JavaScript methods (or functions) enable you to accomplish all sorts of tasks on your website or program. They can count elements on a page, delay the timing of events, and make your web content more interactive and engaging for your audience.
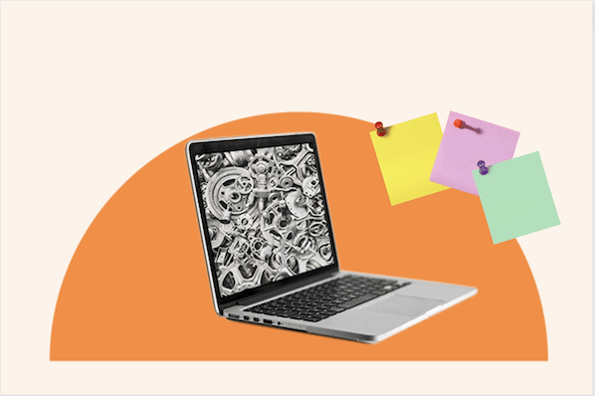
With all that power at your disposal, the tradeoff is keeping track of it all in one place. There are so many functions and methods you can utilize that it's easy to forget one or two that you might really need, when you really need it.

That's why we've compiled this comprehensive list of built-in JavaScript methods. Read on to learn how to define a JavaScript method, how to add parameters and arguments to it, and to familiarize yourself with over 40 definitions and examples of different JavaScript methods.
Table of Contents
- How to Define a JavaScript Function
- JavaScript Function Parameters & Arguments
- JavaScript Functions & Methods
How to Define a JavaScript Function
Defining or declaring a function calls the function into your code with specified parameters. The function is not executed immediately but waits to be triggered before performing its intended action.
JavaScript Function Declaration
In JavaScript, you can define a function using a declaration or an expression. A function declaration begins with the function operator followed by your function’s name and parameters.
JavaScript Function vs. Method
Throughout this guide, you may see some functions referred to as functions while others are referred to as methods. They both execute tasks, but the difference is that methods in JavaScript are connected with an object while functions are not.
JavaScript Declaration vs. Expression
Unlike a declaration, a JavaScript expression stores the function as a variable you can call using the variable’s name. Here’s what a JavaScript expression might look like:
Output: Hello
In this example, the greeting() function is declared as a variable that can be called with its name. The output (Hello) is then printed out on the console.
How to Write a Function or Method in JavaScript
To call a function in JavaScript, you can use the keyword function followed by the function’s name.
JavaScript Function Syntax Example:
See here for the complete breakdown of how to call a JavaScript function.
JavaScript Function Parameters & Arguments
JavaScript functions are declared with parameters and arguments. Parameters specify the values expected when a function is called, while arguments are the actual values passed to the function when it is called.
Default Function Parameter Values
Default function parameter values allow you to set a default value for function parameters.
Default Function Parameter Values Example
Output: Hello HubSpot
In this example, the default function parameter values are used to set a default value of "Hubspot" for the name parameter. The output (Hello HubSpot) is then printed out on the console.
JavaScript Function With Optional Parameters
Optional parameters are parameters that can be used optionally in a function.
Example:
Output: Hello John, Welcome to HubSpot!
In this example, the optional parameter message is set with a default value of "Welcome to HubSpot!". The output (Hello John, Welcome to Hubspot!) is then printed out on the console.
Pass a JavaScript Function as a Parameter
You can pass a JavaScript function as a parameter to another function.
Example:
Output: The result is 15
In this example, the calculateResult() function is used to call the addTwoNumbers() function and pass two numbers as parameters. The output (The result is 15) is then printed out on the console.
Now that you are familiar with how to create a JavaScript function or method, use the list below as a handy resource to improve your development skills.
List of JavaScript Functions
- console.log()
- console.count()
- print()
- callback()
- function()
- recurse()
- setTimeout()
- setInterval()
- alert()
- remove()
- import()
- document.write
- bind()
- Math.min()
- Math.max()
- Math.floor()
- prompt()
- Number()
- then()
- every()
- submit()
- greet()
- require()
1. Function Expression: function
A function expression is a way to define an anonymous function without giving it a name.
JavaScript Function Expression Example
See the Pen JavaScript Function Expression by HubSpot (@hubspot) on CodePen.
Output:
Hello Reader!
In this example, the function expression defines an anonymous function that prints out ‘Hello Reader!’ when called.
2. Name Property: function.name
The name property is used to return the name of a given function.
JavaScript Function Name Example
See the Pen JavaScript Function Name by HubSpot (@hubspot) on CodePen.
Output:
sayHello
In this example, the name property is used to return the name of a function, which in this case is ‘sayHello’. The name property is useful for debugging and tracking purposes.
