Python is a programming language that comes equipped with a number of handy built-in functions that you can use to do all sorts of things with your website like altering text and displaying relevant user information.
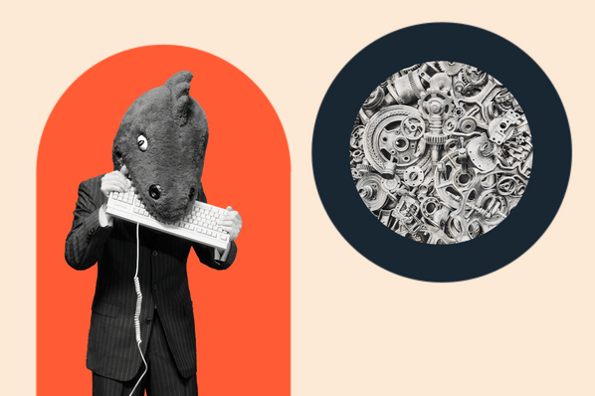
However, while there are plenty of Python functions that will enhance the functionality of your site, keeping track of them all is a lot easier said than done.
That's why we've put together this list of built-in Python functions so you can reference the most common ones during programming. This list will save you a bit of time in your work and help you become more familiar with popular Python functions.
Built-in Python Functions
Python has a number of functions that are built right into the programming language. These functions are commonly used and are available to developers at all times.
This table will help you navigate to specific Python functions. Use this list as well as this interactive code module to hone your programming skills, learn new syntax, and become a Python programming expert.
Please note that this module is updated by a third party, and therefore does not support a small percentage of the examples below.
1. All Function: all()
The all() function returns True if all items in an iterable are true, otherwise, it returns False.
All Function Example:
mylist = [True, True, True]
x = all(mylist)
Output:
True
2. Any Function: any()
The any() function returns True if any item in the iterable is true, otherwise, it returns False.
Any Function Example:
mylist = [False, True, False]
x = any(mylist)
Output:
True
3. Default Parameter Value
The Python default parameter value is the value of a parameter that will be used if no argument is given when calling a Python function. This can be used to set a default value for a parameter.
Default Parameter Value Example:
def greeting(name="John"):
print("Hello " + name)
greeting()
Output:
“Hello John”
// This statement will print “Hello John” because it uses the python default parameter value for name.
How to Pass a Function as Parameter
Passing a function as a parameter allows us to pass a Python function as an argument to another Python function. It is used when we want the Python function to do something based on the result of another Python function.
Example:
def add_numbers(a, b):
return a + b
x= func(x, y)
print("The result is " + str(x))
def process_function(func, x, y):
process_function(add_numbers, 4, 5)
Output:
"The result is 4"
4. Call a Function: your_function_call()
To call a function in Python, use the function name followed by a parenthesis.
Example of Calling a Python Function:
def my_function():
print("Hello from a function")
my_function()
Output:
Hello from a function
5. Call Function: call()
The call() function is used to execute a Python function.
Call Function Example:
def my_func(x):
return print(x)
my_func("Hello")
Output:
'Hello'
6. Print Function: print()
The print() function prints your code's output to the screen.
Print Function Example:
x = 'hello'
print(x)
Output:
hello
7. Write Function: write()
The write() function inserts specified text into a given file.
Write Function Example:
with open("myfile.txt", "w") as file:
file.write("This is a new file")
Output:
// This statement will create a file named “myfile.txt” and write the text “This is a new file” into it.
8. Binary Function: bin()
The binary or bin() function returns the binary value of a specified number.
Binary Function Example:
x = 12
print(bin(x))
Output:
0b1100
9. Boolean Function: bool()
The boolean or bool() function evaluates to either True or False. It's commonly used to check the condition of a statement or expression.
Boolean Function Example:
x = 5
print(x == 5)
Output:
True
// This statement will evaluate to True because x is equal to 5.
10. Elif Function: elif[boolean]
The elif function in Python stands for “else if” and is used when adding conditional expressions after if or between an if else statement.
>> Learn more about the Python elif statement.
Elif Function Example:
x = 10
if x > 5:
print("x is greater than 5")
elif x == 10:
print("x is equal to 10")
Output:
“x is equal to 10”
// This statement will print “x is equal to 10” because the elif statement checks if the condition (x == 10) evaluates to true and prints out the corresponding statement.
11. Evaluate Function: eval()
The evaluate or eval() function evaluates an input as a Python expression.
Evaluate Function Example:
x = ‘print(12)’
eval(x)
Output:
12
12. Signature Function: signature()
The signature() function provides the details of a function without having to scroll through the code.
Signature Function Example:
def add_two_numbers(a, b):
result = a + b
return result
print(signature(add_two_numbers))
Output:
(a, b)
13. Len Function: len()
The len() function returns the number of items present within an object.
Len Function Example:
fruits = ["banana", "orange", "blueberry", "kiwi", "pear", "banana", "grapes"]
x = len(fruits)
print(x)
Output:
7
14. Object Function: object()
The object() function returns an empty object with no parameters.
