You may not know what an API does or even what it stands for. But, you've definitely encountered them. Everyday APIs work behind the scenes to provide you with richer digital experiences.
The movie ticket you bought online, the recipe you shared on Facebook, and the flight you booked on Expedia are all thanks to APIs.
By delivering seamlessly integrated user experiences, APIs present a wealth of business opportunities. But, before discussing how APIs could advance your business, let’s take a closer look at what APIs are and why they’re proliferating.
What is an API?
An API, short for Application Programming Interface, is a software-to-software interface. APIs provide a secure and standardized way for applications to work together. They deliver the information or functionality requested without user intervention.
Because APIs do all the heavy lifting, digital experiences remain virtually effortless to end users.
Here's a video from Mulesoft that does a great job of explaining the purpose of an API with a restaurant analogy:
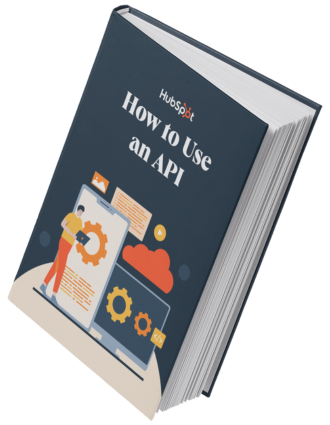
Free Ebook: How to Use an API
Everything you need to know about the history and use of APIs.
- A History of APIs
- Using APIs
- Understanding API Documentation
- And more!
Download Free
All fields are required.
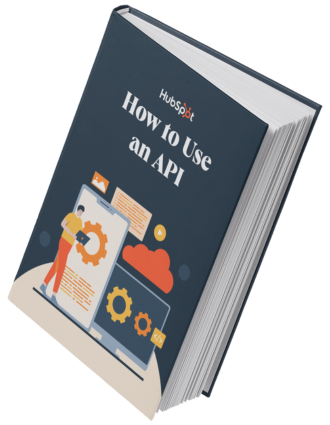
Real-World API Example
Say you want to see the latest box-office hit. Your first step might be searching where and when it’s playing on an online ticketing site like Fandango.
After typing in your zip code and selecting a date, you click “go.” Then, voilà, a list of showtimes at nearby theaters appears within seconds. Here’s what it looks like to the end user.
Although you stay on Fandango’s site the whole time, there are multiple applications at work to make your search possible. When you click “go,” the site uses APIs to request access to each theater’s database.
The act of requesting access to information through an API is called an API call. After the API is called, it retrieves the requested information and sends it back to Fandango. Finally, Fandango’s site displays the most relevant results for you.
By leveraging APIs to access another company’s data, you can extend the functionality of your own product while saving time and money. This can also help you stay agile in the marketplace.
How do APIs work?
APIs are sets of definitions and protocols that allow software components to talk and interact with each other using a simple set of commands. Acting as messengers, APIs deliver one application’s request to another and return a response in real time.
If the server (the application providing the resource) can do what the client (the requesting application) asked, then the API returns the resource needed and a status code that indicates "mission accomplished."
But, sometimes the server can’t do what the client asks. Maybe the client requested a resource that doesn’t exist or that it doesn’t have permission to access. In this scenario, the API return an error status code.
Controlling access to the server in this way is crucial. Rather than give you all of a program’s information or code, an API provides you only with data made available to external users.
API Calls
An API call is the process of a client application submitting a request to a server's API. An API call also comprises everything that happens after the request is submitted. This includes when the API retrieves information from the server and delivers it back to the client.
Request Methods
Most clients want the server to carry out basic functions. These requests may be written as URLs. In this case, the communication between the client and server is dictated by Hyper-Text Transfer Protocol (HTTP) rules. The four most common request methods to a server are:
- GET: To retrieve a resource
- POST: To create a new resource
- PUT: To edit or update an existing resource
- DELETE: To delete a resource
Still confused? Let’s try an analogy. How APIs work is often compared to ordering food at a restaurant. Here, the diner represents the client. The waiter represents the API, and the chef represents the server.
