C programming is a powerful language for building efficient software and creating functions in C is vital for building well-structured code. C functions break down complex tasks into manageable parts and improve your code's readability, making it easier to maintain over time.
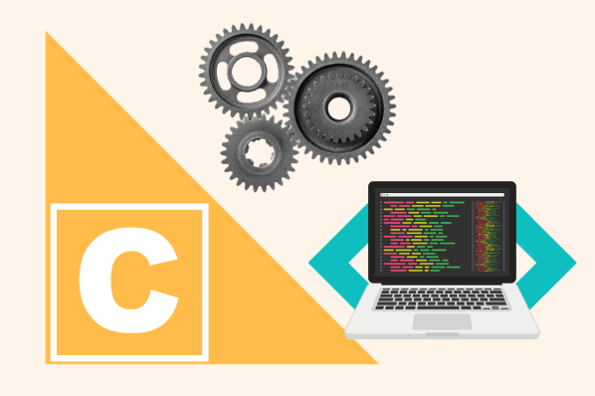
In this post, we‘ll provide a beginner-friendly guide on how to write functions in C. Whether you’re new to programming or looking to strengthen your skills, this article will equip you with the knowledge you need to master function creation, declaration, definition, and invocation.
How to Write Functions in C
C functions are written with three components:
- Function Declaration
- Function Definition
- Function Call
When you combine these together, you get the basic syntax outlined below.
C Function Syntax
Here is the basic syntax for a C function:
return_type function_name (parameter1_type parameter1_name, parameter2_type parameter2_name, ...);
- return_type: This specifies the type of the value that the function will return. If the function doesn't return any value, you can use void as the return type.
- function_name: This is the name of the function. Typically, the name of your function reflects its purpose.
- parameter1_type, parameter2_type: These are the data types of the parameters that the function takes. Parameters are variables used to pass values into the function. You can have multiple parameters, but they need to be separated with commas.
- parameter1_name, parameter2_name: These are the names of the parameters. Each parameter has a corresponding name that refers to the values passed into the function.
Now that you see what goes into a C function, let’s look at each component in more detail.
Declaring Functions in C
You can declare a function in C by specifying its name, return type, and parameters. Function declarations are typically placed at the beginning of your program or in header files to inform the compiler about the functions that will be defined later in the code. This helps the compiler understand the function's signature and how it should be called.
C Function Definition
In C programming, defining a function involves providing the actual implementation or body of the function. It specifies what the function does when it is called. Declaring a function is simply informing the compiler about the existence of the function before its actual definition.
Defining Functions in C
For example, let's define a function named “add” that takes two integers as parameters and returns their sum:
intadd(intnum1,intnum2)
{intsum;sum=num1+num2;returnsum;}
In this example, you can see that we define our function and then identify int num1 and int num 2 as our two parameters.
Calling Functions in C
You can call a function by using its name followed by parentheses that may contain arguments (input values) -- if the function requires any. The function call causes the program to execute the statements within the function's body.
To call a function in C, you would typically use the following syntax:
return_type variable_name = function_name(arguments);
Here's an example of calling the “add” function we defined earlier:
int result = add(5, 3);
In this case, the function call add(5, 3) will compute the sum of 5 and 3, and the value 8 will be assigned to the variable result.
Let's quickly recap declaring, defining, and calling functions in C:
1. Declaring a function specifies the function's prototype (name, return type, and parameters) before its implementation.
2. Defining a function provides the actual implementation or body of the function.
3. Calling a function refers to invoking the function by using its name, passing any required arguments, and optionally capturing the return value.
In short, declaring a function establishes its existence and prototype, defining a function means providing its implementation, and calling a function runs the code within its body.
Before we wrap up, let’s review the two types of C functions.
Types of C Functions
There are two types of C functions: standard library functions and user-defined functions.
Standard Library Functions
Standard library functions are pre-defined functions that are available in the C programming language by default. They are part of the standard library, which is a collection of functions and macros specified in the C language standard.
Standard functions provide a wide range of functionality, and their usage simplifies programming by allowing you to leverage pre-built functions rather than writing the code from scratch.
To use standard library functions, you need to include their respective header files in your C program using the #include directive. For example, #include <stdio.h> includes the header file containing standard I/O functions.
User-Defined Functions
User-defined functions are functions that are created by the programmer to perform specific tasks within a C program. Unlike standard library functions that are provided by the C programming language, user-defined functions are written by the programmer to meet the specific requirements of the program.
User-defined functions allow programmers to modularize their codebase and make it more organized, readable, and maintainable. These functions are reusable, meaning they can be called multiple times from different parts of the program.
To create a user-defined function, you generally follow these two steps:
1. Function Prototype (Declaration): Specify the function's name, return type, and parameter list.
2. Function Definition (Implementation): Provide the actual body of the function, which contains the statements that execute when the function is called.
Here's an example of a simple user-defined function in C that calculates the square of a number:
#include <stdio.h> // Function prototype int square(int num);
intmain()
{
intnumber=5;intresult=square(number);printf("The square of %d is %d\n",number,result);return0;} // Function definition int square(int num)
{ return num * num; }
In this example, we defined a function named square that takes an integer num as an argument and returns its square root. We declared the function's prototype before the main function and provided the function's implementation after the main function. By calling the square function within the main function, we can calculate the square of the number and display the result on the console.
User-defined functions can have any number of parameters. They can return a value or be void and can perform any desired logic based on the program's requirements. They allow you to encapsulate specific functionality, improve code reusability, and make your program more modular and maintainable.
Getting Started With C Functions
C functions allow you to break down complex tasks and improve code structure, readability, and reusability. With user-defined functions, you can tailor your code to specific requirements while leveraging parameters and return types for enhanced flexibility and optimization.
Armed with this knowledge, you are now equipped to elevate your codebase and create modular, scalable, and reliable software solutions. Keep practicing with this syntax so you can continue to explore the C programming language.