Node.js is an open-source platform that allows developers to create powerful JavaScript applications on the server side. Harnessing asynchronous operations, it allows developers to build real-time, data-driven apps with remarkable performance capabilities.
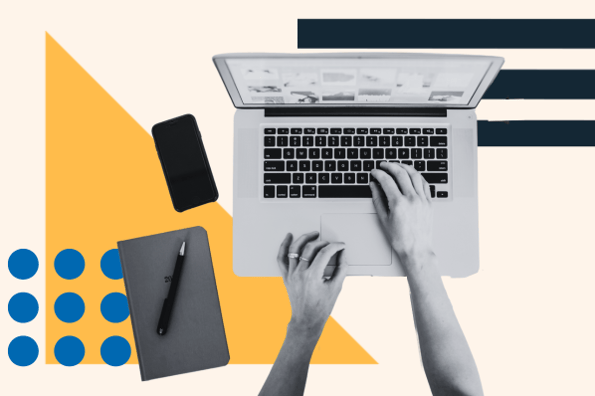
MongoDB is an open-source document database system. Thanks to its simplistic user interface (UI) and ability to manage large datasets, MongoDB is a popular technology among developers.
Coupling Node.js and MongoDB together is great for applications like chatbots, social media apps, or task management apps. They support real-time data and retrieval. In this blog post, we’ll explore how Node.js works and how it can be used with MongoDB to build highly scalable applications.
Table of Contents
- Node.js and JavaScript
- What is Node.js MongoDB?
- Node.js MongoDB Examples
- Setting Up a Node.JS Program Using MongoDB
Node.js and JavaScript
There’s often a misconception that Node.js is another language that replaced JavaScript. Node.js is actually a tool designed to bolster JavaScript capabilities. Let’s take a look at what the two are and how they are different.
Node.js is a highly efficient software that allows developers to access and execute JavaScript code on the server side. The server is an essential component for any website or web application. With this powerful tool, developers can design functional websites with ease.
Node.js is perfect for constructing web applications that require the simultaneous processing of multiple tasks, like accommodating many users at the same time. This is because it employs “asynchronous operations” — a type of programming that allows the application to complete various tasks at once.
JavaScript is a powerful programming language that engages your website visitors. Whether it be an animated slider showcasing images of your products or services, drop-down menus, or even buttons — these are all dynamic features powered by JavaScript.
While JavaScript and Node.js are related, it’s important to know that their functionalities are different. JavaScript is a programming language used to make interactive web pages. Node.js is a runtime environment that allows JavaScript to be run on the server.
Check out a few of their key differences below.
Node.js: How does it work?
Node.js, built on the Google V8 JavaScript engine, creates an event loop in which all incoming requests are monitored as soon as it’s launched. This event loop enables the node to process multiple requests simultaneously and calls a worker thread whenever a request is received.
The response generated by the thread is then sent back to the same event loop for further processing — making Node.js invaluable for many companies such as Netflix, NASA, Linkedin, and others.
Node.js Advantages
Let’s check out some compelling benefits that make Node.js a popular choice among developers:
- Scalability. Node.js supports scaling applications. Servers can be added to the application quickly as traffic increases.
- Support. If you need support using Node.js, there’s a large community online full of helpful tutorials, libraries, and reusable modules.
- Proficiency. Node.js is highly proficient. This is because it uses a non-blocking, event-driven I/O model to support its ability to handle multiple requests at once.
- Flexibility. Node.js is a flexible tool as it can be run on multiple platforms like Windows, macOS, and Linux.
- Straightforward. Since Node.js works with the widely used language JavaScript, it’s relatively easy to learn.
Node.js Disadvantages
Node.js is definitely a popular option for developing applications. However, it’s important to consider the potential disadvantages before you start to build:
- Complexity. Node.js leverages asynchronous techniques that include functions like callbacks and promises. Your team must acknowledge how this can make your code hard to read in the future due to its complexities.
- Constant change. Developers may be forced to frequently update their code base when using Node.js because its platform is constantly changing.
- Memory intensiveness. Because Node.js is memory intensive, it may scare developers from building with it because they have better options with other server-side platforms.
- No multithreading. Unlike Java or C++, Node.js does not support multithreading programming. This may present issues for applications that are CPU-bound.
What is Node.js MongoDB?