Object Function Example:
your_value = object()
print(type(your_value))
print(dir(your_value))
Output:
<class 'object'>
['__class__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__\hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__']
15. Random Function: random()
The random() function is used to generate a pseudo-random number between 0 and 1. It takes no arguments and returns a randomly generated float between 0 and 1.
Random Function Example:
import random
r = random.random()
print(r)
Output:
// This statement will print a random float between 0 and 1.
16. Random Integer: randit()
The random integer or randit() function returns a random integer from a specified range of numbers.
Random Integer Function Example:
import random
print(random.randint(1, 8))
Output:
6
17. Integer Function: int()
The integer or int() function converts a string or decimal number into an integer.
Integer Function Example:
a = int(6.6667)
print(a)
Output:
6
18. Plot Function: plot()
The plot() function is used in Python to plot data points onto a graph. It takes two parameters which are the x and y values of the data points.
Plot Function Example:
import matplotlib.pyplot as plt
x = [-1, 0 ,1]
y = [-2, 0, 2]
plt.plot(x, y)
plt.title("Line Plot")
plt.show()
Output:
// This statement will print a line plot with the title “Line Plot” because it uses Python's plot() function to create a line plot from the given data.
19. Remainder Function: %
The remainder or % operator is used to find the remainder of a division operation.
Remainder Function Example:
print(5 % 2)
Output:
1
20. Count Function: count()
The count() method is a built-in function that returns the number of occurrences of a given element in an iterable.
Count Function Example:
list1 = ["apple", "banana", "cherry", "cherry"]
print(list1.count("cherry"))
Output:
2
21. Round Function: round()
The round() function returns floating-point numbers that are rounded to the specified number of decimals.
Round Function Example:
myvalue = round(3.333333, 2)
print(myvalue)
Output:
3.33
22. Max Function: max()
The maximum or max() function returns the greatest or highest value in an iterable.
Max Function Example:
mylist = max(1, 10, 5, 20, 15, 8)
print(mylist)
Output:
20
23. Min Function: min()
The minimum or min() function in Python returns the smallest value in a given iterable.
Min Function Example:
mylist = min(50, 30, 5, 25, 17, 21, 40)
print(mylist)
Output:
5
24. Absolute Value Function: abs()
The absolute value, or abs(), function returns the absolute value of the specified number.
Absolute Value Function Example:
x = abs(-7.25)
print(x)
Output:
7.25
25. Init Function: __init__()
The __init__() function is a special method that is called when an instance of a class is created. This method allows us to define properties and set initial values for them.
Init Function Example:
def __init__(self, name):
self.name = name
print(Person('John').name)
Output:
'John'
// This statement will print the string ‘John’ because the __init__() method sets the name property to ‘John’.
26. Ord Function: ord()
The ord() function returns the unicode value of a given character.
Ord Function Example:
example = ord("x")
print(example)
Output:
120
27. Get Attribute Function: getattr()
The get attribute or getattr() function returns the attribute value of a specified object.
Get Attribute Function Example:
class dogs:
name = "Yogi"
breed = "Schkipperke"
age = 8
x = getattr(dogs, 'breed')
print(x)
Output:
Schkipperke
28. Identification Function: id()
The identification or id() function in Python returns the unique identity value of a specified object.
Identification Function Example:
mylist = ('dog', 'cat', 'bird')
x = id(mylist)
print(x)
Output:
139713542134272
29. Super Function: super()
The super() function returns an object that is represented in the parent or base class. In Python, the super() function is used to gain access to methods of the parent class.
Super Function Example:
class Dog
def __init__(self, name):
self.name = name
print("Dog created!")
class YellowLab(Dog):
def __init__(self, name):
super().__init__(name)
print("Yellow Lab created!")
my_yellow_lab = YellowLab("Willie")
Output:
Dog created!
Yellow Lab created!
// This statement will print “Dog created!” and then “Yellow Lab created!” because the super() function returns the object in the parent class and the __init__() function is called on that object.
30. Type Function: type()
The type() function returns the class type of an object. This is useful for understanding the structure and content of a given object.
Type Function Example:
mylist = [1, 2, 3]
myvalue = type(mylist)
print(myvalue)
Output:
<class 'list'>
31. Dictionary Function: dict()
The dict() or dictionary function is used to create a dictionary. It takes any number of key-value pairs as arguments and creates a dictionary with those arguments >> Learn more about the Python dictionary function.
Dictionary Function Example:
d = dict(name="John", age=24)
print(d)
Output:
{'name': 'John', 'age': 24}
// This statement will print {'name': 'John', 'age': 24} because it creates a dictionary with two key-value pairs.
32. Locals Function: locals()
The locals() function in Python returns the dictionary for a local symbol table.
Locals Function Example:
mylist = locals()
print(mylist)
Output:
{'__name__': '__main__', '__doc__': None, '__package__': None, '__loader__': <class '_frozen_importlib.BuiltinImporter'>, '__spec__': None, '__annotations__': {}, '__builtins__': <module 'builtins' (built-in)>, 'mylist': {...}}
33. Translate Function: translate()
The translate() function is used to apply a dictionary of translations to a given string. It takes the dictionary and string as arguments and returns a string with all the characters in the string replaced by their translations.
