React is a JavaScript library for building user interfaces (UIs) and single-page applications. Its popularity in the development community is mainly due to its flexibility in building UIs through reusable components. This has led many developers to adopt the library as the front-end framework for their technology stacks.
However, writing CSS styles for those components is notoriously difficult. CSS is a very technical language and often involves many tedious processes that take time away from development. For example, defining CSS styles scoped to global components can lead to errors, necessitating that you rewrite the same CSS repeatedly at different points in the stylesheet.
Fortunately, tools and libraries like Bootstrap CSS exist to make CSS far easier to use. Bootstrap is a free, open-source CSS framework for mobile-first, front-end web development. It is a full-knit UI library offering pre-defined CSS styles and other UI elements to help developers customize their web pages quickly without needing to start from the ground up.
You can easily combine Bootstrap with many other front-end technologies, including React. While React helps you build your functionality, Bootstrap simplifies defining and maintaining the form.
This tutorial demonstrates how to add Bootstrap to a React project and make the most of its CSS-style capabilities.
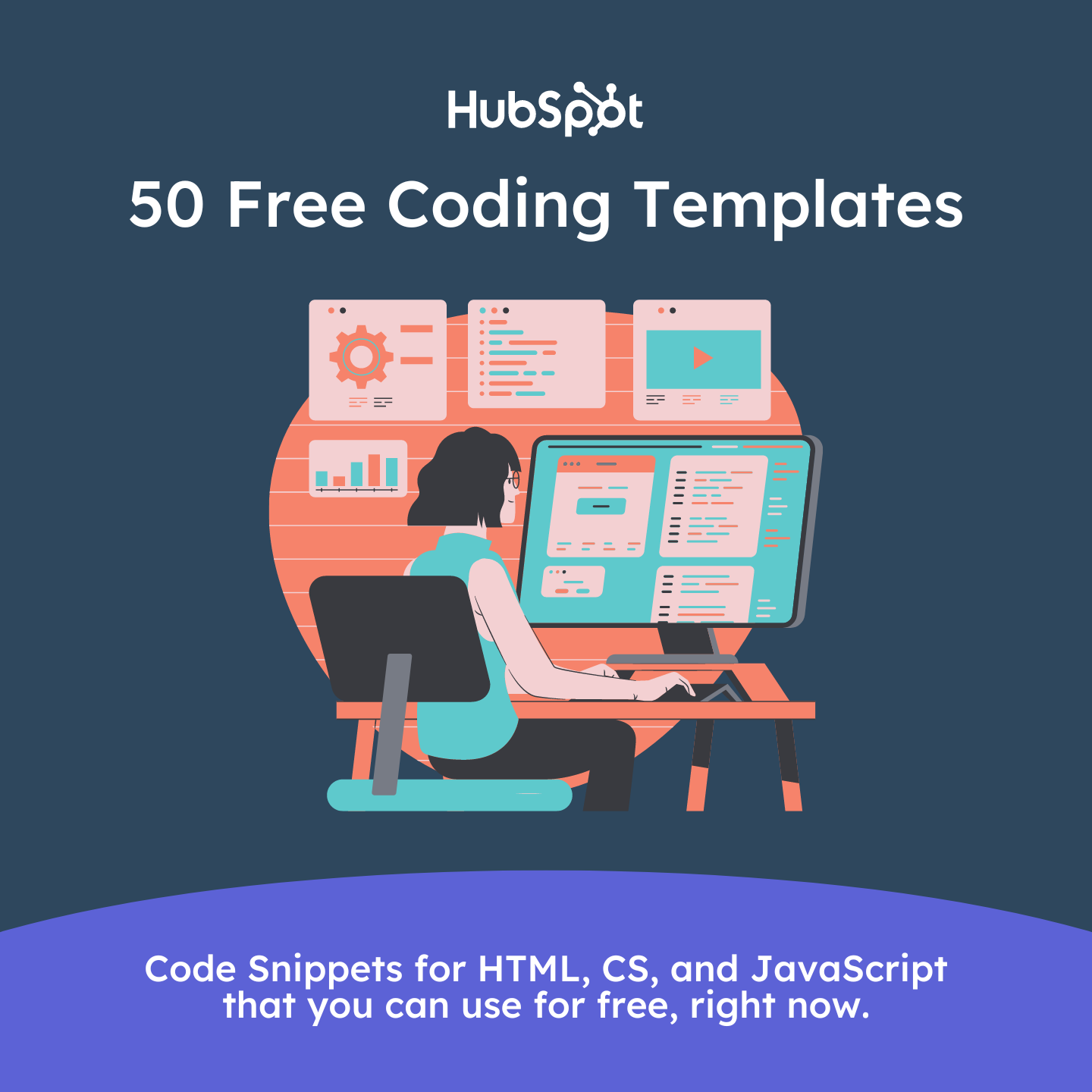
50 Free Coding Templates
Free code snippet templates for HTML, CSS, and JavaScript -- Plus access to GitHub.
