From arithmetic operators that handle basic calculations to logical, bitwise, and comparison operators that facilitate decision-making, each operator in C++ serves a specific purpose for your programming needs.
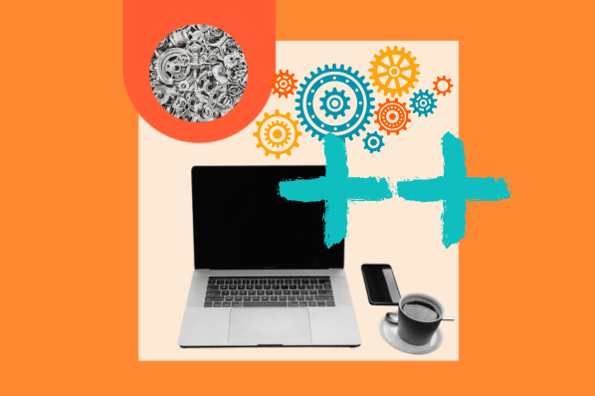
In this post, we'll not only explain what C++ operators do, but we'll also provide a comprehensive list of all types of operators including arithmetic, logical, assignment, and more. Each example will come equipped with a brief definition and a handy code snippet to demonstrate the syntax.
Keep this post handy as a reference to guide you through the various operators available in C++.
Table of Contents:
- What are C++ Operators?
- Arithmetic Operators
- Logical Operators
- Assignment Operators
- Relational Operators
- Bitwise Operators
What are C++ operators?
Operators are special symbols that perform specific operations on one or more operands, such as variables or expressions. They're used to manipulate data and they perform various types of operations -- many of which we'll cover in the sections below.
Operators in C++ are grouped into several categories including arithmetic, relational, logical, bitwise, and assignment operators.
Let's review each along with some examples.
Arithmetic Operators
Arithmetic operators perform basic mathematical operations such as addition, subtraction, multiplication, division, modulus, and increment/decrement. They typically compute these calculations between variables or values in C++ programs.
1. Addition (+)
The addition operator adds two operands or values together.
Example:#include <iostream>
int main() {
int x=5, y=7;
int result = x+y;
std::cout <<"The result of "<< x <<" + "<< y <<" is "<< result << std::endl;
return 0;
}
Output:
The result of 5 + 7 is 12
2. Subtraction (-)
The subtraction operator subtracts the right operand from the left operand.
Example:
#include <iostream>
int main() {
int x=10, y=5;
int result = x-y;
std::cout << "The result of " << x << " - "<< y <<" is "<< result << std::endl;
return 0;
}
Output:
The result of 10 - 5 is 5
3. Multiplication (*)
The multiplication operator multiplies two operands together.
Example:#include <iostream>
int main() {
int x=4, y=3;
int result = x*y;
std::cout << "The result of " << x << " * " << y <<" is "<< result << std::endl;
return 0;
}
Output:
The result of 4 * 3 is 12
4. Division (/)
The division operator divides the left operand by the right operand.
Example:
#include <iostream>
int main() {
int x=10, y=2;
int result = x/y;
std::cout <<"The result of "<< x <<" / "<< y <<" is "<< result << std::endl;
return 0;
}
Output:
The result of 10 / 2 is 5
5. Modulus (%)
Example:#include <iostream>
int main() {
int x=10 y=3;
int result = x%y;
std::cout <<"The result of "<< x <<" % "<< y <<" is "<< result << std::endl;
return 0;
}
Output:
The result of 10 % 3 is 1
6. Increment (++)
The increment operator adds 1 to the value of the variable.
Example:
#include <iostream>
int main() {
int x=5;
x++;
std::cout << "The value of x is now "<< x << std::endl;
return 0;
}
Output:
The value of x is now 6
7. Decrement (--)
The decrement operator subtracts 1 from the value of the variable.
Example:#include <iostream>
int main() {
int x=5;
x--;
std::cout <<"The value of x is now "<< x << std::endl;
return 0;
}
Output:
The value of x is now 4
Logical Operators
Logical operators perform logical operations on Boolean expressions (expressions that evaluate to either true or false). There are three logical operators in C++:
8. AND (&&)
The AND operator returns true if both operands are true, and false otherwise.
