The JavaScript programming language is jam-packed with features, functions, methods, objects, and more, which make programming much easier for all developers.
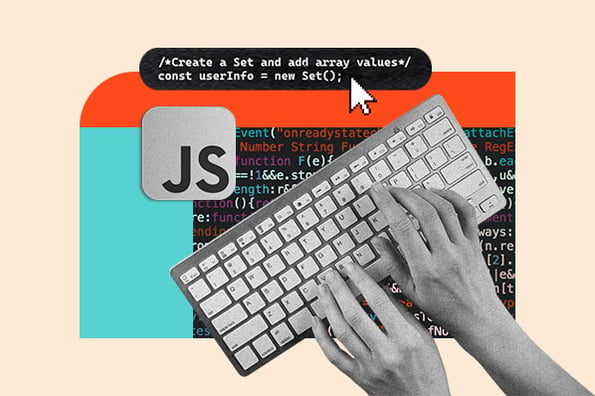
In this post, we will discuss the benefits of the JavaScript set object and how to use it. We will also cover how it works and how to use it in your programs or software. Furthermore, we will look at the methods that manipulate and modify the set object during development.
Without any further delay, let’s jump right into the meat of this post.
What is a set in JavaScript?
JavaScript is a powerful programming language that offers a variety of ways to manage data and collections of data. One of those ways is the JavaScript object known as sets, which is used specifically on data collections. If you aren't familiar with JavaScript you should check out our post on the basics of JavaScript.
Like any other object in JavaScript, there are rules about how sets function and how they are used. Sets are data objects that hold a collection of unique values.
Sets can hold data of any type, but may not contain duplicate items. In the case of adding duplicate and equal items, only the first instance will be saved to the set.
JavaScript is an object-oriented programming language. Meaning, almost everything in it are objects, including sets. The only things in JavaScript that are not objects are the primitive data types — null, undefined, strings, numbers, boolean, and symbols. Primitive data types are frequently used as items within data collections as they are used to document types of information and their values.
Now that you have some background information on sets and their place in the Javascript world, let's look at some examples and discuss how you can use sets in your programming.
JavaScript Set
JavaScript sets can contain any number of items in any data type. Let’s look at some examples of how you can create a new set in your programs.
The following examples will show how to use a set to store a user’s entered data for a hypothetical video game. The following image is a preview of the working codepen at the end of this post and shows an example of creating a set in JavaScript.
In this game, a user would need to enter information about their character, such as their height, name, age, eye color, and more. This is a great way to depict how to use a set optimally. Since many objects in JavaScript can contain any type of data, you can store a set object within another object. Let's create a set object to contain all of the character’s unique non-repeating information.
Let's start by looking at how to use the new keyword to initialize a new set object.
/*Create a Set and add array values*/
const userInfo = new Set();
The above line instantiates a new empty set object, but often you will want to create a new set with values already present. Let’s look at how to create a new set with multiple values.
const userInfo = new Set([
"Play Time: 6hrs", "Deaths: 6"
]);
*Noteworthy caveat: This approach limits the way you can format the values, the numbers in the values here will get represented as strings.
This line of code will create a set with two string objects, which is useful but has limitations on how you can access the values of each item. For example, you might want to be able to access the Play Time and its value 6hrs separately. This code would make that difficult. Instead, you could store each as their item. The following is an example of how you could do that.
const userInfo = new Set([
"Play Time:", "6hrs", "Deaths:", 6
]);
Creating a set with values using the new keyword requires the data to be wrapped in square brackets.
This is more useful for your purposes. It separates the identifier from its value and lets you use an integer as one of our values. This is important because it allows you to set values of multiple data types, which builds on the usefulness of a set. The flexibility of a set is expanded further by adding values as arrays, this would allow you to simulate key-value pairs.
Let’s look at how to create character information for this game using arrays as values.
/*Create a Set and add array values*/
const characterInfo = new Set([
["Name:", "Rick Sanchez"],
["Age:", 70],
["Height:", "6ft 5in"],
["Occupation:", "Scientist"]
]);
The line above creates a new variable named characterInfo that contains a new set object for the character information. The set contains a few pieces of information that should only be declared once for each character, with non-repeating data.
*Noteworthy caveat: In this example, the set information is formatted as four two-item arrays, which essentially act as key-value pairs. With sets, two or more arrays can contain the same values because each array object is treated as a unique item.
Javascript Set Properties
The JavaScript set only comes with one built-in property called the size property. The size property identifies the number of items within the set object. This property works on any set with any data types as items.
The following example shows how to use the set property size on the set created above and print the result to the console.
console.log(characterInfo.size)
This value dictates the number of iterations when looping through a set to access its information, more on that soon.
JavaScript Set Intersection
JavaScript does not provide a native intersection method for the set object and, as such, you’ll need to create a way to perform that function.
The following video explains how you can accomplish an intersection on set objects.
JavaScript Set Methods
There are a lot of methods that manipulate sets and the values within them. Since set items are not immutable, any item within a set is modifiable even after they are added to the set object.
There are quite a few methods. Let's touch softly on what they are and how they work.
- add(): Adds a new item to a set object
- delete(): Removes an item from a set object
- has(): Returns true if a item/value exists (unpredictable on array items)
- clear(): Removes all items from a set object
- values(): Returns an iterator with all the items in a set (the method keys() does the same)
- entries(): Returns an iterator with the [value,value] pairs from a Set (unpredictable with array items)
Iterate Through JavaScript Set
The forEach() method works on this object type as well, which makes it easy to iterate through. Let's look at how to use the forEach() method on the set object created earlier.
characterInfo.forEach((value) => {
console.log(value[0] + " " + value[1]);
})
This would print out each of the two-value arrays from the set object, adding a space between the values for readability.
It is possible to iterate over a set using a for-of loop as well, let's look at that using the userInfo set created earlier in the post.
for (let item of userInfo){
console.log(item);
}
Since the data format in the userInfo set is broken into different pieces for usability purposes the loop process differs from iterating over array items within the set. The above code will iterate through the set for each item within it and log them to the console.
With all of the information above, let’s look at a codepen that shows a working example of this information within a web development environment. Adding JavaScript for loops to your code can expand the flexibility of your sets.
See the Pen JavaScript Set by HubSpot (@hubspot) on CodePen.
Using The JavaScript Set Object
JavaScript sets are powerful and come with several useful methods for manipulating them and the data within them. There are some important points to keep in mind when considering if a set will work for your data collections. Let's look at a few that will inform your decisions on using sets.
- Sets are collections of unique values.
- Sets are not immutable, they can be changed as needed after creation and the items within can be changed after adding them.
- Sets can contain values of any data type and do not have keys associated with the values.
- Set items that are arrays can contain duplicate values.
- Set item arrays may be identical to each other since they are considered separate and unique objects.
- There are many methods for set objects that manipulate and modify set items.
- There are multiple ways to iterate over sets.
Now, you have all the information you need to start using sets in your code. You are encouraged to take advantage of the codepen created for this post. It is a great way to play with sets and learn how they behave.