PHP, or "Hypertext Preprocessor," is the backbone of countless dynamic web applications and websites. And, at its core, PHP relies on a set of fundamental building blocks called keywords which wield immense power in shaping the behavior of your code.
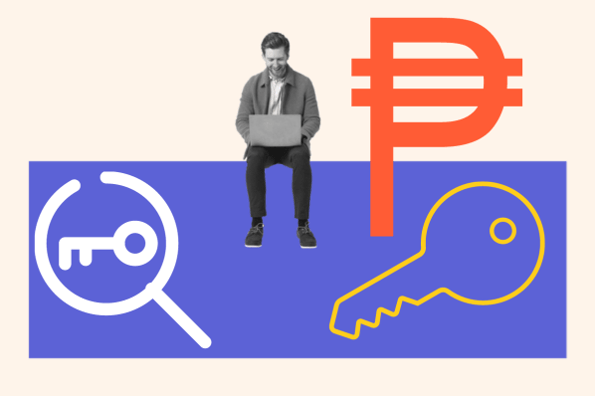
PHP keywords enable developers to write efficient, maintainable, and powerful code. In fact, you can’t get too far in PHP without picking up what these elements are and what they do for your programs.
In this post, we've compiled a comprehensive list of PHP keywords so you can quickly review what each one does as well as an example of it being used in action.
Scroll down to review each one individually, or use the table below to jump to a specific one.
PHP Keywords List
1. and
This is a logical operator used for combining two conditions. It returns true if both conditions are true, and false if otherwise.
Example:
<?php
$x = 5;
$y = 10;
if ($x > 0 and $y > 0) {
echo "Both conditions are true.";
} else {
echo "At least one condition is false.";
}
?>
Output:
Both conditions are true.
2. array
This keyword creates an array, which is a collection of values stored under a single variable name.
Example:
<?php
$fruits = array("apple", "banana", "cherry");
echo "Fruits: " . implode(", ", $fruits);
?>
Output:
Fruits: apple, banana, cherry
3. break
The break keyword exits a loop prematurely.
Example:
<?php
for ($i = 1; $i <= 5; $i++) {
if ($i == 3) {
break;
}
echo $i . " ";
}
?>
Output:
1 2
4. print
The print construct outputs a string or variable to the web page. Unlike echo, this keyword includes parentheses and is less commonly used in modern PHP.
Example:
<?php
$message = "Hello, World!";
print($message);
?>
Output:
Hello, World!
5. class
This is used to define a class in object-oriented programming.
Example:
<?php
class Car {
public $make;
public $model;
public function start() {
echo "Car is starting.";
}
}
$myCar = new Car();
$myCar->start();
?>
Output:
Car is starting.
6. const
This keyword defines a constant value that cannot be changed.
Example:
<?php
const PI = 3.14159;
echo "The value of PI is " . PI;
?>
Output:
The value of PI is 3.14159
7. continue
The continue keyword skips the current iteration in a loop and continues with the next iteration.
Example:
<?php
for ($i = 1; $i <= 5; $i++) {
if ($i == 3) {
continue;
}
echo $i . " ";
}
?>
Output:
1 2 4 5
8. declare
This is used to set execution directives and can be used to set strict types.
Example:
<?php
declare(strict_types=1);
function add(int $a, int $b) {
echo $a + $b;
}
add(5, 3);
?>
Output:
8
9. die
This keyword terminates the script and displays a message to the user.
Example:
<?php
$error_message = "An error occurred.";
die($error_message);
?>
Output:
An error occurred.
10. echo
This keyword outputs text or variables to the web page.
Example:
<?php
$message = "Hello, World!";
echo $message;
?>
Output:
Hello, World!
11. if
This is used for conditional execution of code. It executes a block of code if a specified condition is true
Example:
<?php
$x = 10;
if ($x > 5) {
echo "x is greater than 5.";
} else {
echo "x is not greater than 5.";
}
?>
Output:
x is greater than 5.
12. else
This keyword is used in an if statement to define the block of code that will be executed if the condition is false.
Example:
<?php
$x = 5;
if ($x > 10) {
echo "Condition is true.";
} else {
echo "Condition is false.";
}
?>
Output:
Condition is false.
13. elseif
This keyword adds multiple conditions to an if statement.
Example:
<?php
$x = 15;
if ($x < 10) {
echo "X is less than 10.";
} elseif ($x > 10) {
echo "X is greater than 10.";
} else {
echo "X is equal to 10.";
}
?>
Output:
X is greater than 10.
14. switch
The switch keyword is used for conditional control flow. It allows you to test a variable against multiple possible values and execute different code blocks based on the matching case.
