Python lists are a powerful data type that allows you to create very powerful collections of data. We can use lists to collect and organize everything from users to settings and even information like dictionaries.
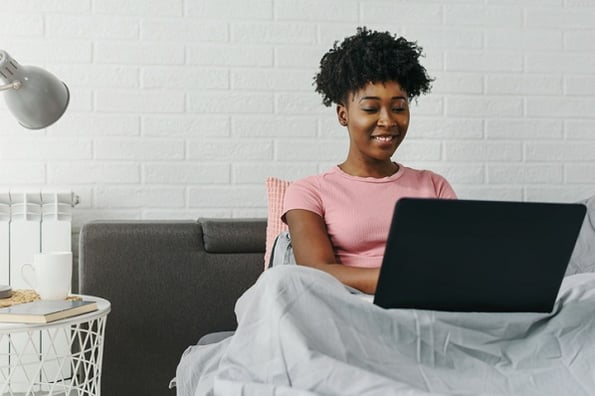
In this post, we will be discussing in-depth what python lists are, how to use them, and the tools provided for interacting with them. And, what the heck? Why not do it with a list of snakes and varying information related to them.
There's a lot to cover here so, let's slither right into it.
What is a Python list?
A Python list is a native data type built into the python programming language. In Python, lists are similar to arrays found in many other languages; however, they are treated as variables with many items in Python. Nevertheless, lists are still considered a data type for storing data collections.
Python has four built-in data types used for storing data collections: list, tuple, set, and dictionary. These data types differ in function, performance, and malleability.
For now, our focus will be on Python lists, how they work, and how they can be manipulated to serve our needs. But, first, let's look at the basics behind what a list is and what it is capable of.
A Python list is:
1. Ordered: This means that items inside a list are indexed and accessed by their indexed location.
2. Editable: The items inside a list can be edited, and items can be added or removed from the list.
3. Dynamic: Lists can contain various data types and even objects; this means that they can also support multidimensional sets of data, much like an array or many objects.
4. Non-Unique: Essentially, this means that the list can contain duplicate items without throwing an error.
If you are familiar with programming, this may seem like a Python list is the same as, say, an array in some other languages; let's be clear about this, they are very different. Python has a native array data type for that exact reason.
Creating a Python List
Creating a Python list is very simple, and with not one, but two ways to create them, we have a lot more control over how and where we can instantiate a list. The first method for creating a Python list is using the bracket — [ ] — notation; let's see how we accomplish this.
snekList = ["Boa", "Python", "Danger Noodle", "Garter"]
That's it, and the bracket notation is fairly straightforward; you simply wrap the contents you want to add to your list with the square brackets. The second way is using the list constructor, and the syntax for it is a bit different so let's look at that next.
snekList = list(("Boa", "Python", "Danger Noodle", "Garter"))
In this case, the output of both lines of code would amount to the same output and would be identical in every way.
print(snekList)
Would print the following output:
["Boa", "Python", "Danger Noodle", "Garter"]
However, while that is sometimes true, there are caveats to consider when working with more dynamic data collections. Such as, converting a dictionary data collection using the list constructor would strip the data down to only the keys from that collection. In contrast, the bracket notation would keep all keys and values as one item in the list.
Let's take the previous snekList list and convert it into a dictionary object.
snekList = {"Boa": "Risky Noodle", "Python": "Risky Noodle", "Cobra": "Danger Noodle", "Garter":"Safe Noodle"}
If the above dictionary was converted to a list using the constructor, the resulting output would filter out all values in this dictionary.
list(snekList)
Instead, it would create a list of those snakes — keys — and remove their respective safety designation — values — printing the result of this action would create an output of the following.
print(snekList)
["Boa", "Python", "Cobra", "Garter"]
This result contrasts with the bracket notation, which takes this dictionary object and preserves it as a single item in the list. So let's see what that looks like, next.
[snekList]
print(snekList)
[{"Boa": "Risky Noodle", "Python": "Risky Noodle", "Cobra": "Danger Noodle", "Garter":"Safe Noodle"}]
We can now dive into this concept a little more; next, let's review the data types that Python lists are compatible with.
Python List Data Types
Python lists are much friendlier with mixing data types than the others are. A Python list lets you have lists that contain many items of all the same type or a mixture of different data types. Furthermore, lists are compatible with any data type, including integers, strings, booleans, nested lists, and objects.
What this means is that they have the potential to be a very powerful tool in your Python knowledge bank. Let's build on that tool further by looking at how you can manipulate your Python lists.
Manipulating Python Lists
Python has some native functions that we can use to help you assert better control over how you can use them. Let's take a look at some of the most commonly used functions as they relate to lists.
Python Native Functions
The most relevant functions are the length function and the type functions, which do what they sound like. For example, in the case of length, it calculates the number of items within a list.
It is important to note that if you use this on a list with dictionaries, nested lists, or similar, the function will only return each top-level item. That is to say that the length function will not look at or count items inside of nested lists, only the nested list.
