In C programming, the Standard Library is a treasure trove of handy tools and functions that can turn your code from a mere collection of statements into a robust, efficient, and feature-rich application.
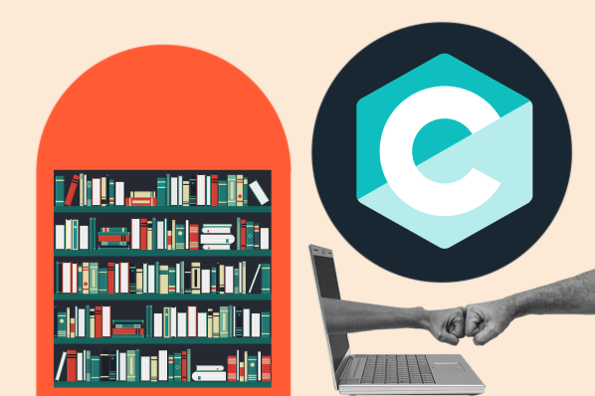
These pre-defined functions can simplify your coding tasks, boost efficiency, and save you valuable development time. Whether you're a seasoned programmer or just starting out, understanding and utilizing Standard Library functions is essential for coding scalable C programs.
In this post, we’ve curated a list of 55 Standard Library functions for C programmers, spanning from input/output operations to string manipulation, memory management, mathematical calculations, and more.
We'll explore not only their definition and syntax but also an example of each that you can reference for your programming endeavors.
Table of Contents
Standard Library Functions in C
The Standard Library is an integral part of C programming, offering a set of tools and functionalities that programmers can utilize to save time and effort. These functions have been extensively tested and optimized, making them reliable and efficient for use in various projects.
Character-Type Functions <ctype.h>
Character-type functions operate on individual characters or perform character-related operations.
1. isalpha()
This function checks if a character is an alphabetical character.
Example:
#include<stdio.h>
#include<ctype.h>
int main(){
char ch='A';
if(isalpha(ch)){
printf("%c is an alphabetic character.\n",ch);
}else{
printf("%c is not an alphabetic character.\n",ch);
}
return0;
}
Output:
A is an alphabetic character.
2. isdigit()
This function checks if a character is a decimal digit.
Example:
#include<stdio.h>
#include<ctype.h>
int main(){
char ch='5';
if(isdigit(ch)){
printf("%c is a digit.\n",ch);
}else{
printf("%c is not a digit.\n",ch);
}
return0;
}
Output:
5 is a digit.
3. islower()
This function checks if a character is a lowercase alphabetic character.
Example:
#include<stdio.h>
#include<ctype.h>
int main(){
char ch='a';
if(islower(ch)){
printf("%c is a lowercase letter.\n",ch);
}else{
printf("%c is not a lowercase letter.\n",ch);
}
return0;
}
Output:
a is a lowercase letter.
4. isupper()
This function checks if a character is an uppercase alphabetic character.
Example:
#include<stdio.h>
#include<ctype.h>
int main(){
char ch='A';
if(isupper(ch)){
printf("%c is an uppercase letter.\n",ch);
}else{
printf("%c is not an uppercase letter.\n",ch);
}
return0;
}
Output:
A is an uppercase letter.
5. tolower()
This function converts an uppercase character to its lowercase equivalent.
Example:
#include<stdio.h>
#include<ctype.h>
int main(){
char ch='A';
char lowercase=tolower(ch);
printf("%c converted to lowercase is %c.\n",ch,lowercase);
return0;
}
Output:
A converted to lowercase is a.
6. toupper()
This function converts a lowercase character to its uppercase equivalent.
Example:
#include<stdio.h>
#include<ctype.h>
int main(){
char ch='a';
char uppercase=toupper(ch);
printf("%c converted to uppercase is %c.\n",ch,uppercase);
return0;
}
Output:
a converted to uppercase is A.
Assert Functions <assert.h>
The <assert.h> header provides the assert() macro, which is commonly used for debugging and ensuring that certain conditions hold true during program execution. While assert() is the primary function of this header, here are a few functions/macros that are commonly used in conjunction with itt:
7. assert()
This function checks if a given expression is true. If the expression is false, it triggers an assertion failure, causing the program to terminate and prompt an error message.
