An array is a collection of values like integers, strings, or even other arrays. Arrays group elements together, making them easy to manage, modify, and manipulate.
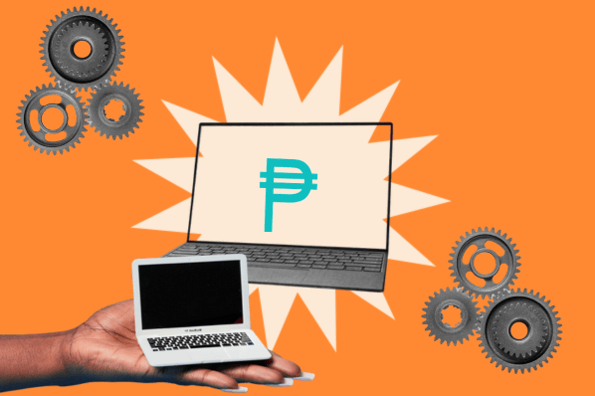
As you'll see from the list below, there are tons of array functions in PHP and it’s tough to keep track of them all. That’s why we’ve put together this cheat sheet so you can quickly reference your options as you program different projects.
From creating arrays to calculating with them, this post serves as a handy resource for all of your PHP array needs.
Table of Contents:
PHP Array Functions
Here’s a complete list of PHP array functions. We know there are a lot, so check out the quick links and table of contents above if you’re looking for a specific one.
Creating Arrays
1. array()
The array() function is used to create an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
echo "Fruits: ";
foreach ($fruits as $fruit) {
echo $fruit . " ";
}
?>
Output:
Fruits: apple banana cherry
2. array_combine()
The array_combine() function creates a new array by combining two arrays where one array provides keys and the other provides values.
Example:
<?php
$keys = array("a", "b", "c");
$values = array(1, 2, 3);
$combined = array_combine($keys, $values);
echo "Combined Array: ";
foreach ($combined as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Combined Array: [a => 1] [b => 2] [c => 3]
3. array_chunk()
array_chunk() splits an array into bits of a specified size and returns a multi-dimensional array with those bits.
Example:
<?php
$numbers = array(1, 2, 3, 4, 5, 6, 7, 8);
$chunks = array_chunk($numbers, 3);
echo "Chunks of Array: ";
foreach ($chunks as $chunk) {
echo "[" . implode(", ", $chunk) . "] ";
}
?>
Output:
Chunks of Array: [1, 2, 3] [4, 5, 6] [7, 8]
4. compact()
The compact() function creates an array by using variables as keys and their values as the corresponding array values.
Example:
<?php
$name = "John";
$age = 30;
$info = compact("name", "age");
echo "Info Array: ";
foreach ($info as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Info Array: [name => John] [age => 30]
5. range()
range() generates an array containing a start and an end value, with optional step increments.
Example:
<?php
$even_numbers = range(2, 10, 2);;
echo "Even Numbers: ";
foreach ($even_numbers as $number) {
echo $number . " ";
}
?>
Output:
Even Numbers: 2 4 6 8 10
6. list()
The list() function is used to assign values to a list of variables from an array. It's often used in conjunction with functions like explode() or preg_match() to extract values from arrays easily.
Example:
<?php
$info = array("John", "Doe", 30);
list($first_name, $last_name, $age) = $info;
echo "First Name: " . $first_name . "; ";
echo "Last Name: " . $last_name . "; ";
echo "Age: " . $age . "; ";
?>
Output:
First Name: John Last Name: Doe Age: 30
7. array_fill()
array_fill() creates an array with a specified number of elements, each having the same value.
Example:
<?php
$filledArray = array_fill(0, 3, "hello");
echo "Filled Array: ";
foreach ($filledArray as $item) {
echo $item . " ";
}
?>
Output:
Filled Array: hello hello hello
8. array_fill_keys()
array_fill_keys() creates an array using specified keys and the same value for each key.
