Today, front-end frameworks and libraries are becoming an essential part of the modern web development stack. React.js is a front-end library that has gradually become the go-to framework for modern web development within the JavaScript community.
For those who are new to web development, or trying to figure out what all the fuss is about, let’s look at React, how it works, and what makes it different from other JavaScript frameworks.Download Now: An Introduction to JavaScript
[Free Guide]
What is React.js?
React.js is an open-source JavaScript library, crafted with precision by Facebook, that aims to simplify the intricate process of building interactive user interfaces. Imagine a user interface built with React as a collection of components, each responsible for outputting a small, reusable piece of HTML code.
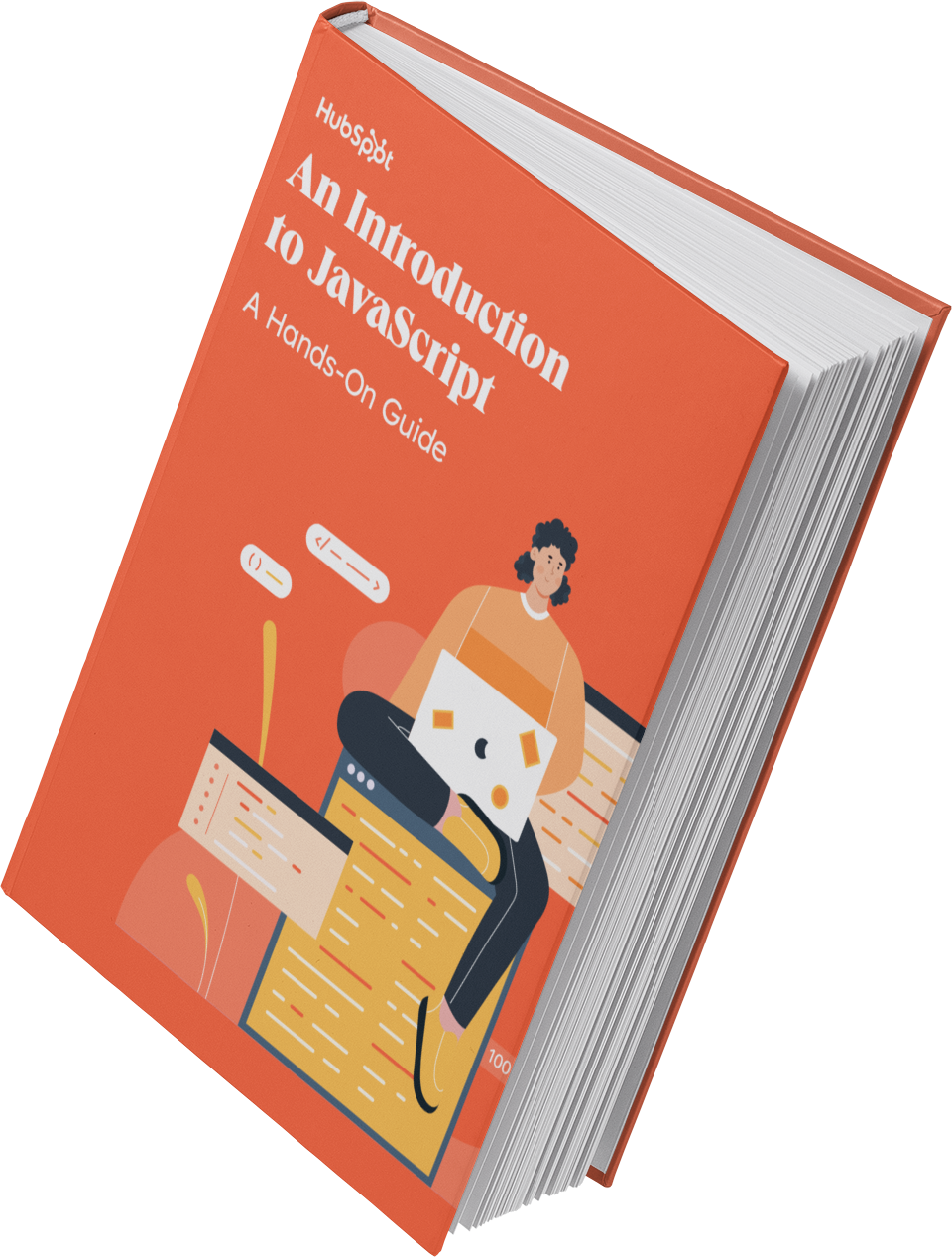
An Introduction to JavaScript
Learn the basics of one of the world's most popular programming languages. This guide covers:
- What JavaScript Is
- Object-Oriented Programming
- Data Types, Variables, and Operators
- And More!