We have checked out how JavaScript and Node.js are the perfect duo to build full-stack applications for data-driven apps. However, we have yet to discuss where that data will be stored. That’s where MongoDB comes in.
MongoDB is a document-oriented NoSQL database that offers flexible and scalable features. MongoDB stores data in collections of documents, similar to JSON objects, as opposed to the typical relational databases that keep data in tables with predetermined rows and columns.
Node.js and MongoDB are two separate technologies that complement each other to build powerful applications that can store and manipulate data.
In more detail, Node.js’ asynchronous, event-driven programming model, coupled with MongoDB’s dynamic and schema-less approach to storing and managing data, simplifies the application’s ability to manage data.
Node.js MongoDB Examples
ChatBot
If your team wants to feature a chatbot on an application, consider using MongoDB and Node.js. Users can receive instant messages and notifications. This data can be stored and managed in a database like MongoDB. Users sometimes send multiple requests or messages at a time.
Node.js model can easily handle this as its asynchronous approach supports handling simultaneous requests.
Ecommerce Website
Using Node.js and MongoDB is the perfect combo for building a high-performing ecommerce website. Think about a large retailer that specializes in selling shoes. Their inventory may consist of hundreds of different styles.
They can use JavaScript to create their UI, MongoDB to provide a flexible schema design that stores and manages their inventory data, and Node.js to take care of the backend logic while effortlessly working the user requests on the website.
IoT Application
Companies like Samsung, GE, and LG have popularized Bluetooth appliances like washers and dryers. For instance, GE has an application called SmartHQ that allows users to connect their appliances to their phones via Bluetooth.
If your team wants to offer smart appliances, Node.js and MongoDB are great options to add to your tech stack.
MongoDB is perfect for hosting IoT applications due to its reliable storage and retrieval of large amounts of unstructured data from connected devices. Node.js, on the other hand, comes in handy when it’s time to process application data or communicate with connected devices.
These two technologies make a formidable pair to help you build robust IoT solutions quickly and efficiently.
Setting Up a Node.js Program Using MongoDB
Now that we know what Node.js and MongoDB are, let’s dive into the nitty gritty. Here’s your guide to setting up Node.js using MongoDB for any website or application.
1. Download Node.js.
The first step is to download the installer package from https://nodejs.org/en. Follow the prompts to download the package with the file extension .pkg.
Verify that you have installed it correctly by navigating to your terminal and running the command: node –v
2. Download the MongoDB driver for Node.js.
This driver uses npm, which allows your Node.js program to talk to your MongoDB database. Navigate to your terminal and run the following command: npm install MongoDB.
3. Create a project folder.
This is where all of your files and dependencies will be housed for your project.
Navigate to the terminal and run the command: mkdir node-mongodb-project. Change into the project by running the command: cd node-mongodb-project.
Create a packjage.json file inside of your project by running the command: npm init -y
The y flag will give it default values. If you want to customize, leave the -y out.
4. Create a free MongoDB Atlas account.
The easiest way to use MongoDB is to create a MongoDB Atlas account. You can get started for free!
If you need help creating a cluster, check out the video from MongoDB.
Now, you can create a cluster and load it with mock data of your choice. While still in Atlas, use the cluster connection wizard to get your cluster ready to connect to your Node.js application.
Be sure to copy your connection string for the next section.
5. Connect the database with the Node.js application.
Enter this line of code to connect the application to the database.
After connecting the application with the database, create your asynchronous function names main() that will connect to your MongoDB cluster.
Next, you will add your connection string to your main() function.
Be sure to update your username and password with what you established during the connection wizard setup. Remember, this is not the same information as your MongoDB Atlas login.
Pay close attention to your try function. Notice it has a console.log statement. If your database is successfully connected with no errors, you will see “Database Created” printed in your console.
This is what your entire main() function looks like with code.
6. Run your Node.js script.
Now, it’s time to print our code. Run the following command to print your data to the console: node <file-name.js>
7. Node.js MongoDB Tutorial
For a further explanation of this process, check out this cool tutorial that walks you through how to connect Node.js MongoDB.
Start using Node.js MongoDB today.
Learning Node.js and MongoDB is a fast and easy process, as the two technologies are very intuitive and user-friendly. Whether you’re a beginner or a seasoned programmer, Node.js and MongoDB will help make your development workflow quick and easy.