Interested in getting started with WordPress plugin development?
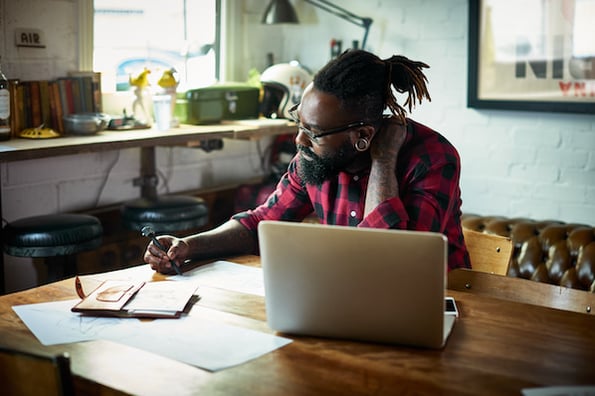
Whether you want to create a custom plugin for your own site or release your plugin publicly, this WordPress plugin development tutorial will help you learn how to begin. Plus, we’ll walk you through the steps you’ll take to start using it on your WordPress site and/or sharing it with the world.
To start, we'll cover a general introduction to WordPress plugin development, including the basic elements of WordPress plugins. Then, we'll go through a step-by-step tutorial on how to make a WordPress plugin and install it on a live site.
Here's everything you'll find in our WordPress plugin development tutorial:
What skills do you need for WordPress plugin development?
You don't need to be an expert developer to follow this WordPress plugin development tutorial. However, you’ll need some coding knowledge to successfully create a plugin.
WordPress plugins are primarily written in PHP, so a basic understanding of how PHP works is one of the most essential elements for plugin development.
Beyond that, you'll need some basic HTML and CSS knowledge, which will help you control your plugin's output. JavaScript can also be important, and is essential if you want to work with the new block-based approach that WordPress introduced in WordPress 5.0 with the block editor (AKA Gutenberg).
With that being said, if you don't feel comfortable working in JavaScript, you can avoid it and use the older shortcode-based approach.
Speaking of shortcodes, let's talk about the basic building blocks of WordPress plugin development.
Understanding the Basics of WordPress Plugin Development
If you want to customize your WordPress site, you can't just go ahead and edit the core WordPress files. This is because those files are completely overwritten every time you update your WordPress site.
To get around this, WordPress plugins let you add, modify, and extend functionality using one or more PHP functions.
To create a WordPress plugin, you'll rely on a few basic elements:
- Hooks (actions and filters)
- Blocks
- Shortcodes
- Widgets
Given WordPress' new focus on the Gutenberg editor, however, shortcodes and widgets are being phased out and are not as important as they once were.
Hooks (Actions and Filters)
Hooks are what allow you to interact with different parts of WordPress without needing to edit the core files.
For example, a hook lets you execute a code function at a certain point in time or on a certain part of the website.
WordPress hooks come in two primary formats - action hooks and filter hooks:
- Action hooks - these let you add a new process to WordPress. More specifically, they allow you to run a function at a specific point in time on the WordPress site. For instance, an action hook would enable you to run something when a user saves a post.
- Filter hooks - these let you modify a process to change or manipulate its data without needing to edit the source itself. More specifically, they allow you to retrieve and modify data before saving it to the database or rendering code on the front-end.
To review:
- Action hooks let you do things.
- Filter hooks let you change things.
Action Hook Example
Here's an example of an action hook:
This action executes the hello_dolly function when admin_notices is called.
In plain language, this is what allows the Hello Dolly plugin to display song lyrics in the WordPress admin interface.
Here's another example from the Holly Dolly plugin:
This action hook lets the plugin save its CSS to the <head> section of pages in the WordPress dashboard using the admin_head hook.
Filter Hook Example
Here's an example of a filter hook:
This code modifies the title of blog posts to be all uppercase. The filter hook grabs the title of the post using the_title and then executes the upper_case title function to modify the existing title to be all uppercase.
Blocks
Blocks have been an important part of how WordPress plugins work since the block editor ("Gutenberg") was released in WordPress 5.0.
Depending on your plugin's functionality, you might want to consider using blocks to let users interact with your plugin, such as inserting plugin content or even creating content using your plugin.
However, in order to utilize blocks in your plugin, you'll need to have a solid understanding of JavaScript, Node.js, React, and Redux, which has turned off some developers since WordPress plugin development used to be much more PHP-focused.
