Strings are the building blocks of data in PHP and they’re essential in nearly every aspect of web development. From data parsing to text manipulation, string functions alleviate complex problems and help you build dynamic websites and applications.
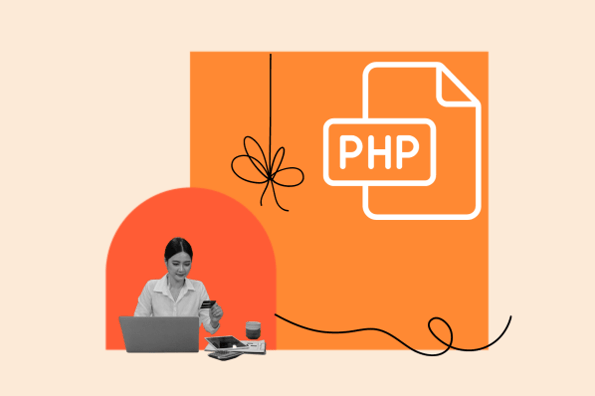
This blog post explores PHP string functions, including their diverse applications and how they can save you time and effort in your coding endeavors.
Read on for a comprehensive list of PHP string functions, or check out this table of jump links to navigate to one of your choosing.
PHP String Functions List
1. String Length: strlen()
This function returns the length of a string.
Example:
<?php
$text = "Hello, World!";
$length = strlen($text);
echo "The length of the string is $length characters.";
?>
Output:
The length of the string is 13 characters.
2. String Position: strpos()
This function finds the position of a substring in a string.
Example:
<?php
$text = "This is a test string.";
$pos = strpos($text, "test");
echo "The word 'test' starts at position $pos.";
?>
Output:
The word 'test' starts at position 10.
3. String Postion: stripos()
Similar to strpos(), this function also finds the position of a substring in a string.
Example:
<?php
$text = "This is a test string.";
$pos = stripos($text, "Test");
echo "The word 'Test' starts at position $pos.";
?>
Output:
The word 'Test' starts at position 10.
4. Substring Function: substr()
This function extracts a substring from a string.
Example:
<?php
$text = "Hello, World!";
$substring = substr($text, 0, 5);
echo "The first 5 characters are: $substring";
?>
Output:
The first 5 characters are: Hello
5. String Replace: str_replace()
This function replaces all occurrences of a substring with another substring.
Example:
<?php
$text = "Hello, World!";
$newText = str_replace("World", "Universe", $text);
echo "Replaced: $newText";
?>
Output:
Replaced: Hello, Universe!
6. String Repeat: str_repeat()
This function repeats a string a specified number of times.
Example:
<?php
$text = "La ";
$repeatedText = str_repeat($text, 3);
echo "Repetition: $repeatedText";
?>
Output:
Repetition: La La La
7. First Character Uppercase: ucfirst()
This function converts the first character of a string to uppercase.
Example:
<?php
$text = "hello, world!";
$capitalized = ucfirst($text);
echo "Capitalized: $capitalized";
?>
Output:
Capitalized: Hello, world!
8. Capitalize All Words: ucwords()
This function converts the first character of each word in a string to uppercase.
Example:
<?php
$text = "hello, world!";
$capitalizedWords = ucwords($text);
echo "Capitalized Words: $capitalizedWords";
?>
Output:
Capitalized Words: Hello, World!
9. First Character Lowercase: lcfirst()
This function converts the first character of a string to lowercase.
Example:
<?php
$text = "Hello, World!";
$lowercased = lcfirst($text);
echo "Lowercased: $lowercased";
?>
Output:
Lowercased: hello, World!
10. Convert String to Uppercase: strtoupper()
This converts a string to all uppercase characters.
Example:
<?php
$text = "Hello, World!";
$uppercase = strtoupper($text);
echo "Uppercase: $uppercase";
?>
Output:
Uppercase: HELLO, WORLD!
11. Convert String to Lowercase: strtolower()
This function converts a string to lowercase.
Example:
<?php
$text = "Hello, World!";
$lowercase = strtolower($text);
echo "Lowercase: $lowercase";
?>
Output:
Lowercase: hello, world
12. Trim String: trim()
This removes whitespace or other characters from the beginning and end of a string.
