JavaScript is a versatile programming language used extensively in modern web development. One of the fundamental features of JavaScript is its ability to manipulate data, including converting it from one data type to another. The toString() method is one way to change data types.
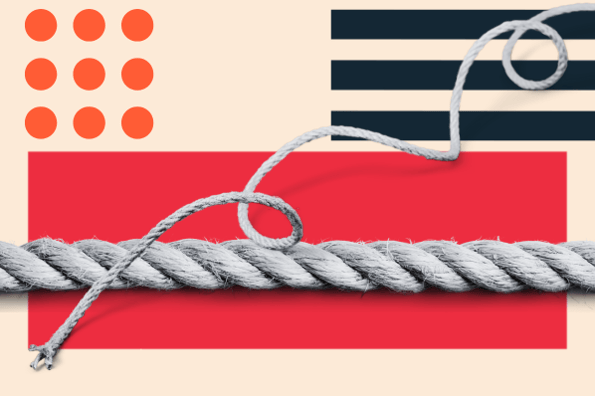
The toString() method in JavaScript changes a value into a string. It works for all data types and objects and gives a standard way to show the value as a string. This new string can be used for tasks like printing, joining with other strings, or modifying the data.
For beginners in JavaScript, it’s important to grasp the toString() method. This method lets you modify and arrange data to work with other systems and programs. Often, the toString() method is used to turn numbers, booleans, arrays, and objects into strings. If needed, it can be customized to give personalized output for objects and classes.
Table of Contents
- What is toString() in JavaScript?
- How to Use toString() in Javascript
- Customizing the toString() Method
What is toString() in JavaScript?
The toString() method in JavaScript converts a value to a string. This method is available for all JavaScript objects and data types and provides a standardized way to represent the value as a string.
Understanding the toString() method is important for JavaScript developers as it allows them to manipulate and format data in ways that are compatible with other programs and systems. Additionally, the toString() method is used frequently in debugging and troubleshooting scenarios to better understand the values of different variables and objects.
Overall, a good understanding of the toString() method can help developers write cleaner, more efficient code and improve their overall development skills.
How to Use toString() in JavaScript
To use the toString() method in JavaScript, you must have a specific value or object you want to transform into a string. The general syntax for using the toString() method is outlined below:
To convert a JavaScript value or object to a string, use the toString() method and call it on the value object using dot notation.
The behavior of the toString() method can be customized by adding parameters. The radix parameter is commonly used in the toString() method when converting numbers to strings. It determines the base to use when representing the number and can be set anywhere between 2 and 36, with a default value of 10.
This is a code module in JavaScript with three examples that illustrate the implementation of the toString() method:
In Example 1, the toString() function converts a number into a string. This string is subsequently printed to the console.
In Example 2, the toString() method transforms an array into a string. The resulting string uses commas to separate array elements and is also displayed on the console.
In Example 3, to convert a number to a binary string, we use the toString() method and set the radix parameter to 2, representing binary. In this case, we call the toString() method on the binaryNum object and log the resulting binary string to the console.
Customizing the toString() Method
To customize the behavior of the toString() method for specific objects and classes, you can use it in addition to converting JavaScript values and objects to strings. This feature allows you to manage the output of the toString() method and provides more useful information about the object or class being converted.
To customize how an object or class is converted, override the default toString() method. This involves creating a new toString() method for the object or class, replacing the default method when the object is converted to a string.
Here’s an example of how to override the default toString() method for a custom class in JavaScript:
We created a Person class with a constructor that takes a name and age parameter. The default toString() method for the Person class was overridden with a new one that returns a string containing the person’s name and age.
To get the custom output, we created a new Person object and called the toString() method.
To customize the string representation of built-in JavaScript objects like arrays and dates, you can override the toString() method. See below for an example of overriding the toString() method for an array:
We define a new toString() method for the arr array and use the join() method to join all the elements with a hyphen. We get the customized output by calling the toString() method on the arr array.
Defining a custom toString() method in your JavaScript code can give developers more helpful information about objects and classes. This can be done by customizing the method's output to provide meaningful details.
Getting Started
The toString() method is a crucial aspect of JavaScript that enables developers to convert JavaScript objects and values into strings. It’s a necessary method that all developers should comprehend.
Understanding the toString() method is an important skill for JavaScript developers at any level, as it helps in manipulating strings and building complex applications in JavaScript.