 0)
In the above example, the arguments passed are for the year, month, day, hour, minutes, seconds, and milliseconds. The recommendation is for a minimum of three arguments starting with the year, month, and day. Most interpreters will accept less than three, yet providing one parameter will result in the number getting interpreted in milliseconds.
The date object counts time using milliseconds since midnight Jan 1, 1970. You can retrieve this date from the date object by passing the number zero, as seen below.
const firstDate = new Date(0)
console.log(firstDate);
This code would create a timestamp of the first second of the Date object’s range. The result would look like the example below.
"1970-01-01T00:00:00.000Z"
Arguments passed to the Date object should be an integer in milliseconds if only providing one integer. If you pass in a string such as “this string here” the interpreter will throw an error invalid date, yet it will try to interpret dates in the form of strings to the closest it can.
It is ill-advised to use strings due to the room for interpretation that can be prone to issues. Let’s look at the example below and discuss what’s happening.
const dateTwo = new Date("December 25, 2021");
This line of code provides a string date that gets interpreted as expected despite lacking specificity. Because of this, the dates get interpreted to the earliest date associated with the interpretation. In the end, this means that strings as arguments open the door for errors and issues. It is also important to note that months start counting at zero instead of one.
The best practice is to parse strings into integers before providing them as an argument. However, if you must use a string try to stick with the three standard formats that the Object accepts as numbers.
Type Example
ISO Date "2015-03-25" (The International Standard)
Short Date "03/25/2015"
Long Date "Mar 25 2015" or "25 Mar 2015"
JavaScript Date Properties
Interestingly the Date object in JavaScript doesn’t have any native or static properties. However, using the prototype you can create and add properties to any date object you create. For example, if you want to tie multiple pieces of information to a date object you can use the prototype. Let’s look at an example of how to do that next.
const todaysDate = new Date();
Date.prototype.xMasDay = new Date(2021, 12, 25);
What’s unique about this is that it will add this new date to the date object as a property, as such instances of the date object will also be able to access it. Elaborating on this, the variable above has a current timestamp, after which the property xMasDay was added directly to the date object. The code below shows how you could access that property from the todaysDate variable and see how much time has passed.
>
const msTimePassed = todaysDate.getTime() - todaysDate.xMasDay.getTime();
>The line of code above gets the respective time for each object in milliseconds for each date. It then subtracts the Date object’s property xMasDay and identifies the difference between it and the current date. This technique helps create historical dates and add them as properties for your date objects.
JavaScript Date Methods
The JavaScript Date object has many methods that manipulate how data gets returned. Let’s look at how these Date objects return data as a string, and then modify a date using a method.
The Date object has many getter and setter methods, each serving a different purpose. Some getters allow you to get the month, day, year, hour, and more. Equally, some setters will enable you to set the different parts of a created timestamp. First, let's look at the syntax for the methods used on the Date object.
const newDateObj = new Date();
newDateObj.methodName();
Now let’s look at some of the methods for the Date object and see what they return.
Alternatively, there are setters to complete the opposite of each of these tasks. In other words, each of the above getter methods has an opposite setter method.
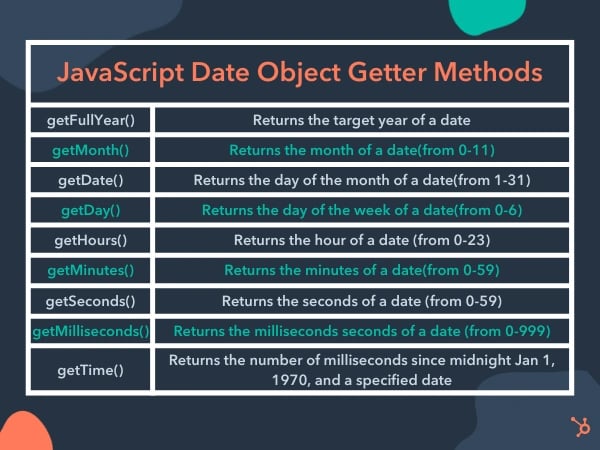
While this is not an extensive list of methods available to the Date object, they are some of the most common.
Getting Started With JavaScript Date Object
The list of getter and setter methods available to the Date object is immense and would take a long time to cover all of them. The best way to familiarize yourself with the Date object is to practice using it and combine it with the methods available. Learn other methods and see how they work together with the various date objects you create.