JavaScript is a powerful programming language, and as with any like it, it needs to handle many data types. To facilitate this, JavaScript offers ways to store data collections, like with the array data type.
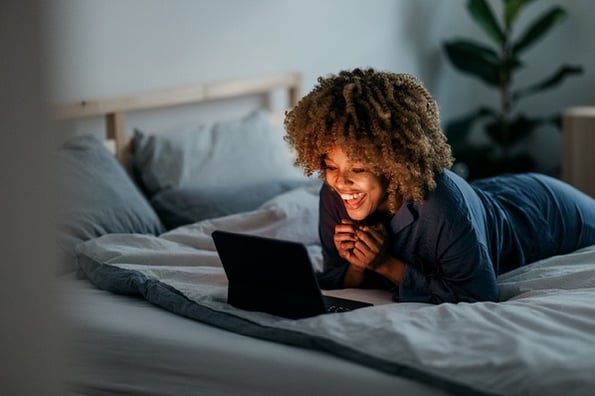
This post explains how to interact with array data using array methods. We'll review a comprehensive list of different methods and explain how they work. You will also see some examples of the syntax and an explanation of the behavior.
Let’s dive in.
How to Use JavaScript Arrays
JavaScript arrays are used to store data collections and are robust by design. They are powerful enough to work with any type or size of data, and because of this capability, there are several ways to interact with them. The more robust the array is, the more you can do with it.
This is how we arrive at JavaScript array methods, as you can use these functions to apply arrays to several tasks.
Let’s discuss array methods and why you will need them in your JavaScript software development.
What are JavaScript Array Methods?
JavaScript array methods are simple functions within the JavaScript programming language used on arrays to create different outcomes, handle tasks, and more.
JavaScript arrays only come with three static methods, which means those methods belong to the original class. This behavior is contrary to non-static methods, which do not belong to the array class. Instead, the non-static methods are used on the instance of a class.
Let’s break this down. When you create an array, the process uses the class blueprint to create an instance of the class. An array instance is an array you create with access to static and other methods.
For example, one static method from the array class is called isArray(), which identifies arrays and belongs to the array class. However, the concat() method is not in the array class and is used on several data types.
JavaScript Array Methods: 15 Definitions & Examples
This section will review the top 15 most common JavaScript array methods programmers use in their software development. The following image is a nice little cheat sheet of the methods you will see examples of.
Now that you understand what the above methods do, it’s a good time to look at some examples of how they behave. Below is a list of examples, one example for each of the above methods.
Use this handy Code Pen module to test out some of these JavaScript array methods.
See the Pen Filter Method by HubSpot (@hubspot) on CodePen.
1. Some Method: some()
The some method checks for any passing elements.
const ages = [3, 10, 18, 20];
ages.some(age => age > 5);
/*Would return boolean true*/
2. Every Method: every()
The every method checks if every element in an array passes a test.
const ages = [3, 10, 18, 20];
console.log(ages.every(age => age > 2));
/*Would return true*/
3. Reduce Method: reduce()
The reduce method reduces arrays to a single value.
const ages = [0, 1, 2, 3, 4];
ages.reduce((acc, curVal, id, arr) => acc + curVal, 1);
/*Would return 11*/
4. Map Method: map()
The Map method creates a new array from a function run on each array element.
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(value => value * 2);
/*Creates a new array with original values doubled*/
5. Flat Method: flat()
The flat method flattens multidimensional arrays to a dictated level.
const arr4 = [1, 2, [3, 4, [5, 6, [7, 8, [9, 10]]]]]
console.log(arr4.flat(1));
/*Would return (5) [1, 2, 3, 4, [5, 6, [7, 8, [9, 10]]]]*/
console.log(arr4.flat(2));
/*Would return (7) [1, 2, 3, 4, 5, 6, [7, 8, [9, 10]]]*/
console.log(arr4.flat(Infinity));
/*Would return (10) [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]*/
6. Filter Method: filter()
The array filter method tests an array and returns any element that satisfies the test parameters.
const array = [-3, -2, -1, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13];
console.log(array.filter(num => num > 0 && num <= 10));
/*Would return (10) [1,2,3,4,5,6,7,8,9,10]*/
7. For Each Method: forEach()
The forEach() method executes a function for each element in an array.
const primeNums = [2,3,5,7,11,13];
array.forEach((item) => console.log(item));
/*Would print each array item to the console*/
8. Find Index Method: findIndex()
The find index method returns the index of the first element in an array to pass a test.
const nums = [4, 6, 8, 9, 12];
nums.findIndex( num => num == 4));
/*Would return 0 which is the index of the item with value 4*/
9. Find Method: find()
The find method tests an array and returns the first element that satisfies the test parameters.
const nums = [1, 2, 3, 4]
console.log(nums.find( num => num == 3 ))
/*Would return the matching value 3, no match returns undefined*/
10. Sort Method: sort()
The sort method in JavaScript is used to organize items in an array.
const nums = [4, 2, 5, 1, 3]
nums.sort((a, b) => a - b)
/*Would return original array modified with values sorted*/
11. Concat Method: concat()
The concat method returns an array of one or more joined arrays.
const alpha = ['a', 'b', 'c'];
const numeric = [1, 2, 3];
const alphaNumeric = alpha.concat(numeric);
console.log(alphaNumeric)
12. Fill Method: fill()
The fill method fills values with the provided value from start to end.
const original = [1, 2, 3]
const result = original.fill(4)
/*Would modify array, and return with all values changed to provided value*/
13. Includes Method: includes()
The includes method checks if an array contains a specific element.
const nums = [1, 2, 3];
console.log(nums.includes(2));
/*Would return true*/
console.log(nums.includes(4));
/*Would return false*/
14. Reverse Method: reverse()
The reverse method reverses the order of elements in an array.
const nums = [1, 2, 3];
console.log(nums.reverse());
/*Would return array with values in reverse order*/
15. Flat Map Method: flatMap()
The flat map method returns a function against each array element and then flattens it.
const array = [[1], [2], [3], [4], [5]]
const a = array.flatMap(arr => arr * 10)
/*With .flat() and .map(), identical in behavior to the above*/
const b = array.flat().map(arr => arr * 10)
/*Both would return [10, 20, 30, 40, 50]*/
Looking for more array methods? Here are a few that we dive a bit deeper into:
Getting Started With JavaScript Array Methods
We've learned a lot about what JavaScript array methods are and how they work. We talked about the syntax and the structure of different methods, and you saw a cheat sheet of the fifteen most popular methods for arrays. Finally, you saw each method used with an example to help drive the concepts home.
Moving forward, you can use that cheat sheet as a reference point to practice different tasks. Bookmark it and add to it over time, as it's a great way to keep track of those neat little tricks and tips. Before you know it, you will begin committing things to memory in no time.