As a HubSpot Technical Blog Writer with a background in coding, I've personally discovered that coding is one of the most valuable skills you can build.
If you’re searching for “how to learn coding,” it might be because you want to advance your career or develop other skills in the computer programming world. And believe me, if I can dive into this world, so can you.
As a matter of fact, anyone can learn to code with time, dedication, and internet access. If you’re reading this, that includes you. My journey into coding, though challenging, has been one of the most rewarding experiences of my professional life, and I'm thrilled to share these insights with you.
Table of Contents
- What is coding?
- Why learn to code?
- How to Start Coding
- Coding vs. Programming
- Programming Languages
- Tips for Coding for Beginners
- Our Favorite Coding Resources
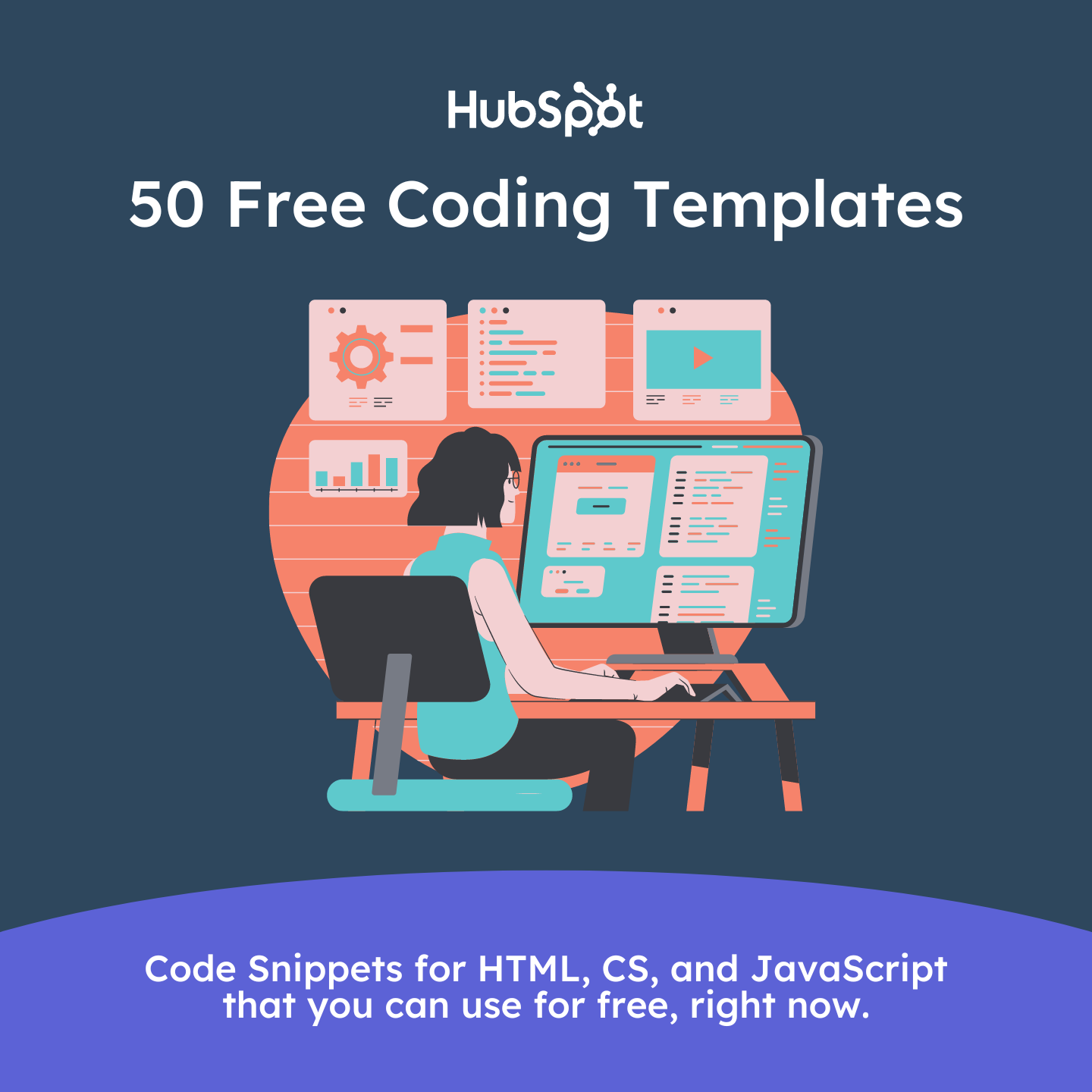
50 Free Coding Templates
Free code snippet templates for HTML, CSS, and JavaScript -- Plus access to GitHub.
- Navigation Menus & Breadcrumbs Templates
- Button Transition Templates
- CSS Effects Templates
- And more!
Download Free
All fields are required.
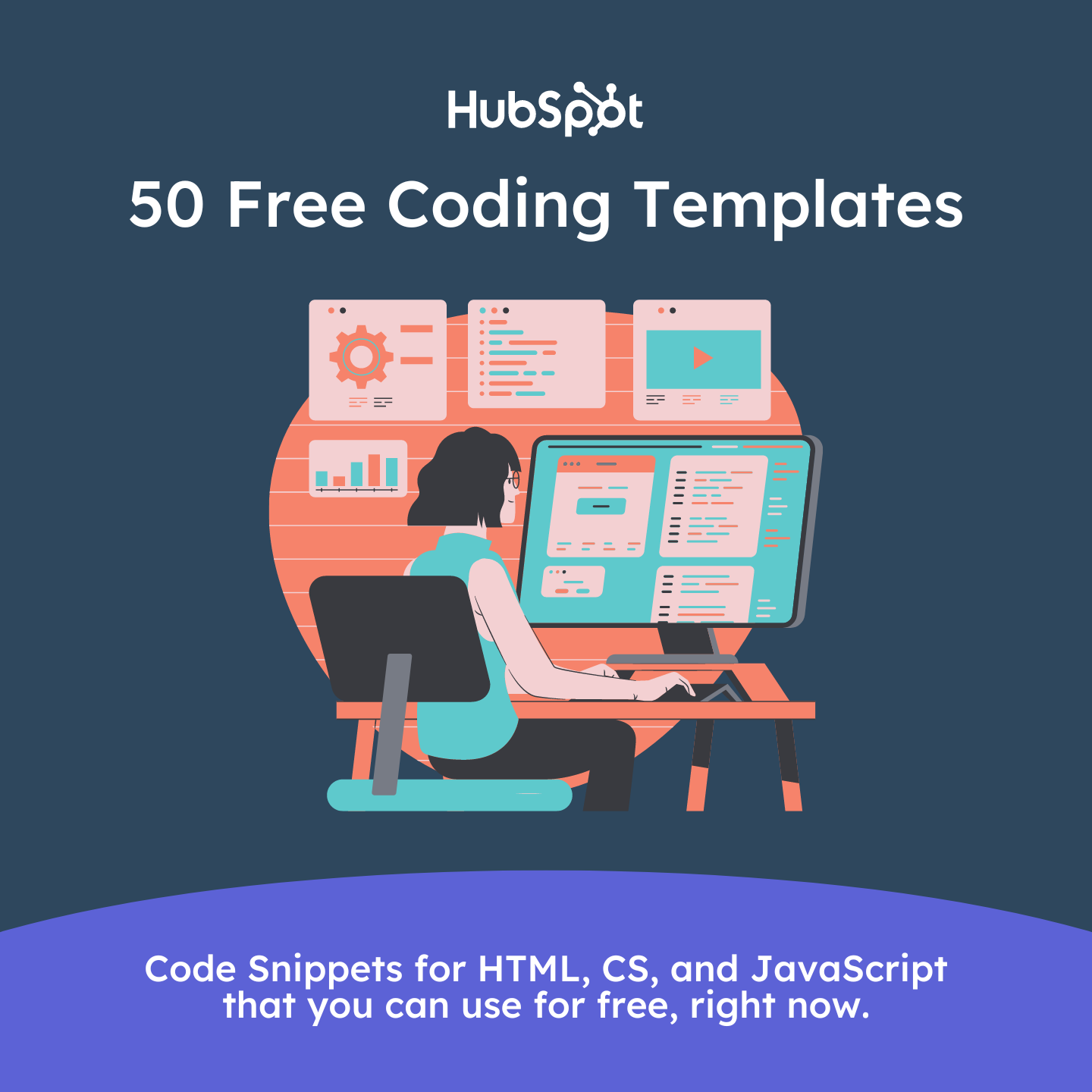
What is coding?
Coding is the process of using programming languages to give instructions to a computer. These instructions tell the computer how to perform specific tasks and power the websites, software, and applications people use every day.
Programming languages like Python, Java, and C++ provide the syntax and structure needed to write these instructions in a way that computers can understand and execute.
Why learn to code?
Before diving into your first lesson, I suggest that you think about why you want to code in the first place.
In my opinion, this will help you determine which programming language you learn first, what sorts of projects you want to complete, and ultimately, what you want to make of your skills.
Coding helps build professional skills.
In my journey, I've seen how coding opens doors to numerous opportunities, not just in tech but in various industries. It's a skill that empowers you to create, innovate, and solve problems in new ways.
