If you're a developer who's worked with C++, you know how important it is to understand its syntax. And, if you're new to the language, you might be looking at all of the elements in front of you and wondering just how it all works.
As a programming language, C++ is known for its power and flexibility, but it can be complex and overwhelming without a firm grasp of its syntax. In this post, we'll explore the building blocks of C++ syntax, including variables, comments, references, statements, and examples of each one.
Whether you're a beginner or an experienced developer looking to refresh your knowledge, this post will serve as a handy reference for all of your C++ syntax needs.
Table of Contents:
C++ Syntax Overview
The syntax for C++ includes variables, comments, statements, functions, and control structures. Let’s review each of these elements as well as their purpose in the sections below.
What are C++ Variables?
Variables in C++ are used to store data and must be properly declared before they can be used. C++ has several built-in data types, including int, double, char, bool, and others.
Let’s look at these four in more detail.
- Int: This is short for "integer" and it holds whole numbers (positive, negative, or zero) with no fractional part.
- Double: This holds floating-point numbers, which are numbers with decimal places.
- Char: This is short for "character" and it holds individual characters such as letters, numbers, and symbols.
- Bool: This is short for "boolean" and it holds only two values: true or false.
What are C++ Identifiers?
Variables in C++ must be defined with a unique name. To do that, we can use an identifier like x or y.
What are C++ Constants?
Constants are values that can't be modified or changed during the execution of a program. They're used to define values that are known and fixed at compile time. Constants are declared using the const keyword and can be of any data type.
There are two types of constants in C++:
- Literal Constants: These are values that are specified directly in the code, such as numbers or strings. For example: int i = 10; or char c = 'A';
- Symbolic Constants: These are named constants that are declared using the const and #define keywords. They provide a way to associate a name with a value and make code more readable and maintainable.
C++ Comments
Comments add descriptive text within the source code to explain what the program does and why it does it.
There are two types of comments in C++:
1. Single-line Comments: Single-line comments begin with
// and are used for brief comments that only require one line. Everything that comes after
// is treated as a comment and the compiler ignores it.
// This is a single-line comment
2. Multi-line Comments: Multi-line comments are enclosed between /* and */ and can cover multiple lines of code or text.
/* This is a
multi-line comment
*/
C++ References
References are variables that refer to other variables – like an alias. Once a reference is initialized to a variable, it can’t be changed to target another variable. References are used to pass variables to functions without copying data.
Here’s an example:
#include <iostream>
intmain(){
intnumber=10;
// Reference variable
int&ref=number;
std::cout<<"Number: "<<number<<std::endl;// Output: Number: 10
std::cout<<"Reference: "<<ref<<std::endl;// Output: Reference: 10
// Modifying the reference will modify the original variable
ref=20;
std::cout<<"Number: "<<number<<std::endl;// Output: Number: 20
std::cout<<"Reference: "<<ref<<std::endl;// Output: Reference: 20
return0;
}
Output:
Number: 10
Reference: 10
Number: 20
Reference: 20
In this example, we declare an integer variable number and initialize it with a value of 10. Then, we declare a reference variable using ref o refer to the number using the & symbol.
Any changes made to ref will affect the original variable number. For instance, if we modify the value of ref to 20 the value of number is also updated to 20.
C++ Statements
Statements are instructions that tell the computer to perform a specific task. Statements can range from simple variable assignments to complex control structures like loops and conditional statements.
There are many different types of statements you can create, but here are a few of the most common:
1. Expression Statements
When an expression is followed by a semicolon it becomes an expression statement. This statement evaluates the expression and discards the result.
Example:
x = 5; // assigns the value 5 to the variable x
2. Declaration Statements
A declaration of a variable or a function is a declaration statement. It introduces a new identifier into the program.
Example:
inta,b;// declares two integer variables a and b
3. Jump Statements
A jump statement transfers control to another part of the program, such as a function call or loop iteration. The main types of jump statements are break, continue, and return.
Example:
for(inti=0;i<10;i++){
if(i==5){
break;// exits the loop when i equals 5
}
cout<<i<<endl;
}
4. Conditional Statements
Conditional statements allow the program to take different paths based on a condition. In C++, the main type of conditional statement is the if statement.
Example:
if(x>10){
cout<<"x is greater than 10"<<endl;
}else{
cout<<"x is less than or equal to 10"<<endl;
}
5. Loop Statement
A loop statement causes a section of code to be executed repeatedly. The main types of loop statements are for, while, and do-while.
Example:
for(inti=0;i<10;i++){
cout<<i<<endl;
}
6. Compound Statement
A compound statement is a sequence of statements enclosed in curly braces. It allows multiple statements to be treated as a single statement.
Example:
{
inta=5;
intb=10;
cout<<"The sum of a and b is "<<a+b<<endl;
}
7. Break Statement
A break statement causes the innermost loop or switch statement to exit immediately. When this statement encounters a loop or switch, control is transferred to the first statement.