- Navigation Menus & Breadcrumbs Templates
- Button Transition Templates
- CSS Effects Templates
- And more!
Download Free
All fields are required.
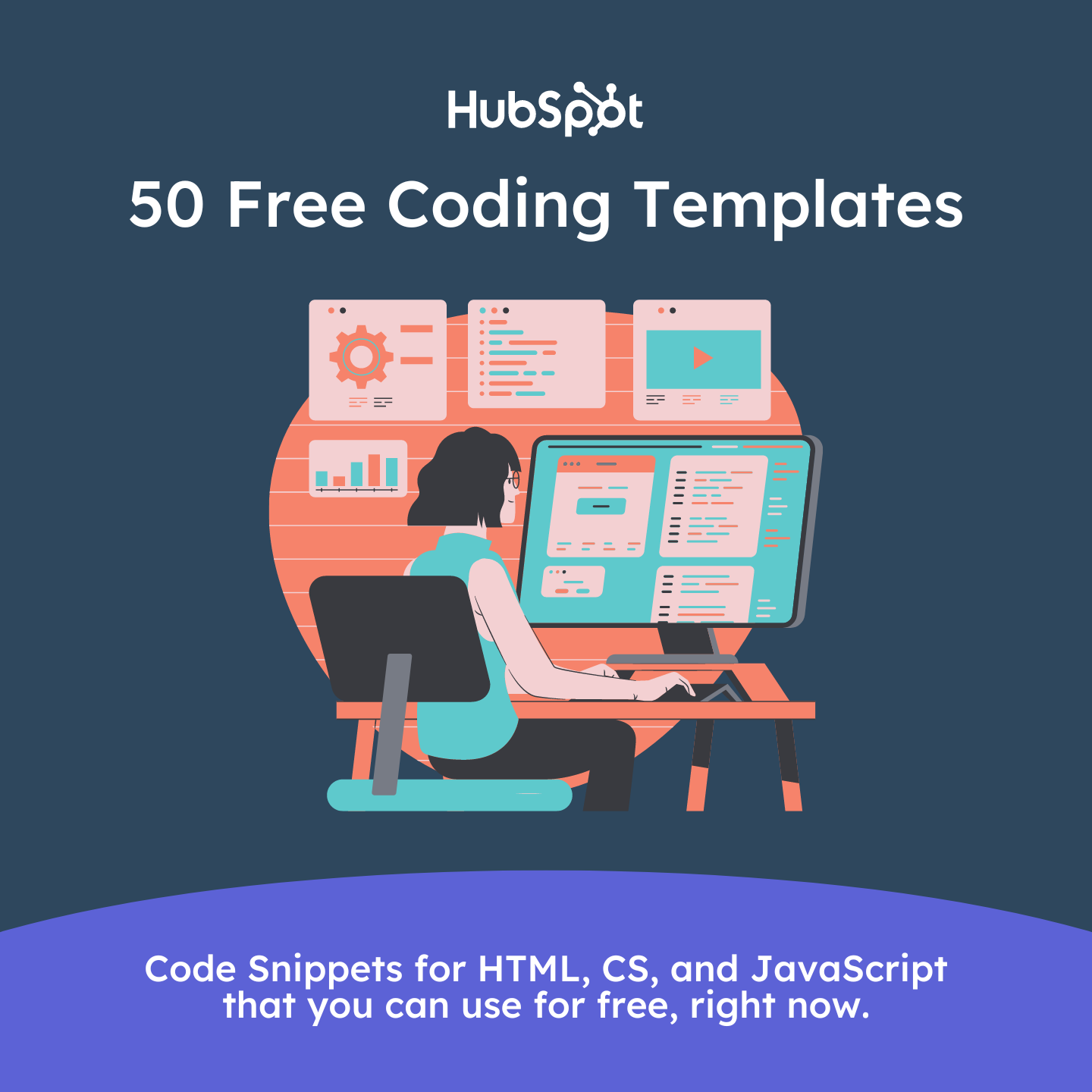
Using Bootstrap with React
For this project, you’ll use React to build a simple counter component. Then, you’ll add Bootstrap CSS to the project to customize the front-end UI.
