In PHP programming, you often need to manage or manipulate date and time values. However, with the vast array of functions at your fingertips, it can be tricky to keep track of all of the options available.
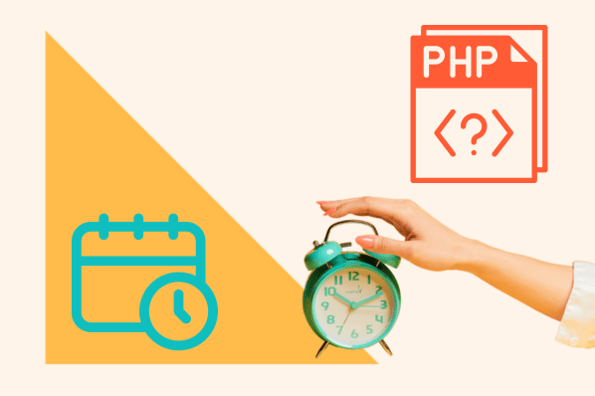
That’s why we’ve put together this list of date and time functions so you can quickly reference them as needed while programming. We've also included a brief review of how to obtain date and time values and how to set a timezone for your PHP project.
How to Get the Date & Time in PHP
There are two ways to obtain the date and time in PHP. You can use the date() function or the DateTime class.
Using the date() Function
The date() function obtains the current date and time as a formatted string. It also allows you to specify the format of the output.
Here's an example:
$currentDate = date('Y-m-d H:i:s');
echo "Current date and time: $currentDate";
Output:
Current date and time: 2023-10-16 15:36:02
In this example, Y represents the year, m represents the month, d represents the day, H represents the hour, i represents minutes, and s represents seconds.
Using the DateTime Class
The DateTime class offers a bit more flexibility. Not only can you format the output to fit your needs, but you can also specify your timezone which is important when dealing with dates and times in different locations around the world.
Let’s look at an example:
$dateTime = new DateTime();
$currentDate = $dateTime->format('Y-m-d H:i:s');
echo "Current date and time: $currentDate";
Output:
Current date and time: 2023-10-16 15:38:32
Here, we created a DateTime object. We can now use it to set the time zone to New York, Paris, Hong Kong, or wherever we need to. Let’s explore that in the next example.
How to Set a Timezone
To set a timezone, we’ll need to apply the DateTimeZone() function to the DateTime object.
Example:
$dateTime = new DateTime('now', new DateTimeZone('America/New_York'));
$currentTime = $dateTime->format('H:i:s');
echo "Current time in New York: $currentTime";
Output:
Current time in New York: 16:45:21
With the DateTimeZone() function set to America/New_York, this code will display the current time in New York City. If we changed it to Europe/London it would provide us with the time in London.
For a complete list of date and time functions in PHP, check out the section below. Or, use this handy table to jump to the exact function that you’re looking for.
PHP Date & Time Functions
1. date()
The date() function formats the current date and time.
Example:
<?php
$currentDate = date('Y-m-d H:i:s');
echo "Current date and time: " . $currentDate;
?>
Output:
Current date and time: 2023-10-16 14:13:13
2. time()
The time() function returns the current time in seconds since the Unix Epoch.
Example:
<?php
$currentTimestamp = time();
echo "Current Unix timestamp: " . $currentTimestamp;
?>
Output:
Current Unix timestamp: 1697033672
3. strtotime()
This function parses a string into a Unix timestamp.
Example:
<?php
$dateString = "2023-10-15 15:30:00";
$timestamp = strtotime($dateString);
echo "Unix timestamp for $dateString: " . $timestamp;
?>
Output:
Unix timestamp for 2023-10-15 15:30:00: 1697383800
4. mktime()
This function returns the Unix timestamp for a date.
Example:
<?php
$timestamp = mktime(12, 30, 0, 10, 15, 2023);
echo "Unix timestamp for October 15, 2023, 12:30 PM: " . $timestamp;
?>
Output:
Unix timestamp for October 15, 2023, 12:30 PM: 1697373000
5. checkdate()
This function validates a Gregorian calendar date.
