You won’t get very far in PHP without learning how to use functions.
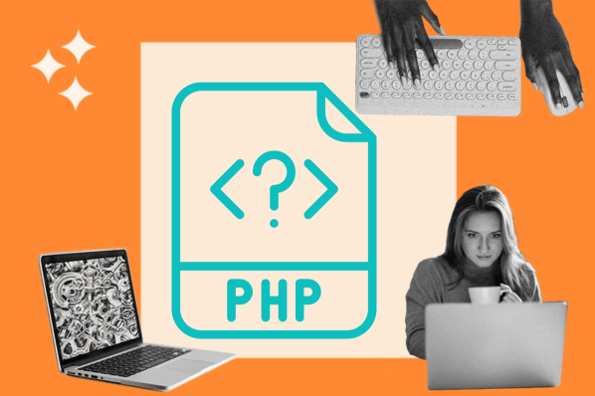
Functions are the building blocks of most coding languages and they enable you to execute a range of tasks from math calculations to string manipulation.
PHP is no exception, and there are plenty of functions to choose from as well as important components that help make them operate.
In this post, we’ll go over the basics of using PHP functions, including the different types and how to call them in your code. Then, we’ll point you to a directory of PHP functions as well as go over a short list of miscellaneous functions that you’ll want to keep handy.
Table of Contents
How to Use Functions in PHP
First, let’s review the difference between built-in and anonymous functions. Then, we’ll explain how to call functions in PHP and include elements that help your code operate like parameters and arguments.
What are PHP built-in functions?
Built-in functions are pre-defined functions that are included within the core PHP language. These functions are used without any special requirements or additional installation.
PHP has a wide range of built-in functions that perform various tasks, including:
- String Functions: These functions manipulate strings. Examples include strlen() to calculate string length, strtoupper() to convert strings to uppercase, and substr() to extract a portion of a string.
- Mathematical Functions: PHP provides numerous functions for mathematical operations. Some popular ones include sqrt() for calculating the square root, pow() for raising a number to a power, and rand() for generating random numbers.
- Array Functions: These functions are used for working with arrays, like array_push() to add elements to an array, array_pop() to remove and return the last element of an array, and array_sum() to calculate the sum of all elements in an array.
- Date and Time Functions: These functions deal with dates and times. Examples include date() to get the current date and time, strtotime() to parse date and time descriptions, and mktime() to create a Unix timestamp.
- File System Functions: PHP provides functions for working with the file system. Some common ones include file_exists() to check if a file exists, fopen() to open a file, fwrite() to write to a file, and fclose() to close a file.
- Database Functions: These include functions like mysqli_connect() to establish a connection to a MySQL database,mysqli_query() to execute a SQL query, and mysqli_fetch_assoc() to fetch results from a database query.
What are anonymous functions?
Anonymous functions, also known as closures, are functions without names. They can be assigned to variables or called directly, making them useful for creating functions on the fly.
The general syntax for creating an anonymous function in PHP is as follows:
$functionVariable = function (/* parameters */) { // function body };
What are arrow functions?
Arrow functions, also known as short closures, were implemented in PHP 7.4. They define anonymous functions in a more concise way. These functions enable you to create short, single-expression functions without the need for the function keyword or curly braces.
The basic syntax for an arrow function is as follows:
fn (argument_list) => expression
PHP Function Arguments
Function arguments are variables that are passed to a function when it’s called. These arguments provide input to the function and can be used within its code to perform specific actions or calculations.
How to Pass Variables to Functions
To pass a variable to a function, start with defining the function and specifying its parameters – like so:
<?php
$name = "Alice";
function greet($name) {
echo "Hello, " . $name . "!";
}
?>
Here, $name is the function argument. When greet() is called, its value, “Alice” is passed as an argument and assigned to the $name variable within the function.
PHP Function Parameters
Function parameters are placeholders or variables declared within the parentheses of a function. They define the input required by the function when it’s called and pass values or variables into the function for processing.
Let’s revisit that last example.
function greet($name) {
echo "Hello, " . $name . "!";
}
$name is also a function parameter. It serves as a placeholder for the value that will be passed when calling the greet() function. The value passed to this function is assigned to $name which personalizes the message.
How to Call Functions in PHP
To call a function, first make sure the function is defined in your PHP code. Functions are defined before they are called, either in the same file or in an included file.
Then, add the function name followed by parentheses that may contain any required arguments.