Example:#include <iostream>
int main() {
bool x=true, y=false;
bool result = x&&y;
std::cout<<"The result of "<<x<<" && "<<y<<" is "<<std::boolalpha<<result <<std::endl;
return 0;
}
Output:
The result of 1 && 0 is false
9. OR (||)
The OR operator returns true if at least one of the operands is true, and false otherwise.
Example:#include <iostream>
int main() {
bool x=true, y=false;
bool result=x||y;
std::cout << "The result of "<< x <<" || "<< y <<" is "<< std::boolalpha << result << std::endl;
return 0;
}
Output:
The result of 1 || 0 is true
10. NOT (!)
The NOT operator returns the opposite of the boolean value of the operand.
Example:#include <iostream>
int main() {
bool x=true;
bool result=!x;
std::cout << "The result of !"<< x <<" is "<< std::boolalpha << result << std::endl;
return 0;
}
Output:
The result of !1 is false
Assignment Operators
Assignment operators assign a value to a variable. These operators can be combined with arithmetic operators to perform an operation and assign the result to a variable in a single step.
11. Assignment (=)
The assignment operator assigns the value of the right operand to the left operand.
Example:#include <iostream>
int main() {
int x=5, y;
y = x;
std::cout <<"The value of y is "<< y << std::endl;
return 0;
}
Output:
The value of y is 5
12. Addition assignment (+=)
The addition assignment operator adds the value of the right operand to the value of the left operand, then assigns the result back to the left operand.
Example:#include <iostream>
int main() {
int x=5
x+ =3;
std::cout << "The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 8
13. Subtraction assignment (-=)
The subtraction assignment operator subtracts the value of the right operand from the value of the left operand, then assigns the result back to the left operand.
Example:#include <iostream>
Output:
int main() {
int x=10
x-=3;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
The value of x is 7
14. Multiplication assignment (*=)
The multiplication assignment operator multiplies the value of the left operand by the value of the right operand, then assigns the result back to the left operand.
Example:#include <iostream>
int main() {
int x=4
x*=3;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 12
15. Division assignment (/=)
The division assignment operator divides the value of the left operand by the value of the right operand, then assigns the result back to the left operand.
Example:#include <iostream>
int main() {
int x=10
x/=2;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 5
16. Modulus assignment (%=)
The modulus assignment operator divides the value of the left operand by the value of the right operand, calculates the remainder, and assigns the result back to the left operand.
Example:
#include <iostream>
int main() {
int x=10
x%=3;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 1
17. Left shift assignment (<<=)
The left shift operator shifts the bits of the left operand leftwards by the number of positions specified in the right operand and assigns the result back to the left operand.
Example:#include <iostream>
int main() {
int x=5
x<<=3;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 40
18. Right shift assignment (>>=)
The right shift operator shifts the bits of the left operand rightwards by the number of positions specified in the right operand and assigns the result back to the left operand.
Example:#include <iostream>
int main() {
int x=5
x>>=3;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 5
19. Bitwise AND assignment (&=)
This operator performs a bitwise AND operation on the left and right operands and assigns the result back to the left operand.
Example:#include <iostream>
int main() {
int x=5
x&=3;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 1
20. Bitwise OR assignment (|=)
This operator performs a bitwise OR operation on the left and right operands and assigns the result back to the left operand.
Example:#include <iostream>
int main() {
int x=5
x|=3;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 7
21. Bitwise XOR assignment (^=)
This operator performs a bitwise XOR operation on the left and right operands and assigns the result back to the left operand.
Example:#include <iostream>
int main() {
int x=5
x^=3;
std::cout <<"The value of x is "<< x << std::endl;
return 0;
}
Output:
The value of x is 6
Relational Operators
Relational operators compare two values and return a Boolean value (either true or false) indicating whether the comparison is true or false. C++ has six relational operators:
22. Equality (==)
The equality operator compares the equality of two operands and returns true if they are equal, and false otherwise.
Example:#include <iostream>
int main() {
int x=5, y=5;
bool result=x==y;
std::cout <<"The result of "<< x <<" == "<< y <<" is "<< std::boolalpha << result << std::endl;
return 0;
}
Output:
The result of 5 == 5 is true
23. Inequality (!=)
The inequality operator compares two operands and returns true if they are not equal, and false otherwise.