Example:
<?php
$month = 2;
$day = 29;
$year = 2024;
if (checkdate($month, $day, $year)) {
echo "The date is valid: $year-$month-$day";
} else {
echo "The date is not valid: $year-$month-$day";
}
?>
Output:
The date is valid: 2024-2-29
6. strftime()
This function formats a local time and/or date according to local settings. Note: This function has been deprecated in recent PHP versions.
Example:
<?php
setlocale(LC_TIME, 'en_US.UTF-8');
$formattedDate = strftime('%A, %B %d, %Y %H:%M:%S');
echo "Formatted date: $formattedDate";
?>
Output:
Formatted date: Wednesday, October 16, 2023 14:53:47
7. getdate()
The getdate() function returns date/time information.
Example:
<?php
$dateInfo = getdate();
print_r($dateInfo);
?>
Output:
Array
(
[seconds] => 47
[minutes] => 54
[hours] => 14
[mday] => 16
[wday] => 3
[mon] => 10
[year] => 2023
[yday] => 283
[weekday] => Wednesday
[month] => October
[0] => 1697036087
)
8. gettimeofday()
This function returns the current time.
Example:
<?php
$timeInfo = gettimeofday();
print_r($timeInfo);
?>
Output:
Array
(
[sec] => 1697036130
[usec] => 773136
[minuteswest] => 0
[dsttime] => 0
)
9. gmdate()
gmdate() formats a GMT/UTC date/time.
Example:
<?php
$utcDate = gmdate('Y-m-d H:i:s');
echo "UTC date and time: $utcDate";
?>
Output:
UTC date and time: 2023-10-16 14:57:31
10. gmmktime()
This function returns the Unix timestamp for a GMT date.
Example:
<?php
$timestamp = gmmktime(12, 30, 0, 10, 15, 2023);
echo "UTC timestamp for October 15, 2023, 12:30 PM: $timestamp";
?>
Output:
UTC timestamp for October 15, 2023, 12:30 PM: 1697373000
16. gmstrftime()
This function formats a GMT/UTC time and/or date. Note: This function has been deprecated in recent PHP versions.
Example:
<?php
setlocale(LC_TIME, 'en_US.UTF-8');
$utcFormattedDate = gmstrftime('%A, %B %d, %Y %H:%M:%S');
echo "Formatted UTC date: $utcFormattedDate";
?>
Output:
Formatted UTC date: Wednesday, October 16, 2023 14:58:24
12. idate()
The idate() function formats a local time/date according to local settings.
Example:
<?php
$date = idate('H', time()); // Use 'H' for the hour
echo "Current hour: $date";
?>
Output:
Current hour: 15
13. microtime()
microtime() returns the current Unix timestamp with microseconds.
Example:
<?php
$microtime = microtime(true);
echo "Current Unix timestamp with microseconds: $microtime";
?>
Output:
Current Unix timestamp with microseconds: 1697036452.6927
14. strptime()
This function parses a time/date generated with strftime().
Example:
<?php
$dateString = '2023-10-15 15:30:00';
$format = 'Y-m-d H:i:s';
$date = DateTime::createFromFormat($format, $dateString);
echo "Parsed Date: " . $date->format($format);
?>
Output:
Parsed Date: 2023-10-15 15:30:00
15. date_add()
The date_add() function adds days, months, years, hours, minutes, and seconds to a date.
Example:
<?php
$date = new DateTime('2023-10-15');
$date->add(new DateInterval('P3D')); // Add 3 days
echo "New Date: " . $date->format('Y-m-d');
?>
Output:
New Date: 2023-10-18
16. date_create()
This function returns a new DateTime object.
Example:
<?php
$date = date_create('2023-10-15');
echo "Created Date: " . date_format($date, 'Y-m-d');
?>
Output:
Created Date: 2023-10-15
17. date_diff()
This function returns the difference between two DateTime objects.
Example:
<?php
$date1 = new DateTime('2023-10-15');
$date2 = new DateTime('2023-10-20');
$interval = $date1->diff($date2);
echo "Difference: " . $interval->format('%R%a days');
?>
Output:
Difference: +5 days
18. date_format()
This function returns a date formatted according to a given format.