Example:
#include <assert.h>
int main() {
int x = 10;
assert(x == 9); // If false, the program will terminate with an error message.
return0;
}
Output:
Assertion `x == 9' failed.
8. NDEBUG
This macro controls whether assertions are enabled or disabled. When NDEBUG is defined, assertions are turned off. When it's not defined, assertions are active.
Example:
#define NDEBUG
#include <assert.h>
Output:
In this example, assertions aren’t active because they are defined.
9. static_assert()
This macro performs compile-time assertion checking. It verifies that a given expression is true at compile time, and if not, it results in a compilation error.
Example:
#include <assert.h>
static_assert(sizeof(int) >= 4, "int must be at least 4 bytes");
Output:
Checks the size of the integer to make sure it is at least 4 bytes.
Localization Functions <locale.h>
Localization is the process of adapting software to different languages and regions. In C programming, this is primarily done with the setlocale() function, however, this function can be used in a variety of ways.
10. setlocale(LC_ALL,)
Setting the locale to LC_ALL affects all aspects of a program's behavior related to language and region.
Example:
#include <locale.h>
#include <stdio.h>
int main() {
// Set the locale for all aspects of localization
setlocale(LC_ALL, "fr_FR.UTF-8"); // Example: French locale
printf("Bonjour le monde!\n"); // Display a message in French
return0;
}
Output:
Sets all aspects of localization to French.
11. setlocale(LC_TIME,)
Setting the locale to LC_TIME affects the formatting of dates and times.
Example:
#include <locale.h>
#include <stdio.h>
#include <time.h>
int main() {
// Set the locale for time and date formatting
setlocale(LC_TIME, "ja_JP.UTF-8"); // Example: Japanese locale
time_t rawtime;
struct tm *timeinfo;
time(&rawtime);
timeinfo = localtime(&rawtime);
char buffer[80];
strftime(buffer, sizeof(buffer), "%A, %B %d, %Y", timeinfo);
printf("Date and time in Japanese format: %s\n", buffer);
return0;
}
Output:
This sets the date and time to Japan’s timezone and formatting.
12.setlocale(LC_CTYPE,)
Setting the locale to LC_CTYPE affects character classification functions and character encoding.
Example:
#include <locale.h>
#include <stdio.h>
#include <wctype.h>
int main() {
// Set the locale for character classification and encoding
setlocale(LC_CTYPE, "ru_RU.UTF-8"); // Example: Russian locale
wint_t wideChar = L'Э'; // Cyrillic letter "E" (Э) in Russian
if (iswalpha(wideChar)) {
printf("%lc is an alphabetic character in Russian.\n", wideChar);
} else {
printf("%lc is not an alphabetic character in Russian.\n", wideChar);
}
return0;
}
Output:
Set the locale for character classification to Russian.
Math Functions <math.h>
These are just a sample of the math functions you can use with the <math.h> header. For more examples, check out this blog post.
13. sqrt()
This function calculates the square root of a given number.
Example:
#include<stdio.h>
#include<math.h>
intmain(){
doublenumber=25.0;
doubleresult=sqrt(number);
printf("Square root of %.2f is %.2f\n",number,result);
return0;
}
Output:
Square root of 25.00 is 5.00
14. sin()
This function calculates the sine of an angle in radians.
Example:
#include <stdio.h>
#include <math.h>
int main() {
double angle = 1.047; // 60 degrees in radians
double sineValue = sin(angle);
printf("Sine of %.2f radians is %.2f\n", angle, sineValue);
return0;
}
Output:
Sine of 1.05 radians is 0.87
15. exp()
This function calculates the exponential value of a given number.
Example:
#include<stdio.h>
#include<math.h>
intmain(){
doublex=2.0;
doubleresult=exp(x);
printf("e raised to the power of %.2f is %.2f\n",x,result);
return0;
}
Output:
e raised to the power of 2.00 is 7.39
16. round()
This function rounds a floating-point number to the nearest integer value.