Download Free
All fields are required.
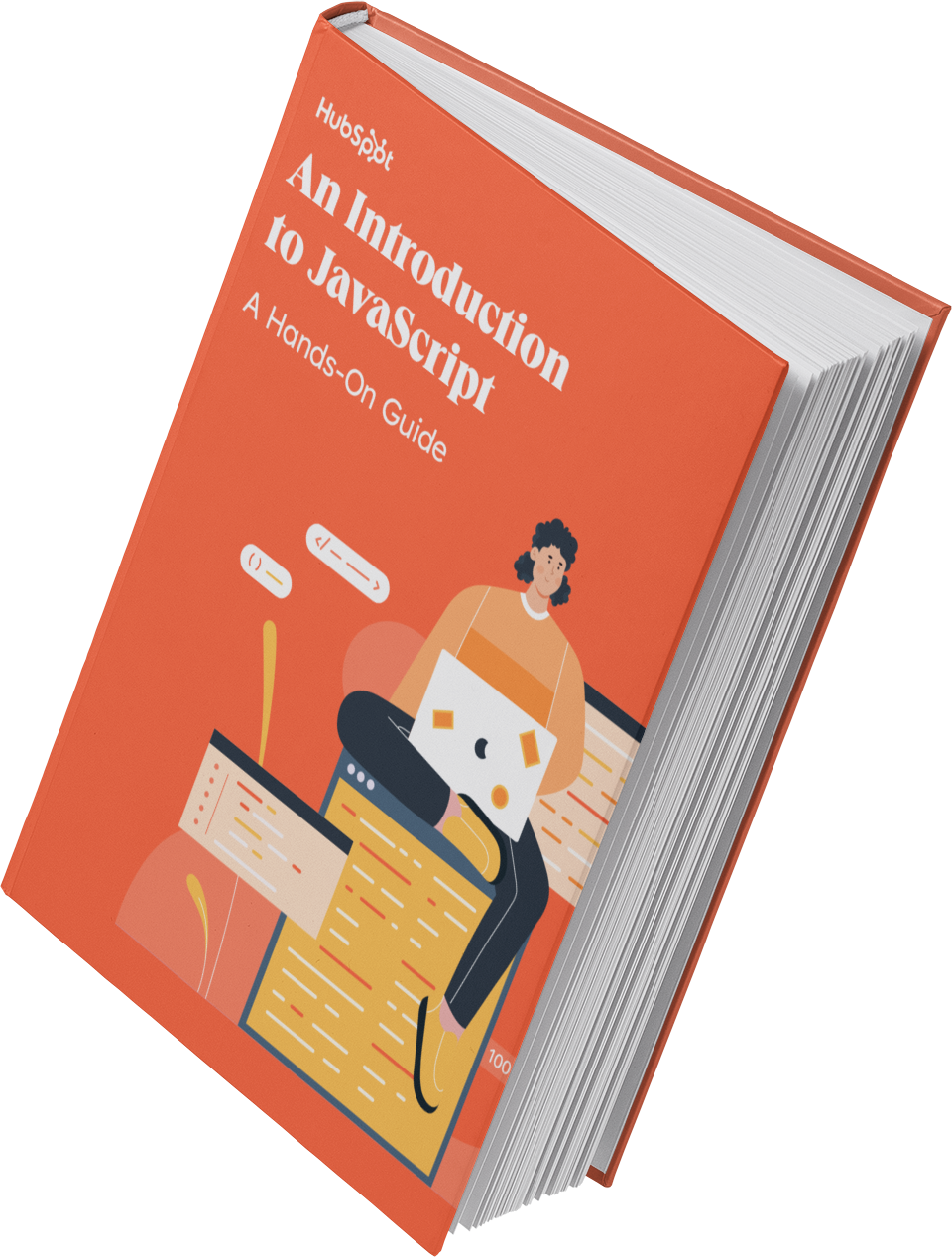
In React, you develop your applications by creating reusable components that you can think of as independent Lego blocks. These components are individual pieces of a final interface, which, when assembled, form the application’s entire user interface.