Example:
<?php
$date = new DateTime('2023-10-15');
$formattedDate = date_format($date, 'l, F j, Y');
echo "Formatted Date: " . $formattedDate;
?>
Output:
Formatted Date: Sunday, October 15, 2023
19. date_sunrise()
The date_sunrise() function returns the time of sunrise for a given day and location. Note: This function is deprecated in recent PHP versions.
Example:
<?php
$latitude = 37.7749; // Latitude for San Francisco
$longitude = -122.4194; // Longitude for San Francisco
$sunrise = date_sunrise(time(), SUNFUNCS_RET_STRING,$latitude,$longitude);
echo "Sunrise time: $sunrise";
?>
Output:
Sunrise time: 14:13
20. date_sunset()
The date_sunset() function returns the time of sunset for a given day and location. Note: This function is deprecated in recent PHP versions.
Example:
<?php
$latitude = 37.7749; // Latitude for San Francisco
$longitude = -122.4194; // Longitude for San Francisco
$sunset = date_sunset(time(), SUNFUNCS_RET_STRING, $latitude, $longitude);
echo "Sunset time: $sunset";
?>
Output:
Sunset time: 01:39
21. date_default_timezone_get()
This function returns the default timezone used by all date/time functions in a script.
Example:
<?php
$timezone = date_default_timezone_get();
echo "Default Time Zone: $timezone";
?>
Output:
Default Time Zone: UTC
22. date_default_timezone_set()
This sets the default timezone used by all date/time functions in a script.
Example:
<?php
date_default_timezone_set('America/New_York');
$timezone = date_default_timezone_get();
echo "Updated Default Time Zone: $timezone";
?>
Output:
Updated Default Time Zone: America/New_York
23. date_modify()
This function alters the timestamp of a DateTime object.
Example:
<?php
$date = new DateTime('2023-10-15');
$date->modify('+3 days');
echo "Modified Date: " . $date->format('Y-m-d');
?>
Output:
Modified Date: 2023-10-18
24. date_offset_get()
This function returns the timezone offset from GMT of the given DateTime object.
Example:
<?php
$date = new DateTime('2023-10-15', new DateTimeZone('America/New_York'));
$offset = $date->getOffset();
echo "Time Zone Offset: " . $offset;
?>
Output:
Time Zone Offset: -14400
25. date_parse()
Date_parse() returns an associative array with detailed info about a given date.
Example:
<?php
$dateString = '2023-10-15 15:30:00';
$dateInfo = date_parse($dateString);
print_r($dateInfo);
?>
Output:
Array
(
[year] => 2023
[month] => 10
[day] => 15
[hour] => 15
[minute] => 30
[second] => 0
[fraction] => 0
[warning_count] => 0
[warnings] => Array
(
)
[error_count] => 0
[errors] => Array
(
)
[is_localtime] =>
)
26. date_parse_from_format()
This function returns an associative array with detailed info about a date formatted according to a specified format.
Example:
<?php
$dateString = '15-10-2023';
$format = 'd-m-Y';
$dateInfo = date_parse_from_format($format, $dateString);
print_r($dateInfo);
?>
Output:
Array
(
[year] => 2023
[month] => 10
[day] => 15
[hour] =>
[minute] =>
[second] =>
[fraction] =>
[warning_count] => 0
[warnings] => Array
(
)
[error_count] => 0
[errors] => Array
(
)
[is_localtime] =>
)
27. date_sub()
The date_sub() function subtracts days, months, years, hours, minutes, and seconds from a date.
Example:
<?php
$date = new DateTime('2023-10-15');
$interval = new DateInterval('P3D'); // Subtract 3 days
$date->sub($interval);
echo "New Date: " . $date->format('Y-m-d');
?>
Output:
New Date: 2023-10-12
28. date_timestamp_get()
This function retrieves the Unix timestamp from a DateTime object.
Example:
<?php
$dateTime = new DateTime("2023-10-06 15:30:00");
$timestamp = date_timestamp_get($dateTime);
echo "Unix Timestamp: " . $timestamp;
?>
Output:
Unix Timestamp: 1696606200
29. date_timestamp_set()
This sets the date and time based on an Unix timestamp.