Example:
#include<iostream>
usingnamespacestd;
intmain(){
for(inti=1;i<=10;i++){
if(i==5){
break;// exits the loop when the value of i is 5
}
cout<<i<<endl;
}
return0;
}
Output:
1234
8. Continue Statement
A continue statement causes the current iteration of a loop to end immediately and jumps to the next iteration of the loop.
Example:
#include<iostream>
usingnamespacestd;
intmain(){
for(inti=1;i<=10;i++){
if(i==5){
continue;// skips the rest of the code in the current iteration when i equals 5
}
cout<<i<<endl;
}
}
Output:
1
2
3
4
6
7
8
9
10
Getting Started With C++
C++ syntax is unique, but mastering it will make you a more efficient web developer. For example, variables will help you store data and modify them with constants. Comments will allow you to document code and make it more readable for others. Finally, with references, you can create efficient and flexible code, and use statements to control the program and execute specific actions.
All of these skills are necessary for writing effective and efficient code in C++. Keep this guide handy in case you ever need to brush up on these skills in the future.
Author's Note: This post was written/edited by a human with the assistance of generative AI.
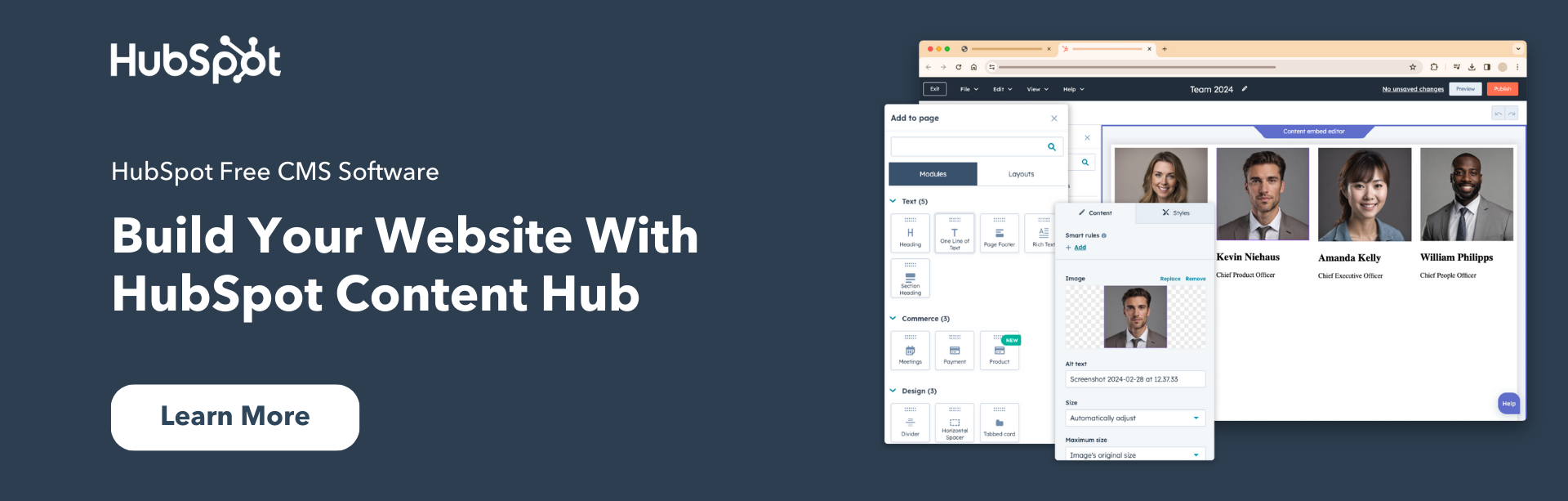
C++ Syntax: Variables, Comments, References, Statements & More
Updated:
Published:
If you're a developer who's worked with C++, you know how important it is to understand its syntax. And, if you're new to the language, you might be looking at all of the elements in front of you and wondering just how it all works.
As a programming language, C++ is known for its power and flexibility, but it can be complex and overwhelming without a firm grasp of its syntax. In this post, we'll explore the building blocks of C++ syntax, including variables, comments, references, statements, and examples of each one.
Whether you're a beginner or an experienced developer looking to refresh your knowledge, this post will serve as a handy reference for all of your C++ syntax needs.
Table of Contents:
C++ Syntax Overview
The syntax for C++ includes variables, comments, statements, functions, and control structures. Let’s review each of these elements as well as their purpose in the sections below.
What are C++ Variables?
Variables in C++ are used to store data and must be properly declared before they can be used. C++ has several built-in data types, including int, double, char, bool, and others.
Let’s look at these four in more detail.
What are C++ Identifiers?
Variables in C++ must be defined with a unique name. To do that, we can use an identifier like x or y.
What are C++ Constants?