You can always start without blocks and add them later if it fits with your plugin.
The Block Editor Handbook is the best place to get started. There's also a tutorial to help you create your first block.
Shortcodes
Shortcodes are the "old" way of giving users a way to interact with your plugin.
In the past, pretty much all WordPress plugins relied on shortcodes to provide users with the ability to insert plugin content into a post or page. However, in the "modern" WordPress way, plugins can opt to use blocks instead of shortcodes.
With that being said, many plugins currently support both blocks and shortcodes, so you'll want to think about including shortcode support in your plugin.
You can add shortcodes using theadd_shortcode function.
Here's an example:
When a user adds the [datetoday] shortcode to the editor, it will display the current year. This works by having the [datetoday] shortcode return the year_shortcode function.
Widgets
Widgets are an older WordPress concept that you might not want to integrate in plugins going forward. This is because WordPress 5.8 replaced the older widget system with blocks.
However, if a site is using the Classic Widgets plugin, the site owner will still be able to use the older widget system, so you might want to consider building widget compatibility into your plugin.
You can do this by working with the WP_Widget class.
Components of a WordPress Plugin
A WordPress plugin will have a few different components, some of which are required no matter what and some of which will depend on the complexity of your plugin.
At a bare minimum, a plugin needs these elements.
- Main plugin folder - this organizes the file(s) of your plugin.
- Main plugin file (.php) with header - this contains the plugin information, along with some/all of the plugin's code.
Many plugins include these additional elements:
- Subfolders - you can use these to organize plugin files and assets.
- Scripts - you can enqueue JavaScript when needed.
- Stylesheets - you can enqueue CSS when needed.
- readme.txt - this is essential if you plan to submit your plugin to WordPress.org, as WordPress.org uses this to populate your plugin's page in the directory.
Here's an example of the folder structure for a simple plugin (Hello Dolly):
You can see that it's just two files in a folder - the main .php file and the readme.txt file.
And here's an example of a much more complex plugin (Elementor):
You can see that Elementor stores a lot of separate assets in subfolders.
How to Make a WordPress Plugin
Now that you understand the basics of WordPress plugin development, let's get into the actual steps for how to make a WordPress plugin:
- Prepare the basics (name and requirements)
- Create your plugin folder and structure
- Add the plugin file header
- Write/add the code
- Deploy to WordPress
These steps are not equal in the time that you'll devote to them. Step four, where you actually write the code for your plugin, will obviously take up the bulk of your time, especially for more complex plugins.
With that being said, all five steps are important when it comes to creating a plugin to successfully use on your WordPress site.
For this example, we'll be recreating the Hello Dolly plugin that's been with WordPress since the beginning. However, these steps are universal and you can easily adapt them to your own use case.
1. Prepare the pasics (name and requirements).
Before you start working with the actual code and files of your plugin, you'll want to do a little work to prepare the basics.
First, you'll want to clearly work out the requirements for your plugin before you start coding. Here are some common questions that you should be able to answer:
- What are the features of the plugin?
- How will users control those features?
- What input does the plugin need to accept from users?
- What will the front-end design look like (if applicable)?
- Will the plugin integrate with other plugins or services?
- What potential compatibility issues might the plugin have?
The answers to these questions will impact how you approach coding your plugin.
If you want to release the plugin publicly, you'll also need to come up with a name for your plugin. To avoid issues with your plugin's name, here are a few tips:
- Check to see if there's already a plugin that exists with that name, as this might cause confusion.
- Keep trademark issues in mind. For example, you can't use "WordPress" in your plugin's official name, though you can use the "WP" abbreviation. Similarly, be careful using other brands' trademarks. For example, a lot of plugins that used "Instagram Feed" in their name had to change their names.
For our example, our plugin name will be Hello Dolly, just like the original.
2. Create your plugin folder and structure.
By default, WordPress stores all plugins inside the …/wp-content/plugins folder.
All of your plugin's files will be contained inside a folder in that directory. For example - …/wp-content/plugins/hello-dolly/.
The complexity of this folder will depend on the complexity of your plugin.
For simple plugins, you can just use a single PHP file. In such an example, all of the functionality of your plugin would be available at …wp-content/plugins/hello-dolly/plugin-file.php.
For more complex plugins, you might need more complex structures. For example, you might create separate subdirectories for different types of files.
In such cases, you might store CSS and JavaScript files in wp-content/plugins/hello-dolly/assets, templates in wp-content/plugins/hello-dolly/templates, and so on.