As technology continues to weave into our daily lives, coding skills will become more desired among candidates — according to job board Indeed.com, several of the most in-demand skills fall under computing.
If you’re looking to make a career pivot into tech or to switch to a more technical role within your field, knowing at least one relevant programming language is a must.
This doesn’t just apply to developers, though. Web designers should know HTML, CSS, and JavaScript. Project managers should know the inner workings of the tools they help craft.
Even if you run a simple WordPress website, familiarizing yourself with front-end languages and some PHP goes a long way.
Even if you’re not pursuing a strictly technical role, coding experience is an asset. It shows technical know-how, the ability to grasp abstract concepts, and that you can solve complex problems.
Finally, coding knowledge enables you to take up freelance work or a full-time career.
Coding can help you earn more.
The average entry-level salary in the U.S. is $40,153 in 2022. But the average starting salary for a programmer is more than twice as much, at $85,293.
There's no denying the financial advantages of coding skills. The tech industry is known for its lucrative salary packages, and as someone who transitioned into this field, the financial uplift was a significant motivator.
We mentioned earlier that there are differences between coders and programmers. As you continue to learn about coding, you may start to specialize. Many of the highest coding salaries come from the ability to offer your skills in a specific type of coding.
For example, as you continue to code, you may become a developer. Besides writing code, developers also debug software and work with source code. Developers usually specialize in a specific programming language.
Developers often earn higher salaries than programmers and have high projected job growth. According to the U.S. Bureau of Labor Statistics, jobs for developers should grow by 22% by 2030.
Coding lets you create things.
One of the coolest benefits of learning how to code is the ability to bring your ideas to life. Have a concept for a website, app, or computer game? Now, you can build it exactly how you want, then share it with the world. I still remember the thrill of my first successful program—it was a simple task automation, but it felt like magic.
Whether you want to monetize your project, post it on an open-source platform like GitHub, or just make things as a hobby, you’ll have the knowledge and the tools to do so.
It’s gratifying to know you can build programs that, until now, you’ve never fully understood. Plus, projects are essential to the learning and job-seeking process.
Coding can help you better understand the world around you.
Learning even just the basics of computer programming will help you understand the components of the growing technology landscape.
You’ll gain an entirely new perspective on the technologies in your life and an appreciation for how it all comes together.
Coding is fun.
It’s cheesy but true — for me, learning to code is a rewarding and enjoyable experience. Once I had the basics down and started on my own projects, the process felt less like learning and more like leisure. After all, if you’re not enjoying it, why pursue it as a career?
How to Start Coding
- Figure out why you want to learn to code.
- Choose which coding language you want to learn first.
- Take online courses.
- Watch video tutorials.
- Read books and ebooks.
- Use tools that make learning to code easier.
- Check out how other people code.
- Complete coding projects.
- Find a mentor and a community.
- Consider enrolling in a coding boot camp.
Thanks to the internet, there’s never been a better time to learn to code. But the sheer volume of options can stall some new coders before they even begin.
1. Figure out why you want to learn to code.
It’s tempting to jump right in when you start coding. But if you don’t have an end goal, you may become frustrated and stop learning before you get to the fun.
So, before you start studying, I want you to think about why you want to know how to code. Think about the projects you want to complete, why this skill excites you, and what resources you have available.
For example, say you want to become a developer to earn more income for your family. Do you know where you want to work and what projects they need developers for? Are you ready to put in the time to learn to code, learn the right programming languages, and build projects that show you have what it takes?
Setting a broad goal like that is important but can be overwhelming. Instead, I suggest that you start with smaller, more specific goals. For example, say you want to build a mobile app for your friend who is training for a half-marathon one year from now.
This goal will:
- Help you build the skills you need.
- Give you a sense of which programming language to start with.
- Set a deadline so you can manage your time while learning.
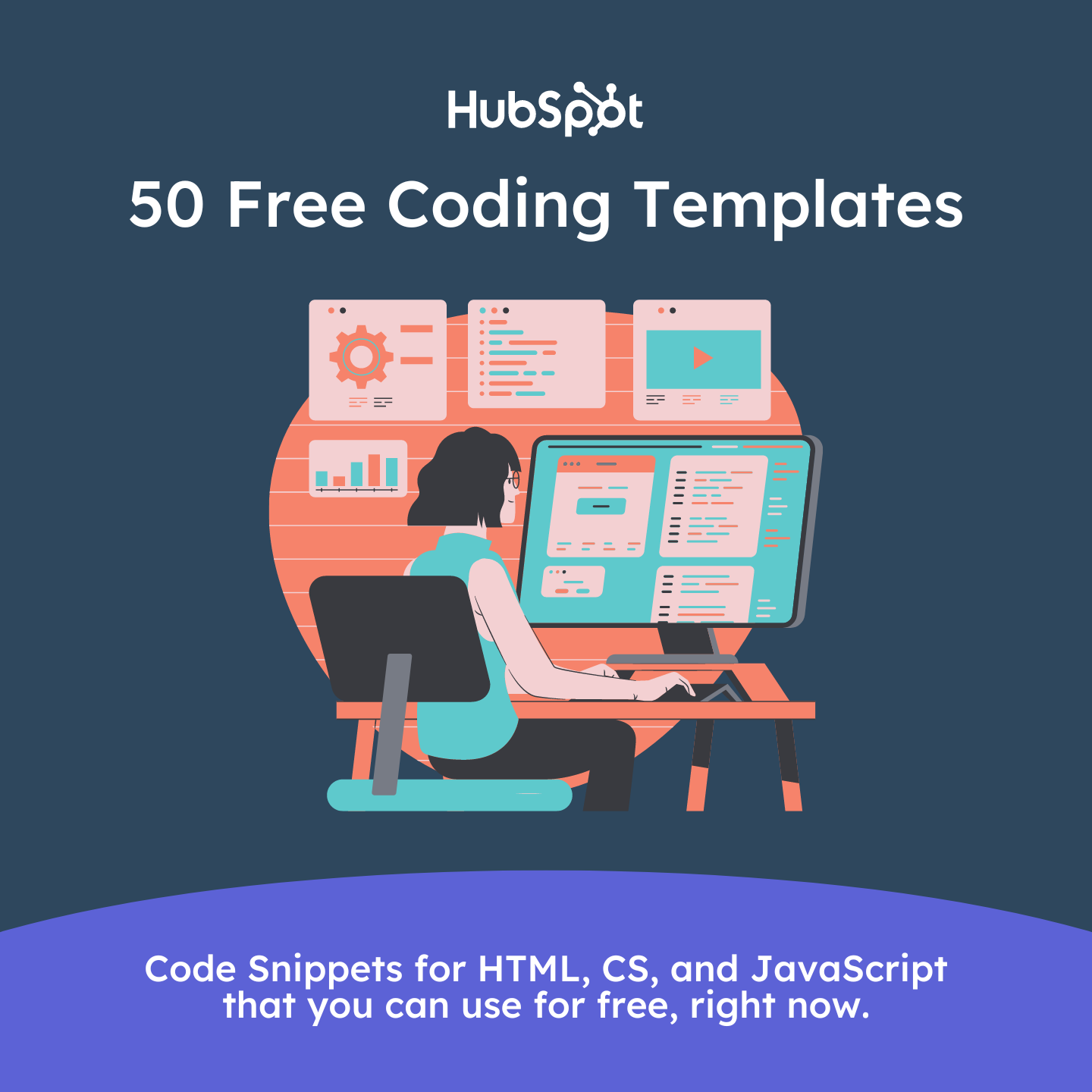
50 Free Coding Templates
Free code snippet templates for HTML, CSS, and JavaScript -- Plus access to GitHub.
- Navigation Menus & Breadcrumbs Templates
- Button Transition Templates
- CSS Effects Templates
- And more!
Download Free
All fields are required.
