Like many languages, JavaScript comes with various ways to loop — or iterate — through data collections. Iterating over arrays is a task you will frequently need to complete in your programming journey.
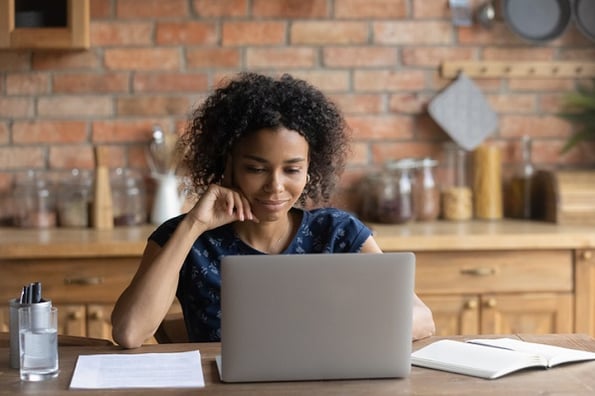
In this post, you will discover the forEach() method, what it does, and what that can mean for you and your software. You will also see some code snippets showcasing syntax, use cases, and a codepen with live examples of how to use it. The links below can be used to help you navigate through the post.
- For Each Syntax
- For Each Parameter Syntax
- How to Use For-Each in JavaScript
- JavaScript For-Each Examples
Let’s get started.
What is JavaScript For-each?
The JavaScript forEach() method is a powerful method used to modify and interact with data collections. The method is chained to a variable — collection objects — and iterates over each item in the array until it ends.
The forEach method enables the modification of data collections such as arrays, sets, and object collections. However, it can be used for several other purposes, such as displaying information, creating new data collections, and even confirming information. These are not the only ways the forEach method can be used, but more on that later.
The forEach loop has a fairly standard syntax. However, it can look a little confusing at first due to its nature. So, next, let's look at the syntax of the forEach loop and build on that further by looking at some examples.
For-each Syntax
The syntax of the JavaScript forEach method is relatively straightforward. It accepts one function as its parameter and a value typically referred to as thisValue. However, before we dive into what that means, let’s see what this looks like in its most basic form.
array.forEach(function(currVal, index, arr), thisValue)
In this code snippet, we have an array with the generic name array and chained onto it is the forEach method.
The forEach method accepts:
- function (required): This function provides the action to perform for each item in the target collection.
- thisValue (optional): This parameter is used to identify a this value, which can be accessed within the loop using the this keyword.
Within the forEach method is a generic function that accepts three parameters of its own. Let’s break this function down to understand how it works.
For-each Parameter Syntax
The forEach function parameter:
The function parameter looks very familiar because it is the standard syntax for declaring or calling a function. Developers can do this one of two ways; the first is to write the function directly into the forEach method, as shown below.
firstNames.forEach(
function(currVal, id, arr) {
/*do neat stuff here*/
},
thisVal);
The other option is to write the function outside the scope of the forEach method and then call it the function as the forEach parameter, which you can see below.
/*Declaring the function, and calling it as the forEach param*/
array.forEach(coolFunct);
function coolFunct (item) {
/*do neat stuff here*/
}
Now let's go over the parts of the function itself to understand better what it needs to work correctly.
function(currVal, id, arr)
- currVal (required): This value holds the current iteration item in the loop.
- index (optional): This value is the index location of the item in the current iteration.
- arr (optional): This value contains the array that the current item belongs to.
Now that we’ve discussed the first parameter of the forEach method, let’s look at the following optional parameter, thisValue.
The forEach thisValue parameter:
thisVal
- thisVal (optional): Allows access to a JavaScript object outside the forEach loop.
Now that you know what parameters the forEach method accepts and how they work, let's discuss how to use it.
How to Use For-each in JavaScript
Using the forEach method to loop through collections of information simplifies the vital task of data management in any software. But how does this affect you and your work as a software developer?
Some examples of using a forEach method to iterate over information include displaying user information and collating data. With that in mind, you might find it challenging to identify when to use the forEach method over other looping approaches.
The traditional loops that come with JavaScript allow breaking out of or ending them conditionally. Two great examples of this would be using the break and return keywords which signal that the loop should end.
In contrast, the forEach loop does not allow the premature ending of its execution. Instead, once the loop starts, the loop will run until completion in every case — excluding a breaking error occurrence.
JavaScript For-each Examples
The following codepen is an excellent example of using the forEach method to its maximum potential. This example includes both the optional parameters of the forEach method and the required function it accepts.
These codepens provide the HTML, CSS and JavaScript used to create the visual preview that you will see. Feel free to ignore the HTML and CSS and focus primarily on JavaScript. If you find yourself curious about either, you can find posts on HTML and CSS here.
See the Pen Untitled by HubSpot (@hubspot) on CodePen.
Getting Started With Using the JavaScript For-each Loop
This post covers the basics of forEach loops and how you can use them in your software development. You’ve learned what a for each loop is, starting with the basic syntactic structure, and its optional and required parameters. Additionally, you know how to use the function and the parameter in a meaningful way.