You look over the menu, pick the meal you want, and place your order with the waiter. The waiter brings your request to the chef. The chef executes it. Then, the waiter brings you your meal. You enjoy it all the better for not having had to make it yourself.
But let’s say too many guests show up at the restaurant, and there’s not enough space to accommodate them. Let’s say this keeps happening as the restaurant grows in popularity. What then? That’s where API authorization comes into play.
What is API authorization?
API authorization determines what actions a client can perform once they are authenticated by the API. Authentication comes before authorization. This is where the client's identity is confirmed via an API key, authentication tokens, or other credentials.
Back to our restaurant example. Authentication is like a code to get into a speakeasy. Once the security team hears the secret words, it knows you can be trusted.
After the identity is confirmed by the API, it checks its permissions and determines if the request should be allowed. This is API authorization and it's critical to security.
One common form of authentication used in the authorization process is an API key.
What is an API key?
An API key is a unique identifier used to authenticate calls to an API. The key is made up of a string of letters and numbers that identify the client. (Remember, this is the application or site making the request.)
The key can grant or deny that request based on the client’s access permissions. The key also tracks the number of requests made for usage and billing purposes.
API keys are less secure than authentication tokens. However, this method has advantages over basic authentication, which only requires a username and password.
By restricting access only to those with keys, a company can control the availability of its data. This ensures that only a specific, trusted group of clients can access its server’s resources.
Continuing our example above, think of an API key as a reservation and the API as an exclusive restaurant. By enforcing that patrons must have a reservation to eat at the restaurant, you can keep the number at capacity. The kitchen can adequately and efficiently serve every guest.
Similarly, by allowing only clients with an API key to access and use your resources, you help ensure your software is used safely. You can also guarantee that you can handle the number of incoming requests.
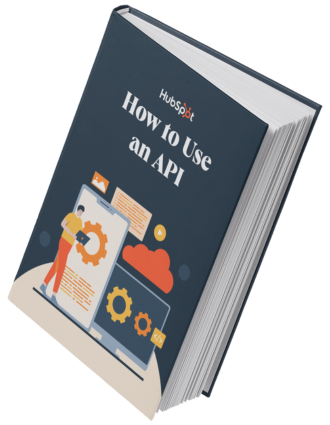
Free Ebook: How to Use an API
Everything you need to know about the history and use of APIs.
- A History of APIs
- Using APIs
- Understanding API Documentation
- And more!
Download Free
All fields are required.
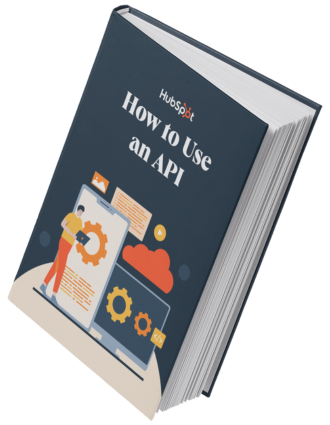
What are APIs used for?
A better question might be what are APIs not used for? Want to embed Instagram photos on your e-commerce app? There’s an API for that. Want to provide instant access to thousands of hotels on your travel blog? There’s an API for that.
Generally speaking, business applications of APIs include:
- Data sharing. Any time a program needs to get data from a third party (e.g., a travel app compiling flight times from airlines), data can be shared through an API.
- App integrations. When two digital applications work in conjunction — HubSpot and Gmail, for example — an API is likely involved.
- Embedded content. To embed a piece of content that is hosted on a different website — like a YouTube video — a request is made to the embedded content's owner to retrieve it.
- Internal systems. APIs aren't only for sharing data externally. Businesses frequently divide their software infrastructure into smaller components. These elements communicate with each other through APIs, like in a microservice architecture.
These examples hit upon some of the most common reasons companies use APIs. Below, we dive into more examples of APIs and their business applications.
