Python and strings these terms together conjure an image of a snake made of strings, which isn't too far from the truth.
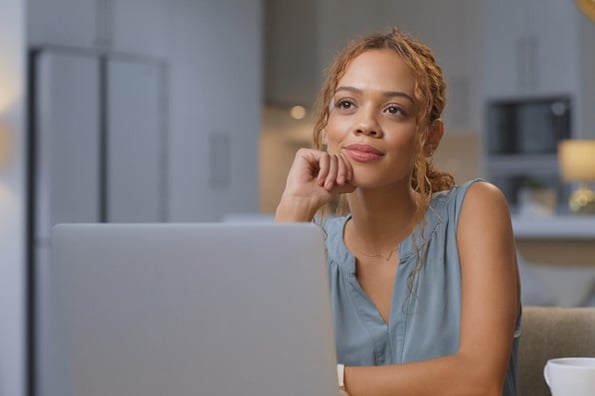
This post will cover the basics of Python strings; you will learn about the various syntax you can use to create strings and how to work with them meaningfully. You will also learn about the different operations you can perform against strings and ways to format them. All of these concepts will come with code blocks to show examples of each.
Without further ado, let's slither right in!
What are strings in Python?
In most programming languages, strings are just a collection of characters stored within a variable for various uses. Python strings are no different and offer several benefits, such as creating messages for clients to improve their user experience. The Python language is very robust, and so is the subject of Python strings.
Strings can be used for much more than that; they can also provide ways to monitor and control the behavior of the software application itself. Furthermore, they are also frequently used to help facilitate further development of your software and support testing and debugging tasks. The next video helps further illuminate the subject of Python strings.
Now that you know some different ways strings are used in Python, let's look at the syntax of defining strings.
Define String Python
There are multiple ways to define strings in Python. They have one thing in common: you use quotation marks to define your strings. There are three different syntaxes for defining strings, and when you use them together, you can nest them to add quotes to the string itself. In the code below, you can see all three ways to define a Python string.
Each of the three examples above is a valid string expression and gets interpreted as having the same value. It is interesting to note that the first two are often used together to create strings with quotes in them. In Python, the length of a string is dictated by the number of characters, including special characters and spaces. This next image shows the way Python string lengths are calculated.
Python String Examples
There is much more to Python strings than just the above, such as nesting quotes within strings and even multiline strings. Let's look at some examples of both in the code blocks below.
The above strings would also be evaluated as equal in value and result in the same string when printed to the console or terminal. The inner quotes will be preserved, meaning if you define your string with single quotes and use double quotes inside, the string will print with double quotes. Passing these variables into the print function results in the following strings.
The above shows that the printed string will reflect the quoted text's quotation marks.
Triple Quote Strings
The third string from both examples uses three quotes — either three single or three double — a syntax primarily used for multiline string or formatting.
These strings share the same quality regarding how the quotation marks get preserved when quoting text within a string. Triple quotes for defining strings will also allow you to create a string with a double-quoted text without needing to escape apostrophes within.
String Character Escaping
Sometimes you will find yourself working with a string that may have invalid characters; in this case, you'll need to escape the incompatible characters. Escaping characters within strings are simple and only requires using a backslash before the target character. Let's look at how to do this in the following examples.
The strings above all have a single quote within the quoted text. These single quotes must escape preventing the unintentional breaking out of the string. If you do not escape special characters in your strings, the code will cause an error.
You can also escape quotes within a string by using an escape at each quotation mark shown in the example below.
Each of the strings above use either double quotes or triple quotes. Because of this format, the double escape signals a different translation. With this format, the text within the two backslashes gets preserved and will not need to escape the single quote. Let's look at an example of another string with a special character text.
In the above example, escaping the symbol' \x61', which gets translated into the character 'a', an essential distinction as special characters, will become helpful at some point. If you don't escape the character, it gets printed as it is in the string.
Printing Raw Strings
You can also print raw strings, which will print anything within a string exactly as it is written, that includes escaped special characters. The backslash will also get printed if you escape a quote within a raw string.
Raw strings are useful for debugging strings and values within your software and can help identify and eliminate potential errors.
Python String Operations
There are many ways to interact with Python strings, such as finding substrings and even string concatenation. However, it is important to note that Python strings are immutable, meaning you cannot change strings. You cannot change or delete individual string characters, but you can reassign a string variable with a new value.
Let's look at some code examples of how you can interact with strings.
Accessing Strings and String Characters
There are multiple ways to access strings and the characters within, and you will likely need to do this at some point.
Regardless of how you do it, the process will likely all have something in common: the concept of iterating over characters. One way to do this is with loops, which allow you to iterate through the characters in the string. Let's check that out next.
Iterating Through Strings
You can also use loops to iterate over the characters within a string. Doing this gives you the ability to interact with the individual characters.
Substring Checks
Checking for a string within a string is typically referred to as either substring checks or string membership checks. These checks do behind-the-scenes iteration to find the target string. This is useful as you will not need to iterate through the string manually. Performing membership tests can save you time and help keep your code clean and uncluttered.
It is worth noting that this type of membership check is case-sensitive. Searching for a capital letter will only return true if that letter is found as an uppercase letter.
Concatenating Strings
Sometimes, you will find yourself in a situation where the best approach is to combine two or more strings into a new value. Concatenation is the process of combining two or more strings or characters to create new strings. Because strings are immutable, you can't alter the value of a string, but you can overwrite it, and string concatenation is one common way this is done.
This pattern is a low-level recursive pattern for updating strings with new values and is also used to build custom messages for users. You can also do real-time concatenation, such as concatenating on the fly during a call to the print function.
These code blocks both show valid ways to concatenate strings together, and with string concatenation, there is a lot you can do with user messages, notes, comments, and much more.
Moving Forward With Python Strings
In this post, you have learned all of the beginner basics of Python strings, how they work and what you can do with them. Moving forward, you can practice your newfound skills by using strings in your code to perform various tasks. You can also learn about Python string methods, a vast subject with a lot you can learn.
As always, the best thing you can do is practice all the information you have learned by using this information in your software development.