Example:
#include<stdio.h>
#include<math.h>
intmain(){
doublenum1=7.3;
doublenum2=7.7;
longrounded1=round(num1);
longrounded2=round(num2);
printf("Rounded value of %.2f is %ld\n",num1,rounded1);
printf("Rounded value of %.2f is %ld\n",num2,rounded2);
return0;
}
Output:
Rounded value of 7.30 is 7
Rounded value of 7.70 is 8
String Functions <string.h>
Similar to the math functions, these are just a few examples of C functions that use the <string.h> header. For more examples, check out this dedicated blog post.
17. strcpy()
This function copies one string to another.
Example:
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, world!";
char destination[20];
strcpy(destination, source);
printf("Copied string: %s\n", destination);
return0;
}
Output:
Copied string: Hello, World!
18. strlen()
This function calculates the length of a string.
Example:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "C programming";
size_t length = strlen(str);
printf("Length of the string: %zu\n", length);
return0;
}
Output:
Length of the string: 13
19. strcat()
This function concatenates two strings into one.
Example:
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello, ";
char str2[] = "world!";
strcat(str1, str2);
printf("Concatenated string: %s\n", str1);
return0;
}
Output:
Concatenated string: Hello, world!
20. strcmp()
This function compares two strings lexically.
Example:
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "apple";
char str2[] = "banana";
int result = strcmp(str1, str2);
if (result < 0) {
printf("%s comes before %s\n", str1, str2);
} elseif (result > 0) {
printf("%s comes after %s\n", str1, str2);
} else {
printf("%s and %s are the same\n", str1, str2);
}
return0;
}
Output:
apple comes before banana
Date Time Functions <time.h>
Date time functions allow you to manipulate the current date and time of your program. Here are a few functions that you can use with this header.
21. time()
This function gives you the current time.
Example:
#include<stdio.h>
#include<time.h>
intmain(){
time_tcurrentTime;
time(¤tTime);
printf("Current time: %s",ctime(¤tTime));
return0;
}
Output:
Current time: Tue Sep 19 16:08:16 2023
22. strftime()
This function formats time into a custom date and time representation.
Example:
#include <stdio.h>
#include <time.h>
int main() {
struct tm timeinfo;
time_t currentTime;
time(¤tTime);
localtime_r(¤tTime, &timeinfo);
char buffer[80];
strftime(buffer, sizeof(buffer), "%A, %B %d, %Y %H:%M:%S", &timeinfo);
printf("Custom date and time format: %s\n", buffer);
return0;
}
Output:
Custom date and time format: Tuesday, September 19, 2023 16:09:05
23. difftime()
This function calculates the difference in seconds between two time values.
Example:
#include <stdio.h>
#include <time.h>
int main() {
time_t startTime, endTime;
time(&startTime);
// Perform some time-consuming task
time(&endTime);
double elapsedSeconds = difftime(endTime, startTime);
printf("Elapsed time: %.2f seconds\n", elapsedSeconds);
return0;
}
Output:
Elapsed time: 0.00 seconds
24. sleep()
This function suspends program execution for a specified number of seconds.
Example:
#include <stdio.h>
#include <time.h>
int main() {
printf("Starting a 5-second sleep...\n");
sleep(5); // Sleep for 5 seconds
printf("Sleep complete!\n");
return0;
}
Output:
Starting a 5-second sleep…
Sleep complete!
25. mktime()
Converts a struct tm time structure back to a time_t value. This can be used for date and time arithmetic.
Example:
#include <stdio.h>
#include <time.h>
int main() {
struct tm timeinfo = {0}; // Initialize a struct tm
timeinfo.tm_year = 121; // Year 2021 (since 1900)
timeinfo.tm_mon = 6; // July (0-based)
timeinfo.tm_mday = 1; // 1st day of the month
time_t result = mktime(&timeinfo);
printf("Epoch time for July 1, 2021: %ld\n", (long)result);
return0;
}
Output:
Epoch time for July 1, 2021: 1625097600
26. clock()
This function measures CPU time used by a program.