API Examples
You’ve probably heard of a few without searching an API marketplace or knowing much about APIs. Below we’ll look more closely at several examples in action.
HubSpot APIs
HubSpot’s platform appeals to businesses with three main needs:
- Those looking to build an app and put it in front of thousands of potential users.
- Those looking to create a custom integration between their app and HubSpot’s application.
- Those looking to build or extend the functionality of their website.
HubSpot's APIs can help you gather data from transactional software and store contacts. There are also ways to engage with third-party tools and custom apps in one CRM.
Twitter APIs
If you work for a brand that loves Twitter, you can use the social media platform’s APIs to drive user engagement on your site.
Twitter has a whole platform offering APIs that allow you to dig through Twitter’s massive archive. You can use Twitter APIs to find old Tweets, embed timelines within your website, or manage ad campaigns. Other APIs can do the following:
- Filter and stream Tweets in real time.
- Deliver personalized customer service through direct messages.
- Subscribe to the real-time activities of more than 15 accounts via webhooks.
There are also a ton of technical resources and tools available with documentation. This can help you harness the full potential of its global communication network.
Instagram APIs
Instagram’s platform offers a range of APIs to help your business with content publishing, metrics, and more.
One potential use case is to embed user-generated Instagram photos on your app. User-generated content (UGC) is a strong form of social proof that can entice leads to convert.
Developers plug into this platform to build apps and services that target three groups:
- Individuals looking to share their own content with third-party apps.
- Brands and advertisers needing to understand and manage their audience and media rights.
- Broadcasters wanting to discover content and get digital rights to media or share it with proper attribution.
YouTube APIs
YouTube APIs enable you to add functionality to your site and access YouTube’s library of videos.
Let’s walk through a few reasons you might want to use one of YouTube's APIs:
- To play videos directly in your app.
- To let users search content and upload videos.
- To create and manage playlists.
- To gain insight into how users are interacting with your videos and channel
- To schedule live broadcasts.
You’ll need to get a YouTube API key to unlock its potential.
Spotify Web APIs
If you’re in the music industry, check out the Spotify Web API. You can now easily add music player functionalities to your website or application.
Using Spotify’s multitude of APIs, you can get complete access to the Spotify Data Catalog. This includes albums, musical artists, and tracks. To retrieve user-related data, such as private playlists, users must authorize your app to access their accounts.
The functionalities are nearly identical to what you would find on the native Spotify platform. With the Browse API, for example, your users can access genres, categories, new releases, and playlist recommendations. Spotify also offers Episodes, Follow, Library, and Playlist APIs.
Stuck? Spotify published a list of example apps so you can see how their APIs have been used in the past.
Google Maps APIs
The Google Maps APIs are essential for anyone building a location-based app. For example, if you’re building a real estate app, you can use the Google Maps APIs to include a street view of the property.
The Google platform has an expansive library of APIs with unlimited uses. Want to allow users to request directions? How about including Places on your website? There’s even an API if you’re building a ridesharing app. Google has divided the APIs by usage and platform (web, iOS, or Android), so choose the correct one for your project.
Like most examples, these APIs are paid based on monthly usage. The high level of functionality they offer makes them well worth the price.
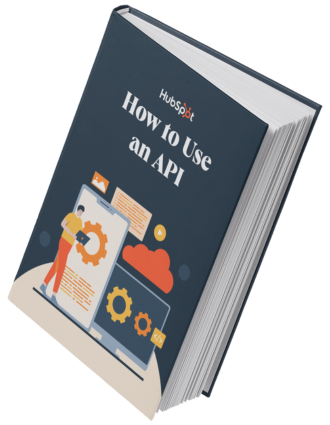
Free Ebook: How to Use an API
Everything you need to know about the history and use of APIs.
- A History of APIs
- Using APIs
- Understanding API Documentation
- And more!
Download Free
All fields are required.