Example:
<?php
$text = " Hello, World! ";
$trimmed = trim($text);
echo "Trimmed: $trimmed";
?>
Output:
Trimmed: Hello, World!
13. Left Trim: ltrim()
This function removes whitespace from the beginning of a string.
Example:
<?php
$text = " Hello, World! ";
$leftTrimmed = ltrim($text);
echo "Left Trimmed: $leftTrimmed";
?>
Output:
Left Trimmed: Hello, World!
14. Right Trim: rtrim()
This function removes whitespace from the end of a string.
Example:
<?php
$text = " Hello, World! ";
$rightTrimmed = rtrim($text);
echo "Right Trimmed: $rightTrimmed";
?>
Output:
Right Trimmed: Hello, World!
15. String Reverse: strrev()
This function reverses the order of a string.
Example:
<?php
$text = "Hello, World!";
$reversed = strrev($text);
echo "Reversed: $reversed";
?>
Output:
Reversed: !dlroW ,olleH
16. String Compare: strcmp()
This function compares two strings and returns 0 if they are equal, a positive number if the first string is greater, and a negative number if the second string is greater.
Example:
<?php
$string1 = "apple";
$string2 = "banana"; $result = strcmp($string1, $string2);
echo "Comparison Result: $result";
?>
Output:
Comparison Result: -1
17. Case-Insenstive Comparison: strcasecmp()
This function performs the same task as strcmp(), however, this function is not case-sensitive.
Example:
<?php
$string1 = "apple";
$string2 = "Apple";
$result = strcasecmp($string1, $string2);
echo "Case-Insensitive Comparison Result: $result";
?>
Output:
Case-Insensitive Comparison Result: 0
18. Natural Order Comparison: strnatcmp()
This function performs a natural order string comparison.
Example:
<?php
$string1 = "img2.png";
$string2 = "img10.png";
$result = strnatcmp($string1, $string2);
echo "Natural Comparison Result: $result";
?>
Output:
Natural Comparison Result: -1
19. Case-Insensitive Natural Comparison: strnatcasecmp()
This is the case-insensitive version of strnatcmp().
Example:
<?php
$string1 = "IMG2.png";
$string2 = "img10.png";
$result = strnatcasecmp($string1, $string2);
echo "Case-Insensitive Natural Comparison Result: $result";
?>
Output:
Case-Insensitive Natural Comparison Result: -1
20. Find String: strstr()
This function finds the first occurrence of a substring and returns the part of the string from that occurrence to the end.
Example:
<?php
$text = "This is a test string.";
$substring = strstr($text, "test");
echo "Found: $substring";
?>
Output:
Found: test string.
21. Find String (Case-Insensitive): stristr()
This is the case-insensitive version of strstr().
Example:
<?php
$text = "This is a test string.";
$substring = stristr($text, "Test");
echo "Found (Case-Insensitive): $substring";
?>
Output:
Found (Case-Insensitive): test string.
22. Find Last Occurrence: strrchr()
This function finds the last occurrence of a character and returns the part of the string from that occurrence to the end.
Example:
<?php
$text = "This is a test string.";
$substring = strrchr($text, "t");
echo "Last Occurrence: $substring";
?>
Output:
Last Occurrence: tring.
23. String Shuffle: str_shuffle()
This function randomly shuffles the characters in a string.
Example:
<?php
$text = "Hello, World!";
$shuffled = str_shuffle($text);
echo "Shuffled: $shuffled";
?>
Output:
Shuffled: ldHoeWl,r ol!
24. Wordwrap: wordwrap()
This function wraps a string to a specified number of characters per line, breaking lines at word boundaries.
Example:
<?php
$text = "This is a long sentence that should be wrapped.";
$wrapped = wordwrap($text, 15, "<br>");
echo "Wrapped:<br>$wrapped";
?>
Output:
Wrapped:<br>This is a long<br>sentence that<br>should be<br>wrapped.
25. Explode Function: explode()
The explode function splits a string into an array by a specified delimiter.