Example:
<?php
$keys = array("a", "b", "c");
$filledArray = array_fill_keys($keys, "hello");
echo "Filled Array with Keys: ";
foreach ($filledArray as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Filled Array with Keys: [a => hello] [b => hello] [c => hello]
9. array_flip()
array_flip() flips the keys and values of an array, creating a new array where the original values become keys, and the original keys become values.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$flipped = array_flip($fruits);
echo "Flipped Array: ";
foreach ($flipped as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Flipped Array: [apple => 0] [banana => 1] [cherry => 2]
10. array_slice()
array_slice() extracts a portion of an array based on a starting index and length, creating a new array containing the selected elements.
Example:
<?php
$fruits = array("apple", "banana", "cherry", "date", "fig");
$slice = array_slice($fruits, 1, 3);
echo "Sliced Array: ";
foreach ($slice as $item) {
echo $item . " ";
}
?>
Output:
Sliced Array: banana cherry date
Comparing Arrays
11. array_diff()
array_diff() calculates the difference between two or more arrays and returns an array containing the values that are present in the first array but not in the other arrays.
Example:
<?php
$arr1 = array("a", "b", "c");
$arr2 = array("b", "c", "d");
$difference = array_diff($arr1, $arr2);
echo "Array Difference: ";
foreach ($difference as $item) {
echo $item . " ";
}
?>
Output:
Array Difference: a
12. array_diff_key()
array_diff_key() computes the difference of arrays using keys for comparison and returns an array containing values from the first array whose keys are not present in the other arrays.
Example:
<?php
$arr1 = array("a" => 1, "b" => 2, "c" => 3);
$arr2 = array("b" => 2, "c" => 4, "d" => 5);
$difference = array_diff_key($arr1, $arr2);
echo "Array Difference by Key: ";
foreach ($difference as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Array Difference by Key: [a => 1]
13. array_diff_assoc()
array_diff_assoc() computes the difference of arrays with an additional index check.
Example:
<?php
$arr1 = array("a" => 1, "b" => 2, "c" => 3);
$arr2 = array("b" => 2, "c" => 4, "d" => 5);
$difference = array_diff_assoc($arr1, $arr2);
echo "Array Difference (assoc): ";
foreach ($difference as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Array Difference (assoc): [a => 1] [c => 3]
14. array_intersect()
The array_intersect() function calculates the intersection of two or more arrays and returns an array containing values that are present in all input arrays.
Example:
<?php
$arr1 = array("a", "b", "c");
$arr2 = array("b", "c", "d");
$intersection = array_intersect($arr1, $arr2);
echo "Array Intersection: ";
foreach ($intersection as $item) {
echo $item . " ";
}
?>
Output:
Array Intersection: b c
15. array_intersect_assoc()
array_intersect_assoc() computes the intersection of arrays with an additional index check, comparing both values and keys.
Example:
<?php
$arr1 = array("a" => 1, "b" => 2, "c" => 3);
$arr2 = array("b" => 2, "c" => 4, "d" => 5);
$intersection = array_intersect_assoc($arr1, $arr2);
echo "Array Intersection (assoc): ";
foreach ($intersection as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Array Intersection (assoc): [b => 2]
16. array_intersect_key()
array_intersect_key() computes the intersection of arrays using keys for comparison and returns an array containing values from the first array that have matching keys in the other arrays.
Example:
<?php
$arr1 = array("a" => 1, "b" => 2, "c" => 3);
$arr2 = array("b" => 2, "c" => 4, "d" => 5);
$intersection = array_intersect_key($arr1, $arr2);
echo "Array Intersection by Key: ";
foreach ($intersection as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Array Intersection by Key: [b => 2] [c => 3]
17. array_key_exists()
array_key_exists() checks if a specified key exists and returns true if the key is found, and false otherwise.