To follow this tutorial, you’ll need:
- Node.js installed on your computer
- A basic understanding of how React works
1. Set ip a React application.
To create a React application, open the Node.js terminal and run the following command:
npx create-react-app <name-of-your-app-here>
Replace <name-of-your-app-here> with what you would like to name your React application.
Once the command has run successfully, you should see the following message in your terminal:
Now, navigate to the directory of your React application and run the app locally.
Return to the Node.js terminal and run the following commands:
cd <name-of-app>
npm start
This will launch a development server where you can preview your site. You can now view it at http://localhost:3000 in a web browser. The result should look like this:
2. Add Bootstrap to the React project.
With your React application up and running, you’re ready to add Bootstrap as your CSS framework.
There are different ways to do this. However, the most convenient option is to include it as a dependency in your React application by installing the Bootstrap npm package.
First, navigate to the root directory of your React application in the Node.js terminal and run the following command:
npm install bootstrap
Or, if you are using yarn, use this instead:
yarn add bootstrap
These commands will install the most recent Bootstrap version on your React app.
Next, add the following line to the top of the ./src/index.js file.
import 'bootstrap/dist/css/bootstrap.css';
Note: It’s important to import Bootstrap at the top of your app’s entry file before other CSS files. This ensures that the styles from your CSS files will take precedence over Bootstrap’s styles, making it easier to alter the look of your application from Bootstrap’s default theme if necessary.
Now, you’re ready to import Bootstrap’s classes and styles into your React application and style your components.
3. Create the counter component.
In this section, you’ll turn your empty React project into a functioning counter component with a very basic UI. Your finished application will display a numerical value. Below, you’ll create two buttons that will increase or decrease that value by one by using React’s state management hook.
To create your basic counter component, navigate to the ./src folder of your React application. In the folder, create a new JavaScript file called Counter.js and populate it with the following code:
The above code uses React’s useState hook to create the state variable count and set its initial value to 0.
Then, it defines the add() and subtract() functions to modify the value of count and pass them as event handlers to the Add and Remove buttons.
Next, view your Counter component by clearing the content of the ./App.js file and populating it with the following code:
Run the app and preview its output in a browser. When rendered, it should look like the following image:
Clicking the Add and Remove buttons will update the value of the state variable, count.
4. Style with Bootstrap classes.
Your counter component is now functional, but it currently looks a bit bland. This is where you can use Bootstrap classes to add some form to this function.
Bootstrap offers several style classes to help you quickly style your website or web-based application. These include:
- Layout classes to control the layout and position of elements in the viewport
- Component classes to help you style standalone components like navbars, progress bars, and buttons
- Utility classes to write CSS styles for individual properties like margins, borders, and colors
For this project, you’ll use all three classes to change the size, font, and placement of the text, as well as add some style to the buttons.
To begin, open the ./Counter.js file and replace the HTML in its return statement with the following code:
Now, when you preview your React app in a browser, it should look like this:
You’ve been able to give the component a much better look and layout by using just a few Bootstrap classes.
Combining Bootstrap with React
In this tutorial, you made a basic React application look much better using just a few Bootstrap classes — without needing any CSS language knowledge.