Example:
<?php
$text = "apple,banana,orange";
$fruits = explode(",", $text);
print_r($fruits);
?>
Output:
Array
(
[0] => apple
[1] => banana
[2] => orange
)
26. Implode String: implode()
This function joins the elements of an array into a string using a specified delimiter.
Example:
<?php
$fruits = ["apple", "banana", "orange"];
$text = implode(", ", $fruits);
echo "Fruits: $text";
?>
Output:
Fruits: apple, banana, orange
27. Join String: join()
The join function is an alias for the implode() function.
Example:
<?php
$fruits = ["apple", "banana", "orange"];
$text = join(", ", $fruits);
echo "Fruits: $text";
?>
Output:
Fruits: apple, banana, orange
28. String Split: str_split()
The string split function splits a string into an array of characters.
Example:
<?php
$text = "Hello";
$characters = str_split($text);
print_r($characters);
?>
Output:
Array
(
[0] => H
[1] => e
[2] => l
[3] => l
[4] => o
)
29. Chunk Split: chunk_split()
This function splits a string into smaller chunks and adds a delimiter.
Example:
<?php
$text = "1234567890";
$chunked = chunk_split($text, 2, "-");
echo "Chunked: $chunked";
?>
Output:
Chunked: 12-34-56-78-90-
30. Add Slashes: addslashes()
This function puts special characters in a string to prevent SQL injection and other security vulnerabilities.
Example:
<?php
$text = "I'm using PHP's functions.";
$escaped = addslashes($text);
echo "Escaped: $escaped";
?>
Output:
Escaped: I\'m using PHP\'s functions.
31. Remove Slashes: stripslashes()
This function removes slashes added by the addslashes() function.
Example:
<?php
$text = "I\'m using PHP\'s functions.";
$unescaped = stripslashes($text);
echo "Unescaped: $unescaped";
?>
Output:
Unescaped: I'm using PHP's functions.
32. Add C Slashes: addcslashes()
This function creates a quote string with slashes in a C-like style.
Example:
<?php
$text = "Hello, World!";
$quoted = addcslashes($text, 'W');
echo "Quoted: $quoted";
?>
Output:
Quoted: Hello, \World!
33. Special Characters to HTML: htmlspecialchars()
This function converts special characters to their HTML entities.
Example:
<?php
$text = "This is <b>bold</b>";
$escaped = htmlspecialchars($text);
echo "Escaped: $escaped";
?>
Output:
Escaped: This is <b>bold</b>
34. String to HTML: htmlentities()
This function converts all applicable characters to their HTML entities.
Example:
<?php
$text = "This is <b>bold</b>";
$escaped = htmlentities($text);
echo "Escaped: $escaped";
?>
Output:
Escaped: This is <b>bold</b>
35. Decode HTML: html_entity_decode()
This decodes HTML entities back to their corresponding characters.
Example:
<?php
$text = "This is <b>bold</b>";
$decoded = html_entity_decode($text);
echo "Decoded: $decoded";
?>
Output:
Decoded: This is <b>bold</b>
36. Remove Tags: strip_tags()
This function removes HTML and PHP tags from a string.
Example:
<?php
$text = "This is <b>bold</b> and <i>italic</i>.";
$stripped = strip_tags($text);
echo "Stripped: $stripped";
?>
Output:
Stripped: This is bold and italic.
37. Decode Special Characters: htmlspecialchars_decode()
This function decodes HTML entities back to their corresponding characters.
Example:
<?php
$text = "This is <b>bold</b>";
$decoded = htmlspecialchars_decode($text);
echo "Decoded: $decoded";
?>
Output:
Decoded: This is <b>bold</b>
38. Insert Line Break: nl2br()
This function inserts HTML line breaks before all new lines in a string.
Example:
<?php
$text = "This is a\nmultiline\nstring.";
$brText = nl2br($text);
echo "With Line Breaks:<br>$brText";
?>
Output:
With Line Breaks:<br>This is a<br />
multiline<br />
string.
39. Replace Substring: substr_replace()
This function replaces a portion of a string with another string.
Example:
<?php
$text = "Hello, World!";
$replacement = "Universe";
$newText = substr_replace($text, $replacement, 7, 5);
echo "Replaced: $newText";
?>
Output:
Replaced: Hello, Universe!