Example:
#include <stdio.h>
#include <time.h>
int main() {
clock_t start, end;
double cpu_time_used;
start = clock();
// Perform some time-consuming task
end = clock();
cpu_time_used = ((double)(end - start)) / CLOCKS_PER_SEC;
printf("CPU time used: %f seconds\n", cpu_time_used);
return0;
}
Output:
CPU time used: 0.000001 seconds
27. asctime()
Converts a struct tm time structure into a string.
Example:
#include <stdio.h>
#include <time.h>
int main() {
struct tm timeinfo = {0}; // Initialize a struct tm
timeinfo.tm_year = 121; // Year 2021 (since 1900)
timeinfo.tm_mon = 6; // July (0-based)
timeinfo.tm_mday = 1;
char* timeString = asctime(&timeinfo);
printf("Formatted date and time: %s\n", timeString);
return0;
}
Output:
Formatted date and time: Sun Jul 1 00:00:00 2021
28. localtime()
This function converts a time_t value into a struct tm time structure for local time.
Example:
#include <stdio.h>
#include <time.h>
int main() {
time_t currentTime;
struct tm *timeinfo;
time(¤tTime);
timeinfo = localtime(¤tTime);
printf("Current local time: %s", asctime(timeinfo));
return0;
}
Output:
Current local time: Tue Sep 19 16:18:02 2023
29. nanosleep()
This function makes the program sleep for a specified number of nanoseconds, allowing precise control over timing.
Example:
#include <stdio.h>
#include <time.h>
int main() {
struct timespec sleepTime;
sleepTime.tv_sec = 0;
sleepTime.tv_nsec = 500000000; // 500 milliseconds
printf("Sleeping for 500 milliseconds...\n");
nanosleep(&sleepTime, NULL);
printf("Sleep complete!\n");
return0;
}
Output:
Sleeping for 500 milliseconds...
Sleep complete!
Standard Input/Output Functions <stdio.h>
These are the standard input and output functions provided by C programming. They are some of the most fundamental functions you’ll use with this language.
30. printf()
This function prints formatted output to the standard output (usually the console).
Example:
#include <stdio.h>
int main() {
int age = 30;
printf("My age is %d years.\n", age);
return0;
}
Output:
My age is 30 years.
31. scanf()
This function reads formatted input from the standard input.
Example:
#include <stdio.h>
int main() {
int age;
printf("Enter your age: ");
scanf("%d", &age);
printf("You entered: %d years\n", age);
return0;
}
Output:
Enter your age: 27
You entered: 27 years
32. fopen(), fprintf(), fclose()
These functions are typically used together. They open a file, write formatted data to it, and then close the file.
Example:
#include <stdio.h>
int main() {
FILE *file;
file = fopen("output.txt", "w");
if (file != NULL) {
fprintf(file, "Hello, File!\n");
fclose(file);
} else {
printf("Failed to open the file.\n");
}
return0;
}
Output:
This would print “Hello, File!” to the opened file.
33. getchar()
This function reads a single character from the standard input.
Example:
#include <stdio.h>
int main() {
char ch;
printf("Enter a character: ");
ch = getchar();
printf("You entered: %c\n", ch);
return0;
}
Output:
Enter a character: G
You entered: G
34. putchar()
This function adds a single character to the standard output.
Example:
#include <stdio.h>
int main() {
char ch = 'A';
putchar(ch);
putchar('\n'); // Output a newline character
return0;
}
Output:
A
35. fgets()
This function reads a line of text from the standard input or a file.
Example:
#include <stdio.h>
int main() {
char buffer[100];
printf("Enter a line of text: ");
fgets(buffer, sizeof(buffer), stdin);
printf("You entered: %s", buffer);
return0;
}
Output:
Enter a line of text: HubSpot
You entered: HubSpot
36. puts()
This function writes a string to the standard output with an automatic newline character.
Example:
#include <stdio.h>
int main() {
char greeting[] = "Hello, World!";
puts(greeting);
return0;
}
Output:
Hello, World!
37. Boolean Function
A Boolean function returns either true or false based on some condition or criteria. Conventionally, 0 is used to represent false, and any non-zero value (usually 1) is used to represent true.