Constants are values that can't be modified or changed during the execution of a program. They're used to define values that are known and fixed at compile time. Constants are declared using the const keyword and can be of any data type.
There are two types of constants in C++:
C++ Comments
Comments add descriptive text within the source code to explain what the program does and why it does it.
There are two types of comments in C++:
1. Single-line Comments: Single-line comments begin with // and are used for brief comments that only require one line. Everything that comes after // is treated as a comment and the compiler ignores it.// This is a single-line comment
2. Multi-line Comments: Multi-line comments are enclosed between /* and */ and can cover multiple lines of code or text.
/* This is a
multi-line comment
*/
C++ References
References are variables that refer to other variables – like an alias. Once a reference is initialized to a variable, it can’t be changed to target another variable. References are used to pass variables to functions without copying data.
Here’s an example:
#include <iostream>
intmain(){
intnumber=10;
// Reference variable
int&ref=number;
std::cout<<"Number: "<<number<<std::endl;// Output: Number: 10
std::cout<<"Reference: "<<ref<<std::endl;// Output: Reference: 10
// Modifying the reference will modify the original variable
ref=20;
std::cout<<"Number: "<<number<<std::endl;// Output: Number: 20
std::cout<<"Reference: "<<ref<<std::endl;// Output: Reference: 20
return0;
}
Output:
Number: 10
Reference: 10
Number: 20
Reference: 20
In this example, we declare an integer variable number and initialize it with a value of 10. Then, we declare a reference variable using ref o refer to the number using the & symbol.
Any changes made to ref will affect the original variable number. For instance, if we modify the value of ref to 20 the value of number is also updated to 20.
C++ Statements
Statements are instructions that tell the computer to perform a specific task. Statements can range from simple variable assignments to complex control structures like loops and conditional statements.
There are many different types of statements you can create, but here are a few of the most common:
1. Expression Statements
When an expression is followed by a semicolon it becomes an expression statement. This statement evaluates the expression and discards the result.
Example:
x = 5; // assigns the value 5 to the variable x
2. Declaration Statements
A declaration of a variable or a function is a declaration statement. It introduces a new identifier into the program.
Example:
inta,b;// declares two integer variables a and b
3. Jump Statements
A jump statement transfers control to another part of the program, such as a function call or loop iteration. The main types of jump statements are break, continue, and return.
Example:
for(inti=0;i<10;i++){
if(i==5){
break;// exits the loop when i equals 5
}
cout<<i<<endl;
}
4. Conditional Statements
Conditional statements allow the program to take different paths based on a condition. In C++, the main type of conditional statement is the if statement.
Example:
if(x>10){
cout<<"x is greater than 10"<<endl;
}else{
cout<<"x is less than or equal to 10"<<endl;
}
5. Loop Statement
A loop statement causes a section of code to be executed repeatedly. The main types of loop statements are for, while, and do-while.
Example:
for(inti=0;i<10;i++){
cout<<i<<endl;
}
6. Compound Statement
A compound statement is a sequence of statements enclosed in curly braces. It allows multiple statements to be treated as a single statement.
Example:
{
inta=5;
intb=10;
cout<<"The sum of a and b is "<<a+b<<endl;
}
7. Break Statement
A break statement causes the innermost loop or switch statement to exit immediately. When this statement encounters a loop or switch, control is transferred to the first statement.
Example:
#include<iostream>
usingnamespacestd;
intmain(){
for(inti=1;i<=10;i++){
if(i==5){
break;// exits the loop when the value of i is 5
}
cout<<i<<endl;
}
return0;
}
Output:
1234
8. Continue Statement
A continue statement causes the current iteration of a loop to end immediately and jumps to the next iteration of the loop.
Example:
#include<iostream>
usingnamespacestd;
intmain(){
for(inti=1;i<=10;i++){
if(i==5){
continue;// skips the rest of the code in the current iteration when i equals 5
}
cout<<i<<endl;
}
}
Output:
1
2
3
4
6
7
8
9
10
Getting Started With C++
C++ syntax is unique, but mastering it will make you a more efficient web developer. For example, variables will help you store data and modify them with constants. Comments will allow you to document code and make it more readable for others. Finally, with references, you can create efficient and flexible code, and use statements to control the program and execute specific actions.
All of these skills are necessary for writing effective and efficient code in C++. Keep this guide handy in case you ever need to brush up on these skills in the future.
Author's Note: This post was written/edited by a human with the assistance of generative AI.
Don't forget to share this post!
Related Articles
16 Math Functions That Every C Programmer Should Know
10 Essential String Functions for C Programmers
55 Standard Library Functions for C Programmers
How to Write Functions in C Programming
C++ String Functions: 10 Definitions + Examples
C++ Math Functions: 20 Definitions + Examples
C++ Class Methods & Constructors: Everything You Need to Know
C++ Functions List: 20 Definitions & Examples
How to Call a Function in C++
33 C++ Operators [Definitions + Examples]