Example:
<?php
$person = array("name" => "John", "age" => 30, "country" => "USA");
$exists = array_key_exists("age", $person);
echo "Key 'age' Exists: " . ($exists ? "Yes" : "No");
?>
Output:
Key 'age' Exists: Yes
18. in_array()
The in_array() function checks if a specified value exists in an array. It returns true if the value is found, and false if otherwise.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$result = in_array("banana", $fruits);
echo "Value 'banana' Exists: " . ($result ? "Yes" : "No");
?>
Output:
Value 'banana' Exists: Yes
19. array_search
array_search() looks for a value in an array and returns the corresponding key if the value is found. If the value is not found, it returns false.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$key = array_search("banana", $fruits);
echo "Key for 'banana': " . $key;
?>
Output:
Key for 'banana': 1
20. array_count_values()
The array_count_values() function counts the frequency of values in an array and returns an associative array where the keys are the unique values from the input array.
Example:
<?php
$numbers = array(1, 2, 2, 3, 3, 3);
$counted = array_count_values($numbers);
echo "Counted Values: ";
foreach ($counted as $value => $count) {
echo "$value: $count, ";
}
?>
Output:
Counted Values: 1: 1, 2: 2, 3: 3,
Modifying Arrays
21. array_merge()
The array_merge() function merges two or more arrays into a single array.
Example:
<?php
$arr1 = array("a", "b");
$arr2 = array("c", "d");
$merged = array_merge($arr1, $arr2);
echo "Merged Array: ";
foreach ($merged as $item) {
echo $item . " ";
}
?>
Output:
Merged Array: a b c d
22. array_merge_recursive()
array_merge_recursive() is used to merge multidimensional arrays.
Example:
<?php
$arr1 = array("a" => "apple", "b" => "banana");
$arr2 = array("b" => "blueberry", "c" => "cherry");
$merged = array_merge_recursive($arr1, $arr2);
echo "Merged Array (Recursive): ";
foreach ($merged as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
["a" => "apple", "b" => ["banana", "blueberry"], "c" => "cherry"]
23. array_replace()
array_replace() replaces the values of the first array with the values from the second array for matching keys.
Example:
<?php
$arr1 = array("a" => "apple", "b" => "banana");
$arr2 = array("b" => "blueberry", "c" => "cherry");
$replaced = array_replace($arr1, $arr2);
echo "Replaced Array: ";
foreach ($replaced as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Replaced Array: [a => apple] [b => blueberry] [c => cherry]
24. array_push()
array_push() adds one or more elements to the end of an array.
Example:
<?php
$fruits = array("apple", "banana");
array_push($fruits, "cherry", "date");
echo "Updated Array: ";
foreach ($fruits as $fruit) {
echo $fruit . " ";
}
?>
Output:
Updated Array: apple banana cherry date
25. array_reduce()
array_reduce() reduces an array to a single value using a callback function.
Example:
<?php
$numbers = array(1, 2, 3, 4, 5);
$sum = array_reduce($numbers, function ($carry, $item) {
return $carry + $item;
});
echo "Sum of Numbers: " . $sum;
?>
Output:
Sum of Numbers: 15
26. array_pop()
The array_pop() function removes and returns the last element from an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$lastFruit = array_pop($fruits);
echo "Last Fruit Removed: " . $lastFruit;
?>
Output:
Last Fruit Removed: cherry
27. array_shift()
The array_shift() function removes and returns the first element from an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$firstFruit = array_shift($fruits);
echo "First Fruit Removed: " . $firstFruit;
?>
Output:
First Fruit Removed: apple
28. array_unshift()
array_unshift() adds one or more elements to the beginning of an array.
Example:
<?php
$fruits = array("banana", "cherry");
array_unshift($fruits, "apple", "date");
echo "Updated Array: ";
foreach ($fruits as $fruit) {
echo $fruit . " ";
}
?>
Output:
Updated Array: apple date banana cherry
29. arsort()
arsort() sorts an associative array in descending order, preserving key-value associations.
Example:
<?php
$fruits = array("banana" => 2, "cherry" => 1, "apple" => 3); arsort($fruits);
echo "Sorted Array (Reverse): ";
foreach ($fruits as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Sorted Array (Reverse): [apple => 3] [banana => 2] [cherry => 1]
30. asort()
asort() sorts an associative array in ascending order.