Example:
#include<stdio.h>
// Boolean function to check if a number is even
intisEven(intnumber){
if(number%2==0){
return1;// True, the number is even
}else{
return0;// False, the number is odd
}
}
intmain(){
intnum;
printf("Enter an integer: ");
scanf("%d",&num);
if(isEven(num)){
printf("%d is even.\n",num);
}else{
printf("%d is odd.\n",num);
}
return0;
}
Output:
Enter an integer: 5
5 is odd.
Standard Utility Functions <stdlib.h>
Standard utility functions are commonly used to provide general-purpose functionality. These functions are not specific to any particular domain or task but serve as fundamental tools for solving common programming problems.
38. malloc()
This function allocates a specified number of bytes of memory on the heap.
Example:
#include <stdio.h>
#include <stdlib.h>
int main() {
int *arr = (int *)malloc(5 * sizeof(int));
if (arr != NULL) {
for (int i = 0; i < 5; i++) {
arr[i] = i * 10;
printf("arr[%d] = %d\n", i, arr[i]);
}
free(arr); // Don't forget to free the allocated memory
} else {
printf("Memory allocation failed.\n");
}
return0;
}
Output:
arr[0] = 0
arr[1] = 10
arr[2] = 20
arr[3] = 30
arr[4] = 40
39. free()
This function releases memory previously allocated with malloc(), calloc(), or realloc().
Example:
#include <stdlib.h>
int main() {
int *arr = (int *)malloc(5 * sizeof(int));
// ... (allocate and use memory)
free(arr); // Release the allocated memory
return0;
}
Output:
Allocated memory is released.
40. rand()
This function generates a pseudo-random integer.
Example:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
srand(time(NULL)); // Seed the random number generator
int randomNum = rand();
printf("Random number: %d\n", randomNum);
return0;
}
Output:
Random number: 1088745074
41. exit()
This function terminates the program and returns an exit status.
Example:
#include <stdlib.h>
int main() {
// ... (program logic)
exit(0); // Exit with status 0 (success)
}
Output:
Exited program successfully.
42. atexit()
This function registers a function to be called at program exit.
Example:
#include <stdio.h>
#include <stdlib.h>
void exitHandler() {
printf("Exiting program...\n");
}
int main() {
atexit(exitHandler);
// ... (program logic)
return0;
}
Output:
Exiting program...
44. system()
This function invokes a command interpreter to run a shell command.
Example:
#include <stdlib.h>
int main() {
system("echo Hello, World!");
return0;
}
Output:
echo Hello, World!
45. getenv()
This function retrieves the value of an environment variable.
Example:
#include <stdio.h>
#include <stdlib.h>
int main() {
char *path = getenv("PATH");
if (path != NULL) {
printf("PATH environment variable: %s\n", path);
} else {
printf("PATH environment variable not found.\n");
}
return0;
}
Output:
PATH environment variable: /rbin
Signal Handling Functions <signal.h>
Signal handling functions allow programmers to manage and respond to various signals sent to a program by the operating system or other processes.
46. signal()
This sets a function to be called when a specified signal occurs.
Example:
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
// Signal handler function
void handleSignal(int signum) {
printf("Signal %d received.\n", signum);
}
int main() {
// Register the signal handler for SIGINT (Ctrl+C)
signal(SIGINT, handleSignal);
printf("Waiting for a signal...\n");
while (1) {
// Your program logic here
}
return 0;
}
Output:
Waiting for a signal…
47. raise()
This function raises a specified signal.
Example:
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
int main() {
printf("Raising a custom signal...\n");
raise(SIGUSR1); // Raise a custom signal (SIGUSR1)
printf("Signal raised.\n");
return 0;
}
Output:
Raising a custom signal...
User defined signal 1
48. sigaction()
This function sets a custom handler for a signal and provides more control over signal handling.
Example:
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
// Signal handler function
void handleSignal(int signum) {
printf("Signal %d received.\n", signum);
}
int main() {
struct sigaction action;
action.sa_handler = handleSignal;
sigemptyset(&action.sa_mask);
action.sa_flags = 0;
// Register the signal handler using sigaction for SIGINT
sigaction(SIGINT, &action, NULL);
printf("Waiting for a signal...\n");
while (1) {
// Your program logic here
}
return 0;
}
Output:
Waiting for a signal…
49. sigprocmask()
This function manipulates the signal mask.