To take your React application to the next level with styling, consider the following questions:
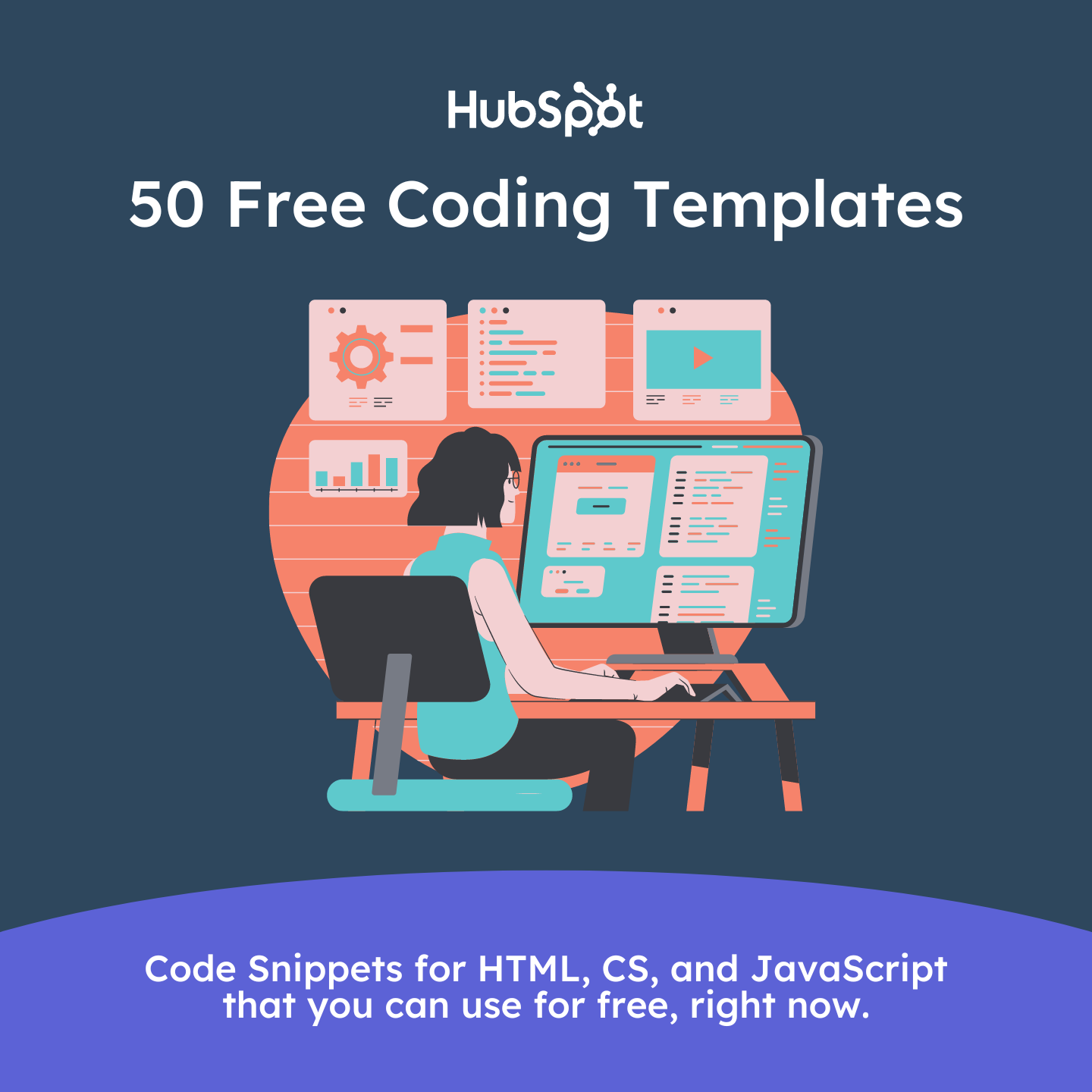
50 Free Coding Templates
Free code snippet templates for HTML, CSS, and JavaScript -- Plus access to GitHub.
- Navigation Menus & Breadcrumbs Templates
- Button Transition Templates
- CSS Effects Templates
- And more!
Download Free
All fields are required.