Example:
<?php
$fruits = array("banana" => 2, "cherry" => 1, "apple" => 3); asort($fruits);;
echo "Sorted Array: ";
foreach ($fruits as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Sorted Array: [cherry => 1] [banana => 2] [apple => 3]
31. rsort()
rsort() sorts an indexed array in descending order.
Example:
<?php
$numbers = array(5, 3, 1, 4, 2);
rsort($numbers);
echo "Reverse Sorted Numbers: ";
foreach ($numbers as $number) {
echo $number . " ";
}
?>
Output:
Reverse Sorted Numbers: 5 4 3 2 1
32. usort()
usort() sorts an indexed array using a user-defined comparison function.
Example:
<?php
$numbers = array(5, 3, 1, 4, 2);
usort($numbers, function($a, $b) {
return $a - $b;
});
echo "Sorted Numbers (User-Defined): ";
foreach ($numbers as $number) {
echo $number . " ";
}
?>
Output:
Sorted Numbers (User-Defined): 1 2 3 4 5
33. array_multisort()
array_multisort() performs a multi-dimensional sorting of an array.
Example:
<?php
$names = array("John", "Alice", "Bob");
$ages = array(30, 25, 35);
array_multisort($names, $ages);
echo "Sorted Names: ";
foreach ($names as $name) {
echo $name . " ";
}
echo "; ";
echo "Sorted Ages: ";
foreach ($ages as $age) {
echo $age . " ";
}
?>
Output:
Sorted Names: Alice Bob John ; Sorted Ages: 25 35 30
34. shuffle()
shuffle() randomly shuffles the elements of an array.
Example:
<?php
$cards = array("A", "2", "3", "4", "5");
shuffle($cards);
echo "Shuffled Cards: ";
foreach ($cards as $card) {
echo $card . " ";
}
?>
Output:
Shuffled Cards: 5 2 3 A 4
35. natsort()
This function sorts an array using a "natural order" algorithm, which is useful for sorting arrays with numbers.
Example:
<?php
$fruits = array("apple10", "apple2", "apple1");
natsort($fruits);
echo "Naturally Sorted Fruits: ";
foreach ($fruits as $fruit) {
echo $fruit . " ";
}
?>
Output:
Naturally Sorted Fruits: apple1 apple2 apple10
36. array_filter()
array_filter() filters the elements of an array using a callback function and returns a new array containing only the elements that pass the callback's test.
Example:
<?php
$numbers = array(1, 2, 3, 4, 5);
$filtered = array_filter($numbers, function($n) {
return $n % 2 == 0; // Filter even numbers.
});
echo "Filtered Numbers (Even): ";
foreach ($filtered as $number) {
echo $number . " ";
}
?>
Output:
Filtered Numbers (Even): 2 4
37. array_pad()
array_pad() pads an array to a specified length with a specified value.
Example:
<?php
$numbers = array(1, 2, 3);
$padded = array_pad($numbers, 5, 0);
echo "Padded Numbers: ";
foreach ($padded as $number) {
echo $number . " ";
}
?>
Output:
Padded Numbers: 1 2 3 0 0
38. array_map()
array_map() applies a callback function to each element of an array and returns a new array containing the results of the callback function for each element.
Example:
<?php
$numbers = array(1, 2, 3, 4, 5);
$squared = array_map(function($n) {
return $n * $n;
}, $numbers);
echo "Squared Numbers: ";
foreach ($squared as $number) {
echo $number . " ";
}
?>
Output:
Squared Numbers: 1 4 9 16 25
39. array_change_key_case()
This function changes the case of keys in an array to either lowercase or uppercase.