React’s primary role in an application is to handle the view layer of that application just like the V in a model-view-controller (MVC) pattern by providing the best and most efficient rendering execution. Rather than dealing with the whole user interface as a single unit, React.js encourages developers to separate these complex UIs into individual reusable components that form the building blocks of the whole UI. In doing so, the ReactJS framework combines the speed and efficiency of JavaScript with a more efficient method of manipulating the DOM to render web pages faster and create highly dynamic and responsive web applications.
A Brief History of React.js
Back in 2011, Facebook had a massive user base and faced a challenging task. It wanted to offer users a richer user experience by building a more dynamic and more responsive user interface that was fast and highly performant.
Jordan Walke, one of Facebook’s software engineers, created React to do just that. React simplified the development process by providing a more organized and structured way of building dynamic and interactive user interfaces with reusable components.
Facebook’s newsfeed used it first. Due to its revolutionary approach to DOM manipulation and user interfaces, React dramatically changed Facebook’s approach to web development and quickly became popular in JavaScript’s ecosystem after its release to the open-source community.
What does React.js do?
Typically, you request a webpage by typing its URL into your web browser. Your browser then sends a request for that webpage, which your browser renders. If you click a link on that webpage to go to another page on the website, a new request is sent to the server to get that new page.
This back-and-forth loading pattern between your browser (the client) and the server continues for every new page or resource you try to access on a website. This typical approach to loading websites works just fine, but consider a very data-driven website. The back and forth loading of the full webpage would be redundant and create a poor user experience.
Additionally, when data changes in a traditional JavaScript application, it requires manual DOM manipulation to reflect these changes. You must identify which data changed and update the DOM to reflect those changes, resulting in a full page reload.
React takes a different approach by letting you build what’s known as a single-page application (SPA). A single-page application loads only a single HTML document on the first request. Then, it updates the specific portion, content, or body of the webpage that needs updating using JavaScript.
This pattern is known as client-side routing because the client doesn’t have to reload the full webpage to get a new page each time a user makes a new request. Instead, React intercepts the request and only fetches and changes the sections that need changing without having to trigger a full page reload. This approach results in better performance and a more dynamic user experience.
React relies on a virtual DOM, which is a copy of the actual DOM. React’s virtual DOM is immediately reloaded to reflect this new change whenever there is a change in the data state. After which, React compares the virtual DOM to the actual DOM to figure out what exactly has changed.
React then figures out the least expensive way to patch the actual DOM with that update without rendering the actual DOM. As a result, React’s components and UIs very quickly reflect the changes since you don’t have to reload an entire page every time something updates.
How to Use React.js
In contrast to other frameworks like Angular, React doesn’t enforce strict rules for code conventions or file organization. This means developers and teams are free to set conventions that suit them best and implement React however they see fit. With React, you can use as much or as little as you need due to its flexibility.
Using React, you can create a single button, a few pieces of an interface, or your entire app’s user interface. You can gradually adopt and integrate it into an already existing application with a sprinkle of interactivity or, better yet, use it to build full-fledged powerful React applications from the ground up, depending on your need.
