The C++ Standard Library provides a wide range of mathematical functions that are useful for all sorts of programming tasks. You can add, subtract, find absolute values, and more by calling arithmetic functions into your project.
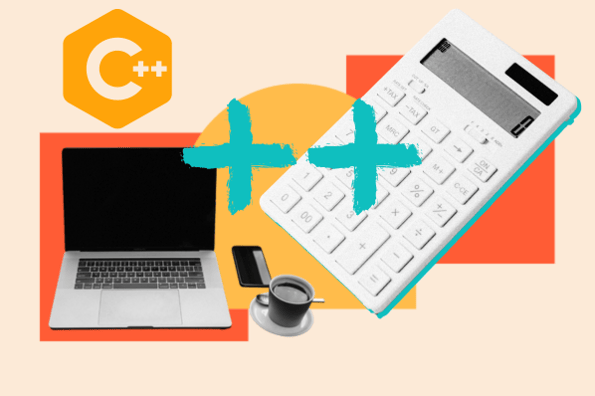
For this post, we've curated a list of functions that you should have at your disposal when calculating mathematical equations in C++. We'll review what each one does and look at an example so you can see how it works in a live use case.
Keep this post handy as a reference guide so you can return to it whenever you need a quick reminder on C++ math functions.
C++ Math Functions
1. Absolute Value: abs(x)
This function returns the absolute value of a number.
Example:
#include <iostream>
#include <cmath>
int main() {
int x = -5;
int absoluteValue = abs(x);
std::cout << "Absolute value of " << x << " is: " << absoluteValue << std::endl;
return 0;
}
Output:
Absolute value of -5 is: 5
2. Square Root: sqrt(x)
This function returns the square root of a number.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 25.0;
double squareRoot = sqrt(x);
std::cout << "Square root of " << x << " is: " << squareRoot << std::endl;
return 0;
}
Output:
Square root of 25 is: 5
3. Cube Root: cbrt(x)
This function returns the cube root of a number.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 27.0;
double cubeRoot = cbrt(x);
std::cout << "Cube root of " << x << " is: " << cubeRoot << std::endl;
return 0;
}
Output:
Cube root of 27 is: 3
4. Power: pow(x, y)
This function multiples x to the power of y.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 2.0;
double y = 3.0;
double result = pow(x, y);
std::cout << x << " raised to the power of " << y << " is: " << result << std::endl;
return 0;
}
Output:
2 raised to the power of 3 is: 8
5. Exponential: exp(x)
This function multiples the value of e (2.71828) to the power of x.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 1.0;
double result = exp(x);
std::cout << "Exponential of " << x << " is: " << result << std::endl;
return 0;
}
Output:
Exponential of 1 is: 2.71828
6. Natural Logarithm: log(x)
This function returns the natural logarithm of a number.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 10.0;
double naturalLog = log(x);
std::cout << "Natural logarithm of " << x << " is: " << naturalLog << std::endl;
return 0;
}
Output:
Natural logarithm of 10 is: 2.30259
7. Base-10 Logarithm: log10(x)
This function calculates the base-10 logarithm of a number.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 100.0;
double log10Value = log10(x);
std::cout << "Base-10 logarithm of " << x << " is: " << log10Value << std::endl;
return 0;
}
Output:
Base-10 logarithm of 100 is: 2
8. Base-2 Logarithm: log2(x)
This function calculates the base-2 logarithm of a number.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 16.0;
double log2Value = log2(x);
std::cout << "Base-2 logarithm of " << x << " is: " << log2Value << std::endl;
return 0;
}
Output:
Base-2 logarithm of 16 is: 4=
9. Floor: floor(x)
This function returns the largest integer that’s less than or equal to x.
Example:
#include <iostream>
#include <cmath>
int main() {
double x = 4.9;
double floorValue = floor(x);
std::cout << "Floor value of " << x << " is: " << floorValue << std::endl;
return 0;
}
Output:
Floor value of 4.9 is: 4
10. Ceiling: ceil(x)
This function returns the smallest integer that’s greater than or equal to x.
Example:
#include <iostream>
#include <cmath>
int main() {
double x = 4.1;
double ceilValue = ceil(x);
std::cout << "Ceil value of " << x << " is: " << ceilValue << std::endl;
return 0;
}
Output:
Ceil value of 4.1 is: 5
11. Round: round(x)
This function rounds x to the nearest integer.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 4.5;
double roundedValue = round(x);
std::cout << "Rounded value of " << x << " is: " << roundedValue << std::endl;
return 0;
}
Output:
Rounded value of 4.5 is: 5
12. Sine: sin(x)
This function returns the sine of x in radians.
Example:
#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double sineValue = sin(x);
std::cout << "Sine of " << x << " is: " << sineValue << std::endl;
return 0;
}
Output:
Sine of 0.5 is: 0.479426
13. Cosine: cos(x)
This function returns the cosine of x in radians.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double cosineValue = cos(x);
std::cout << "Cosine of " << x << " is: " << sineValue << std::endl;
return 0;
}
Output:
Cosine of 0.5 is: 0.877583
14. Tangent: tan(x)
This function returns the tangent of x in radians.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double tangentValue = tan(x);
std::cout << "Tangent of " << x << " is: " << sineValue << std::endl;
return 0;
}
Output:
Tangent of 0.5 is: 0.546302
15. Arcsine: asin(x)
This function returns the arcsine of x in radians.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double arcsineValue = asin(x);
std::cout << "Arcsine of " << x << " is: " << sineValue << std::endl;
return 0;
}
Output:
Arcsine of 0.5 is: 0.523599 radians
16. Arccosine: acos(x)
This function returns the arccosine of x in radians.
Example:
#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double arccosineValue = acos(x);
std::cout << "Arccosine of " << x << " is: " << sineValue << std::endl;
return 0;
}
Output:
Arccosine of 0.5 is: 1.0472 radians
17. Arctangent: atan(x)
This function returns the arctangent of x in radians.
Example:
#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double arctangentValue = atan(x);
std::cout << "Arctangent of " << x << " is: " << sineValue << std::endl;
return 0;
}
Output:
Arctangent of 0.5 is: 0.463648 radians
18. Hyperbolic Sine: sinh(x)
This function returns the hyperbolic sine of x.
Example:
#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double hyperbolicSine = sinh(x);
std::cout << "Hyperbolic sine of " << x << " is: " << hyperbolicSine << std::endl;
return 0;
}
Output:
Hyperbolic sine of 0.5 is: 0.521095
19. Hyperbolic Cosine: cosh(x)
This function returns the hyperbolic cosine of x.
Example:#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double hyperbolicCosine = cosh(x);
std::cout << "Hyperbolic cosine of " << x << " is: " << hyperbolicSine << std::endl;
return 0;
}
Output:
Hyperbolic cosine of 0.5 is: 1.12763
20. Hyperbolic Tangent: tanh(x)
This function returns the hyperbolic tangent of x.
Example:
#include <iostream>
#include <cmath>
int main() {
double x = 0.5;
double hyperbolicTangent = tanh(x);
std::cout << "Hyperbolic tangent of " << x << " is: " << hyperbolicSine << std::endl;
return 0;
}
Output:
Hyperbolic tangent of 0.5 is: 0.462117
Using Math Functions in C++
Mathematical functions will save you a lot of time regardless of the programming language that you're working in. Getting familiar with these C++ functions will help you write effective code that efficiently pulls in specific pieces of data and information. We hope you keep this post bookmarked as a handy reference point that you can return to whenever you need to brush up on your C++ coding skills.
Author's Note: This post was written/edited by a human with the assistance of generative AI.