40. Count Substring: substr_count()
This function counts the number of non-overlapping occurrences of a substring in a string.
Example:
<?php
$text = "The quick brown fox jumps over the lazy dog.";
$count = substr_count($text, "the");
echo "Substring Count: $count";
?>
Output:
Substring Count: 1
41. String Portion: strpbrk()
This function searches a string for any of a set of characters and returns the portion of the string from the first occurrence of any of those characters.
Example:
<?php
$text = "ABC-123";
$portion = strpbrk($text, "0123456789");
echo "Portion: $portion";
?>
Output:
Portion: 123
42. Soundex: soundex()
This function calculates the soundex key of a string.
Example:
<?php
$text1 = "Smith";
$text2 = "Smyth";
$soundex1 = soundex($text1);
$soundex2 = soundex($text2);
echo "Soundex of '$text1': $soundex1<br>";
echo "Soundex of '$text2': $soundex2";
?>
Output:
Soundex of 'Smith': S530<br>Soundex of 'Smyth': S530
43. Metaphone: metaphone()
This function calculates the metaphone key of a string.
Example:
<?php
$text1 = "Smith";
$text2 = "Smyth";
$metaphone1 = metaphone($text1);
$metaphone2 = metaphone($text2);
echo "Metaphone of '$text1': $metaphone1<br>";
echo "Metaphone of '$text2': $metaphone2";
?>
Output:
Metaphone of 'Smith': SM0<br>Metaphone of 'Smyth': SM0
44. Locale-Based Comparison: strcoll()
This performs a locale-based string comparison.
Example:
<?php
$text1 = "café";
$text2 = "cafe";
$result = strcoll($text1, $text2);
echo "Comparison Result: $result";
?>
Output:
Comparison Result: 94
45. Segment Length: strcspn()
This returns the length of the initial segment of a string that contains no characters from a given mask.
Example:
<?php
$text = "abcde12345";
$mask = "1234567890";
$length = strcspn($text, $mask);
echo "Length: $length";
?>
Output:
Length: 5
46. Pad String: str_pad()
This function pads a string to a specified length with another string.
Example:
<?php
$text = "Hello";
$padded = str_pad($text, 10, ".");
echo "Padded: $padded";
?>
Output:
Padded: Hello.....
47. Translate String: strtr()
This function translates certain characters in a string.
Example:
<?php
$text = "hello, world!";
$trans = strtr($text, "lo", "12");
echo "Translated: $trans";
?>
Output:
Translated: he112, w2r1d!
48. String Similarity: similar_text()
This function calculates the similarity between two strings.
Example:
<?php
$text1 = "hello, world!";
$text2 = "world, hello!"; similar_text($text1, $text2, $percent);
echo "Similarity: $percent%";
?>
Output:
Similarity: 46.153846153846%
49. Levenshtein Distance: levenshtein()
This function calculates the Levenshtein distance between two strings.
Example:
<?php
$text1 = "kitten";
$text2 = "sitting";
$distance = levenshtein($text1, $text2);
echo "Levenshtein Distance: $distance";
?>
Output:
Levenshtein Distance: 3
50. Cyclic Redundancy Check: crc32()
This calculates the 32-bit CRC (Cyclic Redundancy Check) of a string.
Example:
<?php
$text = "Hello, World!";
$crc = crc32($text);
echo "CRC32: $crc";
?>
Output:
CRC32: 3964322768
51. MD5 Hash: md5()
This function calculates the MD5 hash of a string.
Example:
<?php
$text = "Hello, World!";
$hash = md5($text);
echo "MD5 Hash: $hash";
?>
Output:
MD5 Hash: 65a8e27d8879283831b664bd8b7f0ad4
52. SHA-1 Hash: sha1()
This function calculates the SHA-1 hash of a string.
Example:
<?php
$text = "Hello, World!";
$hash = sha1($text);
echo "SHA-1 Hash: $hash";
?>
Output:
SHA-1 Hash: 0a0a9f2a6772942557ab5355d76af442f8f65e01
53. Hash Function: hash()
This function calculates the hash value using a specified hash function.
