Python is a robust language with an ever-growing list of libraries that extend its capabilities. Among the libraries offered by Python include argparse, multiprocessing, and subprocess, just to name a few.
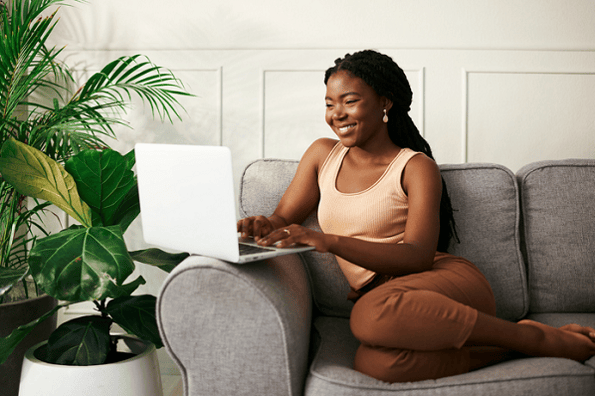
This post will go over the pandas dataframes and what they are. We will go over the concepts behind a dataframe, the syntax, and how to create a dataframe object. You will also see some examples of creating a dataframe object with different data types.
Without further ado, let's dive into the fundamentals of pandas dataframe creation.
What is a pandas dataframe?
A pandas dataframe is a table of indexed data containing both rows and columns of information. The purpose of a dataframe is to help visualize data in a more manageable way for developers. Dataframes are used in many industries, but none more than big-data management as it simplifies the process of working with large datasets.
The pandas library was created in 2008 by Wes McKinney, the necessity for a quantitative data analysis tool for working with data. Since its creation, it has quickly grown into one of the most popular data management tools available through the Python programming libraries.
Now that we’ve talked about the history of pandas, let’s look at how you can use it to create and manage data sets. We will focus on smaller subsets of data to illuminate how you can use it on larger applications for your data needs.
Creating a Pandas Dataframe
In pandas, the need for clear visualization is an inherent part of its use. Data visualization helps speed up development and makes working with data easier by eliminating the pain of unstructured data. However, even well-structured data can become daunting to work with without tables to help visualize it. The following video covers some examples of how to create dataframe tables in pandas.
Enter pandas, with its tables and easy-to-use visualization, managing data has become a much more satisfying task. Moreover, the steps needed to create a table are easy to complete. Like anything in Python, you will need to start by importing the pandas library into the file you will be using for your pandas code.
# Import pandas library
import pandas as pd
The next example shows how to create a pandas dataframe using a list of lists.
Pandas Dataframe: List of lists
# initialize list of lists
data = [ ['Charlie', 46], ['Deandra', 46 ], ['Frank', 59] ]
# Create the pandas DataFrame
df = pd.DataFrame(data, columns = ['name', 'age'])
This code creates a dataframe table consisting of three sets of data, one for each of three people. The first line of code creates a data set made from a list of lists. The data sets contain two pieces of information for each entry: a name and an age. The second line of code creates a dataframe with two columns, one for names and one for ages. Each row in the data frame will consist of information about each entry.
This is useful and makes working with even small datasets simpler. But what if your data isn’t in the form of lists? Can dataframes be created with other data types? The short answer is yes; it can accept many different data types. Let's look at creating a dataframe table with a dict list.
Pandas Dataframe: Dictionary lists
Because dicts come with a key for each entry, the default behavior for dataframe objects is to use the key to assign the columns for the table. As a result, the structure of declaring a dataframe is cleaner.
data = {
'Name':[ 'Charlie', 'Deandra', 'Frank' ],
'Age':[ 46, 46, 59 ]
}
# Create DataFrame
df = pd.DataFrame(data)
The process isn’t much different. However, there is no need to name the columns explicitly with this data structure. As a result, this code creates the same table and structure as the previous code example.
Pandas Dataframe: Explicit Index Names
Finally, one of the most important things to know how to do with dataframe tables is to create explicit row indexes. This can be done both statically and or dynamically based on your needs.
# initialize data of lists.
data = {
'Name':[ 'Charlie', 'Deandra', 'Frank' ],
'Age':[ 46, 46, 59 ]
}
# Creates pandas DataFrame.
df = pd.DataFrame(data,
index =[ 'Bartender', 'Investor', 'Janitor' ])
In fact, with these examples, one could even use the names as row indexes making for a much more straightforward read of the data presented. To create dynamic indexes, you would simply pass in a list of index names. Then, if that list is dynamically generated based on user input, you can store that list in a variable and use that to declare the indexes.
id_names = [ 'Bartender', 'Investor', 'Janitor' ]
df = pd.DataFrame(data,
index = id_names)
This is useful since data typically changes, allowing the indexes to change and grow with your data.
Using Pandas Dataframes in Your Workflow
You have learned a lot about the basics of creating dataframes in this post. You have learned what they are, how they are used and have seen a couple of examples of dataframes with different data types.
With this information, start exploring dataframes further and learn more about how they work. Your next steps could include learning how to use other data types to populate your dataframes or practicing with larger, more complicated data sets.