3. Inline Function
The inline function syntax is used to write a single line of code for a function.
Inline Function Example
See the Pen Inline Function JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Hello Reader!
In this example, the inline function syntax defines a function that prints out ‘Hello Reader!’ when called.
Inline Function vs. Anonymous Function
An anonymous function has no name, whereas the inline function is a function that is assigned a variable that acts similarly to a name.
Anonymous Function Parameters
Anonymous functions can take in any number of parameters specified in the function’s parenthesis.
4. Console Log Method: console.log()
The console.log() method prints a message to the console.
Console Log Method Example:
See the Pen Console log Method by HubSpot (@hubspot) on CodePen.
Output:
My name is HubSpot
In this example, the console.log() method is used to write the message ‘My name is HubSpot’ on the console. The output is then printed out on the console.
5. Print Method: print()
The print() method prints the output of the contents to your screen.
Print Method Example
See the Pen Print Method Example by HubSpot (@hubspot) on CodePen.
Output:
A window opens that displays ‘Hello Reader!’. The print() method displays a given string in the browser window in this example.
6. Callback Function: callback()
A callback function is a function that is passed as an argument located inside of another function.
Callback Function Example:
See the Pen JavaScript Callback Function by HubSpot (@hubspot) on CodePen.
Output:
Hello User
Welcome to HubSpot!
In this example, the anonymous callback function logs “Welcome to HubSpot!” after a greeting of “Hello User”.
Callback Function With Parameters
Callback functions can also include parameters, like the one in the example below:
Output: Result is 15
In this example, the doSomething() function takes in two parameters: param and callback. After some calculations, the result is passed to the callback function as a parameter.
7. JavaScript Generator: function*
The function* generator defines a generator function, which returns an iterator object.
Generator Function Example
See the Pen Untitled by HubSpot (@hubspot) on CodePen.
Output:
Hello
In this example, the function* generator defines a generator function that yields two strings - 'Hello' and 'Reader!'. The next() method returns the first string, ‘Hello’.
8. Immediately Invoked Function: function()
The immediately-invoked function expression (IIFE) executes a function immediately after it is defined.
Immediately Invoked Function Example
See the Pen Immediately Invoked Function JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Hello Reader!
In this example, an anonymous function is declared and immediately invoked, which prints out ‘Hello Reader!’.
9. Recursive Function: recurse()
A recursive function calls itself and is used to solve problems.
Recursive Function Example
See the Pen Recursive Function JavaScript by HubSpot (@hubspot) on CodePen.
Output:
3
In this example, the function calls itself until it reaches the base case of n being equal to 1. The result is 3.
10. Lambda Function
A lambda function in JavaScript contains one or more parameters and has a single expression. This allows you to pass a function as a parameter to another function.
Lambda Function Example
See the Pen JavaScript Lambda Function by HubSpot (@hubspot) on CodePen.
Output:
3
In this example, the lambda function takes two parameters - ‘a’ and ‘b’ - and adds them together for a result of 3.
11. Delay Function: setTimeout()
The setTimeout() function can be used to delay a function from executing until a specified time.
Delay Function Example
See the Pen JavaScript Delay Function by HubSpot (@hubspot) on CodePen.
Output:
Hello Reader!
In this example, the setTimeout() function is used to delay the printing of ‘Hello Reader!’ for 3 seconds.
12. Set Interval Method: setInterval()
The setInterval() method executes a function repeatedly, with a fixed time delay between each call.
Set Interval Method Example
See the Pen Set Interval method javascript by HubSpot (@hubspot) on CodePen.
Output:
0
1
In this example, the setInterval() method is used to execute a function (console.log(i++)) repeatedly, with a fixed time delay of 1 second (1000 milliseconds) between each call. The output is then printed out on the console.
13. Alert Method: alert()
The alert() method displays an alert window that the user must dismiss on the page.
Alert Method Example
See the Pen Alert Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
An alert window is opened that displays ‘Hello Reader!’ and requires the user to dismiss it before continuing.
14. Remove Method: remove()
The remove() method can be used to remove an element from the DOM.
Remove Method Example
See the Pen remove method javascript by HubSpot (@hubspot) on CodePen.
Output:
<body></body>
In this example, the remove() method removes a div element from the DOM. After removing the element, console.log(document.body) returns an empty body tag.
15. Import Method: import()
The import() method imports a module from another script.
Import Method Example
See the Pen Import Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Hello HubSpotter!
In this example, the import() method is used to import a module (sayHello) from another script (hello.js). The output is then printed out on the console.
16. Documentation Function: document.write()
The document.write() method is used to write content to the web page’s output stream.