Example:
<?php
$text = "Hello, World!";
$hash = hash('sha256', $text);
echo "SHA-256 Hash: $hash";
?>
Output:
SHA-256 Hash: dffd6021bb2bd5b0af676290809ec3a53191dd81c7f70a4b28688a362182986f
54. Crypt: crypt()
The crypt() function creates a one-way string hashing for password storage and security.
Example:
<?php
$password = "mysecretpassword";
$hashedPassword = crypt($password, '$2a$07$usesomesillystringforsalt$');
echo "Hashed Password: $hashedPassword";
?>
Output:
Hashed Password: $2a$07$usesomesillystringforeZ2/FbMazlxeaWs2I.edlhZE1aZ4Qcci
55. String Word Count: str_word_count()
This function counts the number of words in a string.
Example:
<?php
$text = "This is a simple sentence.";
$wordCount = str_word_count($text);
echo "Word Count: $wordCount";
?>
Output:
Word Count: 5
56. Money Formatting: money_format()
This function formats a number as a currency string.
Example:
<?php
$amount = 12345.67;
$formatted = money_format('%i', $amount);
echo "Formatted: $formatted";
?>
Output:
USD 12,345.67
57. String Replace (Case-Insensitive): str_ireplace()
This is the case-insensitive version of str_replace(). It replaces all occurrences of a substring with another substring in a string.
Example:
<?php
$text = "Hello, world! Hello, universe!";
$newText = str_ireplace("hello", "hi", $text);
echo "Replaced: $newText";
?>
Output:
Replaced: hi, world! hi, universe!
58. Character Information: count_chars()
This returns information about characters used in a string.
<?php
$text = "apple";
$info = count_chars($text, 1);
print_r($info);
?>
Output:
Array
(
[97] => 1
[101] => 1
[108] => 1
[112] => 2
)
59. Parse a CSV: str_getcsv()
This function parses a CSV string into an array.
Example:
<?php
$csv = "apple,banana,orange";
$array = str_getcsv($csv);
print_r($array);
?>
Output:
Array
(
[0] => apple
[1] => banana
[2] => orange
)
60. Quote Meta Characters: quotemeta()
This function quotes meta characters in a string.
Example:
<?php
$text = "This is a (test).";
$quoted = quotemeta($text);
echo "Quoted: $quoted";
?>
Output:
Quoted: This is a \(test\)\.
61. Encode URL: urlencode()
This function URL-encodes a string.
Example:
<?php
$text = "Hello, World!";
$encoded = urlencode($text);
echo "Encoded: $encoded";
?>
Output:
Encoded: Hello%2C+World%21
62. Decode Encoded-URL: urldecode()
This function decodes a URL-encoded string.
Example:
<?php
$encoded = "Hello%2C+World%21";
$decoded = urldecode($encoded);
echo "Decoded: $decoded";
?>
Output:
Decoded: Hello, World!
63. Raw URL-Encode a String: rawurlencode()
This function raw URL-encodes a string.
Example:
<?php
$encoded = "Hello%2C%20World%21";
$decoded = rawurldecode($encoded);
echo "Raw Decoded: $decoded";
?>
Output:
Raw Decoded: Hello, World!
64. Decode Raw Url-Encoded String: rawurldecode()
This function decodes a raw URL-encoded string.
Example:
<?php
$encoded = "Hello%2C%20World%21";
$decoded = rawurldecode($encoded);
echo "Raw Decoded: $decoded";
?>
Output:
Raw Decoded: Hello, World!
65. Parse URL: parse_url()
This function parses a URL and returns its components.
Example:
<?php
$url = "https://www.example.com/path/file.php?param=value#fragment";
$components = parse_url($url);
print_r($components);
?>
Output:
Array
(
[scheme] => https
[host] => www.example.com
[path] => /path/file.php
[query] => param=value
[fragment] => fragment
)
66. Parse a Query String: parse_str()
This function parses a query string into variables.
Example:
<?php
$query = "param1=value1¶m2=value2";
parse_str($query, $vars);
print_r($vars);
?>
Output:
Array
(
[param1] => value1
[param2] => value2
)
67. Return a Character: chr()
This function returns a character specified by an ASCII value.