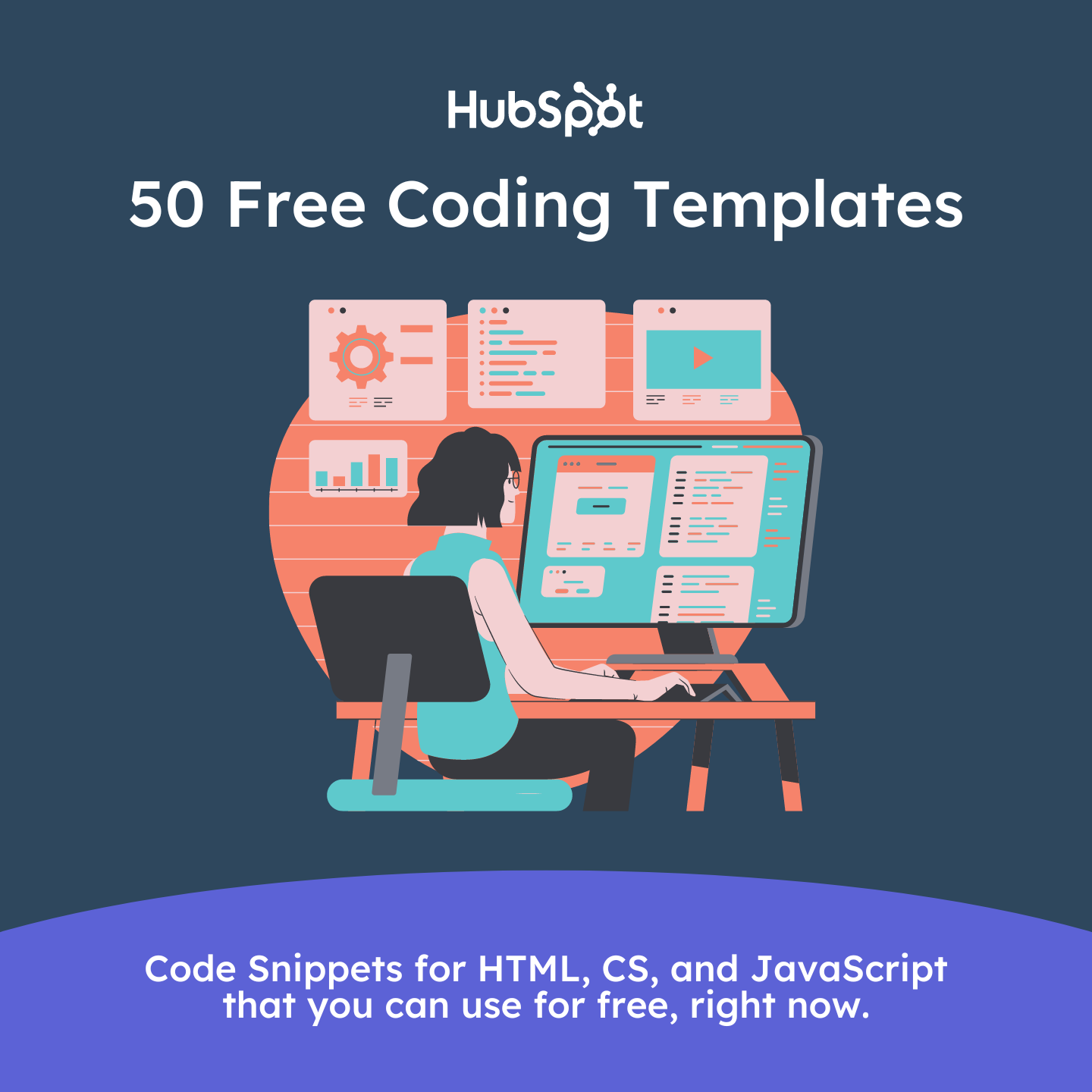
2. Choose which coding language you want to learn first.
If you’re trying to figure out which programming language to start with, I want you to think about your long-term goals. Are you coding for fun or to advance at work? Do you have a flexible timeline, or do you need to finish a project in a hurry?
When I was in my beginning stages, I started with a language that doesn’t use data structures or algorithms. So, for me, HTML or CSS were great places to start. But languages like Java and Python are also great for beginners, and they have a wide range of applications.
It can sometimes take months to learn a programming language, so take some time to make this decision to make sure that your time is well spent.
3. Take online courses.
Learning to code no longer requires a classroom setting. Today, there are thousands of online programming courses that cover everything from basic HTML to data structures to complex algorithms.
Your first course should introduce the basics of a language and contain interactive modules and assignments to guide your learning.
For me, courses create a structure for learning, which is essential as concepts in computer science build on each other. An organized course keeps everything digestible and ensures you’re learning things in the correct order.
Popular free course providers include:
- freeCodeCamp
- W3Schools
- Scaler Topics
- Harvard’s acclaimed Introduction to Computer Science course on edX and the CS50 YouTube channel
These options are great for determining early on whether you’re ready to invest the time to learn a particular language. Plenty of paid courses are available for a fraction of what in-person classes will cost you.
These choices cover a wide range of beginner, intermediate, and advanced CS topics:
Some paid services offer free courses or trials if you want to get a feel for their teaching before you commit.
4. Watch video tutorials.
You’ve probably watched a YouTube tutorial or two — why not do the same for coding?
While online courses are your best bet for hands-on experience, online videos can supplement your learning and occupy your curiosity. My personal favorites include:
- Crash Course Computer Science
- Tom Scott’s The Basics
Both cover broader topics in computing.
5. Read books and ebooks.
Prefer an old-school approach? Pick up a book on your beginner language of choice. Books will introduce you to fundamental concepts and inform your coding.
Learn HTML & CSS with this Free Ebook
Here are some established texts that I recommend for each beginner language.
- HTML/CSS: The Beginner's Guide to HTML and CSS for Marketers
- JavaScript: Eloquent JavaScript: A Modern Introduction to Programming
- Python: Python Crash Course
- C: C Programming Absolute Beginner's Guide
- C++: C++ Primer
- C#: C# 8.0 and .NET Core 3.0 – Modern cross-platform development: Build applications with C#
- Java: Effective Java
- PHP: Head First PHP & MySQL
- Ruby: The Well-Grounded Rubyist
- SQL: SQL in 10 minutes, Sams Teach Yourself
- Swift: Swift Programming: The Big Nerd Ranch Guide
6. Use tools that make learning to code easier.
While it’s great to know that you don’t need any special tools to write code, there are tools that can help.
Code Editor
Text editors include features to make coding easier like color coding, auto-complete, find-and-replace, and dark mode.
In my experience, most professionals use a code editor. This tool can help you write clean code faster. Code editors are like text editors, but they have extra features that help you manage and edit your code like:
- Syntax highlighting
- Code lookup
- Built-in terminal environment
Notepad++, Sublime Text, and Emacs are popular editors for beginners. VS Code is also a great option.
Console
You’ll also want to get to know your computer a little better. When you start coding you should learn how to navigate the console (also called a terminal). This is the text-based interface for your operating system.
The console lets you find files and execute commands on them more quickly than the standard graphical user interface (GUI). Familiarize yourself with how to use it, including the basic UNIX commands, since you’ll be doing things that aren’t possible in the GUI.
Project Management
Many projects start simple but get more complicated as the project goes on. Using a project management tool, you can share files, update timelines, and break out separate parts of coding projects. This makes it easier to keep track of side projects and extra files while sticking to your timeline.
Troubleshooting
As you work on your coding projects, you may run into bugs, roadblocks, and other challenges. This can be super frustrating. Rubber Duck Debugging may sound silly, but it can help you simplify your problem and find useful solutions.
7. Check out how other people code.
It’s easier for some people to edit someone else’s work than to create from a blank page. If this is you, a great way to learn how to code is to go through someone else’s code.
Checking out other programmers' code can inspire you and push your coding skills further. This approach will help you:
- Better understand your own code.
- Understand new code faster.
- Give you tangible examples of quality coding.
Start with a program or code file you like. If you’re unsure what code to start with, GitHub and searchcode are great starting points. Try to start with peer-reviewed code or open-source projects if you can.
If you know what the code does, choose one small section and work backward. This will help you understand the function of every line of code. It’s also a good idea to read the documentation. You can then see the ideas behind the code you’re reviewing.
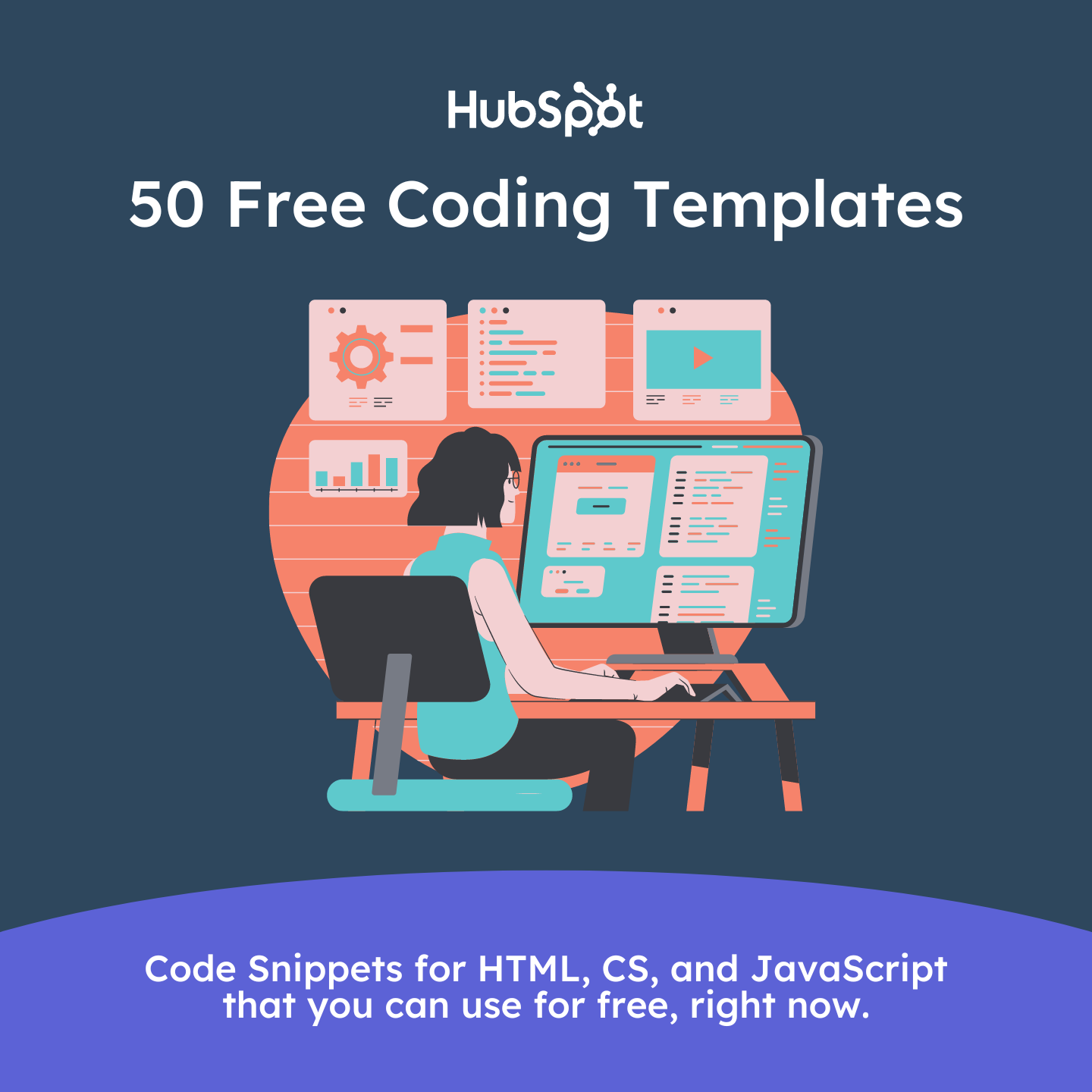
50 Free Coding Templates
Free code snippet templates for HTML, CSS, and JavaScript -- Plus access to GitHub.