Documentation Function Example
See the Pen Documentation Function JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Hello HubSpotter!
In this example, the document.write() method is used to write content to the web page’s output stream. The string 'Hello HubSpotter!' is then printed on the webpage.
17. Bind Method: bind()
The bind method attaches an object to a function. It is used to create a new function from an existing one and set the ‘this’ keyword for that function.
Bind Method Example
See the Pen Bind Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Hello HubSpotter!
In this example, the bind() method is used to create a new function from an existing one and set the ‘this’ keyword for that function. The bound function then prints out ‘Hello HubSpotter!’ when called.
18. Empty Functions: emptyFunction(){}
An empty function is a function that does nothing. It is useful for defining functions before assigning them values or for preventing a code block from executing.
Empty Function Example
See the Pen JavaScript Empty Functions by HubSpot (@hubspot) on CodePen.
Output:
No output
In this example, an empty function is defined and then called. Since the function does nothing, no output is printed on the console.
19. Min Method: Math.min()
The Math.min() method returns the smallest number in a given array or list of numbers.
Min Method Example
See the Pen Min Method by HubSpot (@hubspot) on CodePen.
Output:
-4
In this example, the Math.min() method returns the smallest number in a given array of numbers. The output is printed out on the console, which is -4 – indicating that it is the smallest number in the list.
20. Max Method: Math.max()
The Math.max() method returns the largest number in a given array or list of numbers.
Max Method Example
See the Pen Max Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
4
In this example, the Math.max() method returns the largest number in a given array of numbers. The output is printed out on the console, which is 4 – indicating that it is the largest number in the list.
21. Floor Method: Math.floor()
The floor method rounds a value down to the nearest integer.
Floor Method Example
See the Pen Floor Method Example by HubSpot (@hubspot) on CodePen.
Output:
3
In this example, the Math.floor() method is used to round a number (3.6) down to its nearest integer (3). The output is then printed out on the console.
22. Console Count Method: console.count()
The console.count() method is used to keep track of the number of times a line of code has been called/executed.
Console Count Method Example:
See the Pen console count method javascript by HubSpot (@hubspot) on CodePen.
Output:
Hello: 1
World: 1
Hello: 2
In this example, the console.count() method is used to keep track of the number of times a line of code has been called/executed. The output is then printed out on the console.
23. Init Method
The init method declares a variable and assigns it an initial value.
Init Method Example
See the Pen Init Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
In this example, we use the init method to declare a variable named varName and assign it an initial value.
24. Prompt Method: prompt()
The prompt method launches a dialog box that prompts the user to input text or click on something.
Prompt Method Example
See the Pen Prompt Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Input text from the user’s response
In this example, the prompt() method launches a dialog box that prompts the user to input their name. The output is then printed out on the console.
25. Number Method: Number()
The Number() method converts a given value into a JavaScript number.
Number Method Example:
See the Pen Number Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
10
In this example, the Number() method converts a string ('10') into a number. The output is then printed out on the console.
26. Then Method: then()
The then() method allows you to execute certain instructions after completing an asynchronous operation.
Then Method Example
See the Pen Then Method Example by HubSpot (@hubspot) on CodePen.
Output:
Output from asyncFunc()
In this example, the then() method is used to execute a set of instructions (console.log(value)) after an asynchronous operation has been completed (asyncFunc). The output is then printed out on the console.
27. Every Method: every()
The every() method checks if all elements in an array pass a certain condition.
Every Method Example
See the Pen Every Method Example by HubSpot (@hubspot) on CodePen.
Output:
true
In this example, the every() method is used to check if all elements in an array (numbers) are less than 4. The output is then printed out on the console.
28. Reference Error: ReferenceError
A ReferenceError is an error that occurs when a variable or function isn’t defined.
Reference Error Example:
See the Pen Reference Error JavaScript by HubSpot (@hubspot) on CodePen.
Output:
ReferenceError: sayHello is not defined
In this example, the console throws a ReferenceError because the variable sayHello has not been defined.
29. Function Signature
A function signature is a syntax that defines how a function should be written and what it should return.
Function Signature Example
See the Pen Function Signature JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Function definition
In this example, the function signature defines how a function (add) should be written and what it should return. The output is then printed out on the console.
30. AngularJS ng-click Function: ng-click="myfunction()"
AngularJS's ng-click function runs a custom code when an element is clicked.
AngularJS ng-click Function Example
See the Pen AngularJS NG Click by HubSpot (@hubspot) on CodePen.
Output:
Nothing
In this example, the ng-click function is used to run a custom code (myFunction()) when an element (the button) is clicked. The output is not printed out on the console, as no return statement has been defined in the example.