Example:
<?php
$ascii = 65; // ASCII code for 'A'
$character = chr($ascii);
echo "Character: $character";
?>
Output:
Character: A
68. Generate Query String: http_build_query()
This function generates a URL-encoded query string from an array or object.
Example:
<?php
$data = array('name' => 'John', 'age' => 30);
$query = http_build_query($data);
echo "Query: $query";
?>
Output:
Query: name=John&age=30
69. Convert Binary to Hexadecimal: bin2hex()
This function converts binary data into hexadecimal representation.
Example:
<?php
$binaryData = "\x48\x65\x6c\x6c\x6f"; // Hello in binary
$hexData = bin2hex($binaryData);
echo "Hexadecimal: $hexData";
?>
Output:
Hexadecimal: 48656c6c6f
70. Decode Hexadecimal Binary String: hex2bin()
This function decodes a hexadecimally encoded binary string.
Example:
<?php
$hexData = "48656c6c6f"; // Hexadecimal representation of "Hello"
$binaryData = hex2bin($hexData);
echo "Decoded: $binaryData";
?>
Output:
Decoded: Hello
71. Return ASCII Value: ord()
This function returns the ASCII value of the first character of a string.
Example:
<?php
$text = "A";
$asciiValue = ord($text);
echo "ASCII Value: $asciiValue";
?>
Output:
ASCII Value: 65
72. String Token: strtok()
This function tokenizes a string using a specified delimiter.
Example:
<?php
$text = "apple,banana,orange";
$token = strtok($text, ",");
while ($token !== false) {
echo "Token: $token<br>";
$token = strtok(",");
}
?>
Output:
Token: apple<br>Token: banana<br>Token: orange<br>
73. String Value: strval()
This function returns the string value of a variable.
Example:
<?php
$number = 42;
$stringValue = strval($number);
echo "String Value: $stringValue";
?>
Output:
String Value: 42
74. String Comparison (Case-Insensitive): strncasecmp()
This function performs a case-insensitive string comparison of the first n characters.
Example:
<?php
$text1 = "apple";
$text2 = "Apples";
$result = strncasecmp($text1, $text2, 4);
echo "Comparison Result: $result";
?>
Output:
Comparison Result: 0
75. Find First Occurrence in String: strchr()
This function finds the first occurrence of a character in a string and returns the part of the string from that occurrence to the end.
Example:
<?php
$text = "This is a test string.";
$substring = strchr($text, "test");
echo "Found: $substring";
?>
Output:
Found: test string.
76. Find Last Occurrence of Substring: strrpos()
This function finds the last occurrence of a substring in a string.
Example:
<?php
$text = "This is a test string. This is another test.";
$position = strrpos($text, "test");
echo "Last Occurrence at position: $position";
?>
Output:
Last Occurrence at position: 39
77. Find Last Occurrence of Substring (Case-Insensitive): strripos()
This is the case-insensitive version of strrpos().
Example:
<?php
$text = "This is a test string. This is another test.";
$position = strripos($text, "TEST");
echo "Last Occurrence (Case-Insensitive) at position: $position";
?>
Output:
Last Occurrence (Case-Insensitive) at position: 39
78. Compare Substrings: substr_compare()
This function compares two substrings in a case-sensitive manner.
Example:
<?php
$text1 = "apple";
$text2 = "apples";
$result = substr_compare($text1, $text2, 0, 5);
echo "Comparison Result: $result";
?>
Output:
Comparison Result: 0
79. Echo Function: echo()
The echo() function is used to output one or more strings or variables to the web page.
Example:
<?php
$name = "John";
$age = 30;
echo "Hello, my name is $name and I am $age years old.";
?>
Output:
Hello, my name is John and I am 30 years old.
80. Print Function: print()
This function is used to output a single string or variable to the web page.
Example:
<?php
$name = "Alice";
$age = 25;
print("Hello, my name is $name and I am $age years old.");
?>
Output:
Hello, my name is Alice and I am 25 years old.
Using PHP String Functions
These functions are the backbone of PHP development, enabling you to build powerful websites and applications with precision and efficiency. Keep this list of functions handy so you can quickly reference them as needed and continue to improve your PHP coding skills.