- Navigation Menus & Breadcrumbs Templates
- Button Transition Templates
- CSS Effects Templates
- And more!
Download Free
All fields are required.
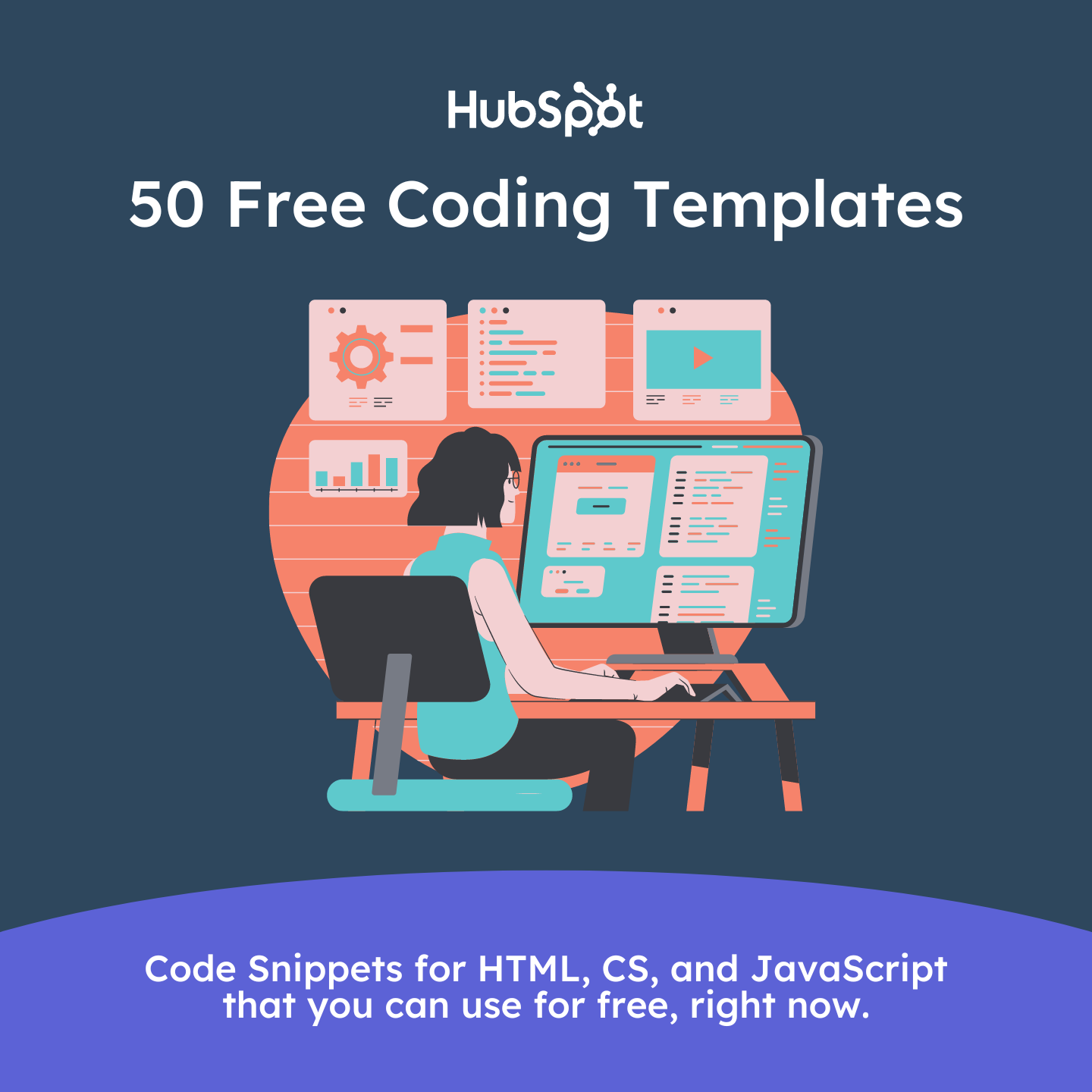
8. Complete coding projects.
You learn programming by doing — there’s no way around it. You can read up on all the concepts and syntax necessary to write functional code, but the ideas won’t fully materialize in your mind unless you put what you learn into practice. That’s where projects come in.
A project is any program (or website) built with your language of choice. When starting, keep projects short-term.
If you’re taking a course, you may be assigned projects designed to solidify a concept. There are also loads of beginner programming projects you can try independently. Some classic projects include:
- A time converter, in which the user submits a number of seconds, and your program gives the equivalent in hours, minutes, days, etc.
- A random number generator, which produces a random number between two values specified by the user.
- A calculator, in which the user specifies their inputs and mathematical operation, and your program gives an output.
- An address book, in which users can submit contact names, then search for contacts stored in your program.
- An alphabetizer, in which the user offers a list of words, and your program sorts them in alphabetical order.
- A hangman game, in which the user tries to guess a hidden word by inputting letters, and your game gives feedback for correct or incorrect guesses. When the user guesses all the letters to your word, they win.
A quick Google search will reveal even more mini-challenges that require applying your skills to real-world problems.
Benefits of Coding Projects
On top of practicing concepts, projects offer two more benefits to your learning.
First, they’ll keep you motivated. Projects help solidify the “why” behind your coding and set clear, tangible benchmarks for your progress. Each completed project means one more skill under your belt. As I was learning, this was very encouraging.
Second, coding projects, especially long-term ones, give you something to show for your work. It’s one thing to put “Python” on your resume — it’s another to show you built an entire website or application from scratch.
Projects are a must for entry-level programming jobs, as they prove competency in a given language.
How to Stay Motivated While You’re Coding
When embarking on longer-term projects, consider things you’re willing to invest time in. Whether it’s a personal website, a mobile application, or a desktop tool, you’ll hit speed bumps along the way. Choosing a project you truly care about ensures you follow through.
Another great way to stay engaged with coding projects is by freelancing. You don’t need to be an expert programmer to build a useful tool for someone. Reach out to a friend, family member, or local business in need of a tool or website — it’s a win-win.
9. Find a mentor and a community.
Having a friend or an online community to guide you can be invaluable to your learning.
First, I recommend finding a mentor. As you progress, you’ll probably encounter issues that, no matter how hard you try, you just can’t crack. This is where mentors can help.
A mentor doesn’t need to be an actual teacher — they can be anyone knowledgeable in your language. They should be able to explain difficult concepts and point you to solutions. An experienced mentor can help you follow coding practices not covered in tutorials and offer advice for navigating a career in tech.
You may also want to join a community. Look for local groups, networking events and meetups in your area, and hackathons where you can make in-person connections with other programmers.
Online developer communities are also a rich resource for beginners.
Be sure to check out:
- Stack Overflow, a forum site for programming questions and discussion.
- GitHub, a code repository for open-source projects with an active developer community.
- Women Who Code, a nonprofit that organizes events, communities, and job postings for women pursuing careers in technology.
- r/learnprogramming, a subreddit (a microsite on Reddit.com) for beginner coders.
10. Consider enrolling in a coding boot camp.
A coding boot camp is a short-term training program that packs a comprehensive coding curriculum into several months. These programs are fast-paced, immersive, and a launchpad for a development career.
Coding boot camps are intensive and expensive — not the kind of thing to dive into without any coding experience.
These programs are primarily for beginners who are set on a career in development and are ready to commit time, energy, and money to get the necessary skills quickly.
While grads tend to find employment in the tech industry, understand that this isn’t a guaranteed outcome. You’ll set aside a decent chunk of your year and savings for such a pursuit. Still, it’s difficult to top an in-person learning environment surrounded by peers and teachers as motivated as you are.
Coding vs. Programming
The terms coding and programming are often used interchangeably, but they don’t always mean the same thing. Both coding and programming mean writing instructions for a computer. But programming might also include algorithms and data structures. Generally, the term programming describes more advanced projects.
