JavaScript has been around for a long time and has made regular updates to improve upon its capabilities. Since its inception, it has garnered support in all modern browsers and is used in modern web development.
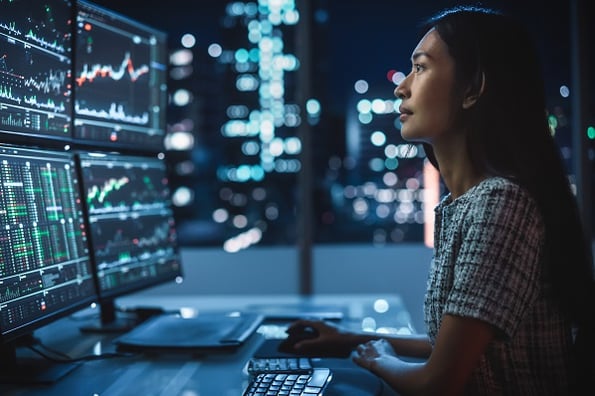
To further improve the functionality of JavaScript, developers have created extensions of the language through the development of JavaScript Libraries. One such library is jQuery, which is very powerful and dramatically improves the extensibility of JavaScript.
The syntax of jQuery is a bit different from standard JavaScript making it vital that you understand the separation between them.
In this post, we will be discussing a jQuery function called replaceWith(), including what it does and why it is important. We will also discuss how it can help improve your workflow by comparing it to the standard JavaScript approach.
What is the jQuery replaceWith() function?
In days of old, when web developers wanted to change content within their software conditionally, they had to use Javascript to refer to objects indirectly. When interacting with the document object model (DOM), JavaScript classifies each HTML element as a NODE. If we wanted to replace a node, we had to identify its parent node and then use it to point at one of its child nodes to change it dynamically.
Enter jQuery, a JavaScript library designed to enhance flexibility and improve functionality. Over time, the jQuery library continued to grow, adding new functions, methods, objects, and other features to the extensibility of JavaScript.
Eventually, developers added the replaceWith() function to simplify replacing DOM nodes with new nodes. We can directly target a DOM node and replace it with either a singular or even multiple new nodes using this new function.
Let's look at that in action, starting with how this is done in JavaScript.
JavaScript Replace Element
In JavaScript, to replace a DOM node, we would first need to create a reference to the target's parent node, then replace its child node. Let's see what that process looks like in standard JavaScript.
<ul id="KiaCars">
<li>Sorento</li>
<li>Seltos</li>
<li>Sportage</li>
</ul>
<p>
Click the button to replace the first item in the list.
</p>
<button onclick="myFunction()">Try it</button>
The above code sets us up with some basic HTML to manipulate; each HTML element is a node, and each element nested inside of another element is a child node. Next, we create a function called "myFunction" and add the code we need to complete this task.
function myFunction(){
}
In standard JavaScript, if you wanted to change an item within the list, you would first need to create the context for the target's parent node.
/* Create a new <li> element */
var listElem = document.createElement("li");
The above line of code selects the unordered list with an id of "KiaCars" which gives us the context we need to target the list item that we want to change. With the list targeted, we can now specify which of its child nodes we are targeting, and we do so using the following line of code.
/* Get the first child node of an <ul> element */
let cars = document.getElementById("KiaCars");
This line of code targets the first child node in the "item" list; we now have a reference of the node that we will change. Now, let's look at the standard JavaScript method for accomplishing this task.
/* Replace the first child node of <ul> with the newly created text node */
cars.replaceChild(listElem, cars.childNodes[0]);
The above code successfully replaces the first list item; the issue with this is that this process is somewhat convoluted. It creates an empty list item since the text within the list item is considered a node. So, to replace the text, we'd need to target the parent node, which is the new list item we just created.
/* Replace the text node of the new <li >with the newly created text node */
var newCar = document.createTextNode("Telluride");
listElem.replaceChild(newCar, listElem.childNodes[0]);
This can quickly become cumbersome and tedious to implement repeatedly, enter replaceWith() from the jQuery library.
How to Use the jQuery replaceWith() Function
The process of dynamically changing content within your web pages is made simpler using the replaceWith() function. This function gives developers the ability to change content with less code by directly targeting and replacing a node.
Let's take a look at how this works.
$(document).ready(function(){
var listElem = document.createElement("li");
$("button").click(function(){
$("li:first").replaceWith(listElem);
listElem.appendChild( document.createTextNode("Water"))
});
});
The syntax of jQuery is slightly different, but the above code accomplishes the same as the JavaScript code from earlier in this post. This code does three things; it waits for the DOM to finish loading, then it attaches an event listener to the button element and sets the action to take place when that event is fired.
The following code is the code that we want to focus on.
$("li:first").replaceWith(listElem);
With this line of code, we start by selecting the first list item, then directly replace it with a new empty list item node. After which, we then add a new text node to the list item replacing the text within that DOM element.
jQuery replaceWith() Best Practices
The jQuery function replaceWith() is a powerful tool that can remove a lot of the previously required heavy lifting of dynamically changing content on a webpage. However, there are a few essential points to remember from this post:
- To use jQuery, you will need to link to the jQuery library.
- replaceWith() is a jQuery function, making it valid JavaScript though it follows a different syntax.
- The simplicity afforded by the replaceWith() function is most noticeable in the ability to directly target the node that you want to change, replace, or modify.
- Using replaceWith() to replace a node will also remove any child nodes of the target.