Have you ever worked on a JavaScript project where you needed to manipulate strings? If so, you may have come across the trim method.
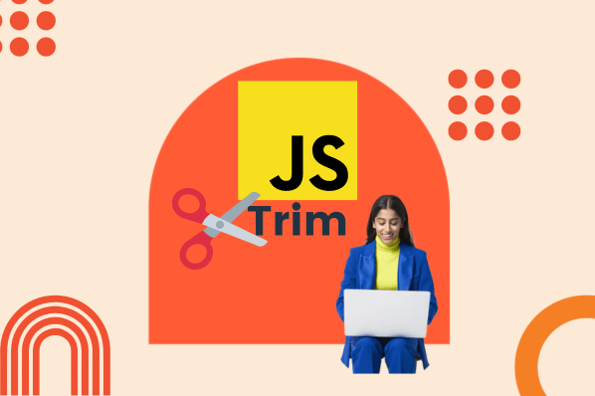
Trim is a powerful tool in JavaScript that allows you to remove whitespace characters from the beginning and end of a string. By using trim, you can improve the efficiency and elegance of your code, and ensure that your user input is properly formatted.
In this blog, we'll explore the basics and advanced techniques of JavaScript trim, examine real-life use cases, and provide tips to help you avoid common mistakes. So let's dive in and say goodbye to whitespace woes.
What is JavaScript trim?
The trim method in JavaScript is a built-in string method that removes whitespace characters from the beginning and end of a string. The whitespace characters include spaces, tabs, and newline characters.
The syntax of the trim method is as follows:
This method returns a new string with the leading and trailing whitespace characters removed. It's important to note that the original string is not modified by the trim method.
Here's an example of using the trim method:
In this example, the original string "str" contains leading and trailing whitespace characters, which are removed by the trim method. The resulting "trimmedStr" variable contains the same string without the whitespace characters.
When using the trim method, it's important to keep in mind that it only removes whitespace characters from the beginning and end of a string. If there are whitespace characters in the middle of the string, they will not be affected by the trim method.
Additionally, the trim method does not modify the original string, but instead returns a new string with the trimmed whitespace characters.
Advanced Techniques for Using JavaScript Trim
While the basic usage of the trim method is simple and straightforward, there are some advanced techniques that you can use to customize and extend the functionality of the method. Here are some examples below.
Regular Expressions and Trim
One advanced technique is to use regular expressions in conjunction with the trim method. Regular expressions are a powerful tool in JavaScript that allow you to search for and manipulate patterns within a string. By using regular expressions with the trim method, you can remove specific characters or patterns from the beginning and end of a string.
For example, let's say you want to remove all non-alphanumeric characters from a string using trim. You can accomplish this using regular expressions in the following way:
In this example, we first use the trim method to remove the leading and trailing whitespace characters from the string. Then, we use the replace method with a regular expression to replace all non-alphanumeric characters with an empty string.
Customizing the Trim Method
Another advanced technique is to create a custom trim method that allows you to remove specific characters from a string. This can be useful if you have a specific set of characters that you want to remove from a string, but don't want to use regular expressions.
Here's an example of how to create a custom trim method:
In this example, we define a "customTrim" method on the String prototype that takes a parameter characters which represents the set of characters to remove from the string. We then loop through the characters and use while loops to remove the specified characters from the beginning and end of the string. Finally, we return the resulting string with the trimmed characters removed.
Chaining Trim with Other String Methods
A final advanced technique is to chain the trim method with other string methods to create more complex string manipulations. For example, you can use the trim method in conjunction with the substring method to extract a specific portion of a string. Here's an example:
In this example, we first use the trim method to remove the whitespace characters from the beginning and end of the string. We then use the substring method to extract the first five characters of the resulting string. By chaining string methods together in this way, you can create powerful and flexible string manipulations.
By using these advanced techniques and combining them with the basic usage of the trim method, you can greatly enhance the functionality and efficiency of your JavaScript code.
Avoiding Common Mistakes With Trim
While the trim method is a powerful tool in JavaScript, it's important to use it correctly to avoid common mistakes. Here are some tips to help you avoid pitfalls when using trim:
Misusing Trim for Non-Whitespace Characters
One common mistake is to use the trim method to remove characters other than whitespace characters. Remember, the trim method is specifically designed to remove leading and trailing whitespace characters, and should not be used to remove other characters.
Using Trim Without Considering Browser Compatibility
Another mistake is to assume that the trim method is available in all web browsers. While the trim method is part of the ECMAScript 5 specification and is widely supported, some older browsers may not support it. To ensure maximum compatibility, use a polyfill or check if the trim method is available before using it in your code.
Overusing Trim and Its Variants
Finally, it's important to avoid overusing the trim method and its variants. While trimming whitespace characters can be useful in many cases, it's important to use it judiciously and only when necessary. Overusing trim can lead to unnecessary complexity and reduce the readability of your code.
The Future of Trim in JavaScript
Trimming whitespace characters from strings is a fundamental aspect of web development, and the trim method in JavaScript provides a powerful and flexible tool for accomplishing this task. By understanding the basics of the trim method, mastering its advanced techniques, and avoiding common mistakes, you can greatly enhance the efficiency and elegance of your JavaScript code.
As web development continues to evolve, the trim method in JavaScript is likely to see continued development and refinement. In the future, there are likely to be even more powerful ways to use trim and other string manipulation methods, so stay tuned.