In web design, a carousel is a slideshow for presenting a series of content. Sounds harmless, right? But some web designers love carousel sliders, and others despise them.
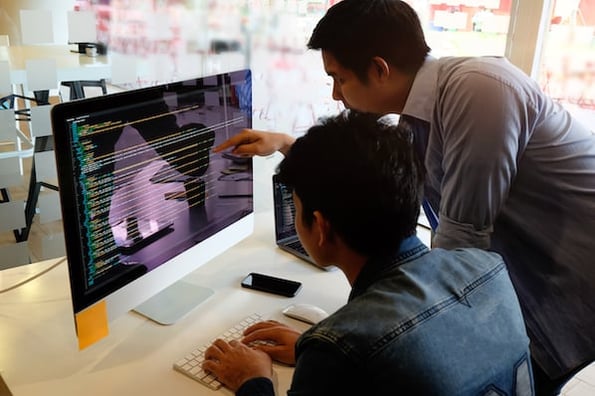
That’s because when done right, a carousel slider can effectively organize serial content or multiple, separate pieces of content, like images. It can also boost engagement and provide a better user experience by enabling visitors to quickly and easily navigate this content, especially on mobile.
Take a look at this awesome carousel slider displaying popular categories on the fashion website ALIÉTTE, for example.
However, when done wrong, carousel sliders cause more harm than good. An automated slider, for example, can be more distracting than informative. A slider with cheesy animations can make your site seem less professional. And a poorly designed carousel can be completely overlooked, which effectively hides the information on other slides from your visitors.
To avoid these potential pitfalls and only reap the benefits of a carousel slider, make sure you understand the do’s and don’ts of this design element. A big “do” is to create a carousel slider with accessibility in mind. Below we’ll cover two ways you can do so on your site: using Bootstrap CSS or using pure CSS. Then we'll walk through how to create an image carousel.
CSS Carousel
There are two ways to create a carousel slider for your website. You can build it from scratch using HTML and CSS only. Or you can use Bootstrap CSS, which is a framework that offers pre-designed components including carousels, to speed up the code development process.
Below we’re going to take an in-depth look at each process and walk through an example carousel build for each.
How to Make a Simple Carousel in Bootstrap CSS
With Bootstrap, you can make a simple carousel using only HTML. To show you how, we’re going to break down the process of making a carousel with slides and controls.
1. Create a parent div element with an id and class attribute.
Let’s start with the parent element. You need a div element to start. Make sure to give it a unique ID name so you can apply optional controls later. This is particularly important if you’re adding multiple carousels on a single page. I’m going to name this example "SimpleCarouselExample."
Then you want to define the element with the .carousel slide class and add the data-ride="carousel" attribute so that the carousel starts animating at page load, without you needing to do anything. Here’s what our HTML looks like so far:
2. Add image elements inside the parent div element.
Now we need to place child elements inside this parent <div> element. Let’s say we want three images in the carousel. Here’s the HTML for those images, with ellipses acting as placeholders for the specific image source and alt text we’d use.
Notice the presence of the .d-block and .w-100. These classes prevent browser default image alignment. More specifically, .d-block is a display property value in Bootstrap that makes an element visible on all screen sizes and .w-100 is a width utility value that makes the element 100% as wide as its container.
3. Wrap each image in a div element.
We need to wrap each of these images in a <div> element with the .carousel-item class so the browser knows they belong in the carousel. Here’s what that looks like for the first image:
Note: The .active class needs to be added to one of the slides. Without this class, the carousel will not be visible.
4. Wrap all these inner divs in another div element.
I’m going to wrap all of these carousel items in a <div> element with the .carousel-inner class, as shown below:
5. Add controls with ARIA attributes.
Now that I have successfully created a carousel with slides only, it’s time to add in the previous and next controls. To create these, add two <span> elements and define them with .carousel-control-prev-icon class and the .carousel-control-next-icon class, respectively.
Notice the ARIA attributes. We’ll explain them in a minute but, first, we have to go over what to add to make the design accessible.
6. Add span elements to make the controls accessible to screen readers.
Some users, particularly those using screen readers, will not see or be able to click the previous and next icons. That’s why you need to add two more span elements that spell out each icon as “Previous” and “Next.” Define these with the .sr-only class so that the text is hidden on all devices except screen readers. You should now have two pairs of spans that look like this:
The aria-hidden attribute tells assistive technologies to skip the carousel’s DOM so that when they go over this code, they read simply read "Previous" or “Next.”
7. Wrap the controls in anchor elements.
Now that we’ve created the icons (and alternative text), we need to wrap the pairs in <a> elements. Define each element with the .carousel-control prev and the .carousel-control-next class, respectively.
You’ll also need to name the a[href]s with the exact same ID as the carousel element. In Bootstrap, control and indicator elements must have a data-target attribute (or href for links) that matches the id of the .carousel element. You’ll assign them each the role of button and the data-slide attribute set to “prev” and “next,” respectively. Here’s what the HTML will look like:
8. Put all the code together.
Put all together, here’s the HTML you’ll need to create a simple carousel with slides and controls:
This is how the carousel will look and behave on the front-end of your site. Notice that it automatically goes to the next slide if I do nothing, but will let me flip back and forth when using the control options.
