Ouroboros is the snake eating its tail; that's what I think of when I think of Python loops — but only because I'm overly visual.
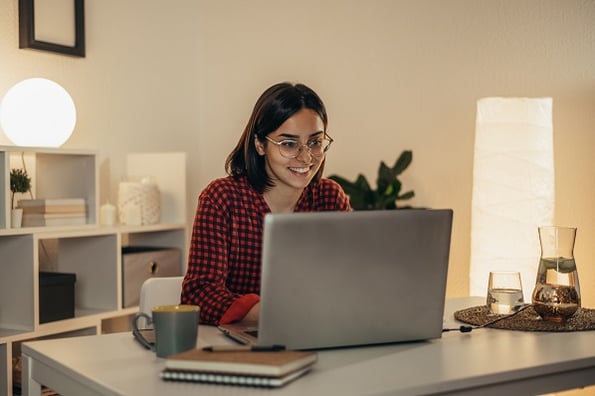
The truth is that it's not really like that. Python is a powerful programming language, and loops are just one of its features.
Today, we will dive into Python for loops, syntax, keywords, and more. There is a lot to cover, but I'll keep it as short and sweet as possible.
So without further ado, let's jump right into this.
How to Create a Loop in Python
Creating a for loop sounds like a daunting task, but Python's syntax makes this easier. The Python language uses a human-readable syntax, making it easy to follow and use. So, let's check out the syntax for a Python for loop.
carCompanies = ["Ford", "Volvo", "Chrysler"]
for x in carCompanies:
print(x)
Let's go over what the code above actually does and how it works one step at a time.
The first line of code creates a variable named carCompanies and assigns it a value of an array; after that, it'll start the for loop and iterate through the carCompanies array. The keyword print prints the value of x each time the loop runs — x being equal to the current item in the collection.
The Else Statement
In addition, the for loop offers the else statement, which will run in the condition that the for loop can’t. An example of this would be if the other statement would execute after completing the maximum number of iterations.
for x in range(6):
print(x)
else:
print("All Done!")
After the above loop runs six times, the else block would be executed and print the text, "All Done!" to the screen.
Powerful Nested Loops
Finally, let's discuss the recursive awesomeness of nested loops, which add a new level of dynamic complexity. With nested loops, the inner loop runs to completion for each time the outer loop runs; let's go over how this works.
carCompanies = ["Ford", "Chrysler", "Volkswagen"]
models = ["Taurus", "New Yorker", "Passat"]
for x in carCompanies:
for y in models:
print(x, y)
The above code would run the outer loop, and during that loop, the inner loop would run till it finishes, then the outer one would run again. That would result in the following being printed to the screen.
Ford Taurus
Ford New Yorker
Ford Passat
The second iteration of the outer loop would result in:
Chrysler Taurus
Chrysler New Yorker
Chrysler Passat
The power of loops doesn't end there, and you can easily loop through strings in almost the same way; let's take a look at that below.
Looping Through a String
Looping through a string is easy to accomplish with Python, and there is more than one way to do it. Lets look at this done directly using a string.
for x in "Ford":
print(x)
The output of the above line of code results in the text, Ford, printed to the screen one letter at a time, each on a new line. Although you can also accomplish this with a string stored in a variable, the result is the same behavior, but this is the most common way to do it.
car = "Ford"
for x in "Ford":
print(x)
The code above results in the same output as the previous approach; this gives us a lot of flexibility with how dynamic our code can be. Storing data in variables outside of our loops allows us to modify that data before modifying it. Furthermore, Python also offers the range function to create an integer list; let's look at that next.
Using range() With Your “for” Loop
Python has a built-in function called range which creates a numerical range of numbers, it takes one required parameter and two optional parameters. By default, the range starts at zero and increments by one number at a time. However, the second and third optional parameters allow you to modify its starting point and how many numbers it will increment.
Next, let's take a quick look at what this looks like and how it works.
for x in range(2, 30, 3):
print(x)
In the example above, the function call creates a range starting at 2, ending at 29, and incrementing by 3. Next, let's look at the range function declared without the second and third parameters.
for x in range(6):
print(x)
This code creates a range from zero to five — omitting the final number in the range — consisting of six numbers.
In Python, you can even step out of our loop based on provided conditions or end the loop entirely.
Using the Python “break” and “pass” Statement
The Python break statement breaks out of a loop completely, which you might want to do after meeting a specific condition or set of conditions. The syntax for this is straightforward. It's just the keyword, break, placed anywhere in the loop.
for x in "Ford":
print(x)
if x == "r":
break
In the example above, as the loop iterates through the string Ford, it will compare the current letter in the string with the string provided — if equal, end the loop. You can create any condition or more than one condition and use the break statement more than once in a loop. A benefit of using more than one break is checking for different kinds of errors in addition to specific conditions.
Python offers two other keywords for loops that expand the functionality of our for loop, the continue and pass statements. The continue statement is used similarly to the break statement, except it moves into the next iteration instead of ending the loop.
The pass statement offered by Python moves through a loop with no statement or action inside. If you create an empty for loop, the code will throw an error — making the keyword very useful.
Final Takeaways: Python “for” Loops
There is a lot of power in Python and for loops are one example of where that power originates. But there is a lot that goes into loops, so let's look at the essential points.
- Else: This runs if the loop cannot.
- Nested loops: The inner loop runs from start to finish for each iteration of the outer loop.
- Keywords in for loops: A few keywords can be used inside a loop to add flexibility and control — such as break, continue, and pass.
Python for loops range in complexity and power based on how they are used. You can create loops to serve all kinds of needs and hopefully, this post has illuminated the concepts of for loops. The information found here should hopefully help you come up with all kinds of creative and powerful uses.