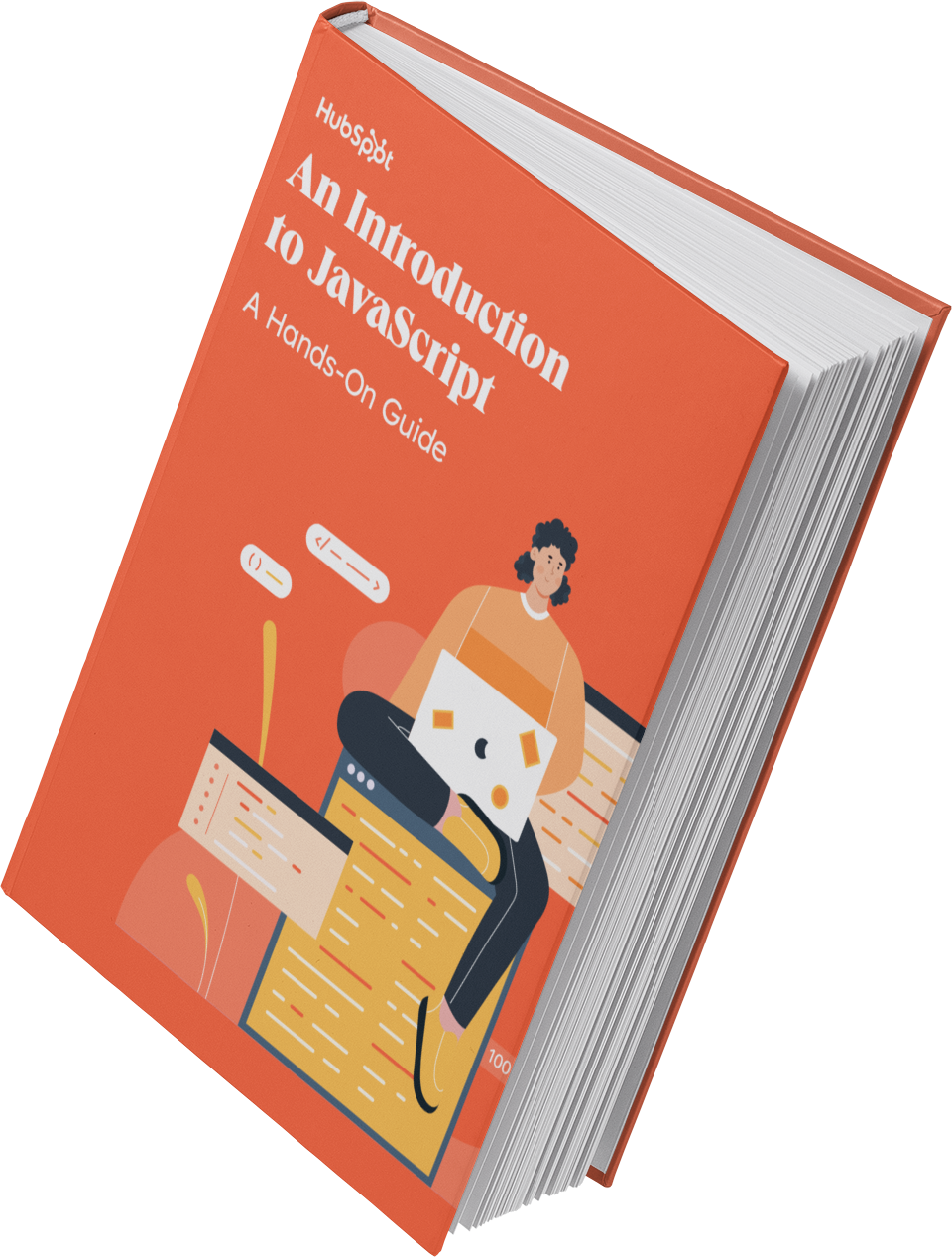
An Introduction to JavaScript
Learn the basics of one of the world's most popular programming languages. This guide covers:
- What JavaScript Is
- Object-Oriented Programming
- Data Types, Variables, and Operators
- And More!
Download Free
All fields are required.