Example:
<?php
$day = "Monday";
switch ($day) {
case "Monday":
echo "It's the start of the week.";
break;
case "Friday":
echo "It's almost the weekend.";
break;
default:
echo "It's a regular day."; }
?>
Output:
It's the start of the week.
15. case
This keyword is used within a switch statement to define different possible values for the evaluated expression.
Example:
<?php
$day = "Monday";
switch ($day) {
case "Monday":
echo "It's the start of the week.";
break;
case "Friday":
echo "It's almost the weekend.";
break;
default:
echo "It's a regular day."; }
?>
Output:
It's the start of the week.
16. empty
The empty keyword checks if a variable is empty or if a value exists.
Example:
<?php
$name = "";
if (empty($name)) {
echo "Name is empty.";
} else {
echo "Name is not empty.";
}
?>
Output:
Name is empty.
17. eval
This evaluates a string as PHP code.
Example:
<?php
$x = 5;
$y = 10;
$result = eval("echo \$x + \$y;");
?>
Output:
15
18. exit
This keyword terminates the script and displays a message to the user.
Example:
<?php
$error_message = "An error occurred.";
exit($error_message);
?>
Output:
An error occurred.
19. for
This keyword is used to create a loop that executes a block of code a specified number of times.
Example:
<?php
for ($i = 0; $i < 5; $i++) {
echo $i . " ";
}
?>
Output:
0 1 2 3 4
20. foreach
This keyword is used to loop through elements in an array or other iterable.
Example:
<?php
$fruits = ["apple", "banana", "cherry"];
foreach ($fruits as $fruit) {
echo $fruit . " ";
}
?>
Output:
apple banana cherry
21. finally
The finally keyword is used in exception handling to specify code that should be executed regardless of whether an exception is thrown or not.
Example:
<?php
try {
$numerator = 10;
$denominator = 0;
if ($denominator === 0) {
throw new Exception("Division by zero is not allowed.");
}
$result = $numerator / $denominator;
echo "Result: $result";
} catch (Exception $e) {
echo "Caught an exception: " . $e->getMessage();
} finally {
echo "\nFinally block executed.";
}
echo "\nAfter try-catch-finally block.";
?>
Output:
Caught an exception: Division by zero is not allowed. Finally block executed. After try-catch-finally block.
22. function
This defines a reusable block of code that can be called with a specific name.
Example:
<?php
function greet($name) {
echo "Hello, $name!";
}
greet("Alice");
?>
Output:
Hello, Alice!
23. include
This keyword is used to include and evaluate a specified file.
Example:
<?php
include("header.php");
?>
Output:
The specified file is evaluated.
24. include_once
Similar to include, this keyword ensures that the file is only included once.
Example:
<?php
include_once("config.php");
?>
Output:
The specified file is only included once.
25. instanceof
Similar to include, this keyword ensures that the file is only included once.
Example:
<?php
class Car {
public $make;
public $model;
public function __construct($make, $model) {
$this->make = $make;
$this->model = $model;
}
}
$car = new Car("Toyota", "Camry");
if ($car instanceof Car) {
echo "It's a Car object. Make: {$car->make}, Model: {$car->model}";
} else {
echo "It's not a Car object.";
}
?>
Output:
It's a Car object. Make: Toyota, Model: Camry
26. namespace
This keyword defines a namespace for a set of codes. Namespaces help developers avoid naming conflicts.
Example:
<?php
namespace MyNamespace;
class MyClass {
public $name;
public function __construct($name) {
$this->name = $name;
}
public function greet() {
return "Hello, {$this->name}!";
}
}
$myObject = new MyClass("Alice");
$greeting = $myObject->greet();
echo $greeting;
?>
Output:
Hello, Alice!
27. new
The new keyword creates a new instance of a class, which allows you to create objects based on that class's blueprint.
Example:
<?php
class Car {
public $make;
public function __construct($make) {
$this->make = $make;
}
}
$myCar = new Car("Toyota");
echo "My car is a {$myCar->make}.";
?>
Output:
My car is a Toyota.
28. or
This is a logical operator used for combining two conditions. It returns true if at least one of the conditions is true.
Example:
<?php
$x = 5;
$y = 10;
if ($x > 0 or $y > 0) {
echo "At least one condition is true.";
} else {
echo "Both conditions are false.";
}
?>
Output:
At least one condition is true.
29. xor
This is also a logical operator used for combining two conditions. It returns true if exactly one of the conditions is true.
Example:
<?php
$a = true;
$b = false;
if ($a xor $b) {
echo "Exactly one condition is true.";
} else {
echo "Either both conditions are true or both are false.";
}
?>
Output:
Exactly one condition is true.
30. return
This is used within a function to specify a value to be returned when the function is called.
Example:
<?php
function add($a, $b) {
return $a + $b;
}
$result = add(5, 3);
echo "The result is: $result";
?>
Output:
The result is: 8
31. throw
This manually throws an exception in PHP. Exceptions are used for error handling.