Translate Function Example:
my_dict = {"a": "A", "b": "B"}
my_string = "ab"
translated_string = my_string.translate(my_dict)
print(translated_string)
Output:
"ab"
34. Globals Function: globals()
The globals() function returns a dictionary that includes all of the symbols and variables for the active program.
Globals Function Example:
print(globals())
Output:
{'__name__': '__main__', '__doc__': None, '__package__': None, '__loader__': <_frozen_importlib_external.SourcelessFileLoader object at 0x2b574e5380d0>, '__spec__': None, '__annotations__': {}, '__builtins__': <module 'builtins' (built-in)>, '__file__': './prog', '__cached__': None}
35. Update Function: update()
The update() function is used to add or update elements in a dictionary. It takes the key and value as arguments and updates the value of the given key if it exists, or adds the element to the dictionary if it doesn't.
Update Function Example:
my_dict = {"a": 1, "b": 2}
my_dict.update({"c": 3})
print(my_dict)
Output:
{"a": 1, "b": 2, "c": 3}
36. Map Function: map()
The map() function provides a specific function for each value in an iterable.
Map Function Example:
return x * 2
nums = (1, 4, 6, 2, 9)
map_nums = map(multiply_by_two, nums)
print(list(map_nums))
Output:
2, 8, 12, 4, 18
37. Date Function: date()
The date() function is a built-in Python module that provides functions for manipulating dates and times.
Date Function Example:
import date
date_1 = date(2020, 4, 8)
date_2 = date(2021, 4, 8)
difference = date_2 - date_1
print(difference.days)
Output:
365
38. Datetime Function: datetime()
The datetime() function provides classes that allow us to manipulate dates and times. This is useful if you need to calculate dates or display information in a certain date and time format.
Datetime Function Example:
import datetime x = datetime.datetime.now()
print(x)
Output:
2023-03-02 02:54:14.727281
39. Zip Function: zip()
The zip() function is used to combine two or more iterables into a single list of tuples. It takes the iterables as arguments and returns a list of tuples containing elements from all the iterables in order.
Zip Function Example:
list_1 = ["a", "b", "c"]
list_2 = ["1", "2", "3"]
list_3 = zip(list_1, list_2)
print(list(list_3))
Output:
(“a”, “1”), (“b”, “2”), (“c”, “3")
40. Read Function: read()
The read() function tells you how many bytes are found within a file.
Read Function Example:
x = open("yourfile.txt")
print(x.read())
Output:
Contents of your file
41. Dir Function: dir()
The dir() function is used to get a list of all the names defined in a Python module. It takes an optional argument which can be used to filter out certain names from the list.
Dir Function Example:
import math
print(dir(math))
Output:
['__doc__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'cbrt', 'ceil', 'comb', 'copysign', 'cos', 'cosh', 'degrees', 'dist', 'e', 'erf', 'erfc', 'exp', 'exp2', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'isqrt', 'lcm', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'nextafter', 'perm', 'pi', 'pow', 'prod', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc', 'ulp']
// This statement will print a list of all the names defined in the Python module math.
42. Modify Function: modify()
The modify() function modifies the value of a global variable from within a Python function. It takes the name of the global variable and new value as arguments and modifies its value within the function.
Modify Function Example:
x = 10
def modify():
global x
x += 5
modify()
print(x)
Output:
15
43. Scope
Scope is the area of code within which a Python function can be used. A function can only access variables defined in its own scope and any variables defined in a higher-level scope.
Scope Example:
def my_func():
x = 10
print(x)
my_func()
Output:
10
44. Combination Function: combination()
The Python combination function is used to generate combinations of elements from a given list. It takes the list of elements and a number as an argument and returns all possible combinations of the given length.
Combination Function Example:
from itertools
import combinations
my_list = ["a", "b", "c"]
comb = combinations(my_list, 2) for i in list(comb):
print(i)
Output:
(“a”, “b”), (“a”, “c”), (“b”, “c”)
// This statement will print (“a”, “b”), (“a”, “c”), (“b”, “c”) because it generates all combinations of length 2 in my_list.
45. Recursive Python Functions
A recursive function is a function that calls itself until a certain condition is met. It takes one or more arguments and returns the result of the recursive call once the condition is met.
Recursive Function Example:
def factorial(n):
if n == 0: // base case
return 1
else: // recursive case
return n * factorial(n-1)
Output:
// This function calculates the factorial of a number by calling itself with the value of n-1 until it reaches the base case (n = 0).
46. Argument Function: *args
The argument or args function is a special argument that allows us to pass an arbitrary number of arguments to a Python function. It is represented by an asterisk (*) and is commonly used in Python functions.
Args Function Example:
sum = 0
for arg in args:
sum += arg return sum
print(add_numbers(1, 2, 3))
Output:
6
// This statement will print 6 because it adds the numbers 1, 2, and 3 using the python function args.
How to Pass a Function as an Argument
Python functions can be passed as arguments to other Python functions. It takes a function name and its argument list as an argument and returns the result of the function.
Example:
def add(x, y):
return x + y
def do_something(func, a, b):
return func(a, b)
print(do_something(add, 1, 2))
Ouput:
3