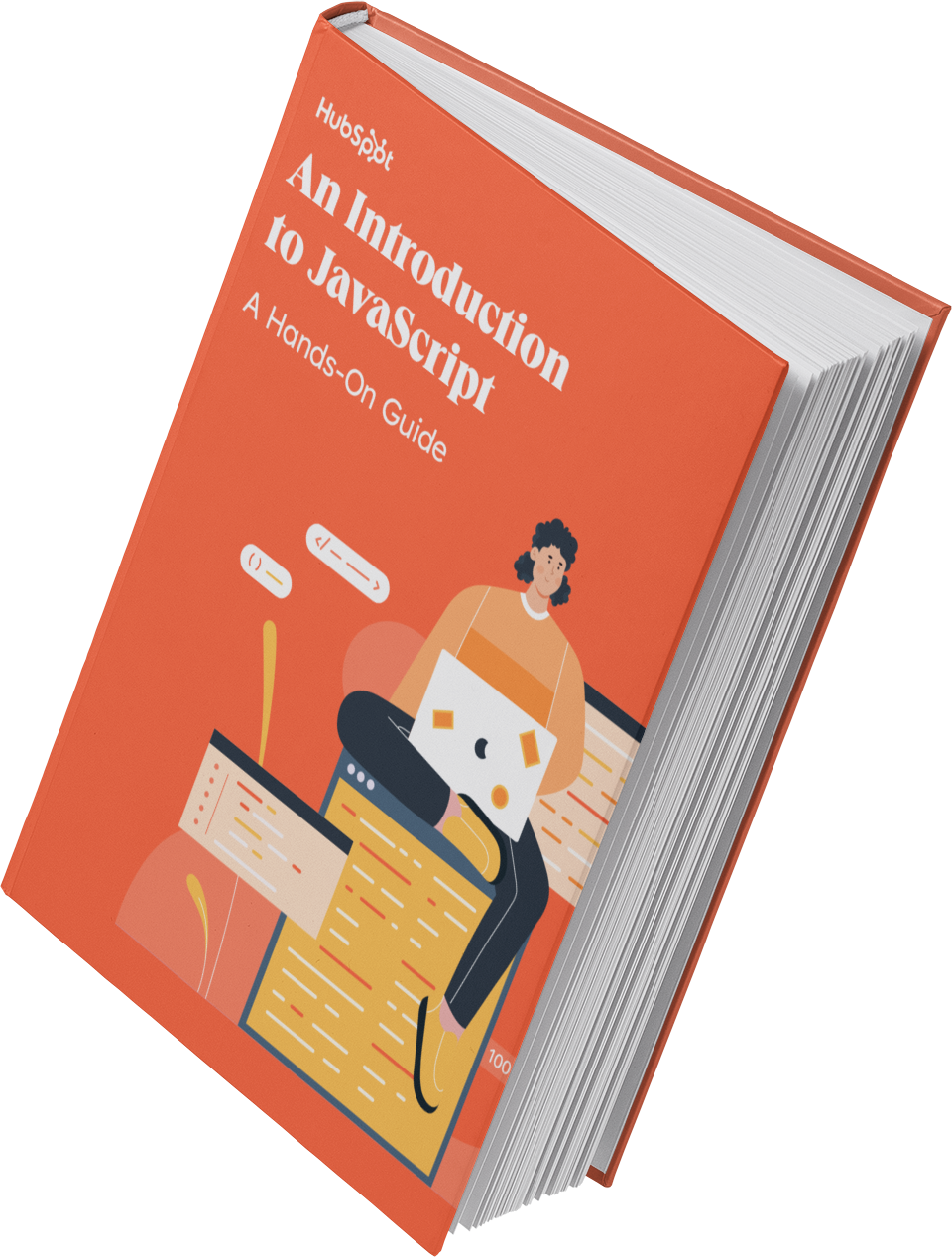
Integrating React into an Existing Website
When looking to add dynamic interactivity to your website, React is an excellent choice. By integrating React, you can create reusable and interactive components that can be placed anywhere on your site, such as sidebars or widgets. Here's a simplified breakdown of the steps to achieve this:
Step 1: Adding CDN Scripts to HTML:
- Begin by adding the core React library API from CDN to your website's HTML index file.