Example:
<?php
function divide($a, $b) {
if ($b === 0) {
throw new Exception("Division by zero is not allowed.");
}
return $a / $b;
}
try {
$result = divide(10, 0);
echo "Result: $result";
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
?>
Output:
ERROR!
Error: Division by zero is not allowed.
32. try
The try keyword encloses a block of code that may throw exceptions. It is followed by one or more catch blocks for exception handling.
Example:
<?php
function divide($a, $b) {
if ($b === 0) {
throw new Exception("Division by zero is not allowed.");
}
return $a / $b;
}
try {
$result = divide(10, 0);
echo "Result: $result";
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
?>
Output:
ERROR!
Error: Division by zero is not allowed.
33. catch
The catch keyword is used in conjunction with try to catch and handle exceptions that are thrown in the try block.
Example:
<?php
function divide($a, $b) {
if ($b === 0) {
throw new Exception("Division by zero is not allowed.");
}
return $a / $b;
}
try {
$result = divide(10, 0);
echo "Result: $result";
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
?>
Output:
ERROR!
Error: Division by zero is not allowed.
34. use
This is used for importing namespaces and classes, allowing you to use them without fully qualifying their names.
Example:
<?php
use MyNamespace\MyClass;
$myObject = new MyClass();
echo "Used MyClass from MyNamespace.";
?>
Output:
Used MyClass from MyNamespace
35. while
This creates a loop that repeatedly executes a block of code as long as a specified condition is true.
Example:
<?php
$i = 1;
while ($i <= 5) {
echo $i . " "; $i++;
}
?>
Output:
1 2 3 4 5
36. unset
The unset keyword removes variables. It can also be used to unset elements in an array.
Example:
<?php
$x = 10;
unset($x);
echo isset($x) ? "Variable is set." : "Variable is not set.";
?>
Output:
Variable is not set.
37. abstract
This keyword declares an abstract class or abstract method. Abstract classes cannot be instantiated, and abstract methods must be implemented by child classes.
Example:
<?php
abstract class Shape {
abstract public function getArea();
}
class Circle extends Shape {
public function getArea() {
return 3.14;
}
}
$circle = new Circle();
$area = $circle->getArea();
echo "The area of the circle is: " . $area;
?>
Output:
The area of the circle is: 3.14
38. callable
This is used to specify that a variable can hold a callable function, method, or closure.
Example:
<?php
function process(callable $callback) {
$result = $callback();
echo "Result: $result";
}
$myFunction = function () {
return "Hello, World!";
};
process($myFunction);
?>
Output:
Result: Hello, World!
39. clone
The clone keyword creates a copy of an object. It's typically used with objects created from classes.
Example:
<?php
class MyClass {
public $name;
}
$original = new MyClass();
$original->name = "Original Object";
$copy = clone $original;
echo $copy->name;
?>
Output:
Original Object
40. final
This keyword indicates that a class or method can’t be extended or overridden by child classes.
Example:
<?php
final class FinalClass {
// Class code
}
class ChildClass /* Error: Cannot extend final class */ {
// Class code
}
?>
Output:
This creates a standalone class that can‘t be overridden by a child class.
41. implements
This keyword is used in class declarations to specify that the class implements one or more interfaces.
Example:
<?php
interface Printable {
public function printInfo();
} class Book implements Printable {
public function printInfo() {
echo "This is a book.";
}
}
$book = new Book();
$book->printInfo();
?>
Output:
This is a book.
42. interface
This keyword declares an interface, which defines a contract for methods that implementing classes must follow.
Example:
<?php
interface Printable {
public function printInfo();
} class Document implements Printable {
public function printInfo() {
echo "This is a document.";
}
}
$document = new Document();
$document->printInfo();
?>
Output:
This is a document.
43. private
This keyword is an access modifier used in class properties and methods. It restricts access to the property or method to only within the class that defines it.
Example:
<?php
class MyClass {
private $myPrivateProperty = "This is private.";
private function myPrivateMethod() {
echo "This is a private method.";
}
public function accessPrivate() {
return $this->myPrivateProperty;
}
}
$object = new MyClass();
echo "Private Property: " . $object->accessPrivate() . "\n";
?>
Output:
Private Property: This is private.
44. protected
This is also an access modifier used in class properties and methods. It restricts access to the property or method to the class that defines it and its subclasses.
Example:
<?php
class ParentClass {
protected $myProtectedProperty = "This is protected.";
}
class ChildClass extends ParentClass {
public function displayProperty() {
echo $this->myProtectedProperty;
}
}
$child = new ChildClass();
$child->displayProperty();
?>
Output:
This is protected.
45. public
This is another access modifier used in class properties and methods. It allows unrestricted access from anywhere.