Example:
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
int main() {
sigset_t newMask, oldMask;
sigemptyset(&newMask);
sigaddset(&newMask, SIGINT);
// Block SIGINT signal
sigprocmask(SIG_BLOCK, &newMask, &oldMask);
printf("SIGINT is blocked. Press Ctrl+C to unblock.\n");
getchar(); // Wait for user input
// Unblock SIGINT signal
sigprocmask(SIG_UNBLOCK, &newMask, NULL);
printf("SIGINT is unblocked. Press Ctrl+C to exit.\n");
while (1) {
// Your program logic here
}
return 0;
}
Output:
SIGINT is blocked. Press Ctrl+C to unblock.
50. kill()
This functions sends a signal to a specified process.
Example:
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
#include <unistd.h>
int main() {
pid_t pid = getpid(); // Get the current process ID
printf("Sending a custom signal to process %d...\n", pid);
kill(pid, SIGUSR1); // Send a custom signal (SIGUSR1) to the current process
return 0;
}
Output:
Sending a custom signal to process 5742...
User defined signal 1
Handling Variable-Length Arguments <stdarg.h>
These functions accept a variable number of arguments. This allows programmers to work with different numbers and types of arguments, providing flexibility and versatility.
51. va_start()
This function initializes the va_list argument list.
Example:
#include <stdio.h>
#include <stdarg.h>
void exampleFunction(int count, ...) {
va_list args;
va_start(args, count);
// Access and process arguments using va_arg
va_end(args);
}
int main() {
// Call exampleFunction or perform other program logic here
return0;
}
Output:
Initalizes the va_list.
52. va_arg()
This function retrieves the next argument from the va_list.
Example:
#include <stdio.h>
#include <stdarg.h>
void printInts(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
int value = va_arg(args, int); // Retrieve an integer argument
printf("%d ", value);
}
va_end(args);
}
int main() {
printInts(3, 10, 20, 30);
return 0;
}
Output:
10 20 30
53. va_end()
This function cleans up the va_list argument list.
Example:
#include <stdio.h>
#include <stdarg.h>
void exampleFunction(int count, ...) {
va_list args;
va_start(args, count);
// Access and process arguments using va_arg
va_end(args);
}
int main() {
// Call exampleFunction or perform other program logic here
return0;
}
Output:
List is cleaned.
54. va_copy()
This function copies the va_list argument list.
Example:
#include <stdio.h>
#include <stdarg.h>
void exampleFunction(int count, ...) {
va_list args1, args2;
va_start(args1, count); // Initialize the first argument list
va_copy(args2, args1); // Copy the first argument list to the second
// Access and process arguments using args1 and args2
va_end(args1); // Clean up the first argument list
va_end(args2); // Clean up the second argument list
}
int main() {
// Call exampleFunction or perform other program logic here
return 0;
}
Output:
This function copies the argument list and cleans it up.
Jump Functions <setjmp.h>
Jump functions are powered by the setjmp() and longjmp() functions.
55. setjmp(), longjmp()
The setjmp() function is used to save the program's state while the longjmp() is used to jump back to that state, allowing non-local control flow.
Example:
#include <stdio.h>
#include <setjmp.h>
jmp_buf buffer;
void foo() {
printf("foo() called\n");
longjmp(buffer, 1); // Jump back to setjmp point
}
int main() {
if (setjmp(buffer) == 0) {
printf("setjmp() called\n");
foo();
} else {
printf("longjmp() called\n");
}
return0;
}
Output:
setjmp() called
foo() called
longjmp() called
Using the Standard Library in C Programming
The Standard Library gives C programmers the ability to elevate their coding skills and accelerate their development process. These functions have been refined and optimized over time, providing tried-and-tested solutions that eliminate the need for manual production.
From seasoned programmers to beginners, these functions can significantly enhance your productivity and give you more time to focus on building the core logic of your applications.