JavaScript is a simple and powerful programming language that creates software and technology for many applications. From web development to programming interfaces and software for NASA, it has grown in popularity since its inception. The language is so robust that there are many topics to learn and many ways to use them.
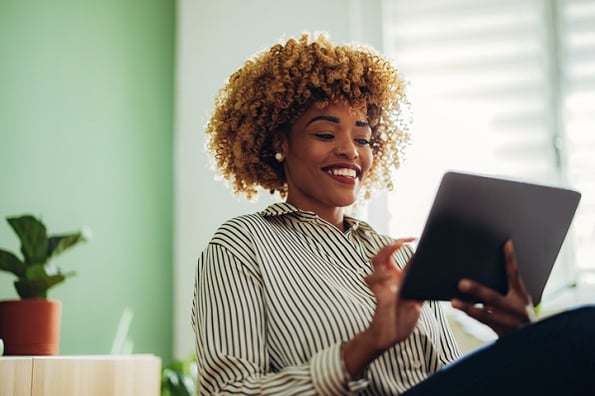
This post will cover JavaScript string methods that modify and manipulate string values. We'll review built-in string methods and others that can perform various tasks on strings. Furthermore, you will see some examples of syntax you can use in CodePen to help solidify your understanding.
Without further delay, let's jump right in.
Table of Contents
- JavaScript Strings Explained
- What are JavaScript string methods?
- Built-in JavaScript String Methods
- How to Use String Methods
- JavaScript String Methods & Examples
JavaScript Strings Explained
The JavaScript string is a data type used to convey information as text, which differs from numerical data types like int. Specifically, an int value can only be a number, but strings can contain any character. Because of this, they are commonly used in software development across different industries and languages.
Because of the popularity and need for string data, JavaScript offers many different methods for interacting with and modifying strings. In fact, there are over 30 distinct methods for modifying and manipulating string values.
What are JavaScript string methods?
The usefulness of strings is boundless, so it's crucial to understand how you can use them to your advantage. Arguably, the most common task you will complete is modifying a string in some way. To facilitate that process, JavaScript includes a string object allowing methods and properties to be executed on string values.
Check out this video on string methods to learn more about what they are.
One use of strings is sterilizing any malicious code from being injected into a program or software. This process happens using a regex — or regular expression — which in no way looks like regular expressions. Regular expressions are patterns on strings to ensure they meet specific formatting requirements.
Built-in JavaScript String Methods
In many languages, strings are objects and thus have several properties and methods to build on their functionality. In JavaScript, however, strings are a primitive data type and thus do not have any built-in methods or properties. Despite this fact, JavaScript does support several methods and a couple of properties on strings.
Because JavaScript treats strings as objects when methods or properties are executed, they still have the ability to be built up. This ability is due to the string object in the JavaScript library or framework, though it’s not intended for creating string objects. Creating string objects results in slower processing and potentially errant results, so use them cautiously.
Now that you've learned the theory behind methods and strings, let's dive into how they work together.
How to Use String Methods in JavaScript
Truthfully, using string methods is relatively easy to do. However, understanding what's happening is a different topic. Let’s clarify this with an example. After all, using a string method is simply a matter of understanding the syntax.
With that in mind, let’s look at a code example of the syntax below.
That is the bare bones of every string method. It starts with a variable holding a primitive string value. You can then chain the desired method to the string variable using the dot notation and finish it off with parenthesis and a semicolon. Here is a code snippet showing how to use the trim() method.
Simple enough, right? Well, what if that method requires a parameter? In that case, the method will accept some criteria on what to do with the string value. This behavior changes things a little.
Let's look at a simple example of a method with parameters. The code below does the same as the code above, removing any whitespace from a string. Only this time, it's done using the replace() method.
The replace method accepts two parameters, the first is what the string method should look for, and the second is the value to replace with the target.
The above code uses a regular expression that equates to “all-white space, inclusive”, which tells JavaScript to remove all occurrences of any white space.
Now that we've reviewed what string methods are and how to use them let's look at a comprehensive list of string methods available in JavaScript.
JavaScript String Methods & Examples
This section will review some of the more popular JavaScript string methods. We've also included this cheatsheet below that you can keep as a handy reference.
For these examples, let's use the following string:
Copy and paste these values into the CodePen below to test them out for yourself.
1. Lowercase Method: toLowerCase()
The lowercase method returns a string with all values converted to lowercase letters.
Lowercase Method Example
2. Uppercase Method: toUpperCase()
The uppercase method returns a string with all values converted to uppercase.
Uppercase Method Example
3. String Method: toString()
The string method returns a string or string object as a string.
String Method Example
4. Substring Method: substring()
The substring method extracts characters from a string between two specified indices.
Substring Method Example
5. Includes Method: includes()
The includes method returns a string if it contains a specified value.
Includes Method Example
6. Match Method: match()
The match method returns a string object with a matching value.
Match Method Example
7. Concat Method: concat()
The contact method returns two or more joined strings.
Concat Method Example
8. Trim Method: trim()
The trim method removes unneeded whitespace from the start and end of a string.
Trim Method Example
9. Eval Method: eval()
The eval method allows code written as a string to be evaluated and executed.
Eval Method Example
10. Replace Method: replace()
The string replace method returns a new string to replace a piece of an existing string.
Replace Method Example
11. Test Method: test()
The test method checks if there is a match in a string. It returns the value, True or False, depending on the outcome.
Test Method Example
12. Repeat Method: repeat()
The repeat() method is used to repeat a given string a specified number of times.
Repeat Method Example
13. Search Method: search()
The search() method is used to search for a pattern in a string and returns the index position of the match.
Search Method Example
14. Escape Method: escape()
The escape method returns a string that’s encoded so it can be transmitted to different computers on different networks.
Note: This function has been deprecated and is not recommended for use. Instead, you can use the encodeURI() function.
Escape Method Example
Getting Started Using JavScript String Methods
This list is not exhaustive; there are many more methods, and some are much less known than others. An example of these lesser-known methods would be the padStart() and padEnd() methods which allow you to add a string to the beginning and end of a string value.
There is a lot to learn under the umbrella of strings and string methods. The best way to solidify all the information is to practice using them. Endless applications use strings, and even more ways methods can help.
Working with string methods, you can learn to understand the boundaries and behaviors and how to use methods together. Combining string methods allows you to perform powerful tasks and do almost anything your software may require.