Example:
<?php
class MyClass {
public $myPublicProperty = "This is public.";
public function myPublicMethod() {
echo "This is a public method.";
}
}
$object = new MyClass();
echo $object->myPublicProperty; // Accessing the public property.
$object->myPublicMethod();
?>
Output:
This is public.This is a public method.
46. static
This declares properties and methods that belong to the class rather than an instance of the class. They can be accessed without creating an instance.
Example:
<?php
class MathUtility {
public static function add($a, $b) {
return $a + $b;
}
}
$result = MathUtility::add(3, 5);
echo "The result of the addition is: " . $result;
?>
Output:
The result of the addition is: 8
47. yield
This is used in the context of generators. It allows a function to yield values one at a time, instead of returning an entire array of values.
Example:
<?php
function generateNumbers() {
yield 1;
yield 2;
yield 3;
}
foreach (generateNumbers() as $number) {
echo $number . " ";
}
?>
Output:
1 2 3
48. global
The global keyword is used to access a global variable from within a function or method.
Example:
<?php
$globalVar = 42;
function accessGlobalVar() {
global $globalVar;
echo "The global variable is: " . $globalVar;
}
accessGlobalVar();
?>
Output:
The global variable is: 42
49. list
This assigns values from an array to a list of variables. It's often used to quickly extract values from an array.
Example:
<?php
$person = ["John", "Doe", 30];
list($firstName, $lastName, $age) = $person;
echo "First Name: $firstName, Last Name: $lastName, Age: $age";
?>
Output:
First Name: John, Last Name: Doe, Age: 30
50. require
This keyword includes a file in PHP. If the file is not found, it will result in a fatal error and stop script execution.
Example:
<?php
require "config.php";
?>
Output:
Includes specified file, or produces error if file is not found.
51. require_once
Similar to require, this checks if the file has been included previously to prevent duplicate inclusions.
Example:
<?php
require_once "config.php";
?>
Output:
The global variable is: 42
52. insteadof
This keyword is used in trait aliasing. It resolves conflicts when multiple traits use the same method name.
Example:
<?php
trait Trait1 {
public function sayHello() {
echo "Hello from Trait1";
}
}
trait Trait2 {
public function sayHello() {
echo "Hello from Trait2";
}
}
class MyClass {
use Trait1, Trait2 {
Trait1::sayHello insteadof Trait2;
}
}
$object = new MyClass();
$object->sayHello();
?>
Output:
Hello from Trait1
53. trait
This keyword defines a reusable set of methods that can be used in multiple classes. It's similar to a class but cannot be instantiated on its own.
Example:
<?php
trait Logging {
public function log($message) {
echo "Log: $message";
}
}
class MyClass {
use Logging;
}
$object = new MyClass();
$object->log("Logging a message.");
?>
Output:
Log: Logging a message.
54. goto
This is a jump statement in PHP, allowing you to jump to another part of the code based on a label.
Example:
<?php
for ($i = 0; $i < 10; $i++) {
if ($i === 5) {
goto myLabel;
}
}
myLabel: echo "Jumped to the label.";
?>
Output:
Jumped to the label.
55. endfor
The endfor keyword terminates a for loop.
Example:
<?php
for ($i = 1; $i <= 5; $i++) {
echo "Iteration $i\n";
} // The 'endfor' keyword can be used to mark the end of the 'for' loop. endfor;
?>
Output:
Iteration 1
Iteration 2
Iteration 3
Iteration 4
Iteration 5
56. endforeach
This keyword terminates a foreach loop in PHP. It signifies the conclusion of the loop's execution.
Example:
<?php
$colors = array("red", "green", "blue");
foreach ($colors as $color):
echo "Color: $color\n";
endforeach;
?>
Output:
Color: red
Color: green
Color: blue
57. endif
This keyword terminates an if statement or conditional block.
Example:
<?php
$condition = true;
if ($condition):
echo "Condition is true.\n";
endif;
echo "This is outside the 'if' block.\n";
?>
Output:
Condition is true.
This is outside the 'if' block.
58. __halt_compiler
This keyword is used in combination with the __halt_compiler() function to mark the end of a script and instruct the PHP parser not to process any code beyond this point.
Example:
<?php
// Some PHP code here
// Data or binary content can be placed here
__halt_compiler();
This content will not be processed by the PHP parser.
?>
Output:
The code featured after halt_compiler will not be parsed.
Keeping Track of PHP Keywords
Keywords are the building blocks of any programming language, so keeping this list handy will make it easier to reference them as needed over time. The more experience you develop with PHP, the faster you’ll be at recalling each of these keywords and using them to your advantage. Bookmark this page to keep it readily accessible, and continue strengthening your PHP skills throughout your programming journey.