While you can code with just a computer and some time, some programming might need specialized software tools. Programming projects are usually bigger and more complex. They may need project management and a more solid knowledge base.
Programming Languages
Coding requires knowledge of at least one coding language, a set of syntax and rules that computers can understand. There are hundreds of coding languages, each one unique in its purpose and what it can do.
When I first stepped into the world of coding, the array of programming languages seemed overwhelming. I began with HTML and CSS, and it was a transformative experience. Diving into HTML opened my eyes to the fundamental structure of web pages, while CSS unlocked the secrets of styling and design. These languages were the perfect starting point for a newbie like me, offering a hands-on introduction to the world of web development.
Programming languages give you a structure for the instructions you’re writing. This language looks like English, but not exactly. Programmers call the terms and grammar in a programming language syntax.
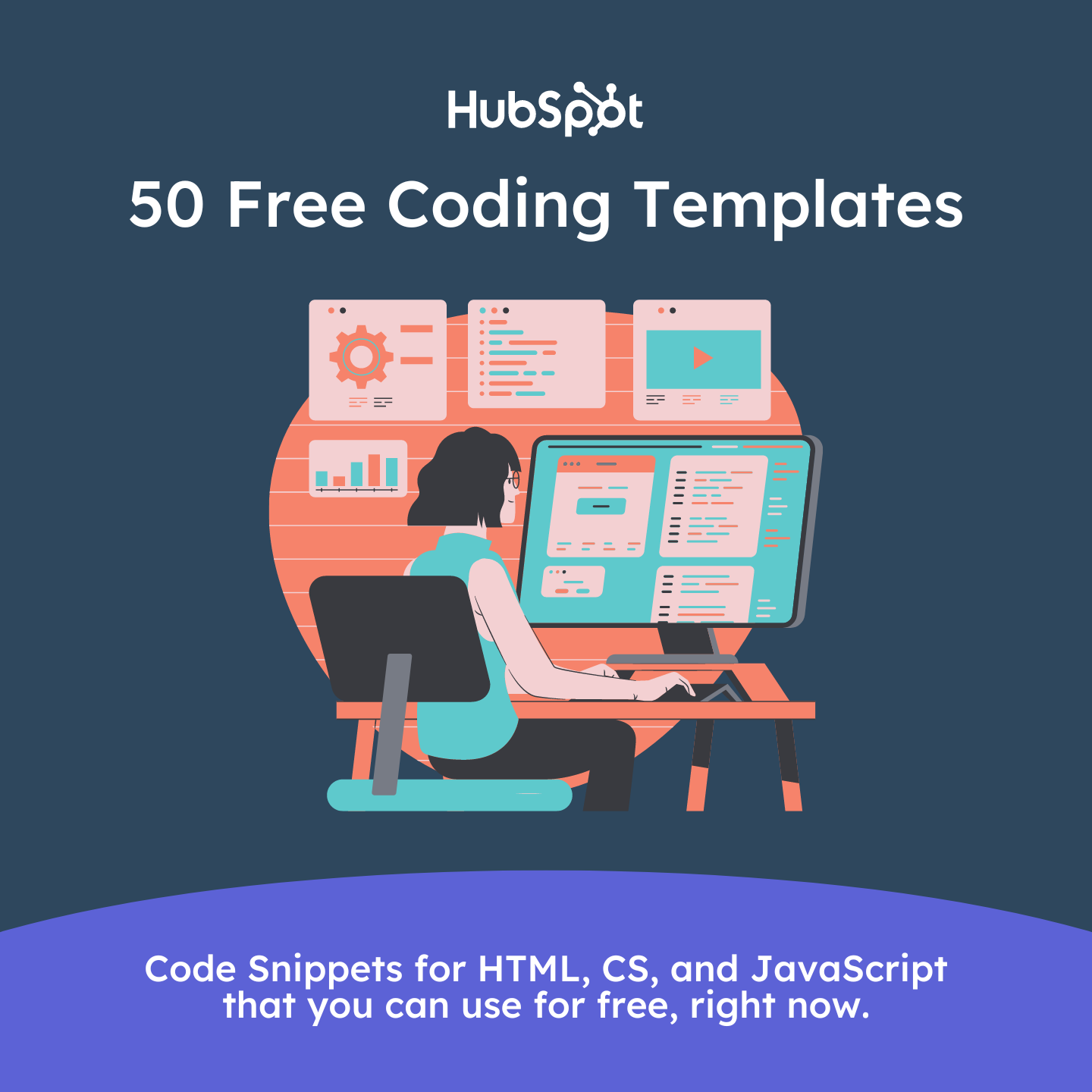
50 Free Coding Templates
Free code snippet templates for HTML, CSS, and JavaScript -- Plus access to GitHub.
- Navigation Menus & Breadcrumbs Templates
- Button Transition Templates
- CSS Effects Templates
- And more!
Download Free
All fields are required.
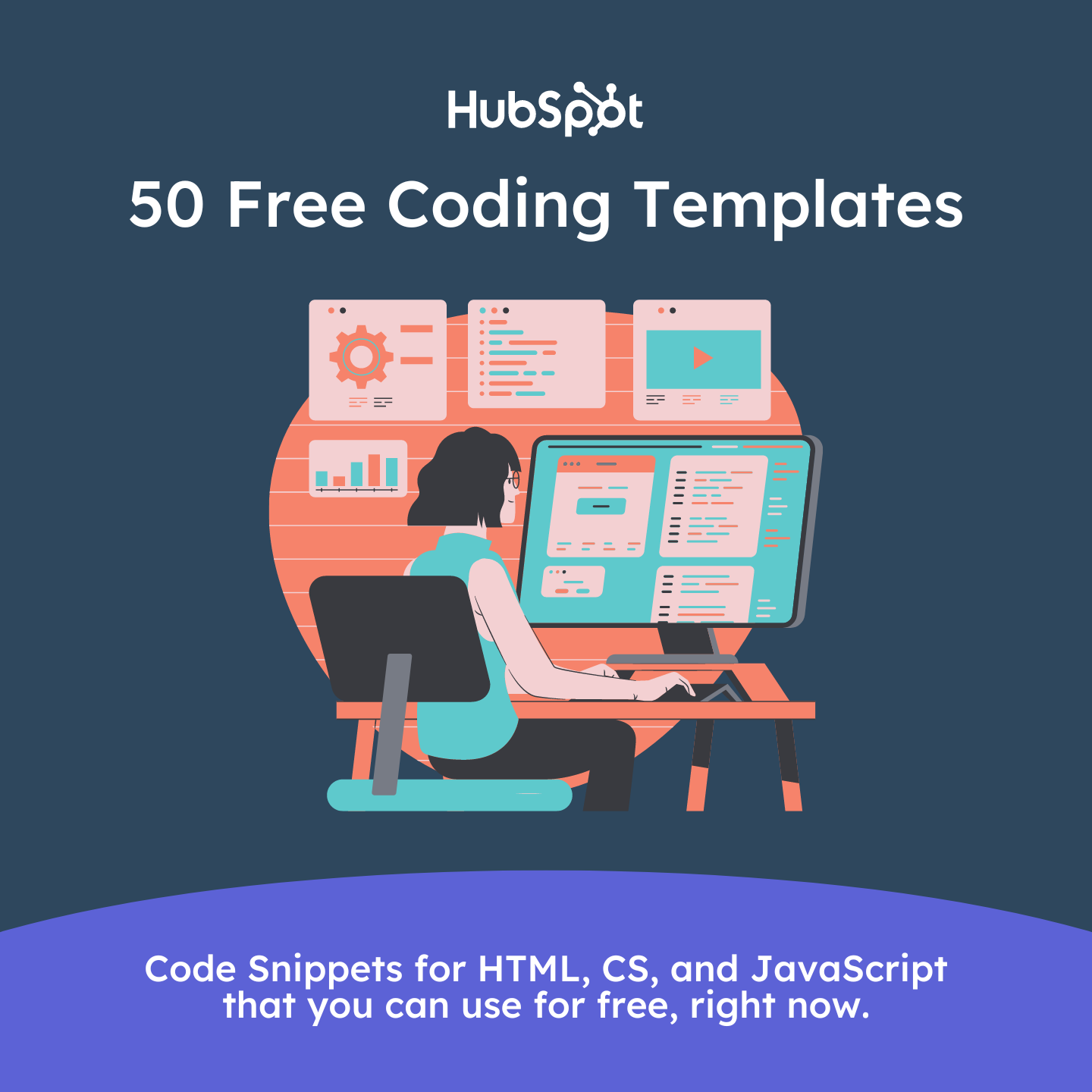
Low-level languages like Assembly or Machine are easier for machines to read than humans, so they can be tough to learn. That said, a middle-level language like C++ is useful to know if you want to write:
- Operating systems
- Database systems
- Image or video processing software
High-level languages are usually easier for beginners. Some focus more on structure, while others are more interactive and can perform more complex functions.
Below are some of the best languages for beginners. Try to get comfortable with just one language that aligns with your goals, then explore others if you’d like.
Don’t worry about choosing the wrong one, though. These languages share underlying concepts, so you can start with one and switch to another.
HTML
Hypertext Markup Language, or HTML, is the foundation of the internet — it’s used to set the content of web pages. When you load a web page, you see an HTML document rendered by your browser.