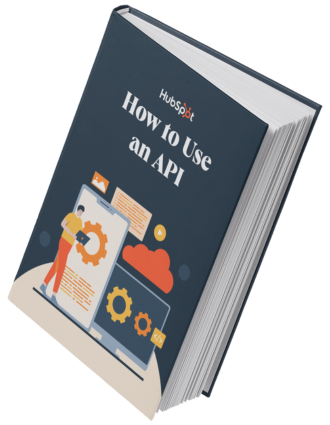
PayPal APIs
The PayPal platform has an expansive library of APIs, so you can integrate PayPal functionality into your app. Now, users don‘t need to access PayPal’s website directly. APIs include:
- The Billing Plans API.
- The Catalog Products API.
- The Disputes API.
- Familiar Invoicing, Orders, and Payments APIs.
If you’re building an e-commerce website or running a membership-based organization, the PayPal APIs will help you manage every step of your transactions.
Should you ever feel stuck, PayPal has a strong support base. Here, you can access frequently asked questions and get help from developers working on PayPal integration projects.
There is a vast wealth of APIs available today. To fully appreciate their potential in the digital economy, let’s take a closer look at how APIs are classified by who they are shared with and why.
Types of APIs
APIs can be categorized by their intended audience and scope. There are four main types of APIs that developers work with:
- Private APIs. These APIs are only made available to a company's internal team to boost productivity and transparency. Developers working for the company can use these APIs as needed. Third-party developers can’t.
- Partner APIs. These APIs are shared externally but only with those with a business relationship with the API company. Some businesses use partner APIs because they want control over who can access their resources and how they get used.
- Open APIs. Open APIs, or public APIs, are available for external use. While some open APIs are free, others require a subscription fee, which is often tiered based on usage.
Composite APIs
Composite APIs allow you to bundle calls or requests to get one unified response from different servers. You would use a composite API if you need data from different applications. Or, instead of making five separate API calls in succession, you can make one using a composite API.
Composite APIs get their own category because they can be combine with the APIs listed above. For example, you can have a composite private API or a composite open API.
For a more detailed explanation, see our guide to the types of APIs.
Why use an API?
Now we know that businesses can use APIs to securely request and share content both internally and externally. You might be thinking, “Why would a company pay another for access to resources it could create? Why would that company agree to share its assets, particularly with competitors?”
All good questions. Before we dive into the answers, let’s split up the benefits of APIs into two groups: API consumers and API providers.
Benefits to API consumers
You may use APIs to request access to another server’s resources, or you may use your own APIs to automate certain tasks. Either way, you fall under the mantle of API consumers. These consumers benefit from using each type of API in several ways.
Increased Productivity
Many companies consume their own APIs. Why? Using APIs internally enables businesses to streamline operations, foster collaboration, and strengthen transparency across the company.
Amazon founder Jeff Bezos understood the vast potential of internal APIs. In 2002, he sent an email instructing every team to communicate through APIs moving forward.
Following what has been coined “The Bezos Mandate,” developers built or turned their well-developed software components into APIs. This established well-managed ways of exchanging data across the company as a result.
Higher User Satisfaction
Companies want to provide the best experience for their users. Rarely can one product satisfy and anticipate every need and expectation. That is why they use APIs to extend the functionality of their products.
For example, OpenTable uses the Maps JavaScript API to embed an interactive map on its site. This lets users get directions to nearby restaurants in just a few clicks. If you’re unfamiliar, check out this map of a steakhouse in Indiana.
Allows More Innovation
Allowing developers — external or internal — to reuse software saves time. This allows them to focus on developing new solutions rather than repeating work that’s already been done.
Let’s revisit the example above. Without the Google Maps API, developers at OpenTable would have to dedicate their time and resources to drawing their own maps.
Worse still, no matter how much time they put in, it would be nearly impossible to make it as detailed or reliable as Google’s existing solution. Instead of wasting time reinventing the wheel, APIs enable developers to focus on creating new tools that deliver more value.