Example:
<?php
$fruits = array("apple" => 2, "Banana" => 3, "CHERRY" => 1);
$lowercaseKeys = array_change_key_case($fruits, CASE_LOWER);
echo "Array with Lowercase Keys: ";
foreach ($lowercaseKeys as $key => $value) {
echo "[$key => $value] ";
}
?>
Output:
Array with Lowercase Keys: [apple => 2] [banana => 3] [cherry => 1]
40. array_reverse()
The reverse array function reverses the order of elements in an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$reversed = array_reverse($fruits);
echo "Reversed Fruits: ";
foreach ($reversed as $fruit) {
echo $fruit . " ";
}
?>
Output:
Reversed Fruits: cherry banana apple
41. array_splice()
array_splice() removes a portion of an array and replaces it with new elements. It can also return the removed elements as a new array.
Example:
<?php
$fruits = array("apple", "banana", "cherry", "date", "fig");
$removed = array_splice($fruits, 2, 2, array("grape", "kiwi"));
echo "Updated Fruits: ";
foreach ($fruits as $fruit) {
echo $fruit . " ";
}
echo "; ";
echo "Removed Fruits: ";
foreach ($removed as $fruit) {
echo $fruit . " ";
}
?>
Output:
Updated Fruits: apple banana grape kiwi fig ; Removed Fruits: cherry date
42. array_unique()
array_unique() removes duplicate values from an array and returns a new array with only the unique values.
Example:
<?php
$numbers = array(1, 2, 3, 2, 4, 5, 5);
$uniqueNumbers = array_unique($numbers);;
echo "Unique Numbers: ";
foreach ($uniqueNumbers as $number) {
echo $number . " ";
}
?>
Output:
Unique Numbers: 1 2 3 4 5
43. array_walk()
array_walk() applies a user-defined callback function to each element of an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
array_walk($fruits, function(&$value) {
$value = strtoupper($value);
});
echo "Modified Fruits: ";
foreach ($fruits as $fruit) {
echo $fruit . " ";
}
?>
Output:
Modified Fruits: APPLE BANANA CHERRY
Array Calculations & Returns
44. array_keys()
array_keys() returns all the keys or a subset of keys from an associative array.
Example:
<?php
$fruits = array("apple" => 2, "banana" => 3, "cherry" => 1);
$keys = array_keys($fruits);
echo "Array Keys: ";
foreach ($keys as $key) {
echo $key . " ";
}
?>
Output:
Array Keys: apple banana cherry
45. array_column()
array_column() extracts the values from a single column of a multi-dimensional array and returns an array containing those values.
Example:
<?php
$people = array(
array("name" => "John", "age" => 30),
array("name" => "Alice", "age" => 25),
array("name" => "Bob", "age" => 35)
);
$names = array_column($people, "name");
echo "Names: ";
foreach ($names as $name) {
echo $name . " ";
}
?>
Output:
Names: John Alice Bob
46. array_rand()
array_rand() returns one or more random keys from an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry", "date", "fig");
$randomKey = array_rand($fruits);
echo "Random Key: " . $randomKey;
?>
Output:
Random Key: 4
47. array_product()
This function calculates the product of all the values in an array.
Example:
<?php
$numbers = array(1, 2, 3, 4, 5);
$product = array_product($numbers);
echo "Product of Numbers: " . $product;
?>
Output:
Product of Numbers: 120
48. array_sum()
array_sum() calculates the sum of all the values in an array.
Example:
<?php
$numbers = array(1, 2, 3, 4, 5);
$sum = array_sum($numbers);
echo "Sum of Numbers: " . $sum;
?>
Output:
Sum of Numbers: 15
49. count()
count() returns the number of elements in an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$count = count($fruits);
echo "Number of Fruits: " . $count;
?>
Output:
Number of Fruits: 3
50. current()
current() returns the current element's value in an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$current = current($fruits);
echo "Current Fruit: " . $current;
?>
Output:
Current Fruit: apple
51. key()
key() returns the current element's key in an array.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$key = key($fruits);
echo "Current Key: " . $key;
?>
Output:
Current Key: 0
52. extract()
The extract function imports variables into the current symbol table from an associative array, using the array keys as variable names and values as variable values.