If you’re unsure whether coding is your thing, HTML is the easiest language to sample.
This is because HTML isn’t technically a programming language — it doesn’t execute scripts, and you can’t build functional programs with it. Still, HTML is everywhere online, so if you want to understand the internet, you’ll first need to understand HTML.
Best for: Beginners eager to step into web development and understand the basics of web page creation.
CSS
You might be less familiar with HTML’s sibling language, CSS. HTML handles what content appears on a web page but doesn’t affect how that content looks.
This is where Cascading Style Sheets, or CSS, comes in. The CSS language handles the styling of HTML — it sets features like colors, sizing, fonts, and even entire page layouts. CSS is also not a programming language. It’s a set of rules applied to HTML.
HTML and CSS are almost always used together, so I recommended learning both. Otherwise, your web pages will look rather plain.
If you’re starting from square one, dive into HTML and CSS.
HTML and CSS are easy to learn largely because they don’t require you to think through the computational logic of programming languages.
Learning HTML and CSS can also feel less abstract than other languages since you see the results of your code quickly — simply create a .html file and open it in your browser. Or, open an existing website and use your inspect tool to peek at the underlying code.
But, if you want your web pages to do things, you’ll need...
JavaScript
JavaScript is a programming language that turns static web pages into dynamic ones. It enables page elements to move, react to user actions like clicks, and handle any operation beyond simply existing on the page.
If you’re interested in web development and already have a feel for HTML and CSS, JavaScript is the next step.
Together, these three languages make up most of your web content. Plus, JavaScript code can be easily tested in your browser.
Pro Tip: Focus on mastering DOM manipulation and asynchronous programming in JavaScript to really bring your web pages to life.
Python
Python is a popular programming language for beginners because of its user-friendly syntax and versatility.
Much of Python code reads like English, which helps beginners learn basic concepts like functions.
Python also has many code libraries. These are groups of pre-built functions that you can plug into your code instead of writing the functions out yourself. With Python, you can build many different types of programs. Many introductory courses also base their projects on this language.
What I like: Its extensive libraries and community support make Python a great choice for both beginners and experienced programmers.
C/C++
C is another popular choice for introductory courses. It’s wordier than Python and often requires beginners to write more code to achieve the same things. This is more work but useful for understanding abstract concepts. With C, you’ll learn skills that can be applied to other, more succinct languages.
C++ is a successor to C. C++ syntax is similar to C with the addition of objects, a powerful variable type that makes programming sophisticated applications easier.
I recommend beginning with C, though, since there are fewer concepts to learn, all of which can be mapped to C++. It will also teach you the basics of function-oriented programming.
Best for: Those intrigued by system-level programming, game development, or applications where performance is critical.
C#
C#, also called C-sharp, is popular for developing video games, mobile and desktop apps, and enterprise software. C# shares a common source with C++, but while C++ and C have many overlaps, C# is more like Java.
C# is easy to learn, and there are many ways to use it. If you plan to use both C++ and C#, you may want to learn C++ first. This will make it easier to understand C# and can help speed up the learning process.
Java
Java (not to be confused with JavaScript) is a general-purpose object-oriented programming language. Like Python, Java’s syntax is easy to read and understand by human programmers — often, complex tasks can be handled by one command.
Java is popularly implemented in Android mobile applications. It’s another great base language with principles that can be intuitively applied to learning other languages.
Pro Tip: Pay close attention to understanding object-oriented programming when tackling Java, as it forms the core of its functionality.
HyperText Preprocessor (PHP)
PHP is a server-side, open-source programming language for developing web applications. It makes it easy to add dynamic information, like updated news stories, to websites.
You can also embed this language into HTML, which makes it easy to add functions to your website without needing external files. PHP is also great for database access, making it simple to access and store data.
Best for: Beginners who want to delve into the server-side aspect of web development.
Ruby
Ruby is a simple language that’s useful for creating automation tools, desktop applications, and rapid prototypes. There is also a popular framework for Ruby, often called Ruby on Rails.
Ruby is another open-source server-side programming language. Its framework also offers default structures for web pages, web services, and databases. This makes it a useful tool for developing web applications.
While this language is easy to read and write, it may not be the best choice for your first programming language. This is because it’s also quite flexible, so it easily accepts changes.
Flexibility is great when you’re first learning how to make a coding project go. But it can impact changes to your code as you maintain the project over time.
What I like: Ruby is an excellent choice for rapid application development due to its 'convention over configuration' philosophy.
SQL
SQL is another useful language for beginners. You can use this language to update, store, and retrieve data from a database. It’s also the standard language for database management systems, according to the American National Standards Institute.
It’s different from the other programming languages since it’s technically a Query Language, so you won’t be building web pages with it.
It can take some time to learn SQL if you don’t already have some understanding of programming. That said, this language is popular in tech and with data professionals, so it has a lot of relevance in the workplace.
What I like: SQL is needed for projects that involves data storage and retrieval, making it a must-learn for web developers.
Swift
If you’re learning to code to create projects for Apple devices, Swift is a good language to start with. Designers at Apple created this language with beginners in mind. And if your primary goal is to develop mobile apps for iOS devices, this should be your language of choice.
While programmers have created most products since 2014 with Swift, you may also want to learn Objective-C.
Developers created this language in the 1980s. It’s what their team built most iOS tools with. It uses some C syntax, so if you’ve learned C or C++ already, you may want to start with Objective-C as you start learning.
Best for: Anyone aspiring to develop apps for Apple's platforms. Swift's modern features and safety make it a go-to for iOS app development.
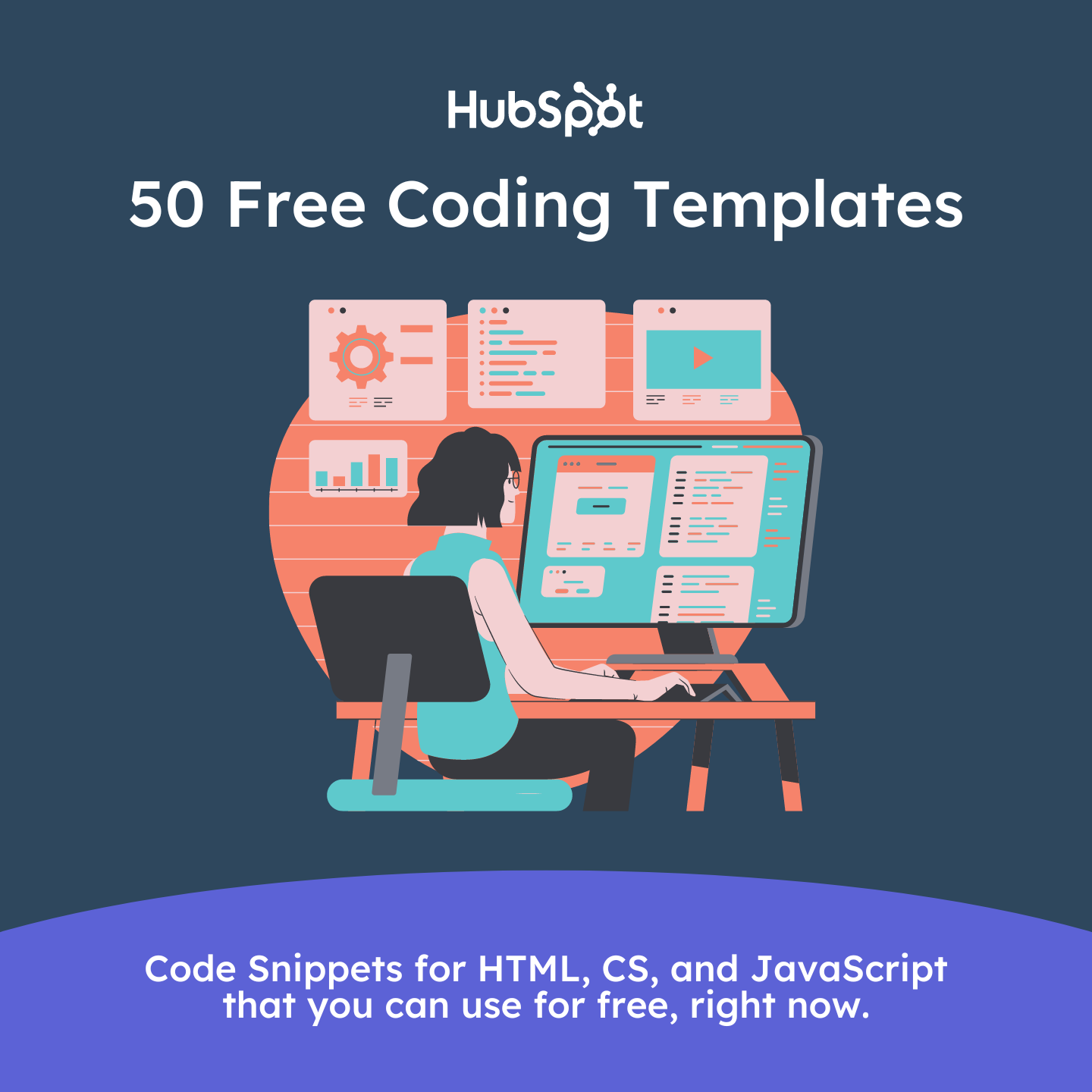
50 Free Coding Templates
Free code snippet templates for HTML, CSS, and JavaScript -- Plus access to GitHub.
