React is a massive programming language and is a highly robust choice for developers, especially those who work in the full-stack field. It created a new paradigm for development choices by integrating the front-end markup with the backend logic. Combining the technologies used to develop software into a streamlined process improves development time.
This post will cover the React Staple known as JSX. You will discover what it is used for and how to leverage it in your React software development. You will also learn the syntax related to JSX and how it differs from JavaScript. Finally, you will see some code examples of JSX to help illuminate the subject matter and drive the topic home.
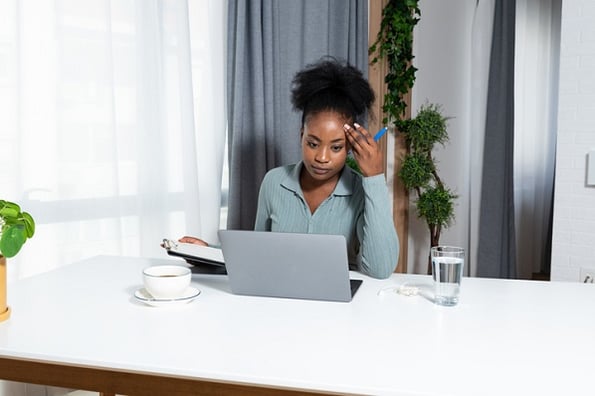
You can use the links below to navigate this post.
Without further ado, let’s get started.
What is JSX in React?
React is a component-driven software development language that runs in the Node.js runtime environment. The popularity of React JS is a result of its goal, which is to simplify the development process and provide a safer software environment.
One popular tool used to this end is JSX, a JavaScript Syntax extension used to expedite and further simplify React development. Because it is an extension of JavaScript, any valid JavaScript expression is considered valid code, further extending its benefits. It is worth noting that while useful JS expressions are useful JSX, the reverse is not true. Check out the next video to learn a little more about this concept.
The syntax for JSX looks like a mixture of JavaScript and HTML. However, JSX must be compiled into JS for the software to work correctly. Therefore, JSX expressions are converted to strings and compiled into the JS equivalent.
These features and behaviors result in a language that can improve development but is also very strict about syntax. Let’s discuss the differences between JS and JSX to illuminate the syntax and behavior of JSX.
JSX Vs. JS
As mentioned above, the syntax for JSX and JS are different, and since JSX is compiled into JS, its syntax must be strict. The result of JSX compilation is JS that communicates with React to create elements. In the end, each JSX expression results in a React.createElement() function call, which makes the elements structure. The image below is a good visual representation of what JSX actually is.
Next, let’s look at the syntax for creating a JSX element tag in the following code.
const element = <h1>All of time and space, you interested?</h1>;
The above code is neither a string nor HTML. Instead, it is an expression stored in a variable that will be compiled into a string and then translated to JS, creating a React element.
Thanks to JSX being an extension of JS, you can perform several JS expressions within your JSX. First, let’s look at an example of using a variable inside your JSX file to create more dynamic code.
const name = 'The Doctor';
const element = <h1>Hello, I’m {name}</h1>;
The above lines of code create a variable with the string value “The Doctor” which is then used inside the JSX expression. The curly brackets are used for interpolation, which supports the creation of highly dynamic code. When this code is compiled, the variable will be replaced with whatever value it holds.
Now let’s look at an example of how this same code would look in JavaScript.
const name = 'The Doctor';
const element = `<h1>Hello, I’m ${name}</h1>`;
let main = document.querySelector(“main”);
main.innerHTML = element;
While this is a simple example, it exposes two issues that JSX works to solve. The first is simplifying code; reducing complexity means cleaner human-readable code. The second concern is data injection, a malicious attempt to compromise your software.
With JSX, the concern of injection attacks — also called XSS (Cross-site-scripting) — is alleviated because all embedded values are escaped before rending.
Next, let’s look at some examples of using JSX with and without JS expressions.
How To Use JSX
Typically, JSX is used in conjunction with React; however, they are not interdependent. Both can be used independently of each other. However, JavaScript is required by JSX to work correctly; this is because JSX is nothing more than syntactic sugar. That is to say that all JSX does is extend the syntax of JS to help create software more efficiently.
Next, let’s examine the JavaScript equivalent to a JSX element. With JSX, any created element is a JavaScript object with keys, values, properties, and methods.
Let’s compare the following JSX example with its JS equal.
const element = (
<h1>
Hello, I’m The Doctor!
</h1>
);
The above code is identical to the following JS code.
const element = document.createElement('h1', 'Hello, I’m The Doctor!');
As you can see, you will likely find yourself using standard JS code with your JSX. To that end, it’s essential to understand how to do that. So let’s look at that next.
let userInput = "The Doctor";
function formatName(userInput) {
if (userInput) {
return `Hello, ${userInput}!`;
}
return "Hello, Doctor Strang-er.";
}
const element = (
<h1>
{formatName(userInput)}
</h1>
);
The code block above showcases standard JS in conjunction with JSX, specifically how you can use standard JS interpolation and if statements. You can also nest child elements within a JSX element, allowing you to modularize your content. By bringing these concepts together, you can create very robust software ready for anything.
Getting Started Using JSX in Your Software Development
The JSX syntax extension is a large subject with a lot to learn, and you have learned the basics needed to get started. However, as mentioned above, JSX is most commonly used with React programming due to React’s structure and intended use.
While it is not required, learning to use JSX in React is probably the best way to build your understanding of JSX and how it can be used. Moving forward, you may find it advantageous to practice JSX with services like CodePen and test the limitations of JSX syntax.