Wheels, hoops, and loops have served humans very well throughout history, but today we will be discussing a different kind of loop. One of the most used loops in modern technology, the powerful programming loop — specifically the JavaScript for loop.
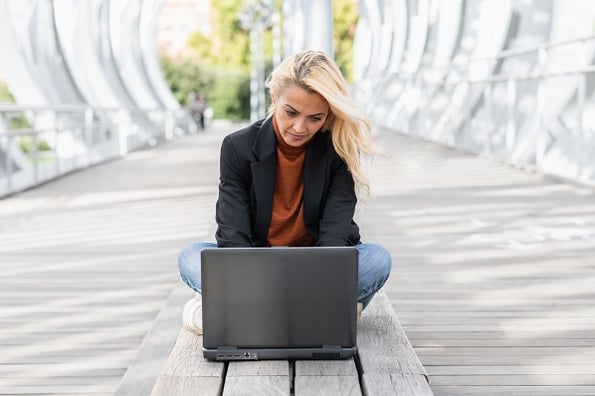
JavaScript is a powerful programming language that offers several methods for iterating through enumerable objects. While there are options that do a lot of the heavy lifting such as Javascript frameworks and plugins, you'll need to use for loops regardless. These methods are called loops, and there are three types that are most commonly used to complete these tasks:
- Standard for loop
- The for-in loop
- And the for-of loop
In this post, we will look at some examples of each and explain how they work.
What is a for loop?
In programming, a for loop is used to iterate through a list and complete repetitive tasks based on a boolean value of either true or false. When writing the code, you create a scope-bound variable that holds the number of times you want the loop to run.
JavaScript for Loop Example
At the beginning of execution, the loop will check if a condition — or conditions — evaluates to true. If the resulting value of the complete expression is true, the loop will perform a given task and restart. This process repeats until either the condition evaluates to false or the loop has reached its maximum iteration.
Next, let’s take a look at an example of a for loop in action.
This syntax is pretty straightforward, but let’s break it down for clarity.
- Enumerator: This is a statement tied to the scope of this block of code — meaning it only exists in the loop. It runs only one time at the beginning of the code block, and is typically used to set a variable which represents the starting point of the loop.
- Condition: The condition is a statement that evaluates to true or false — that value determines whether the loop will continue — if false, the loop stops executing.
- Statement: The statement is a line of code that executes on each iteration regardless of the value of the condition.
With this information, let's take another look at the code block, this time as a practical example.
JavaScript break in for Loops
The JavaScript break keyword is used to force the end of the looping statement in response to a certain condition set by the programmer. This keyword can be used to catch errors during the execution of the code and end the loop preventing errors and running checks in real-time.
An example of how this might be used can be seen below.
JavaScript for Loop Array
The JavaScript for loop can also iterate through an array, collection objects, and even key-value pairs. In JavaScript, a standard for loop will handle arrays just fine, but for more complex structures there are other kinds of for loops.
There are two types of for loops that can do this with ease — the for-of loop, and the for-in loop. Next, we’ll look at an example of each and discuss how they work starting with the for-in loop.
JavaScript for-in Loop
The for-in loop iterates over the enumerable properties of an object, it is comparable to being a two-dimensional iterator, whereas the for-of is comparable to being a three-dimensional iterator.
Let’s take a look at an example of this in action.
- The for-in loop iterates over an object
- Each iteration returns a key
- The key is used to access its value
With this loop, the order in which the indexes are returned may not always be in the same order. If the order of indexes is important then you will be better off using the for-of loop instead.
JavaScript for-of Loop
This is used to loop over iterable objects such as maps, arrays, sets, and more. In the case of any loop, a function can be performed against either the key or the value of the pair.
- variable: For every iteration, the value of the next property is assigned to the variable. Variable can be declared with const, let, or var.
- iterable: An object that has iterable properties.
The syntax here is not much different than for a standard for loop.
On each iteration, the value of the next property is assigned to the current state of value.object.
Final Takeaways on JavaScript for Loops
Javascript for loops are extremely powerful and their use will vary depending on why you need them. With that in mind, let’s go over some important points to consider when deciding which to use.
- The standard for loop is best for performing simple tasks a set number of times based on a passing condition or set of them.
- The for-in loop is great for handling a set of tasks repetitively against an object and its properties, and may not return indexes in the expected order.
- The for-of loop is used to loop over data structures such as Arrays, Strings, Maps, NodeLists, and more.
For loops are very powerful and can be used to create and modify data sets of varying types, master them and it will pay off.