If you're a web developer, you know that functions are an essential component of any programming language -- and C++ is no exception.
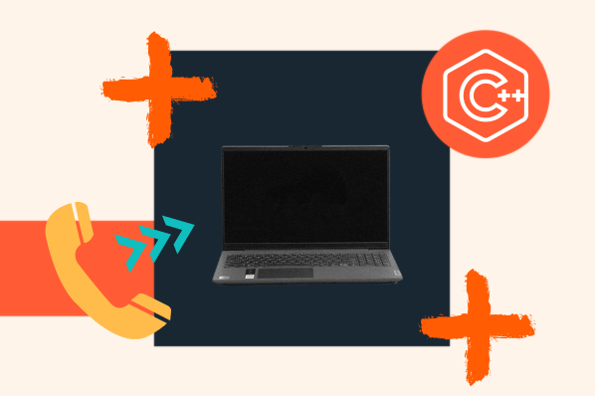
Knowing how to call functions properly in C++ will help you design stunning web pages, create interactive app interfaces, and call specific data from large databases with ease. With that in mind, we've composed this comprehensive guide on how to call a function in C++. In this post, you'll learn how to use arguments and return types and how to apply functions in various ways to C++ projects.
Without further ado, let's get started.
Build your website with HubSpot's Free CMS Software
Table of Contents:
- How to Call a Function in C++
- C++ Function Example
- C++ Declarations & Definitions
- C++ Function Parameters
How to Call a Function in C++
To call a function in C++, you need to provide the name of the function, along with any necessary arguments or parameters. The syntax for calling a function is:
function_name(argument1, argument2, ..., argumentN);
function_name is the name of the function you want to call, and argument1, argument2, ..., argumentN are any inputs or parameters that the function takes. If the function takes no parameters, you can simply use an empty pair of parentheses after the function name.
C++ Function Example
For example, consider the following C++ function that takes two integers as input, adds them together, and returns the result:
#include <iostream>
int add(int x, int y) {
return x + y;
}
To call this function from another part of the program, you would use the following syntax:
int a = 5, b = 7;
int sum = add(a, b);
Here, a and b are two integer variables that are passed as arguments to the add function. The function then returns the sum of a and b, which is stored in the sum variable.
Keep in mind that the type and number of arguments that a function takes must match the type and number of arguments that are passed to it when calling the function. Otherwise, it will result in a compilation or runtime error.
Functions in C++ are composed of two key elements: declarations and definitions. We’ll explore both in the section below.
C++ Function Declarations & Definitions
A function declaration declares the name, return type, and parameter list of a function to the compiler. It tells the compiler what the function looks like without defining its implementation. This is important because it lets the compiler know how to call the function correctly when the function is used in other parts of the program.
The syntax for a function declaration is:
return_type function_name(parameter_list);
In this example, int is the return type of the function, add is the name of the function, and (int x, int y) is the parameter list that specifies two integer parameters called “x” and “y.”
int add(int x, int y);
A function definition is a complete implementation of a function that describes its behavior and how it’s executed. It includes the function name, return type, parameter list, and the body of the function. The function body contains instructions that are executed when the function is called.
The syntax for a function definition is:
return_type function_name(parameter_list) { // function body }
In the next example, int is the return type of the function, add is the name of the function, (int x, int y) is the parameter list, and the function body is return x + y;, which returns the sum of “x” and “y.”
int add(int x, int y) {
return x + y;
}
In the last few examples, we referred to an element called the “parameter list.” Let’s explore what that is in the next section.
C++ Function Parameters
Function parameters are input variables that are passed to functions so they can execute specific tasks. Parameters act as variables inside the function, and their values are used to manipulate data or perform calculations.
Function parameters are defined in the function declaration and definition, and are specified within the parentheses following the function name.
The syntax for function parameters in C++ goes as follows:
return_type function_name(parameter_type parameter_name) { // function body }
parameter_type is the data type of the parameter, and parameter_name is the name of the parameter. Multiple parameters can be specified by separating them with commas.
For example, this function takes two integer parameters and returns their sum:
int addNumbers(int x, int y) {
int sum = x + y;
return sum;
}
int x and int y are the function parameters, and they perform the addition operation inside the function body. The return statement returns the sum of the two numbers to the calling function.
Remember, when calling a function with parameters, the arguments passed to the function must match the data type and order of the parameters in the function definition.
Passing a Function as a Parameter With Function Pointers
In C++, a function can be passed as a parameter to another function just like any other data type. This is called a function pointer, and it allows you to pass a function as an argument to another function, or to store a function address in a variable.
The syntax for defining a function pointer in C++ is:
return_type (*function_name)(parameter_list);
return_type is the return type of the function, function_name is the name of the function pointer, and parameter_list is the list of parameters that the function takes.
To pass a function as a parameter to another function, you need to define the function parameter as a function pointer. Here's an example:
#include <iostream>
int calculate(int x, int y, int (*func)(int, int)) {
int result = func(x, y);
return result;
}
int add(int x, int y) {
return x + y;
}
int subtract(int x, int y) {
return x - y;
}
int main() {
int a = 10, b = 5; // pass the add function as a parameter to the calculate function
int sum = calculate(a, b, add);
std::cout << "Sum: " << sum << std::endl; // pass the subtract function as a parameter to the calculate function
int difference = calculate(a, b, subtract);
std::cout << "Difference: " << difference << std::endl;
return 0;
}
Output:
Sum: 15
Difference: 5
In this example, the calculate function takes three parameters: x, y, and a function pointer, func, that takes two integer parameters and returns an integer.
func performs the calculation on x and y. Two functions, add and subtract, are declared as function pointers.
In the main function, the calculate function is called twice with different functions passed as the third parameter. The add function calculates the sum of a and b, while the subtract function returns the difference between a and b.
Pass Array to Function
You can also use a function pointer to pass an array to a function.
void function(int *array) {
// Do something with the array
}
The array parameter is a pointer to the first element of the array. This means that any changes you make to the array within the function will be reflected in the original array.
In this example, we can use a function to print the elements of an array:
#include <iostream>
void function(int arr[], int size);
int main() {
int array[] = {1, 2, 3, 4, 5};
function(array, 5);
for (int i = 0; i < 5; i++) {
std::cout << array[i] << std::endl;
}
return 0;
}
void function(int arr[], int size) {
for (int i = 0; i < size; i++) {
arr[i] *= 2;
}
}
Output:
2
4
6
8
10
Passing a Reference as a Parameter
To pass a reference parameter to a function, you need to use & in the function declaration. The syntax for that is:
void function_name(type ¶meter_name) { // function body }
type is the data type of the parameter, and ¶meter_name is the reference parameter.
Creating Functions in C++
By now, you should be comfortable with calling C++ functions in several different ways. Going forward, just remember to always carefully consider your approach to functions to ensure efficient and effective code. Keep practicing and experimenting in your programming projects and you'll be a master of C++ function calling in no time.
Author's Note: This post was written/edited by a human with the assistance of generative AI.