31. Form Submit Method: submit()
The form submit method submits an entry to a JavaScript or HTML form.
Form Submit Method Example
See the Pen Form submit Method by HubSpot (@hubspot) on CodePen.
Output:
Output of myFunction()
In this example, the submit() method is used to submit an HTML form. Once the button is clicked, the output from myFunction() will be printed out on the console.
32. Form Action: form action=""
The form action attribute specifies the page URL to be loaded when the form is submitted.
Form Action Example
See the Pen Form Action Example by HubSpot (@hubspot) on CodePen.
Output:
Here, the action attribute is set with a value of "/process-data", which will load this URL when the form is submitted.
33. If Else Method: if(){}else{}
The if/else method executes instructions depending on the evaluated condition.
If Else Method Example
See the Pen If Else Method by HubSpot (@hubspot) on CodePen.
Output:
Number is greater than 5
In this example, the if/else method evaluates the condition (number > 5). Depending on the result of this evaluation, either ‘Number is greater than 5’ or ‘Number is not greater than 5’ will be printed out on the console.
34. Greet Function: greet()
The greet function is a named function created to generate a greeting for a user.
Greet Function Example
See the Pen Greet Function JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Hello John
In this example, the greet() function creates a personalized greeting for John. The output is then printed out on the console.
35. Require Method: require()
The JavaScript require method imports modules, packages, or libraries into your program.
Require Method Example
See the Pen Require Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
18th April 2023, 03:12 pm
In this example, the require() method imports the moment library into the program. The output (18th April 2023, 03:12 pm) is printed on the console.
36. Private Method: #
The private method creates a private function that can only be used inside its parent module.
Private Method Example
See the Pen Private Method JavaScript by HubSpot (@hubspot) on CodePen.
Output:
Hello Reader!
In this example, a private method called ‘sayHello’ is defined using the octothorpe (#). The greet() public method then calls the private method to print out ‘Hello Reader!’.
37. Empty Statement: ;
The empty statement tells the JavaScript interpreter that there is an intentional blank line in the code.
Empty Statement Example
See the Pen Empty Statement by HubSpot (@hubspot) on CodePen.
Output:
Hello HubSpotter!
Goodbye HubSpotter!
In this example, the empty statement is used to indicate that there is an intentional blank line in the code. The output prints out ‘Hello HubSpotter!’ and ‘Goodbye HubSpotter!’ as expected.
38. JavaScript Arrow Functions
The JavaScript arrow or fat arrow function is a feature that was released in 2015 with ES6. These functions are written with a shorter syntax and do not require all of the same elements that a regular JavaScript function calls for.
Arrow Function Example
See the Pen Arrow Functions by HubSpot (@hubspot) on CodePen.
Output:
Hello HubSpot
In this example, we use the arrow function to create a greeting that takes in a name parameter and returns "Hello" with the name. The output (Hello HubSpot) is then printed out on the console.
Arrow Function vs. Regular JS Function
The most fundamental difference between arrow and regular functions in JavaScript is the length of the syntax. Arrow functions are more concise than regular functions and do not require elements like arguments and prototype objects. Arrow functions are typically written with cleaner syntax than regular JavaScript functions.
39. Break Statement
The break statement is used to terminate the execution of a loop or switch statement. In other words, you can use the break statement to stop a JavaScript function.
Break Statement Example
See the Pen Break Statement by HubSpot (@hubspot) on CodePen.
Output:
0
1
2
3
4
In this example, the break statement is used to terminate the execution of a loop (for) when the value of i is 5. The output is then printed out on the console.
How to Use a Break Statement to Exit a Function
You can also use the break statement to exit a function and return a value or expression.
Example:
Output:
Number found or Number not found.
In this example, the break statement is used to exit a function (findNumber) and return a value or expression (‘Number found’ or ‘Number not found’). The output is then printed out on the console.
40. Onload: onload=""
An onload event triggers your code immediately after a page loads.
Onload Event Example
See the Pen Onload Event exampl,e by HubSpot (@hubspot) on CodePen.
Output:
This code would trigger your JavaScript function immediately after a page loads.
41. JavaScript Constructors: this, constructor
JavaScript constructors are functions that create new objects when invoked. They can be used to define classes, and the this keyword can be used to access the properties and methods of an instance of a class.
Object Constructor Example
See the Pen object constructor example by HubSpot (@hubspot) on CodePen.
Output:
Person {name: "John", age: 18}
In this example, the Person constructor creates a new object with the properties name and age.
Class Constructor Example
See the Pen class constructor by HubSpot (@hubspot) on CodePen.
Output:
Car {make: "Ford", model: "Mustang"}
In this example, the Car class constructor creates a new object with the properties make and model.