Example:
<?php
$timestamp = 1634264400; // Unix timestamp for '2023-10-15 12:00:00'
$date = new DateTime();
$date->setTimestamp($timestamp);
echo "Set Date: " . $date->format('Y-m-d H:i:s');
?>
Output:
Set Date: 2021-10-15 02:20:00
30. date_timezone_set()
This function sets the time zone for a DateTime object.
Example:
<?php
$dateTime = new DateTime("2023-10-06 15:30:00");
$timezone = new DateTimeZone("America/New_York");
date_timezone_set($dateTime, $timezone);
echo "Date and Time in New York: " . $dateTime->format("Y-m-d H:i:s") . " ";
echo "Time Zone: " . $dateTime->getTimezone()->getName();
?>
Output:
Date and Time in New York: 2023-10-06 16:30:00 Time Zone: America/New_York
31. date_days_in_month()
This function returns the number of days in a month for a given year and calendar. Note: this function is provided by the Calendar extension, which may not be enabled by default in all PHP installations.
Example:
<?php
$year = 2023;
$month = 2; // February
$daysInMonth = cal_days_in_month(CAL_GREGORIAN, $month, $year);
echo "Days in February 2023: $daysInMonth";
?>
Output:
Days in February 2023: 28
32. date_isodate_set()
The date_isodate_set() function creates a DateTime object by specifying the year, ISO week number, and ISO day number.
Example:
<?php
$date = date_isodate_set(new DateTime(), 2023, 40, 2);
echo "ISO Year: " . $date->format("Y") . " ";
echo "ISO Week Number: " . $date->format("W") . " ";
echo "ISO Day Number: " . $date->format("N") . " ";
echo "Full Date: " . $date->format("Y-m-d") . " ";
?>
Output:
ISO Year: 2023 ISO Week Number: 40 ISO Day Number: 2 Full Date: 2023-10-03
33. date_date_set()
This sets the date to a specified value.
Example:
<?php
$date = new DateTime();
$date->setDate(2023, 10, 15); // Set the date to October 15, 2023
echo "Set Date: " . $date->format('Y-m-d');
?>
Output:
Set Date: 2023-10-15
34. date_get_last_errors()
This function returns the warnings and errors in the last date-related function.
Example:
<?php
$dateString = '2023-13-32'; // Invalid date
$date = date_create($dateString);
$errors = date_get_last_errors();
print_r($errors);
?>
Output:
Array
(
[warning_count] => 0
[warnings] => Array
(
)
[error_count] => 1
[errors] => Array
(
[6] => Unexpected character
)
)
35. date_interval_format()
This function formats the interval.
Example:
<?php
$interval = new DateInterval('P2Y3M4DT5H6M7S'); // A sample interval
$formattedInterval = $interval->format('%Y years, %M months, %D days');
echo "Formatted Interval: " . $formattedInterval;
?>
Output:
Formatted Interval: 02 years, 03 months, 04 days
36. date_locale_set()
The date_locale_set() function sets the locale information.
Example:
<?php
$locale = 'en_US';
setlocale(LC_TIME, $locale);
echo "Current Locale: " . setlocale(LC_TIME, 0); // Display the set locale
?>
Output:
Current Locale: C
37. date_interval_create_from_date_string()
This function creates a DateInterval object based on a date interval string.
Example:
<?php
$intervalString = "2 weeks 3 days 4 hours 30 minutes";
$interval = date_interval_create_from_date_string($intervalString);
echo "Interval: " . $interval->format("%y years, %m months, %d days, %h hours, %i minutes");
?>
Output:
Interval: 0 years, 0 months, 17 days, 4 hours, 30 minutes
38. date_timezone_get()
The date_timezone_get() function returns the time zone associated with a DateTime object.
Example:
<?php
$dateTime = new DateTime("2023-10-06 15:30:00");;
$timezone = date_timezone_get($dateTime);
echo "Time Zone: " . $timezone->getName();
?>
Output:
Time Zone: UTC
Finding Date & Time Values in PHP
These functions will help you schedule tasks, calculate durations, and track events based on specific dates and times. They'll also enable you to manipulate values, allowing you to compare individual dates or extract information from a specified time period.
The more you use them, the more comfortable you'll be at recalling them. Until then, feel free to bookmark this page and use it as a handy reference to guide your programming over time -- pun intended.