JavaScript strings are one of the most used data types in the language; you will undoubtedly use them in your software development process. Therefore, you will need to know how to interact with them with that in mind.
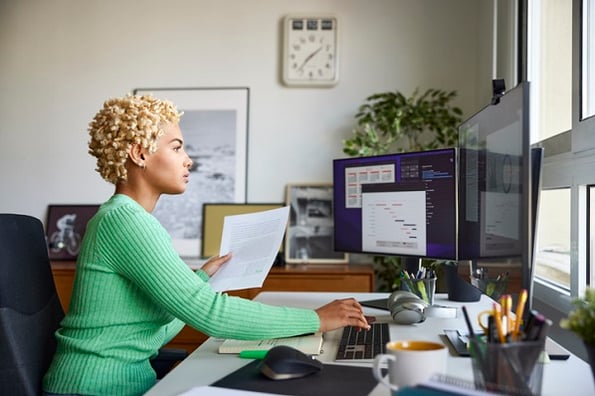
This post will cover the simple string method called split and how to use it. You will learn how it works, discover the syntax, and see code examples. You will also learn how to use it in different ways to accomplish various tasks. Finally, there will be a CodePen at the end with all the post's examples to practice what you’ve learned.
Here are some links you can use for easier navigation of this post.
- How To Split String In JavaScript
- String Split JavaScript Example
- Getting Started Using String Split In JavaScript
Without any further delay, let’s dive right in.
JavaScript Split String
Splitting a string in JavaScript is a valuable process and can be implemented for various uses. Developers will often break strings apart for security reasons, such as checking that the string entered does not contain any malicious code or incorrect characters.
Another use for split strings is creating simple classic games like word guessing or word building games. Eventually, you will use the split method to divide a string into substrings on a long enough timeline. Substrings are the return value of the split method. The following video showcases this process to help illuminate the concept.
When the split method is used on a string, an array of substrings is created from the target. The array of substrings works like any other JavaScript array, and you can access an item in the array using the array-like notation.
Now that we have covered what it is and what it is used for let’s look at the syntax and discover how it works.
How to Split String in JavaScript
The process for splitting a string in JavaScript is relatively simple, as is the syntax for the method. The split method accepts two optional parameters that dictate how the method will behave. Let’s look at the syntax of the split method and dissect it to understand better how it works.
strVar.split(separator, limit);
The above code showcases the syntax of the split method and its two parameters. First, the method is called on a variable with a string value using the dot notation, and then it is given a character to split at and a limit.
The separator parameter identifies what character to split the string at, such as a space or a non-alphanumeric character.
let intro = "Hello! I'm The Doctor.";
let subStr = intro.split("!");
The code above will split the string into an array of substrings containing two items. The value of each item would be; "Hello" and " I'm The Doctor." respectively.
The following parameter determines the number of times this action should occur within the provided string.
let intro = "Hello! I'm The Doctor.";
let subStr = intro.split("", 5);
The code above would result in a five-item array with the following values; "H","e","l","l","o" stopping after a total of five splits have resulted in five array items.
Using the split method without any parameters will result in a single item array with the original string value.
let intro = "Hello! I'm The Doctor.";
let subStr = intro.split();
Splitting the string with no parameters, as seen above, results in a single item array with the value — "Hello! I'm The Doctor." — identical to the original string.
Next, let’s look at some examples of how this method works and how you can use it to perform various functions.
String Split JavaScript Example
To split a string in JavaScript is a simple process yet is very robust in its potential uses. One popular usage is to create a routine that checks and strips special characters from a username. Be sure not to rely on boolean checks using this method as it always return true except in the case of an error.
Let’s look at a few important caveats before looking at some examples.
String Split Method Caveats
There are some important caveats to understand when dealing with the split method. Below is a list that highlights important things to consider.
Caveats for the separator parameter are listed below.
- The split method always returns true.
- Providing no separator parameter returns a one-item array with the original string value.
- A one-item array with the original string value is returned if the separator is not located.
- If the separator is found at the end of a string, the returned array will have an additional empty string item.
- Providing a non-string value as the separator will throw an error message “Uncaught TypeError: intro.split is not a function” and cause your code to fail.
- After a substring is added to the new array, the subsequent passing character and any proceeding it will be assigned to the next array item until the next instance.
- Splitting immediately after a split or at the end of a string creates an empty string as an array item.
Caveats for the limiter parameter are listed below.
- The limiter identifies how many times the method should perform the split on the target string but is often thought of as the maximum number of array items.
- If a number provided for the limit is not met, no adverse behavior should be expected.
- The split limiter should always be equal to or larger than the array it returns.
- Providing zero as the limiter value will return an empty array with no items.
- Calculating the number value for the limiter should be done with care, as the separator parameter can affect the number of splits that need to happen.
Now that you have a firm grip on the caveats of using the split method and its parameters, let’s dive into some code examples of how to use it. Below is a CodePen that contains all the techniques discussed above so that you can experiment with the string split method.
Getting Started Using String Split In JavaScript
The best way to solidify your understanding of the JavaScript split string method is to start using it in your code. To help with that, the CodePen above contains everything discussed in this post, with room to build on it. You can create a copy of the CodePen and use it with this post to build on what you have learned here.
Once you feel confident in your knowledge of the subject, start thinking about ways you can improve your code. For example, you can identify processes that can be simplified by using the string split method and clean up any code. Alternatively, if you don’t have any code that could benefit from this, you should consider it while writing code moving forward.