If you‘re a web developer or designer striving to remain up-to-date with digital technology, it’s crucial to comprehend the modulus functionality of JavaScript. The modulus tool is a powerful feature that can enhance your coding abilities, enabling you to split numbers and manipulate strings and other data types.
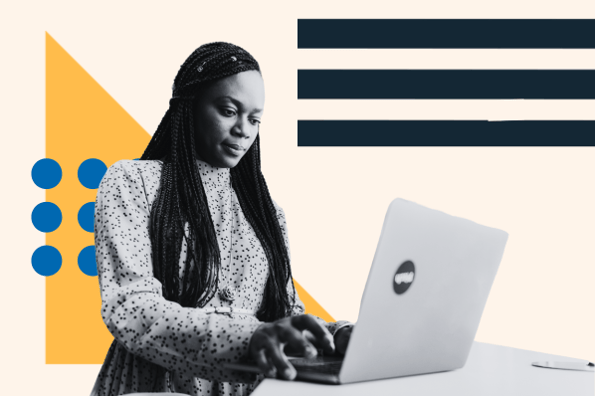
In this blog post, we‘ll explain how to use JavaScript’s modulus to save time and effort while creating more powerful code that yields superior results.
What is a modulus operator in JavaScript?
The operator in JavaScript is denoted with the percent symbol (%), and it calculates the remainder when one number is divided by another.
If we divide 10 by 3, we get a quotient of 3 and a remainder of 1. We can find the remainder directly by using the modulus operator:
The modulus operator works with whole numbers and decimals, as well as positive and negative numbers. It is frequently used in programming for tasks such as checking for even or odd numbers, determining array positions, and creating loops with a specific number of iterations.
How to Calculate Modulus in JavaScript
In JavaScript, we can use the % operator to calculate the modulus. It gives us the remainder of a division operation involving two numbers. The syntax for this operation is as follows:
The dividend is the number being divided.
The divisor is the number dividing the dividend.
The following code module demonstrates how to use the modulus operator for three different scenarios in JavaScript:
In the first example, we perform a calculation on two whole numbers (10 and 3) to find their modulus. The modulus is the remainder obtained when dividing 10 by 3. The result of this calculation is 1.
The second example demonstrates how to find the modulus of two decimal numbers (15.5 and 2.5). The modulus is calculated to be 0.5, representing the remainder when 15.5 is divided by 2.5.
In the third example, we find the modulus using a negative number (-10 and 3). The resulting value is -1, which represents the remainder when -10 is divided by 3. It's important to note that the sign of the value is dependent on the sign of the divisor (-10 in this instance).
Practical Applications of Modulus
The JavaScript modulus operator has several practical applications in programming. Some common examples are listed below.
1. Checking for Even or Odd Numbers
To determine if a number is even or odd, you can use the modulus operator. If the remainder of dividing the number by 2 is 0, it is even. If the remainder is 1, it is odd. Below is an example code:
2. Determining Array Position
The modulus operator can help us find the position of an element in an array. For instance, we could use it to cycle through an array and repeat the cycle after a certain number of iterations. Here's an example code:
This code loops through the array 10 times and uses the modulus operator to find the position of each element. % arr.length makes sure the index remains within the array's bounds, even if the loop iterates more times than there are elements in the array.
3. Creating Loops with a Specific Number of Iterations
The modulus operator can be used to create loops that execute a specific number of times. For example, if we want a loop to execute five times, we can use the modulus operator to check whether the current iteration is a multiple of five. Here is an example code:
This code contains a loop that repeats 20 times and outputs only the values that are divisible by 5. The “% 5” is used to check if the current iteration is a multiple of 5.
4. Implementing Calendar Functions
Here is an example code that demonstrates how the modulus operator can be used to determine the day of the week for a given date:
This code creates a Date object that represents May 9, 2022. The code also uses the getDay() method to find out which day of the week that date falls on. Then, the code uses the modulus operator to access an array of day names and display the result.
Recap of JavaScript Modulus
In JavaScript, the modulus operator is a useful tool that enables developers to carry out calculations involving remainders. It has practical applications in various scenarios, including determining if a number is even or odd, identifying the position of an element in an array, creating loops with a specific number of iterations, and implementing calendar functions.
Developers can make their code more efficient and readable by understanding how to use the modulus operator’s syntax and parameters. It’s crucial to understand the modulus operator in JavaScript programming, whether you are a novice or an expert.