- Navigation Menus & Breadcrumbs Templates
- Button Transition Templates
- CSS Effects Templates
- And more!
Download Free
All fields are required.
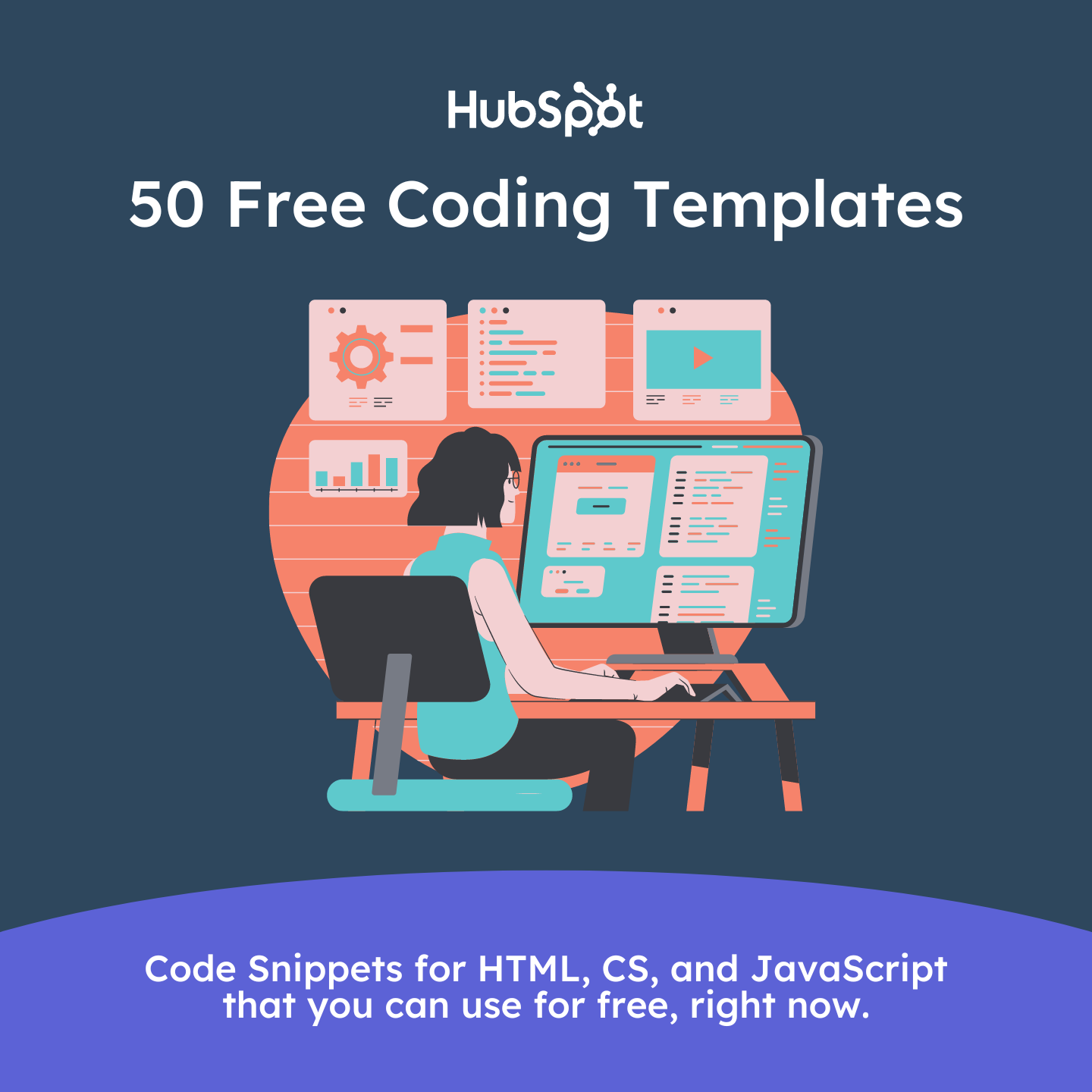
Tips for Coding for Beginners
As someone who ventured into the coding world not too long ago, I understand the mixed feelings of excitement and apprehension that come with learning to code. There are many ways to approach the practice of coding, and some methods and languages will work better for you than others.
No matter what or how you learn, however, remember these beginner tips:
1. Be patient.
At the top of this post, I said anyone can learn to code. However, that doesn’t mean it’s easy.
Coding requires you to approach problems in ways you haven’t before. Certain topics may seem nonsensical to you, yet central to the language you’re learning. Problems that might seem easy at first will become far more complex to implement than you expected. You might spend hours hacking away at an assignment without seeing results.
And we can’t forget debugging. You’ll quickly learn that computers are nit-picky and will only do exactly what you tell them. Tiny typos like a missing semicolon or incorrect operator will break your entire program, and you must track down the culprit.
Even coding for beginners isn’t easy.
All of this can be uncomfortable and discouraging, and that’s okay. Do yourself a favor and progress slowly and steadily, giving yourself time to let everything soak in.
The best programmers were once where you are, and everyone has to learn the same things to start. Go easy on yourself, stick to the goals you’ve set, take breaks, and you’ll be fine.
2. Get comfortable with the fundamentals.
The early days of learning a programming language are all about the essentials. You might start by learning binary, data types, and how to print to the console. From there, you’ll explore topics like:
- Variables.
- Functions.
- Conditional logic.
- Arrays.
- Objects.
It’s essential that you fully grasp each of these concepts before advancing. This is because everything you learn in computer science builds on topics that come before.
If something doesn’t make sense, keep reviewing it until it does. And don’t assume it will make sense later in the context of future lessons. If you’re learning from a tutorial, be sure you understand what each activity is meant to teach you.
Complete all exercises to experience first-hand how each topic applies to coding. And stay patient — you can’t embark on an ambitious project until you grasp the fundamentals.
3. Write clean code from the start.
Here’s something an online course may not teach you: On top of learning how to write your code, you should also practice writing it well.
What does this mean? For any given computation, there will be more than one way to program it. You should always strive to write it in the most concise and humanly readable way that you can.
Developers usually work in teams, so others will be reading your code often. If it’s hard to decipher, fellow developers won’t look forward to working with you.
Even if you decide to freelance, writing clean code ensures you’ll understand your own code. It’s better to build the habit of clean coding now, as it will save you hours trying to decipher your work after you haven’t looked at it in months.
Why is clean code important?
You might be wondering why you need to learn clean code at this point. You’re a beginner, so shouldn’t writing functional programs be the main goal?
Well, yes. This is about establishing good habits early. If you put in the extra work now, you’ll save yourself (and others) some sanity down the road.
A good way to achieve this is by keeping your lines and your functions short. I recommend limiting each line of code to 80 characters max and each function to no more than 15 lines.
While limiting at first, these rules will train you to favor efficient code over the first idea that occurs to you.
Also, make a habit of commenting. Comments are segments of code that aren’t processed by the computer, so you can write whatever you want inside them.
Programmers use comments to clarify the purpose of their code. Learn how comments work in your language and, at the very least, leave comments at the top of your functions explaining the job of each one.
4. Search is your friend.
There’s no shame in using Google to find the solutions to your coding problems. In fact, professional developers do it all the time.
If you’re struggling, someone’s probably been in the same situation and dropped a question to a forum. You’d be surprised at how many solutions you’ll find with super-specific queries.
Plus, it’s quite satisfying to close 20 tabs of Stack Exchange after finally fixing a stubborn bug.
Our Favorite Coding Resources
Coursera
Coursera is an excellent resource in general. They have many programming and coding courses from Google, IBM, as well as various colleges and universities. You can get many paid courses through their monthly subscription, though they have free courses as well.
Coursera also offers financial aid on a per-course basis. If approved, you can get free access to the paid content. It’s worth mentioning that the access granted is only for a limited time through this method. If you don’t touch a course for several months, you will likely lose access.
Another nice thing about Coursera is that it’s treated like a classroom environment. This means you have an instructor for each course that you can ask for help, as well as other students that are currently taking the course. You can also get professional certifications that can help advance or change your career.
MDN Web Docs
If you’re doing web development, MDN Web Docs is the gold standard for documentation. Everything related to HTML, CSS, and JavaScript lives here. This site belongs to the Mozilla Foundation, which you may know for their popular web browser, Firefox.
MDN Web Docs also has many tutorials ranging from complete beginners to the advanced level.
Codecademy
Codecademy is great for diving right in and writing code almost immediately. It gives you small digestible tasks to work on, as well as a sandbox to work in. They have courses for just about every language you can imagine.
Most of their content is free, though they have paid guided projects and career paths you can opt for, as well as career services and professional certifications.
The Odin Project
The Odin Project is an open-source, full-stack curriculum for web development. They have two paths, one using Ruby on Rails and the other using JavaScript (with the React framework).
The amount of information available is a bit daunting, but the best part about The Odin Project is that you will end up with many real projects to hone your skills and show off to potential employers by the end of it.
W3Schools
W3schools has a ton of tutorials that range from typical programming languages, to frameworks and other languages like SQL and HTML. They also have plenty of example code you can play with and reference during your coding adventures.