Benefits to API Providers
Now, let’s focus on why you might want to create and then share one with partners or the general public. Simply put, becoming an API provider unlocks business opportunities. Let’s look into each one below.
Revenue
The easy answer is money. Google, Yelp, Facebook, and thousands of other companies make their APIs public. They monetize APIs so that they become additional lines of revenue.
In fact, at some companies, APIs are the major source of revenue. According to a recent report by MuleSoft Inc., 35% of today’s technology leaders generated more than a quarter of their organizations’ revenue as a direct result of APIs.
Scale
Sharing what you do well with the broadest possible audience helps you scale. A network of users — including third-party developers and consumers — will become reliant on your API's functionality.
Ultimately, this will improve the usage and adoption of your main platform. In other words, APIs expand your customer base and generate new market opportunities in the digital economy.
Amazon Web Services (AWS) is among the most notable examples. This platform allows any company or developer to run its applications on top of Amazon’s infrastructure via APIs.
AWS is used by millions of customers around the world. It's credited with transforming Amazon from an online bookstore to a global digital giant.
Even More Innovation
Yes, I’m listing this again, but with a twist. Above, we discussed how API consumers can use these tools to save time. More often than not, however, the relationship between API consumers and providers is much more give-and-take.
You may recall that Twitter's user interface was a little clunky in the early days. TweetDeck, an independent app at the time, built a better user interface on top of the Twitter platform using its public API.
Twitter later acquired this dashboard so that all users could have better experiences. That’s called a win-win-win, my friends.
How to Use an API
So you’re ready to try your luck with APIs. Now what? Before implementing your own API at your company, it makes the most sense to use others' APIs. Let’s walk through the basics of getting started using an API.
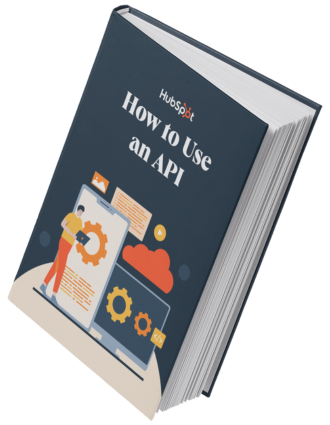
Free Ebook: How to Use an API
Everything you need to know about the history and use of APIs.
- A History of APIs
- Using APIs
- Understanding API Documentation
- And more!
Download Free
All fields are required.
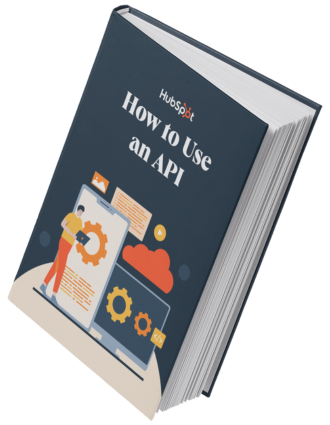
1. Select an API.
First, you’ll want to find an API to incorporate into your business. You might already have your eye on an API, particularly if you’re interested in one of the big wigs like the Facebook API. You might also want to search by cost — you may want to start with a free API before exploring paid APIs, for example.
Once you have an API selected, get your reading glasses on. It’s time to look through the API documentation.
2. Choose your authorization method.
As mentioned earlier, you have a few options when it comes to API authorization. You can use an API key, authentication tokens, or user credentials. In this example, we'll use an API key.
An API key identifies you as a valid client, sets access permissions, and records your interactions with the API.
Some APIs make their keys freely available, while others require clients to pay for one. Either way, you’ll most likely need to sign up for the service. You’ll then have a unique identifier assigned to you, which you will include in your calls.
Always keep your key private, like you would a password. If your key leaks, a bad actor could make API requests on your behalf. You may be able to void your old key and get a new one if such a breach occurs.
3. Review the API documentation.
API Documentation is essentially an instruction manual about how to use an API. In addition to providing documentation, it usually includes examples and tutorials.