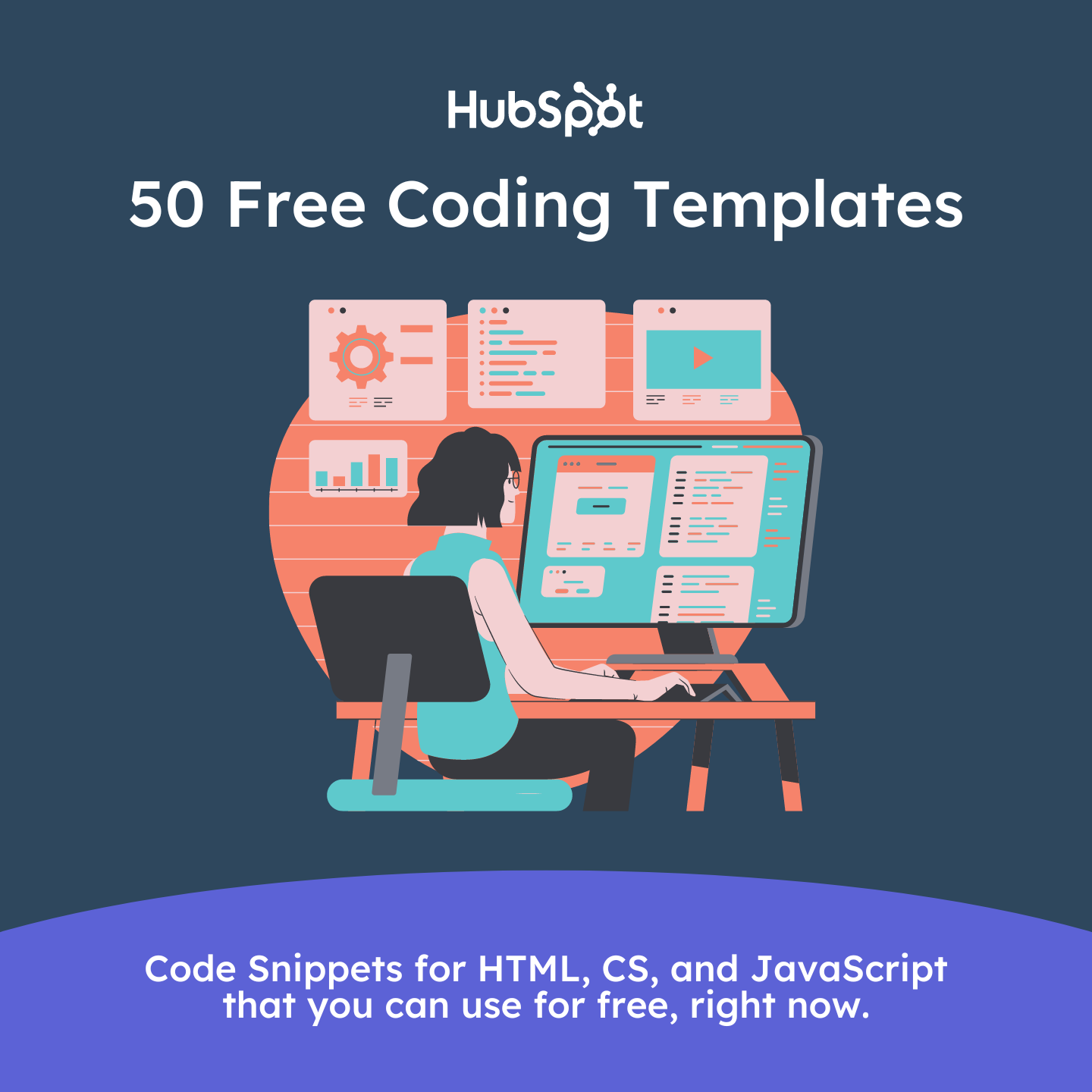
- Will you be able to avoid name collisions and specificity errors?
- Can you maintain and keep track of all your styles as your application grows?
- Will the performance of your application be a concern? Having a large number of CSS styles across multiple stylesheets will lead to a larger CSS bundle size, impacting the performance of your application.
Prioritize a styling technique that overcomes these concerns and provides a straightforward process for adding styling to your React applications. Such an approach saves you time and trouble down the road, making application styling intuitive, efficient, and — most importantly — a breeze.
Whether you’re building a new project from scratch or adding Bootstrap to an existing application, it can save you development time and effort and help you create a more polished and aesthetically appealing website that will look great on any device.