- Next, incorporate the React DOM from the CDN; this is essential for rendering components to the Document Object Model (DOM).
- Then, include Babel from the CDN, which transpiles React code ensuring compatibility across browsers.
Also, don't forget to load your React component file.
The first step is to add the two main CDN scripts to your website’s HTML index file. You need these scripts to load React into your app over a CDN service.
Step 2: Marking the Render Location on Your Website:
- Designate a location in your HTML where the React component will render. This can be done by adding a <div> element with a unique ID, which we will reference in our React code.
Step 3: Crafting the React Component:
- Create a React component, in this case, named like_widget.js.
- This component will have a simple function returning a JSX syntax that renders a "Hello World" message.
- With the help of ReactDOM, we then render this component to the DOM, targeting the unique ID we previously set in our HTML.
Hello World
; // Select the container const container = document.getElementById("like_widget_container"); // Render the component to the DOM ReactDOM.render(If you run the application, it will render “Hello World” to the browser in the exact spot you marked as shown below.
You might have noticed a weird syntax called JavaScript XML (JSX) returned by the ActualWidget function. You use JSX with React to combine HTML and JavaScript with ease. Facebook developed JSX as a syntax extension for JavaScript to extend HTML functionality by embedding it directly into JavaScript code. With JSX, there is no need to separate HTML and JS codes as React allows you to write declarative HTML directly in JavaScript code.
Creating a Fully-Fledged React App
Although you can easily drop React into an existing web application to create small pieces of an interface, it is more practical to use React to build fully-fledged web applications. However, React arguably has heavy tooling configuration requirements that are typically daunting and tedious to set up when creating new React applications.
Luckily, you don’t need to learn this build setup or configure the build tools yourself. Facebook has created a Node package command-line tool called create-react-app to help you generate a boilerplate version of a React application. This package benefits you from working out of the box and provides a consistent structure for React applications that you will recognize when moving between React projects.
Creating a new React project is as simple as running the following commands in your terminal:
React.js Examples
Because of its ability to create fast, efficient, and scalable web applications, React has gained stability and popularity. Thousands of web applications use it today, from well-established companies to new start-ups. Here, we delve into a handful of applications, illustrating how React.js has been instrumental in enhancing user experience and interaction.
As the genesis of React.js, Facebook exemplifies the library’s prowess. React streamlines the platform's real-time features, such as likes, comments, and status updates, ensuring a seamless and dynamic user experience. The modular nature of React facilitates Facebook's constantly evolving interface, adapting to the needs of over 2.8 billion monthly active users.
Instagram's web experience is a canvas of interactive elements, all orchestrated by React.js. Every image, like, story view, and direct message underscores React’s ability to handle intricate user interactions swiftly. The platform’s elegant, user-friendly interface is a testament to React’s prowess in delivering high-performance user interactions.
Airbnb exemplifies how React.js can transform complex data into an intuitive user experience. Each property listing, interactive map, and real-time booking is a symphony of React components working in unison to create a seamless user journey from browsing to booking.
A few other notable mentions are:
- Uber
- Airbnb
- The New York Times
- Khan Academy
- Codecademy
- SoundCloud
- Discord
- WhatsApp Web
React has also grown more robust and can now be used to build native mobile applications using React Native and Desktop apps using Electron.js.
Getting Started With React.JS
This article introduced React.js, provided its history, and showed how React extends the capabilities of JavaScript. It also provided some example use cases for how developers use React.js and some brief code snippets showcasing React.js code and its syntax to highlight why developers choose to use React.js instead of using JavaScript alone. The article concluded with some real-world examples of popular apps built using React.js.