Refer to the documentation for how to get your key, how to send requests, and which resources you can fetch from its server.
It’s hard to understate the importance of good API documentation. A company might offer a powerful API, but if developers can’t quickly learn how to use it, it’s not very valuable
4. Write a request to an endpoint.
Next up, you’ll write your first request. The easiest method is to use an HTTP client to help structure and send your requests. You’ll still need to understand the API’s documentation, but you won’t need much coding knowledge to be successful.
You'll need the proper parameters, headers, and body required to make your request. You should also consider your endpoint URLs and request payload, if applicable.
You can then use a tool like cURL or Postman to send the constructed HTTP request to its endpoint. You can also use a programming language to accomplish this as well.
Here's an example using cURL:
curl -X GET "https://api.example.com/resource" -H "Authorization: Bearer YOUR_ACCESS_TOKEN"
5. Connect your app.
Now that you understand how to make requests to your API of choice, you can sync your application with it. As a marketer, you don't need to worry about this stage of API integration. This is the job of a developer who will employ languages like Python, Java, JavaScript (and NodeJS), PHP, and more.
Chances are, the API you're interacting with is a specific type of API that is considered easier to use than others. These APIs are called REST APIs.
REST APIs
REST is shorthand for a software architectural style called “Representational State Transfer.” It determines how the API should work and makes it easier to use and discover on complex networks like the Internet.
With REST APIs, everything is treated as a resource. This includes data, services, and objects. Each resource is identified by a uniform resource identifier, or URI. In this case, the URI will be a URL, since REST APIs most commonly use HTTP or HTTPS in their schemes.
To request a resource via a REST API, like the Open Weather Map API, you just need to provide its URL.
In a typical REST API, a resource will have two URL patterns assigned to it:
- Plural or collection: This represents a collection or group of resources.
- Singular: This respresents a single resource.
For example, if we wanted to retrieve a list of all users from a server, we might use a plural pattern called "/users." But, if we wanted to get information on one specific user, we would add an additional identifier to the URL like this: /users/{id}. Now we are narrowing our focus down to one specific user.
These are also referred to as endpoints. Each endpoint is also assigned a list of actions the client can request from the server. So, a plural endpoint may be for listing or creating resources. The singular endpoint may be for retrieving, updating, and canceling a specific resource.
The client would have to include the correct HTTP verb (GET, POST, PUT, DELETE) in its request to tell the server what action to take. Here are a few examples:
- GET /records: Retrieves a list of a records.
- POST /records: Creates a new record.
- PUT /records/123: Updates the record with the ID of 123.
- DELETE /records/123: Deletes the record with the ID of 123.
Here are a few data formats that the client and server can use to pass this information back and forth:
- XML (Extensible Markup Language)
- JSON (JavaScript Object Notation)
- HTML (Hypertext Markup Language) -- though this is not as commonly used.
REST API Example
WordPress has a REST API that enables developers to create, read, and update WordPress content remotely. The API does so by carrying JSON objects back and forth between clients and servers.
In other words, by allowing developers to structure how they get data from a server easily, they can spend less time accessing said data. They can now focus on better user experiences.
REST vs. SOAP
REST is usually put head-to-head with Simple Object Access Protocol (SOAP), another way to build applications that work over HTTP.
REST is considered a simpler alternative to SOAP, as it requires writing less code to complete tasks. REST APIs also follow a less rigid structure and logic than SOAP. Another reason people love REST: it provides plenty of conventions but leaves many decisions up to the person designing the API.
Due to its simplicity and flexibility, most developers (including ours at HubSpot) will opt for a REST architecture in their APIs.
The Future of the Digital Economy
There are many business advantages to both consuming and providing an API, but let’s keep it simple. The main reason? To connect your application to the rest of the software world. These connections can empower all types of organizations to create new business models that spur more innovation.
In this way, APIs are not only changing how companies do business. They're changing the way companies think about business.
This post was originally published in October 2019 and has been updated for comprehensiveness.