For example, the greet() function doesn't require any arguments, so you can call it like this:
<?php
greet();
?>
If the function takes arguments, then pass them within the parentheses. For instance, if you have a function called sum(), you can call it like this:
<?php
$result = sum(3, 5);
?>
If the function returns a value, you can assign it to a variable, like we did above. Alternatively, you can directly use the function call wherever you need the returned value.
How to Create User-Defined Functions in PHP
A user-defined function is a function that is created by a programmer to perform a specific set of tasks or calculations. Unlike built-in functions, which are provided by the programming language or libraries, user-defined functions are customized to suit their specific needs.
To create a user-defined function, start by adding the function keyword, followed by the desired name for your function.
Choose a meaningful and descriptive name that reflects the purpose of the function. For example, let's create a function called calculateSum():
<?php
function calculateSum() {
// Function body goes here
}
?>
If your function requires input from a user, you can define parameters within the parentheses after the function name. Parameters act as placeholders for values that are passed into the function when it’s called.
Once the function is defined, add your logic inside the function body. This will be the code that runs when the function is called. Use the parameters, if any, to perform calculations or operations.
For instance, let’s have our calculateSum() function calculate the sum of two numbers:
<?php
function calculateSum($num1, $num2) {
$sum = $num1 + $num2;
echo "The sum is: " . $sum;
}
?>
To use the user-defined function, simply call it by its name followed by parentheses, providing any required arguments, like so:
<?php
calculateSum(3, 5);
?>
There are a ton of PHP functions at your disposal, so covering them all in one blog post is less than ideal. Instead, we’ve put together this table of contents below that directs you to different types of functions like math, array, and string functions. Use these links to navigate to the exact function you’re looking for, or check out the list below of miscellaneous PHP functions.
PHP Miscellaneous Functions
1. Define Function: define()
This function defines a constant. Constants are identifiers that do not change during the execution of a script.
Example:
<?php
define("PI", 3.14159);
echo PI; // Outputs: 3.14159
?>
Output:
3.14159
2. Sleep Function: sleep()
The sleep function pauses the execution of a script for a specified number of seconds.
Example:
<?php
echo "Start\n";
sleep(2); // Pause for 2 seconds
echo "End\n";
?>
Output:
Start
End
3. Exit Function: exit()
This function terminates the script's execution immediately. It can also be used with an optional status code.
Example:
<?php
echo "Before exit\n";
exit(1); // Exit with status code 1
echo "After exit\n"; // This line won't be executed
?>
Output:
Before exit
4. Constant Function: constant()
This function retrieves the value of a constant defined using define().
Example:
<?php
define("GREETING", "Hello, World!");
echo constant("GREETING"); // Outputs: Hello, World!
?>
Output:
Hello, World!
5. Evaluation Function: eval()
This function evaluates a string as PHP code. Note: Due to security concerns, this function is not commonly used.
Example:
<?php
$x = 5;
$y = 10;
$result = eval("return $x * $y;");
echo $result; // Outputs: 50
?>
Output:
50
6. Pack Function: pack()
This function packs data into binary format, which is useful for low-level data manipulation.
Example:
<?php
$packedData = pack("C*", 65, 66, 67);
echo $packedData; // Outputs: ABC
?>
Output:
ABC
7. Unpack Function: unpack()
This function unpacks binary data previously combined with the pack function.
Example:
<?php
$packedData = "ABC";
$unpackedData = unpack("C*", $packedData);
print_r($unpackedData);
?>
Output:
Array
(
[1] => 65
[2] => 66
[3] => 67
)
8. Highlight File Function: highlight_file()
This is used to display the source code of a file.
Example:
<?php
highlight_file("example.php");
?>
Output:
Source code of example.php
9. Get Browser Function: get_browser()
This function obtains information about the user's browser.
Example:
<?php
$browserInfo = get_browser();
print_r($browserInfo);
?>
Output:
Returns information about user’s browser.
10. Unique ID Function: uniqid()
This function generates a unique identifier based on the current timestamp.
Example:
<?php
$uniqueId = uniqid();
echo $uniqueId;
?>
Output:
653696b0d4d80
Getting Started With PHP Functions
As a PHP programmer, you’ll rely on functions to queue up tasks and execute commands within your code. The more familiar you are with each type of function and how to call them, the more efficient you’ll be at completing dynamic and complex projects. Keep this resource handy and check out the other reference pages we’ve provided for a complete list of PHP functions.