Let's look at how the len() function works with lists, using our snekList from above.
snekList = ["Boa", "Python", "Garter"]
print(len(snekList))
The above code results in the output 3, while the code below will also result in the output of 3.
snekList = [{"Boa", "Python", "Garter"},{"Risky Noodle", "Risky Noodle", "Safe Noodle"},"Sneks and their danger level" ]
The next function on the agenda is the type function, which takes an object and determines its data type. There isn't much behind how this works concerning lists, but it is very handy for confirming that a list is actually of the type list. Furthermore, the type of items within the list does not affect its type; let's look at this in action.
Both of these list declarations evaluate to the same result.
snekList = ["Boa", "Python", "Garter"]
snekList = [{"Boa", "Python", "Garter"},{"Risky Noodle", "Risky Noodle", "Safe Noodle"},"Sneks and their danger level" ]
If you print out the type of either of those declarations you will get identical output from both.
print(type(snekList))
<class 'list'>
Python Operators
Native Python operators open an entirely new dynamic for interacting with python lists; you can use them to add, remove, concatenate, merge, print, and more. First, let's go over some of the most popular operators used with lists.
The add + — concatenate — operator is used to merge one or more lists, creating one longer list out of the combination of the target lists.
snekList = ["Boa", "Python", "Garter"]
print(snekList + ["6 feet", "4 feet", "1 foot"])
The above code would result in the following output:
snekList = ["Boa", "Python", "Garter", "6 feet", "4 feet", "1 foot"]
Next up is the slice : — range — operator, which declares a range of items from your list based on their index location. In that way, you would access the items the same way you access an item in your list, using the bracket notation. Let's see what this looks like in action.
print(snekList[0:4])
The above line of code would return the output of the first three items in the list, starting at the index of 0 and ending before the index of 4. In other words, the slice operator starting point is inclusive, and the endpoint is exclusive.
["Boa", "Python", "Garter"]
The multiply * — repeat — operator is used to dictate a number of times that a specific action is to repeat. In the case of a list, this allows a multitude of functionality concerning manipulating list items. Let's print out an example of this.
print(snekList * 3)
This line of code would print out the result of the provided equation, a list that contains the same list items repeated sequentially three times.
["Boa", "Python", "Garter", "Boa", "Python", "Garter", "Boa", "Python", "Garter"]
The assign = — change — operator will directly change the value of a list item, list items, or even the entire list. Let's look at an example of this in practice assuming the snekList items.
snekList = ["Boa", "Python", "Garter"]
snekList = snekList * 3
The above line of code takes the original value of the snekList, multiplies it by three, and assigns the value of that equation to itself. The resulting output is that the list now contains nine items, the first three repeated three times.
print(snekList)
Would result in the following output.
["Boa", "Python", "Garter", "Boa", "Python", "Garter", "Boa", "Python", "Garter"]
Python List Methods
As is common with most programming languages, a method is typically called on the object you want it to run against. Many methods — but not all of them — can take an argument when invoking the method. Calling a method is simple and usually done by chaining it onto the object using a "." as the link between them.
object.method(argument)
Now that we've covered that, let's take a look at the syntax of a method call as it pertains to a python list. First, let's look at three of the most popular methods for interacting with and modifying a Python list.
If you only want to add a new item to the end of your list, you can use the append method to add a new item.
snekList = ["Boa", "Python", "Garter"]
snekList.append("Cobra")
The above line of code appends a new string onto the list; the resulting output — if printed to the screen — would look like the following.
print(snekList)
["Boa", "Python", "Garter", "Cobra"]
The next most popular method is the index method used to return the index of a specified value. For example, let's see what happens if we target the string item "Cobra".
snekList.index("Cobra")
The returned output of this line of code would be the index value of the provided list item; in this case, it would be index 3.
Finally, let's look at the remove method used to target a specified list item and remove it from the list. This method allows for a lot of control, provided you know exactly what you need to target and are sure it is on the list. Let's see how to use that before wrapping up this post.
snekList.remove("Cobra")
The above line successfully removes the item that matches the provided target. In this case, the list item matches the string "Cobra." Therefore, printing the list after this method is called would result in the output below.
print(snekList)
["Boa", "Python", "Garter"]
Final Thoughts on Using Python Lists
There is a lot of power behind the use of Python lists and this tool can bolster your programming skills when you are armed with the right knowledge. Throughout this post, we have covered a lot of content and concepts, and as such, it is a lot to take in. In that vein, it is important to keep in mind that we did not cover all of the methods provided, and there is much more to using these than any one post could cover.
Here is a list of other methods you can try using and see what you come up with.
- .clear(): Removes all the elements from the list
- .copy(): Returns a copy of the list
- .count(): Returns the number of elements with the specified value
- .extend(): Add the elements of a list (or any iterable), to the end of the current list
- .insert(): Adds an element at the specified position
- .pop(): Removes the element at the specified position
- .reverse(): Reverses the order of the list
- .sort(): Sorts the list
With that said, it is highly recommended to play around with the features, methods, functions, and operators to solidify your knowledge. It is also encouraged to mix and match to see how they work and how you can use them in your programming.