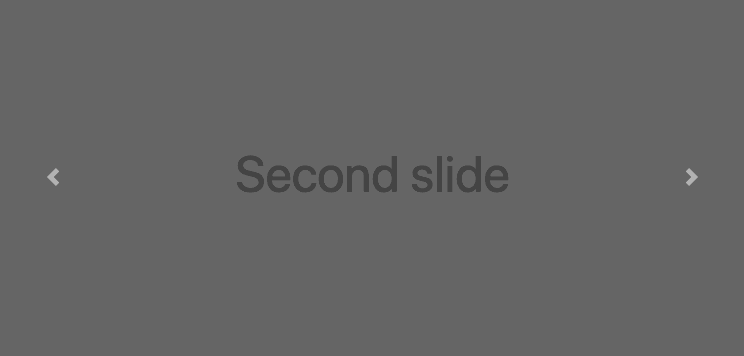
You can find other examples of carousels in Bootstrap’s official documentation.
A Pure CSS Carousel Slider
Before we dive into an example build, it’s important to understand that creating a pure CSS carousel slider requires HTML as well. After all, CSS is a styling language so you need HTML to have something to style.
What a “pure CSS” or “CSS-only” carousel means is that you won’t need JavaScript. This is good news for most site owners since JavaScript is a complex programming language that can be difficult to learn and use correctly.
Now that we have a better idea of the terminology and value of thinking CSS-first, let’s walk through an example of building a CSS-only carousel.
1. Create the slides in HTML.
Let’s say you want to create a carousel with three slides. Then you’d create three <div> elements and wrap them in a parent <div> element with the class .slides, as shown below:
2. Define the display property in CSS.
To style the container, use the CSS selector.slides. To start, set the display property to “flex” to make it a flexbox. Make sure you do the same to the slides so they become flex items as well. Here’s the CSS:
You can learn more about how to use Flexbox to create one-dimensional layouts in Here's the Difference Between Flexbox, CSS Grid, & Bootstrap.
3. Define the overflow-x property to add a scroll bar.
Now we want to make sure users can scroll through these slides manually. To do so, we’re going to set the overflow-x property of the container to auto. This provides a scrolling mechanism for overflowing boxes or, in this case, a slideshow. Here’s the CSS:
4. Optimize the carousel's size for all devices.
Then to each slide in the carousel, we’re going to set the width to 100% so it spans the whole viewport horizontally and set the height to 300px. We’re also going to set the flex-shrink property to 0 so that the slides don’t shrink or wrap on smaller devices. Here’s the CSS:
5. Optimize the carousel's scrolling behavior for all devices.
We can do a few more things to improve the scrolling experience. To have each slide “snap into” place when scrolling from one to the other, we can add snap-points with the scroll-snap-type property and the scroll snap align property. We can also make sure that the sliding action is smooth on both desktop and mobile with the scroll-behavior property. Here’s what the CSS looks like:
6. Style the carousel.
I’m just going to style my three slides so that we can see them better, changing the background color to blue, aligning both the slides and the text in the center of the container, and increasing the font-size. Here’s the CSS:
7. Put all the code together.
All together, here’s the CSS:
Here’s the result:
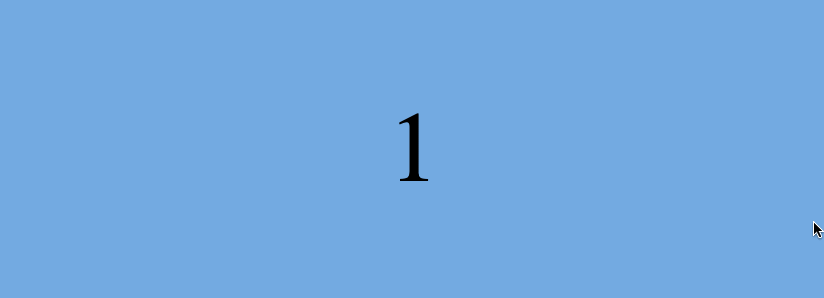
Image Carousel CSS
You can create an image carousel using Bootstrap or "pure" CSS. For the sake of this demo, we'll use Bootstrap since it's easier for creating responsive sliders.
Below is a nearly identical process to the one outlined in the Bootstrap CSS section above — except we'll add indicators instead of controls.
1. Create a parent div element with an id and class attribute.
To start, create a div element and give it a unique ID name so you can apply optional indicators later. I’m going to name this example "ImageCarouselCSS."
Then you want to define the element with the .carousel slide class and add the data-ride attribute set to "carousel." This will ensure the carousel starts animating at page load, without you needing to do anything. Here’s what our HTML looks like so far:
2. Add an ordered list.
Next create an ordered list and define it with the carousel-indicators class name.
Inside the list, place three items. Each will have a data-target attribute defined with the carousel's ID name and a data-slide-to attribute. This attribute will be set to 1 for the first list item element, 2 for the second list item element, and so on.
The .active class needs to be added to one of the list items. Here's the code:
3. Add images inside the parent div element.
Place however many image elements you want to feature in the carousel after the ordered list. Let’s say we want three images of puppies in the carousel. Here’s the HTML for those images:
Remember the .d-block and .w-100 classes prevent browser default image alignment.
4. Wrap each image in a div element.
Wrap each of these images in a <div> element with the .carousel-item class so the browser knows they belong in the carousel.
Remember to add the .active class to one of the images so the carousel will be visible.
Here’s what the code looks like:
5. Wrap all these inner divs in another div element.
Now wrap all of these carousel items in a <div> element with the .carousel-inner class, as shown below:
6. Pull all the code together.
Here’s the HTML all together:
Here's the result:
Adding a Carousel Slider to Your Site
Carousel sliders can help you effectively use space and organize content on your site, while keeping visitors engaged and giving them control over how they read your content. If they’re the right choice for your site, then you can use either of the methods above to create a carousel slider without Javascript. You just need to understand some HTML and CSS.
Editor's note: This post was originally published in June 2020 and has been updated for comprehensiveness.