Example:
<?php
$person = array("name" => "John", "age" => 30);
extract($person);
echo "Name: " . $name . ", Age: " . $age;
?>
Output:
Name: John, Age: 30
53. next()
This function advances the internal pointer of an array to the next element and returns that element's value.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$nextFruit = next($fruits);
echo "Next Fruit: " . $nextFruit;
?>
Output:
Next Fruit: banana
54. prev()
prev() moves the internal pointer of an array to the previous element and returns that element's value.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
end($fruits);
$prevFruit = prev($fruits);
echo "Previous Fruit: " . $prevFruit;
?>
Output:
Previous Fruit: banana
55. end()
end() moves the internal pointer of an array to the last element and returns that element's value.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$lastFruit = end($fruits);
echo "Last Fruit: " . $lastFruit;
?>
Output:
Last Fruit: cherry
56. reset()
reset() moves the internal pointer of an array to the first element and returns that element's value.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$firstFruit = reset($fruits);
echo "First Fruit: " . $firstFruit;
?>
Output:
First Fruit: apple
57. each()
each() returns the current key-value pair from an array and advances the internal pointer to the next element. Note: This function has been removed in PHP versions 7.2 and later – try using foreach instead.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
$pair = each($fruits);
echo "Key: " . $pair['key'] . ", Value: " . $pair['value'];
?>
Output:
Key: 0, Value: apple
Key: 1, Value: banana
Key: 2, Value: cherry
58. file()
file() reads an entire file into an array, with each line of the file as an element in the array.
Example:
<?php
$lines = file("example.txt");
foreach ($lines as $line) {
echo $line . "\n";
}
?>
Output:
$lines is an array with each line of the file as an element
PHP Array Constants
59. SORT_ASC
This constant specifies ascending order.
Example:
<?php
$numbers = array(5, 3, 1, 4, 2);
sort($numbers, SORT_ASC);
foreach ($numbers as $number) {
echo $number . " ";
}
?>
Output:
1 2 3 4 5
60. SORT_DESC
This constant specifies descending order.
Example:
<?php
$numbers = array(5, 3, 1, 4, 2);
rsort($numbers, SORT_DESC);
foreach ($numbers as $number) {
echo $number . " ";
}
?>
Output:
5 4 3 2 1
61. SORT_NUMERIC
This constant indicates that elements should be treated as numeric values when sorting. It ensures that the values are compared numerically rather than as strings.
Example:
<?php
$values = array("10", "2", "25", "7");
sort($values, SORT_NUMERIC);
foreach ($values as $value) {
echo $value . " ";
}
?>
Output:
2 7 10 25
62. SORT_REGULAR
This constant indicates that elements should be compared in their original data types without any special treatment as numbers or strings.
Example:
<?php
$values = array("10", "2", "25", "7");
sort($values, SORT_REGULAR);
foreach ($values as $value) {
echo $value . " ";
}
?>
Output:
2 7 10 25
63. CASE_LOWER
This constant specifies that keys should be converted to lowercase.
Example:
<?php
$fruits = array("Apple" => "red", "Banana" => "yellow");
$lowercaseKeys = array_change_key_case($fruits, CASE_LOWER);
foreach ($lowercaseKeys as $key => $value) {
echo "Key: " . $key . ", Value: " . $value . "\n";
}
?>
Output:
Key: apple, Value: red
Key: banana, Value: yellow
64. CASE_UPPER
This constant specifies that keys should be converted to uppercase.
Example:
<?php
$fruits = array("apple" => "red", "banana" => "yellow");
$uppercaseKeys = array_change_key_case($fruits, CASE_UPPER);
foreach ($uppercaseKeys as $key => $value) {
echo "Key: " . $key . ", Value: " . $value . "\n";
}
?>
Output:
Key: APPLE, Value: red
Key: BANANA, Value: yellow
Getting Started With PHP Array Functions
As you can see, there’s a lot that you can accomplish with arrays in PHP, and knowing how to create and edit them is crucial to enhancing your overall programming skills. Keep this resource handy so you can quickly recall functions when you’re in need and improve your coding skills over time.