Example:#include <iostream>
int main() {
int x=4, y=6;
bool result=x!=y;
std::cout <<"The result of "<< x <<" != "<< y <<" is "<< std::boolalpha << result << std::endl;
return 0;
}
Output:
The result of 4 != 6 is true
24. Less than (<)
The less than operator compares two operands and returns true if the left operand is less than the right operand, and false otherwise.
Example:#include <iostream>
int main() {
int x=4, y=6;
bool result=x<y;
std::cout << "The result of "<< x <<" < "<< y <<" is "<< std::boolalpha << result << std::endl;
return 0;
}
Output:
The result of 4 < 6 is true
25. Greater than (>)
The greater than operator compares two operands and returns true if the left operand is greater than the right operand, and false otherwise.
Example:#include <iostream>
int main() {
int x=6, y=4;
bool result=x>y;
std::cout <<"The result of "<< x <<" > "<< y <<" is "<< std::boolalpha << result << std::endl;
return 0;
}
Output:
The result of 6 > 4 is true
26. Less than or equal to (<=)
This operator compares two operands and returns true if the left operand is less than or equal to the right operand, and false otherwise.
Example:#include <iostream>
using namespacestd;
int main() {
int x=5;
int y=10;
if(x<=y){
cout <<"x is less than or equal to y"<< endl; std::boolalpha << result << std::endl;
}
else{
cout <<"x is greater than or equal to y"<< endl;
}
return 0;
}
Output:
X is less than or equal to Y
27. Greater than or equal to (>=)
This operator compares two operands and returns true if the left operand is greater than or equal to the right operand, and false otherwise.
Example:
#include <iostream>
using namespacestd;
int main() {
int x=5;
int y=10;
if(x<=y){
cout <<"x is greater than or equal to y"<< endl;
}
else{
cout <<"x is less than y"<< endl;
}
return 0;
}
Output:
X is greater than or equal Y
Bitwise Operators
Bitwise operators perform bitwise operations on the binary representations of integers. They manipulate the individual bits in the binary representation of a number, rather than the whole number itself.
28. Bitwise AND (&)
The bitwise AND operator compares each bit of two numbers and sets the resulting bit to 1 only if both bits are 1.
Example:#include <iostream>
int main() {
int a=12;// binary: 1100
int b=25;// binary: 11001
int result=a&b;
std::cout <<"Result: "<< result << std::endl;
}
Output:
Result: 8
29. Bitwise OR (|)
The bitwise OR operator compares each bit of two numbers and sets the resulting bit to 1 if either bit is 1.
Example:#include <iostream>
int main() {
int a=12;// binary: 1100
int b=25;// binary: 11001
int result=a|b;
std::cout <<"Result: "<< result << std::endl;
}
Output:
Result: 29
30. Bitwise XOR (^)
The bitwise XOR operator compares each bit of two numbers and sets the resulting bit to 1 only if one of the bits is 1.
Example:#include <iostream>
int main() {
int a=12;// binary: 1100
int b=25;// binary: 11001
int result=a^b;
std::cout <<"Result: "<< result << std::endl;
}
Output:
Result: 21
31. Bitwise NOT (~)
The bitwise NOT operator will reverse the bits of a number.
Example:#include <iostream>
int main() {
int a=12;// binary: 1100
int b=25;// binary: 11001
int result=~a;
std::cout <<"Result: "<< result << std::endl;
}
Output:
Result: -13
32. Left shift (<<)
The left shift operator shifts the bits of a number to the left by a specified number of positions.
Example:
#include <iostream>
int main() {
int a=12;// binary: 1100
int b=25;// binary: 11001
int result=a<<2;
std::cout <<"Result: "<< result << std::endl;
}
Output:
Result: 48
33. Right shift (>>)
The right shift operator shifts the bits of a number to the right by a specified number of positions.
Example:
#include <iostream>
int main() {
int a=12;// binary: 1100
int b=25;// binary: 11001
int result=a>>2;
std::cout <<"Result: "<< result << std::endl;
}
Output:
Result: 3
Using C++ Operators
By understanding the nuances and applications of each operator, you are now equipped to write cleaner, more efficient code. Going forward, we encourage you to bookmark this guide so you can reference it as you work on new projects or encounter unexpected programming hurdles. Feel free to revisit the explanations and examples provided here to refresh your memory and supercharge your C++ coding.
Author's Note: This post was written/edited by a human with the assistance of generative AI.