The best part is that everything is free. They do have some paid certifications, though all of the actual information about the languages is readily available.
Stack Overflow
Stack Overflow is an incredibly popular and useful site for developers. People ask questions about coding and get feedback. Then, the answers are voted on to determine the best solution. Even when you’re no longer a beginner, you will likely keep returning here to find answers to various questions or issues.
The community is very active, and the public platform is free. You just need to create an account to interact with others.
Coding is more than just the code.
To finish up, I want to share one more valuable piece of advice from my first computer science class. From a beginner’s perspective, it may seem like learning to code means learning how to write code.
This makes sense: When we imagine a computer programmer, we see someone writing out code on a computer — it’s called “coding” after all.
Once you begin, though, you’ll learn that this isn’t the whole story. You’ll spend a lot more time thinking about what to write than actually writing it.
Coding is problem-solving.
This is because coding is more about solving problems than knowing the syntax. Learning to code is learning to think like computers do, deconstruct problems into their components, and address them with the tools you’re given.
So, yes, you’ll learn to write some impressive code and eventually build amazing things. But first, you’ll develop the thinking skills that can get you there.
In my years of coding, this approach to problem-solving has changed how I tackle technical challenges and how I approach problems in general. I hope you’ll experience the same. Keep going. You got this.
Editor's note: This post was originally published in December 2020 and has been updated for comprehensiveness.
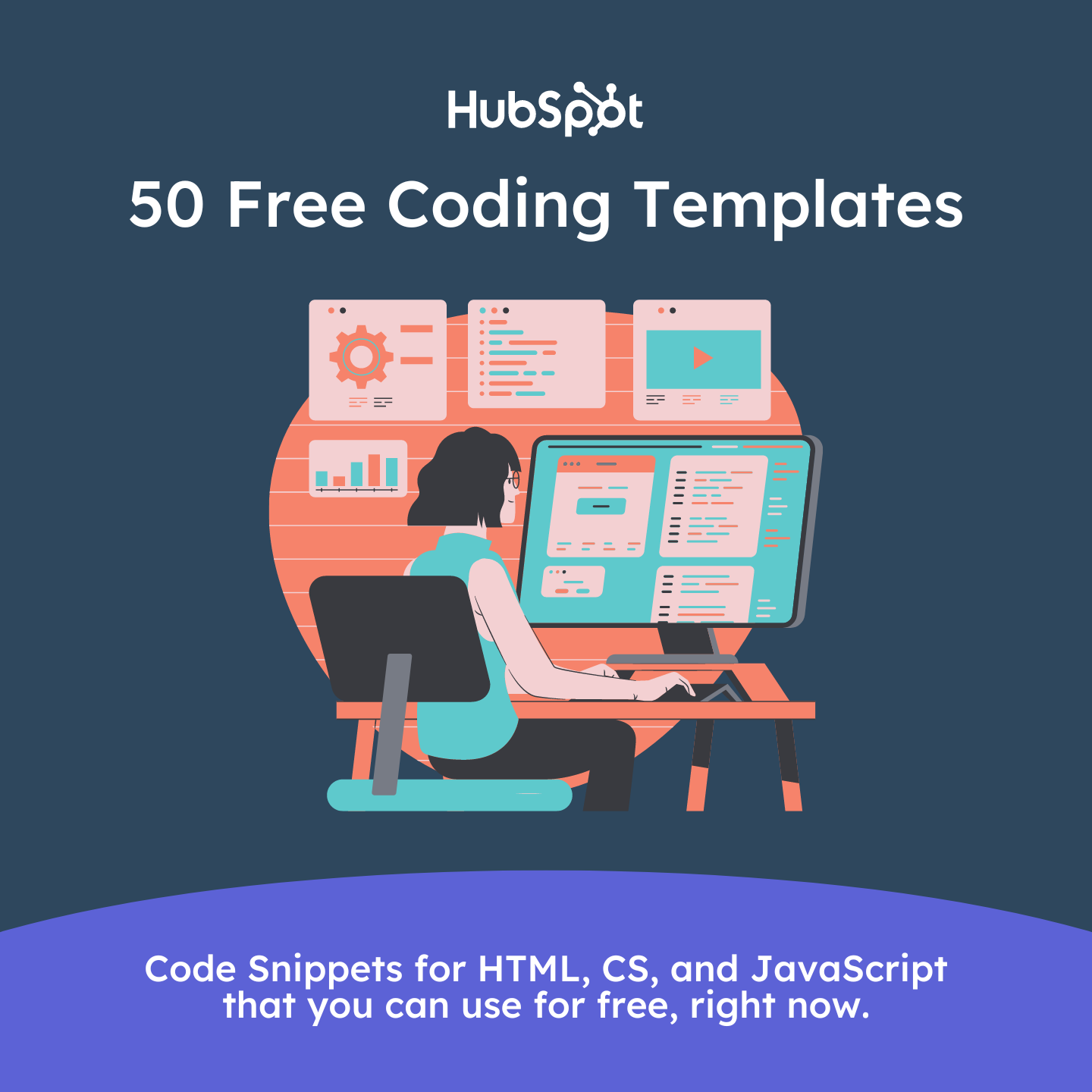
50 Free Coding Templates
Free code snippet templates for HTML, CSS, and JavaScript -- Plus access to GitHub.
- Navigation Menus & Breadcrumbs Templates
- Button Transition Templates
- CSS Effects Templates
- And more!
Download Free
All fields are required.
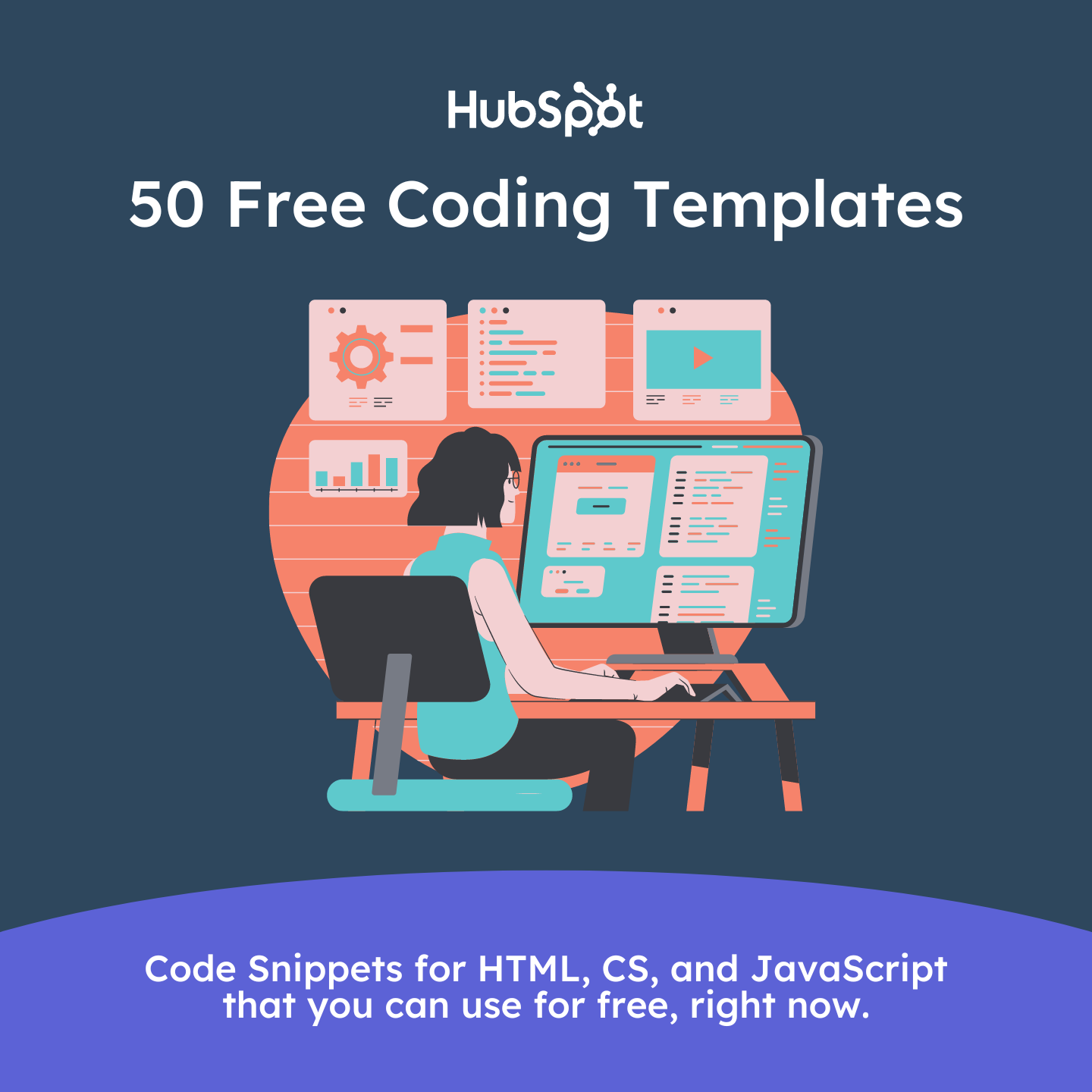