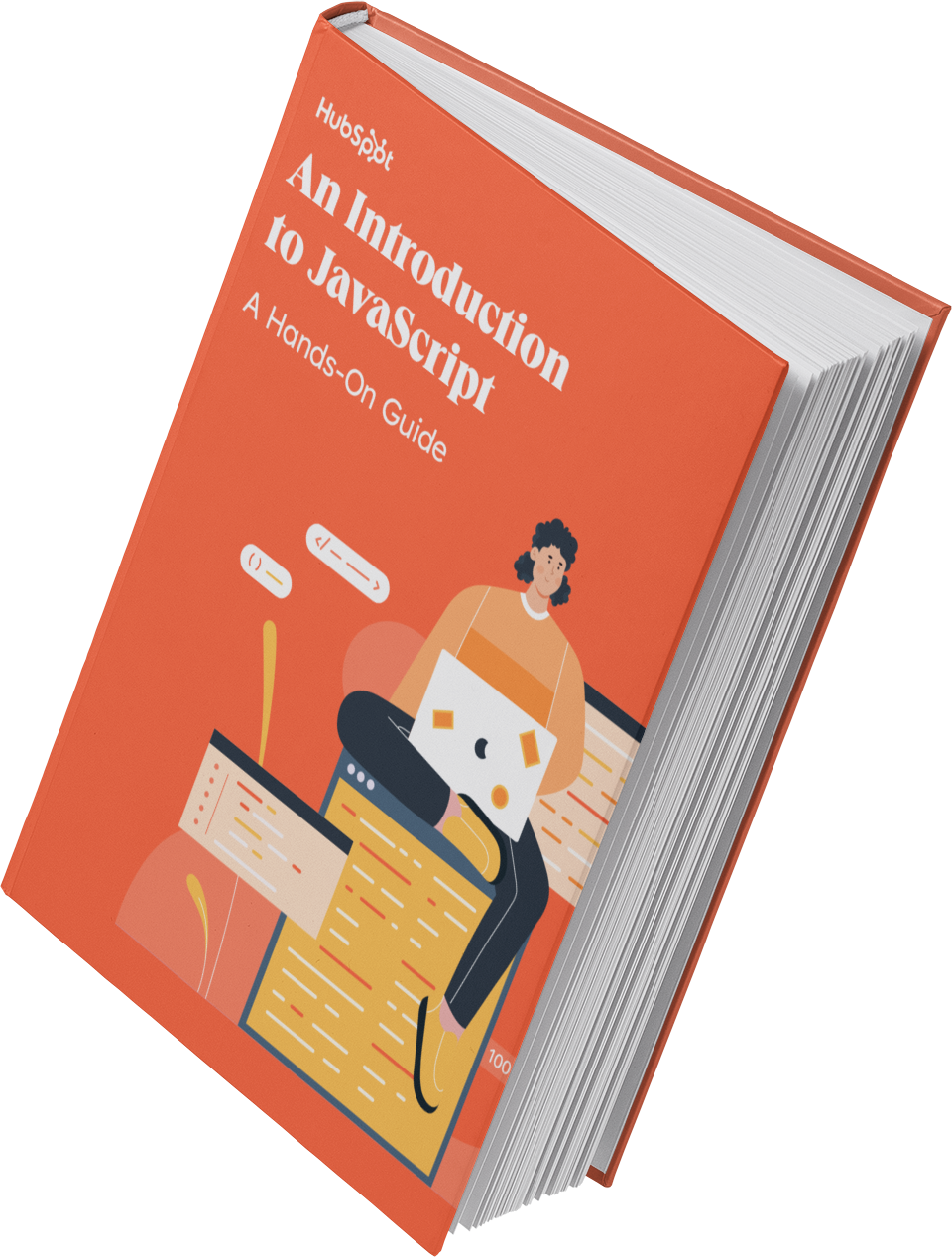
An Introduction to JavaScript
Learn the basics of one of the world's most popular programming languages. This guide covers:
- What JavaScript Is
- Object-Oriented Programming
- Data Types, Variables, and Operators
- And More!
Download Free
All fields are required.