For even more complex plugins, you can also consider the model-view-controller design pattern. Here, the WordPress MVC framework can help you speed up development. We'll talk more about WordPress plugin frameworks a little later in this post.
The WordPress Plugin Handbook has some additional suggestions for creating the structure of your plugin.
For our simple example, though, we only need a single PHP file for the plugin.
3. Add the plugin file header.
When you create your main plugin PHP file, you need to add a file header to help WordPress understand your plugin.
The plugin file header is a PHP comment block that includes basic details about your plugin, such as its name, version number, author, license, and more.
WordPress will use this information to show details about your plugin in the Plugins area of the dashboard.
You can find the full plugin file header at the WordPress Codex.
Here's an example of what it should look like:
4. Write/add the code.
Now it's time for the fun part - adding the actual functionality for your plugin!
Again, this is where the majority of the WordPress plugin development happens, so this will naturally take you more time than the previous steps.
If you need some help here, you can consult the WordPress.org plugin handbook for details on various parts of WordPress functionality. Adam Brown's WordPress hooks list is also a valuable resource, as is WPSeek, which lets you search for WordPress functions, filters, actions, and constants.
We'll also share even more plugin development resources at the end of this post.
Our simple Hello Dolly-based example looks like this - it's exactly 100 lines of code:
This code does two main things.
First, the hello_dolly_get_lyric function lets us define the song lyrics that we want to display and randomly choose a line from it.
Second, the add_action( 'admin_notices', 'hello_dolly' ); action hook uses the admin_notices hook to actually insert the song lyrics inside the WordPress admin dashboard.
The plugin is also doing a few other smaller actions, such as inserting its CSS in the <head> section of relevant pages.
5. Deploy to WordPress.
Once you've finished your plugin, you're ready to package it up and deploy it to WordPress.
The simplest option, and the easiest way to let other people use your plugin, is to just package your main plugin folder as a ZIP file.
You, or others, can then install the plugin directly from the WordPress dashboard by going to Plugins → Add New and uploading the ZIP file.
Once the installation finishes, the plugin will show up in the regular plugin list. Again, you can control the information that appears in the plugins list by using the plugin file header from the third step above.
If this is a custom-built plugin for your own site that you'll be updating in the future, you might want to consider alternative methods for code deployment and management.
For example, you could use GitHub to manage your plugin's code and then deploy straight to WordPress using a tool like WP Pusher. For more on this type of approach, you can read our full WordPress GitHub guide.
WordPress Plugin Development Frameworks and Resources
The above guide gives you a basic approach to WordPress plugin development. However, as you begin to get more advanced, you can start using other tools to speed up your development and build more complex plugins.
To that end, here are some of the best WordPress plugin development frameworks and resources to help you work more efficiently.
WordPress.org Plugin Handbok
The official WordPress.org Plugin Handbook is one of the best resources to learn everything needed for WordPress plugin development. You'll find yourself constantly coming back to this, so it might be worth a bookmark!
WPSeek
WPSeek is a really handy resource for quickly finding the correct WordPress function, filter, action, or constant.
It offers a real-time search with live suggestions that makes it easy to find the right code snippet. For example, here's what it looks like to search for functions that include "admin:"
Each search results page also includes an explanation, parameters, example code, and more.
WordPress Plugin Boilerplate Generator
WordPress Plugin Boilerplate Generator is a free web-based interface that helps you use Tom McFarlin's WordPress Plugin Boilerplate foundation plugin.
All you need to do is enter some basic details about your plugin and it will generate a “standardized, organized, object-oriented foundation for building high-quality WordPress Plugins.”
WordPress MVC (WPMVC)
As we mentioned earlier, WordPress MVC is a free framework for helping you build WordPress plugins using the model-view-controller design pattern.
If you want to use this approach to WordPress plugin development, it's a great way to save time.
Get Started With WordPress Plugin Development Today
If you already know your way around PHP, WordPress plugin development shouldn't feel overwhelming.
The simplest way to get started with this is to create a single file plugin. All you need is a folder and a PHP file with the proper header (and some helpful code, of course).
As you begin to upgrade your knowledge, you can start creating more complex plugins that include more assets. Tools like the WordPress Plugin Boilerplate Generator or WordPress MVC can help you get started with the proper structure for more complex plugins.
Create your first WordPress plugin today and you'll be an expert in no time!