Did you know you can send emails using PHP?
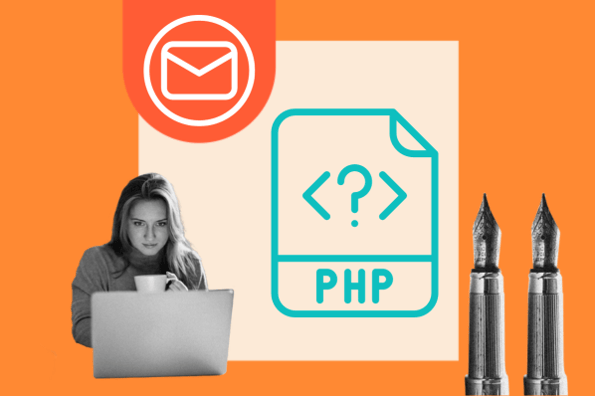
It's great for setting up automatic alerts on your website and you can use it to email multiple recipients at once. Like, for example, when a new product is launched or a sale goes live. You can use PHP to quickly send messages to email subscribers, alerting them to check out your store.
In this post, we'll explain how to send emails in PHP using three simple steps. We'll also provide a complimentary example so you can follow along and try it for yourself.
How to Send Emails With the Mail Function in PHP
Before getting started, you’ll want to make sure that your PHP environment is configured for sending emails. Check your PHP.ini file to make sure the SMTP server settings are defined. If not, consult your hosting provider or system administrator before moving forward.
1. Define the recipient, subject, message, and header.
Before calling the mail function, start with defining the core components of the email: the recipient's email address, subject line, email message, and any additional headers you want to include.
In our example, we’ll email my theoretical coworker, Taylor Slow -- unrelated to Taylor Swift.
<?php
$to = 'tslow@hubspot.com';
$subject = 'Hello from PHP';
$message = 'This is a test email sent from PHP.';
$headers = 'From: cfontanella@hubspot.com';
?>
Note: You’ll need a From header to comply with email standards and to prevent the email from being flagged as spam.
2. Call the mail() function.
Now, we can call the mail function using mail(). The mail function takes in the recipient's email, subject, message, and headers as arguments.
<?php
$to = 'tslow@hubspot.com';
$subject = 'Hello from PHP';
$message = 'This is a test email sent from PHP.';
$headers = 'From: cfontanella@hubspot.com';
$isSent = mail($to, $subject, $message, $headers);
if ($isSent) {
echo'Email sent successfully.';
} else {
echo 'Failed to send the email.';
}
?>
The mail function returns a boolean value to indicate whether the email was sent successfully.
3. Customize your email’s content and format.
You can also enhance the content of your email by including HTML tags or using PHP to dynamically generate the message.
For example, you can send HTML emails using the following syntax:
<?php
$to = 'tslow@hubspot.com';
$subject = 'Hello from PHP';
$message = '<html><body>';
$message .= '<h1>Hello!</h1>';
$message .= '<p>This is a test email sent from PHP.</p>';
$message .= '</body></html>';
$headers = 'From: cfontanella@hubspot.com';
$headers .= "\r\n";
$headers .= "Content-Type: text/html; charset=UTF-8";
$isSent = mail($to, $subject, $message, $headers);
if ($isSent) {
echo'Email sent successfully.';
} else {
echo 'Failed to send the email.';
}
?>
In this example, we specified the Content-Type header as text/html, which changes the email format to HTML.
Sending Emails in PHP
PHP is a fast and efficient programming language -- and this is just one of the hundreds of functions that you can use within it. The PHP mail function can make any website more dynamic and offers an additional way to communicate with your customer base. Remember to keep your messages simple and leverage this technique to stay more connected with your online audience.