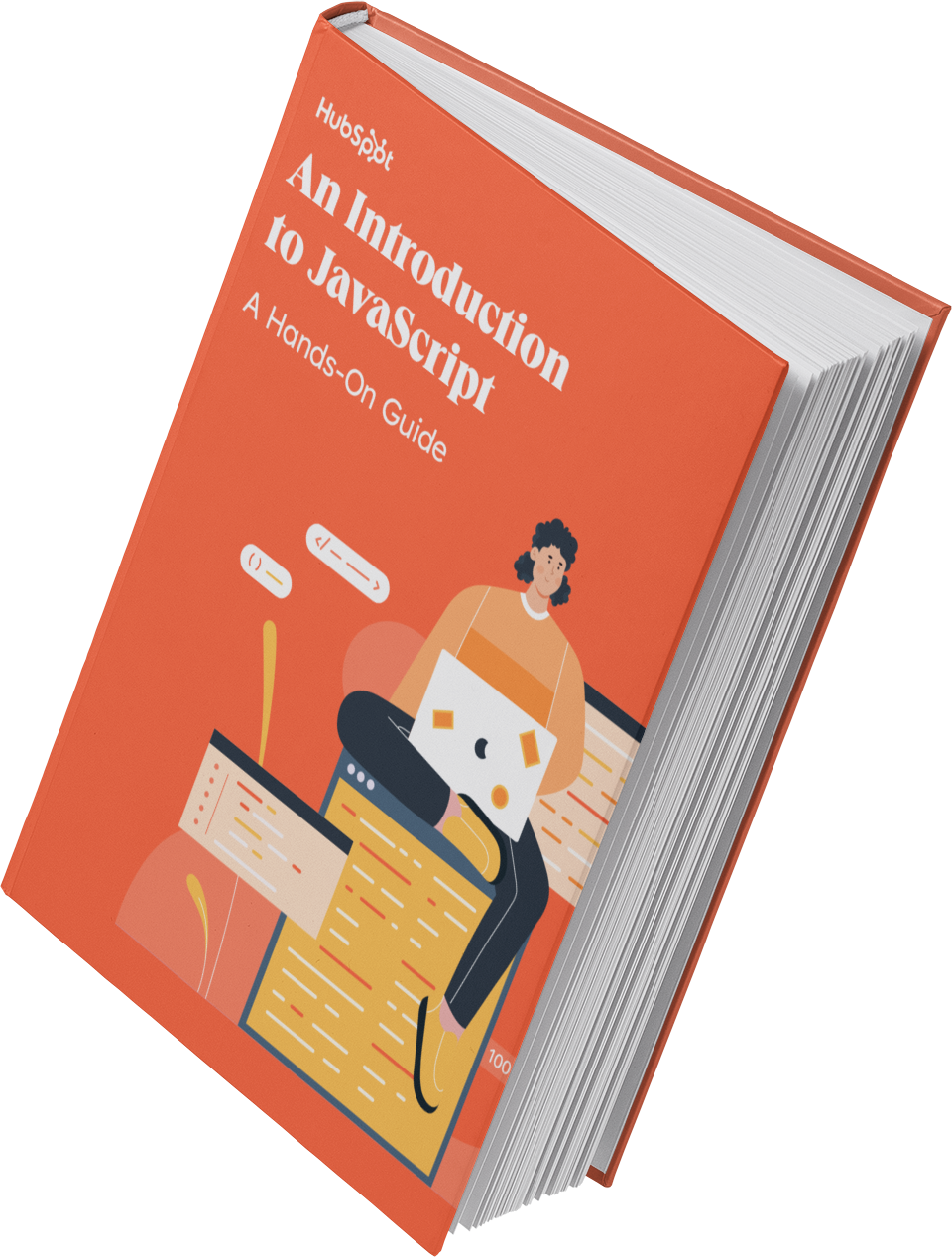
React provides state-of-the-art functionality and is an excellent choice for developers looking for an easy-to-use and highly productive JavaScript framework. Using React, you can build complex UI interactions that communicate with the server in record time with JavaScript-driven pages. Say goodbye to unnecessary full-page reloads and start building with React.
Editor's note: This post was originally published in June 2022 and has been updated for comprehensiveness.